

Introduction to Android

Contents (1)
What is Android? Some stats what the mobile world looks like.
Prepare for development. IDE setup & review, emulator vs real device.
How does an Android app look like? Packages, common components & platform tools
Deeper look into components - devs burden (libs), Services, Receivers, Content Providers


Contents (2)
How to test Android apps? Unit & Instrumented tests. Appium vs Robotium vs Selendroid
Best practices - Kotlin, RxJava, Dagger, MVP/MVVM/MVI/Clean architectures
Material design everything - a cool design theory introduced by Google

What is Android?


Linux Kernel
Display Driver
Camera Driver
WiFi Driver
Binder Driver
C++ Libraries & Android Runtime
OpenGL
SSL
WebKit
SQLite
Application Framework
Activity Manager
Window Manager
View System
Package Manager
Location Manager
Resource Manager
Android OS versions
:format(webp)/cdn.vox-cdn.com/uploads/chorus_image/image/45944284/Find_your_phone_with_Android_Wear.0.0.png)
Android Wear
/cdn.vox-cdn.com/uploads/chorus_image/image/51825123/android_auto_chevy.0.jpg)
Android Auto

Android TV
Android Things
Market Share

- 2 billion monthly active devices (2017)
- 3 500 000 apps

- 1.3 billion monthly active devices (2018)
- 2 200 000 apps



Android in time


2008
2015
2009
2012

Version codes
2.2 -2.3
5.0
7.0
6.0
4.0
4.4
API level
Latest is Android O released in 2017 - 8.0 (API 26-27). What does O mean?
9-10
21-22
24-25


- API level - mostly used in development
- Version codes - used in public especially on update/upgrade
OS Distribution



Data collected during a 7-day period ending on February 5, 2018.

developer.android.com

Guidance for:
- Available Android APIs
- App architecture
- IDE install & usage
- UI Design
- Sample apps


Android Studio

- Announced May 16, 2013
- Developed by JetBrains
- Based on IntelliJ IDEA
- 3 GB RAM min (1 GB for emulator)
- 2 GB free space at least
- Rich layout editor
- Android-specific refactoring & quick fixes
- Supports Android wear
- Many more features
Includes:

NO
RIP ADT
Let's check it out


Device vs Emulator

+ Mock location
+ Emulate performance
+ Mock network connection
+ Easily intercept network traffic using Charles
+ Fast deploy
+ Access apps internal folders that are usually hidden without root
- Eats at least 1 GB RAM
- Hard to test gestures
+ Test custom implementations like Samsung has
+ Doesn't eat RAM
+ Testing using gestures
+ Easy to replicate bugs that users already have
+ Allows push notifications test
- Slower deploy
- No access to other internal device folders unless rooted
- Thousands of different devices
- Expensive to buy phones
First Android app
How does it work?

User: Gmail (ID 1)
User: Messenger (ID 2)
Process: gmail
Virtual Machine 1
Virtual Machine 2
Process: messenger
Multi-user Linux system
The Activity component
Java
XML
class AppsActivity extends Activity {
void onCreate() {
setContentView(XML);
}
}
<?xml version="1.0" encoding="utf-8"?>
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"/>
+

Let's build a sample app


Questions time
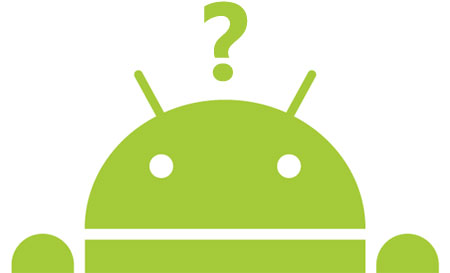
What happens to the XML setContentView is called?
Where are all view ids stored?
Where are instrumented tests placed?
App Details
Unique package name:
- com.google.android.gm - Gmail
- com.facebook.katana - Facebook
- com.instagram.android - Instagram
* visible in browser when app is selected in Google Play
Target API:
- OS version you are targeting from API level 14 - 27
- Phone & Tablet, Wear, TV, Auto or Things
Surest way to fix common errors

Click on the hyperlink and follow the installation process

Activity internals (1)


ViewGroup
View
Unique ID
Activity internals (2)


Instrumented tests
Unit tests
Images
XML for UI
Custom fonts
strings, colors, themes
Activity internals (3)

public final class R {
public static final class anim {
public static final int abc_fade_in=0x7f010000;
}
public static final class color {
public static final int black=0x7f06002a;
}
public static final class id {
public static final int tab_circle_view=0x7f0a06c5;
}
public static final class layout {
public static final int event_logs_seconds=0x7f11032c;
}
}
<com.example.mypackage>.R
with all resource ids - strings, layout, images
Activity Lifecycle
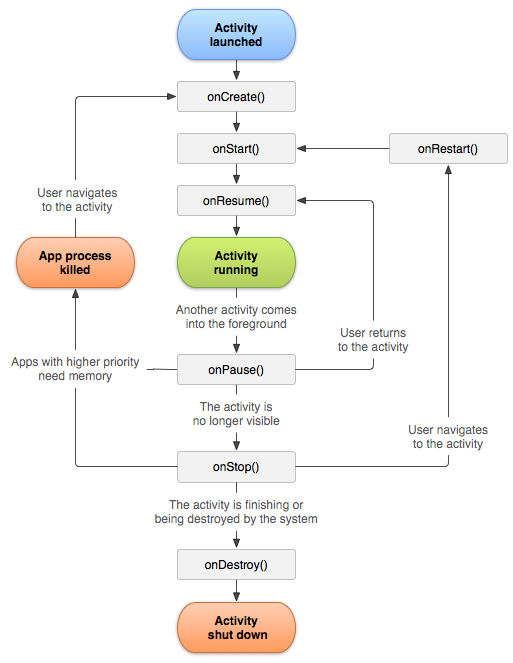


XML Components


Widgets
Layout

Text

Date

Images

Custom Views
Communicating between Activities

Intent i = new Intent(FirstActivity.this, SecondActivity.class);
i.putExtra("KEY", "VALUE");
FirstActivity.this.startActivity(i);
Why Intents are powerful?

Call other apps to:
- Capture photos
- Display photos
- Open URLs
- Play MP3s & videos
- Get current location
- etc

Let's try it!
AndroidManifest.xml
The manifest file describes essential information about your app to the Android build tools, the Android operating system, and Google Play.
What components the app has (like activities)?
What permissions are required from the user?
Which is the first activity that should be started?
Which is the icon displayed in the launcher?
Which is the style of the elements used throughout the app?
Does the app support right to left languages?

build system
Based on:

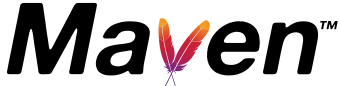
Works with dependencies from:
Flexibility at its finest:
- Build flavors
- Custom scripts
- Custom repositories
- Multi-project builds
- Shared build cache
- Better dependency resolution
Building an APK (1)


Convert Java classes to bytecode
1)
2)
Convert Oracle JVM bytecode to Dalvik

3)
Combine Dalvik bytecode & resources into APK
APK = Android Package Kit
Building an APK (2)

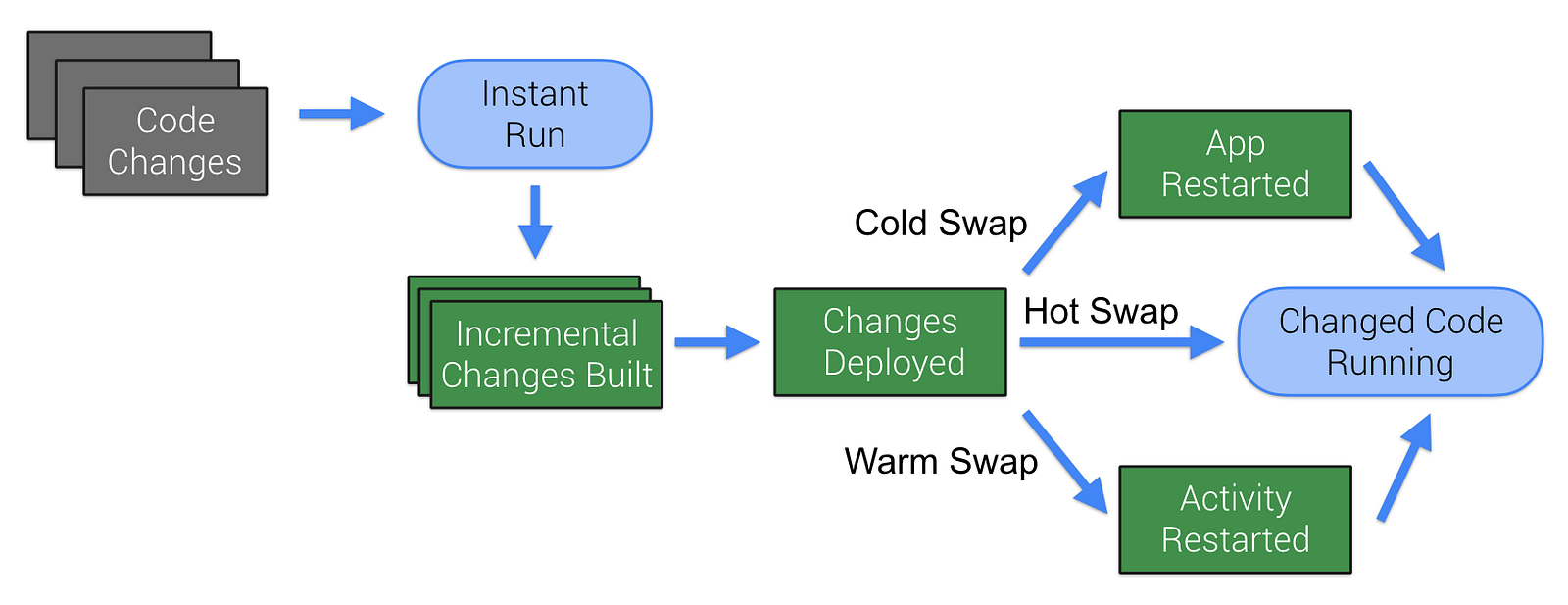
Instant Run
APK install process
Advanced Components
Broadcast Receivers

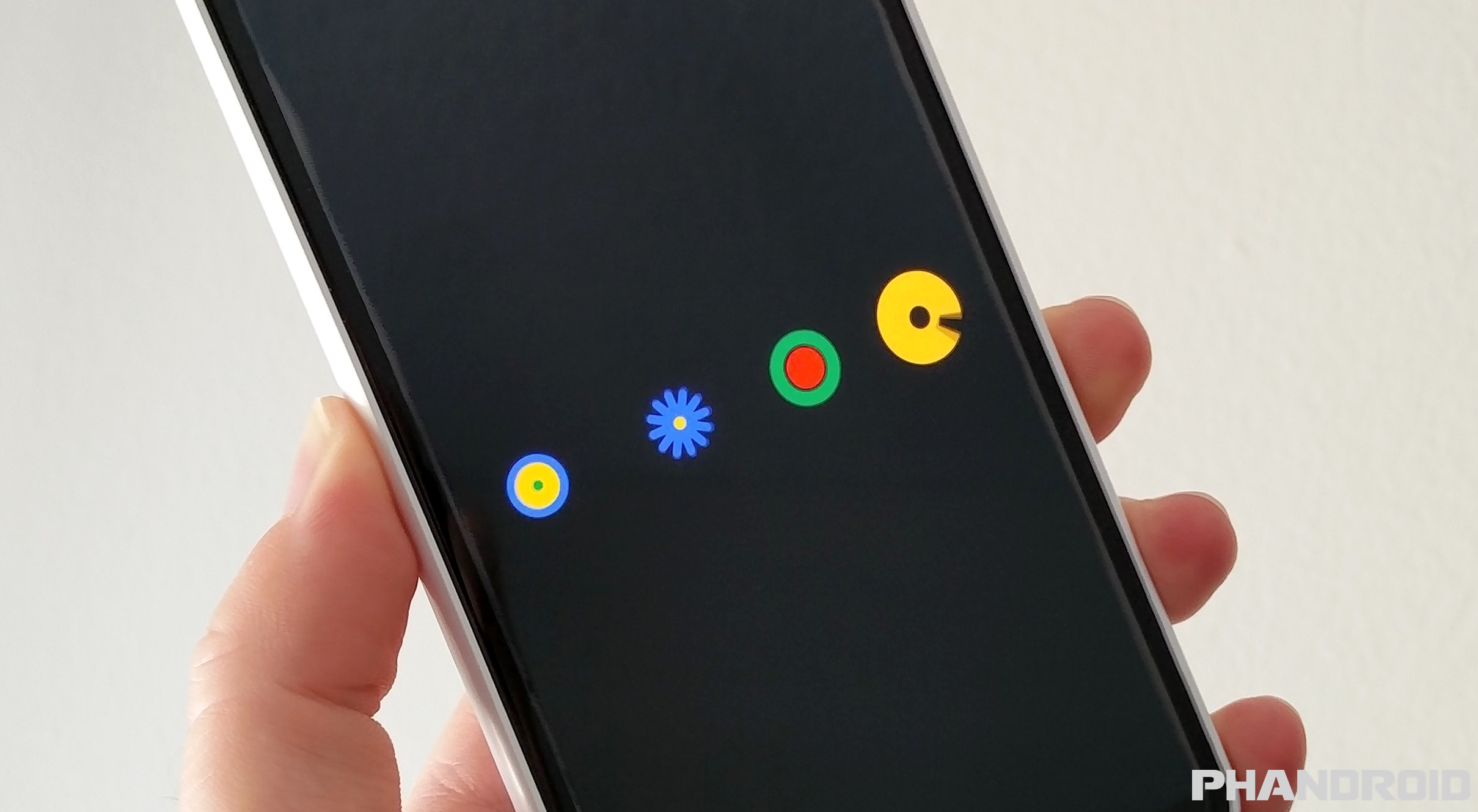


Event types
Services
Advanced Components
Component that can do long-running operations in the background, and does not provide a user interface

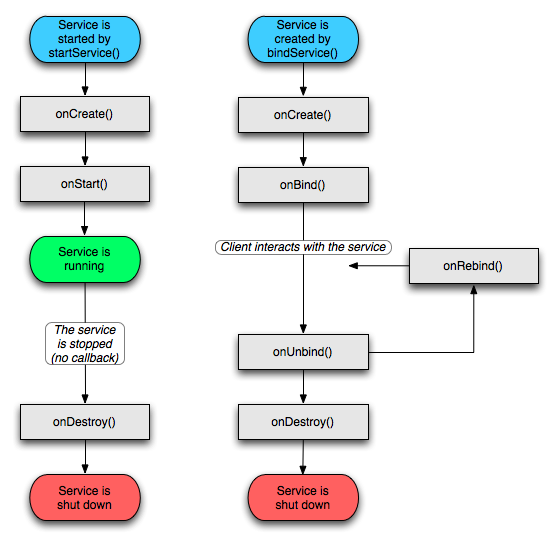
Content Providers
Advanced Components

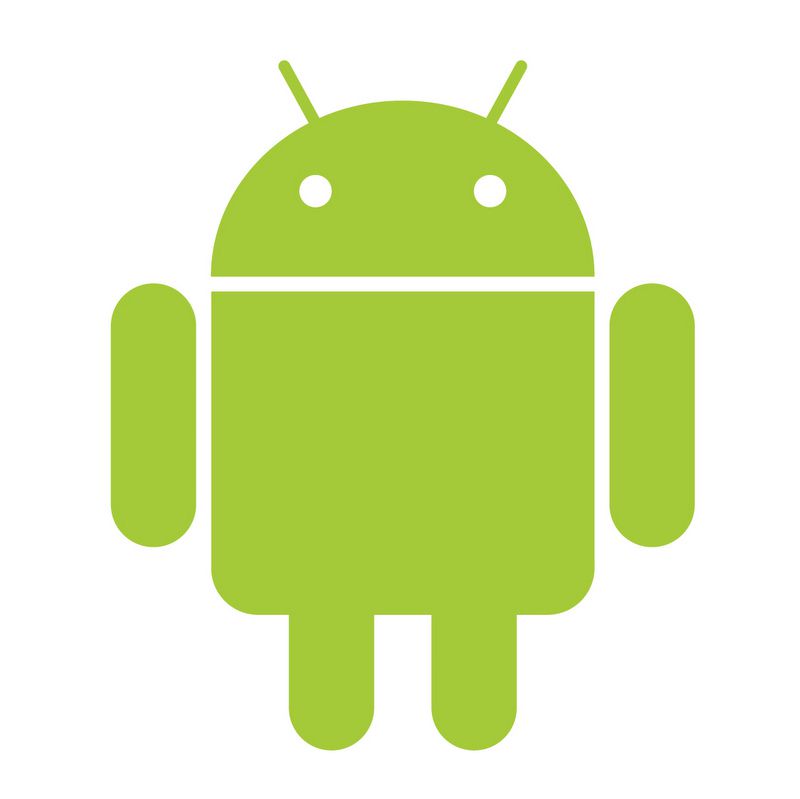
Find contacts containing "anna"
Content Provider
SQLite
Android Fragments
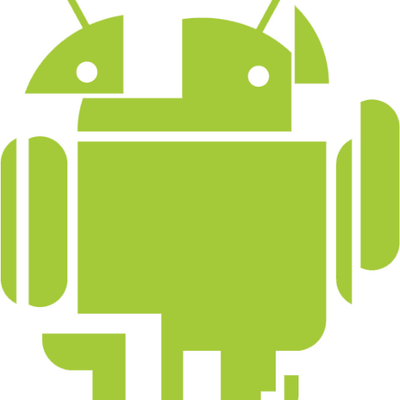



* scary things inside
Dev Burden
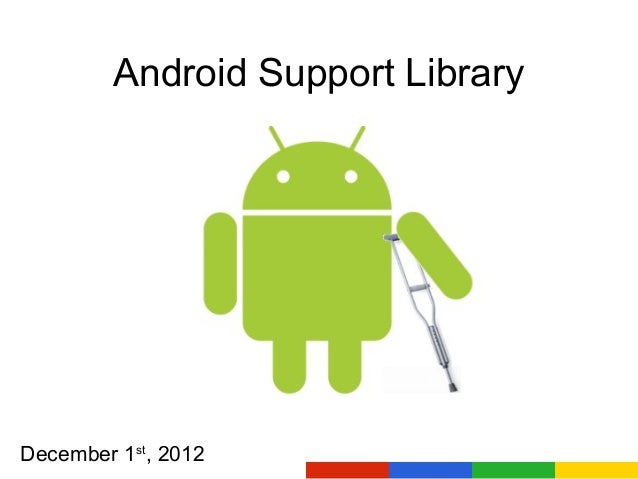
Accessibility
Fragments


android.support.design
(Material Design)
Drawer
CardView
Testing Android

Unit Tests
Instrumentation Tests
Cross-app functional UI testing

The Testing Pyramid
70
20
10
What folders Android has for unit / instrumentation (integration) tests?
Unit Tests

Mocks the Android environment as it uses too many static methods.
- does things inflating Views
- simulates different device conditions
- shadows - ways to test hardware dependencies
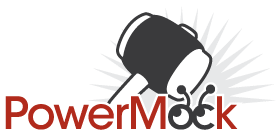
Mock all kinds of objects including static methods
Instrumentation Tests

- Waits for async jobs to finish
- Multiprocess testing
- Test WebViews
- Easily test lists

* Orchestator runs each test in a single instrumentation invocation
* If one test fails, it won't crash the app
Cross-app Functional UI Tests (1)

UI Automator + Viewer
<android-sdk>/tools/uiautomatorviewer
Performs interactions on user apps and system apps
mInstr = getInstrumentation()
mDevice = UiDevice.getInstance(mInstr);
mDevice.pressHome();
mLauncher = new UiSelector()
.description("Apps");
allAppsButton = mDevice.findObject(mLauncher);
// Perform a click on the button to load the launcher.
allAppsButton
.clickAndWaitForNewWindow();
Cross-app Functional UI Tests (2)



Last updated: 3 days ago
Last updated: a year ago
Last updated: beginning of 2018

- Uses UiAutomator / UiAutomator2 for API Level >= 17
- Uses Selendroid for API Level < 17
Best Practices


Or how to raise a good looking monser
class MyKotlinClass {
val name = "Georgi"
val surname = "Mirchev"
}
class MyJavaClass {
public String getName() {
return "Georgi";
}
public String getSurname() {
return "Mirchev";
}
}
println(bob?.department?.head?.name)
if (bob!= null && bob.department != null
&& bob.department.head != ull) {
// Same
}
class Person(val name: String,
var age: Int) {
// val - immutable
// var - mutable
}
class Person() {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
// Getter + Setter for age
}
Dagger & RxJava
Dependency Injection
Observer Pattern
App Component
- GSON parser
- ErrorHandler
User Component
- User provideCurrentUser()
Activity Component
- provideApiService(GsonParser, ErrorHandler, User)

MVP, MVVM, MVI, Clean Architectures

MVP
MVVM
MVI
Material Design
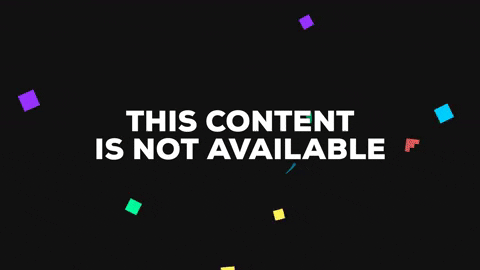

Developed by Google and introduced in 2014
Questions

- How many types of testing are available in Android?
- Which is one of the most important libraries for backwards compatibility?
- Which is the component that listens for system events like "Power Off"?
- APK file consists of ... and ...?
- Which is the BEST mobile OS?
Sources
- Stats - statista.com, macrumors.com, theverge.com, android-dev,
idc.com, Apple 1.3 billion - Version History - medium
- Android Studio - download, YouTube tutorials
- Building and running Android apps, Android build process
- Material Design
- Clean Architecture, MVP, MVVM, MVI
- Dagger 2 in 3 parts
- RxJava Vogella tutorial
- Kotlin
- Android Support Library
- Android Fragments
- Test-driven development, Fundamentals of testing Android
- Appium, Selendroid, Robotium, UiAutomator
- More on Dalvik VMs per app
Thank you!

Introduction to Android
By Georgi Mirchev
Introduction to Android
Introduction to how popular Android us, what are the basic components, how the build process works and some of the best practices popular at the moment.
- 1,519