Teach Me How To JavaScript
Glavin Wiechert
Basics
Browser
Backend
Basics
- History
- Language Syntax & Semantics
- Intro to Callback & Promise style programming
JavaScript History
- Developed by Brendan Eich working for Netscape Communication Corporation
- Called LiveScript when shipped in beta Netscape Navigator 2.0 in Sept 1995
- Renamed JavaScript in Netscape browser v2.0B3
- marketing ploy by Netscape
Node.js History
- Created and first published for Linux use in 2009
- Developed by Ryan Dahl
- Sponsored by Joyent
- npm introduced in 2011
- Create by Isaac Schlueter
- June 2011 - Microsoft partnered with Joyent to create native Windows version of Node.js
- released in July
- Jan 2012 - Isacc Schlueter became project manager
- Jan 2014 - Timothy J Fontaine is new project lead

Syntax & Semantics
- Derived from C
- Case sensitive
- `var` keyword to declare variables
- `=` Assignment Operator
- Literals are fixed values
- Statements are separated by semicolons
- Comments
- // are single-line
- /* multi-
line */
- Single-Threaded
- Event-driven
- Prototype-based (OOP)
- Dynamically typed
- Functions are first class
- supports passing functions as arguments and/or returning functions as values
Literals
- Number literals
- 3.14
- 1001
- 123e5
- String literals
- "John Doe"
- 'John Doe'
- Expression literals
- 5+6
- 5*10
Functions
- `function` keyword, followed by name, followed by () with code placed between {}
- parameters are comma separated between ()
- code within {} can optionally `return`
- invocation of function is `functionName(param1, param2, etc)`
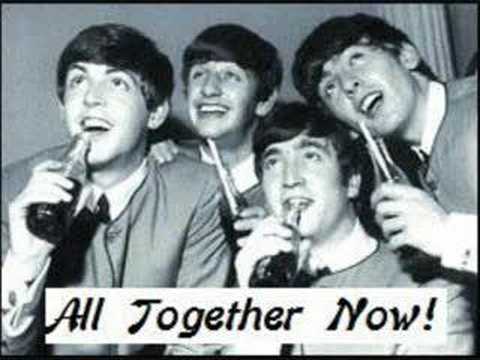
// This is a single-line comment
// Are you ready for more?
var myName = "Glavin"; // Note that you can also put comments after your code statements
var myAge = 20;
// ^ CamelCase is the common form to write variable names in JavaScript
/*
This is a multi-line comment.
This is very helpful,
especially when you like to talk a lot.
*/
function whoami(name, ageInYears) {
// Let's do some arithmetic.
var ageInDays = 365 * ageInYears; // Yes, this math is total BS. Let me have this one :)
// String concatenation is easy in JS!
// Notice how ageInDays is a Number and I can simply + it with a String.
return "My name is "+name+" and I am "+ageInDays+" days old!";
}
/* Invoke a function.
Arguments are myName and myAge.
Inside the function they are mapped arguments to local variables, parameters.
Argument -> Parameter
myName -> name
myAge -> ageInYears
*/
var result = whoami(myName, myAge);
console.log(result);
/*
`result` variable is equal to
"My name is Glavin and I am 7300 days old!"
*/
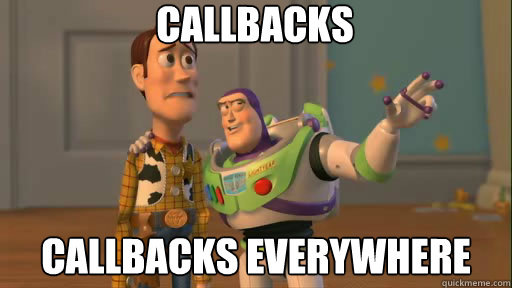
Callbacks vs Promises
Callback
Promise
fs.readFile('test.txt', function (err, data) {
if (err) return console.error(err);
db.insert({
fileName: 'test.txt',
fileContent: data
}, function (err, result) {
if (err) return console.error(err);
smtp.send({
to: 'test@test.com',
subject: 'test',
body: 'This is a test.'
}, function (err) {
if (err) return console.error(err);
console.log("Success email sent.");
});
});
});
fs.readFile('test.txt')
.then(function (data) {
return db.insert({
fileName: 'test.txt',
fileContent: data
});
})
.then(function () {
return smtp.send({
to: 'test@test.com',
subject: 'test',
body: 'This is a test.'
});
})
.then(function () {
console.log("Success email sent.");
}, function (err) {
console.error(err);
});
Callback & Promise style
- Read more at https://promisesaplus.com/
Browser
- Browser APIs
- Web-Workers (Multithreading)
- File API
- Drag'n'Drop
- WebGL
- jQuery
- Manipulating the DOM
- Making HTTP requests to APIs
- Popular Front-End Tools
- Design - Twitter Bootstrap
- Graphing - D3 (NVD3, C3)
- MVC Frameworks - Angular, Ember, Backbone, etc
Internet Explorer

To Web-Developers
Lesson 1:
Check Browser Support
Web-Workers
- 84.71% Browser Support
- Good for CPU intensive tasks that could block UI
- Event Listener Pattern
- Web worker calls `postMessage(message)` to pass back `message` to the main JS thread
- Main JS thread is listening with callback for `onmessage`
- Basic example code:
- Demo
File API
- 87.43% Browser Support
- Simple demo
Drag'n'Drop
- 66.22% Browser Support
- Demo
WebGL
- 74.64% Browser Support
- 9 More Mind-Blowing WebGL demos
- Three.js
jQuery
Let's talk about
the DOM, baby
jQuery and the DOM
- DOM = Document Object Model
- Without jQuery: document.getElementById(), document.getElementsByClassName(), etc
- Pass CSS selector to jQuery and easily get access to jQuery elements!
- jQuery elements, unlike DOMElement, have special features that can be used to make web-dev life easier
// Get the <button> element with the class 'continue'
// and change its HTML to 'Next Step...'
$( "button.continue" ).html( "Next Step..." );
// Show the #banner-message element that is hidden with `display:none`
// in its CSS when any button in `#button-container` is clicked.
var hiddenBox = $( "#banner-message" );
$( "#button-container button" ).on( "click", function( event ) {
hiddenBox.show();
});
Backend Communication
- AJAX requests
- "Asynchronous JavaScript + XML"
- JavaScript demo without jQuery
- jQuery.ajax()
Popular Front-End Tools
- Design
- Twitter Bootstrap
- Graphing
- D3 (NVD3, C3)
- MVC Frameworks
- Angular, Ember, Backbone, etc
Bootstrap
- "Bootstrap is the most popular HTML, CSS, and JS framework for developing responsive, mobile first projects on the web."
MVC Frameworks
- Angular
- Ember
- Backbone
- More!
D3
- Data-Driven Documents
- "Awesome"
- http://d3js.org/
- NVD3
- Reusable charts for D3
- http://nvd3.org/
- C3
- D3 based reusable chart library
- http://c3js.org/
Backend with Node.js
- Native Libraries
- path
- fs (filesystem)
- events (EventEmitter)
- Creating your own Node.js module
- Exposing methods and variables
- Publishing to npm
- Popular Third-Party Libraries
- npm & bower & grunt & yeoman
- Express
- Socket.io
- Node.js servers for the win!
- C++ + Node.js = <3
Event Loop
Why Node.js is Sexy


Event Loop
- Important for developers
- Do not use for Heavy Computational tasks
- Do not allow an exception to bubble up the event loop
- the Node.js instance to terminate (effectively crashing the program)
- Why The Hell Would I Use Node.js
- 1 million concurrent connections!
Working with Files
- Unlike Browser JS, Node.js as built-in support for interacting with File-System
// Require `fs` module
var fs = require("fs");
var fileName = "foo.txt";
// Reading from File System
fs.readFile(fileName, "utf8", function(error, data) {
console.log(data);
});
// Writing to File System
fs.writeFile("/tmp/test", "Hey there!", function(err) {
if(err) {
console.log(err);
} else {
console.log("The file was saved!");
}
});
// Watch file change events
fs.watch(fileName, {
persistent: true
}, function(event, filename) {
console.log(event + " event occurred on " + filename);
});
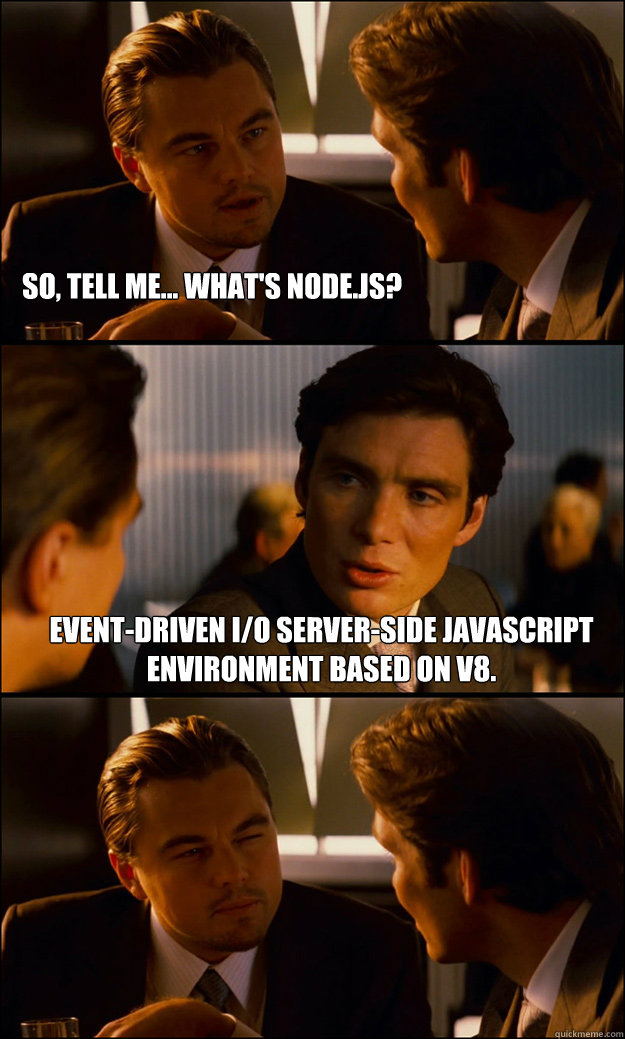
It's Coding Time!


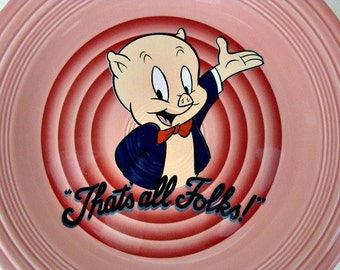
Thanks for watching!
- http://www.sitepoint.com/accessing-the-file-system-in-node-js/
- http://www.toptal.com/nodejs/why-the-hell-would-i-use-node-js
- https://github.com/joyent/node/tree/master/test/addons/hello-world
- http://blog.caustik.com/2012/08/19/node-js-w1m-concurrent-connections/
- http://www.sitepoint.com/accessing-the-file-system-in-node-js/
- http://www.slideshare.net/nsm.nikhil/writing-native-bindings-to-nodejs-in-c
TMHTJavaScript
By Glavin Wiechert
TMHTJavaScript
Teach Me How To JavaScript
- 1,483