Array
Copyright © 直通硅谷
http://www.zhitongguigu.com/
INCEPTION
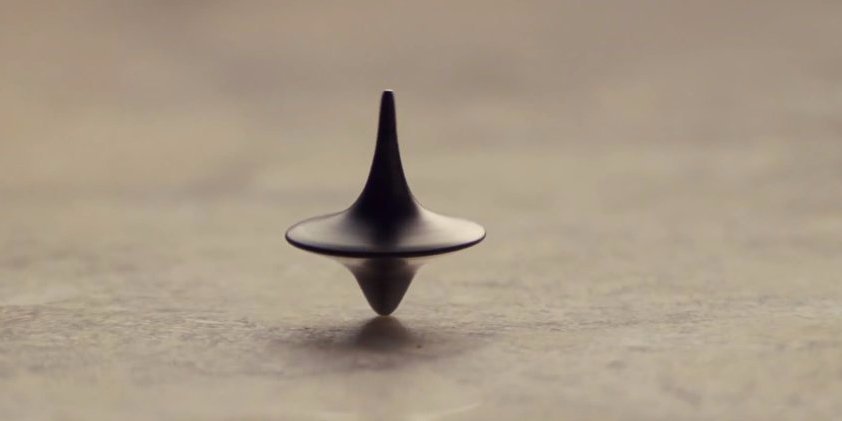
What is Array?
A data structure
consisting of a collection of
elements (values or variables),
each identified by at least one
array index or key.
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Array in Java?
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Just consider it as an Object
Array in Interview
- Continuous space
- Same type elements
- Identified by index
Linked List
Tree
String
Set
HashMap
Stack/Queue
Heap
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Graph
Array in Code
-
Java
- int[] a = new int[5];
- System.out.println(a.length); // a.length = 5
- C++
- int a[5];
- no way to get length.
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Array in Interview
- Continuous space
- addr(elem) = addr(head) + sizeof(elem) * index
- Same type elements
- int[] arr = new int[10];
- Identified by index
-
Accessing any element in the array, using CONSTANT time. O(1) == fast
-
Copyright © 直通硅谷
http://www.zhitongguigu.com/
What is Array?
Copyright © 直通硅谷
http://www.zhitongguigu.com/
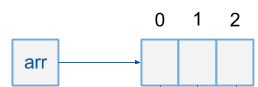
Single-dimension Array
int[] arr = new int[3];
Two-dimensional Array
int[ ][ ] arr = new int[3][ ];
arr[0] = new int[3];
arr[1] = new int[5];
arr[2] = new int[4];
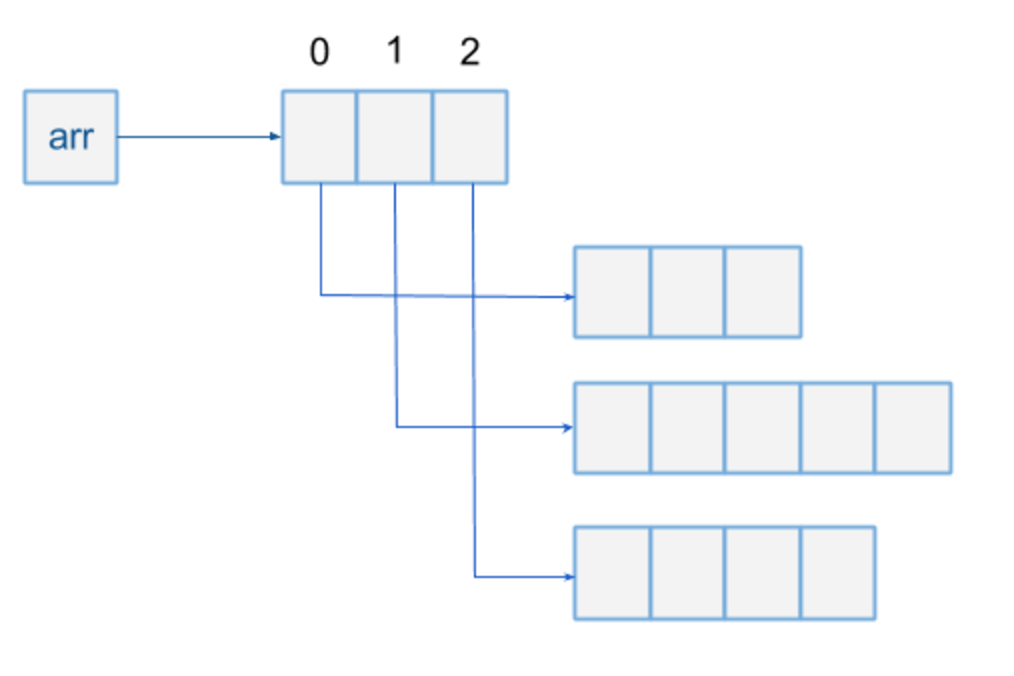
@4e25154f
@4e25154f
WHY use array?
To make code simple and clean
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Read 10 numbers and print out reversely
int a0, a1, a2, ..., a9;
a0 = 1;
a1 = 2;
a2 = 3;
...
System.out.println(a9);
System.out.println(a8);
System.out.println(a7);
...
int[] a = new int[10];
for (int i = 0; i < 10; i++) {
a[i] = i + 1;
}
for (int i = 9; i >= 0; i--) {
System.out.println(a[i]);
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Give me an array of ...
Try it out
Copyright © 直通硅谷
http://www.zhitongguigu.com/
public void moveZeroes(int[] nums) {
}
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.
For example,
given nums = [0, 1, 0, 3, 9], after calling your function,
nums should be [1, 3, 9, 0, 0].
Move Zeros
Try it out
Copyright © 直通硅谷
http://www.zhitongguigu.com/
index: [0, 1, 2, 3, 4]
input: [0, 1, 0, 3, 9]
output: [1, 3, 9, 0, 0]
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.
For example,
given nums = [0, 1, 0, 3, 9], after calling your function,
nums should be [1, 3, 9, 0, 0].
Move Zeros
Try it out
Copyright © 直通硅谷
http://www.zhitongguigu.com/
public void moveZeroes(int[] nums) {
if (nums == null || nums.length == 0) {
return;
}
int p = 0;
for (int i = 0; i < nums.length; i++) {
if (nums[i] != 0) {
nums[p] = nums[i];
p++;
}
}
for (; p < nums.length; p++) {
nums[p] = 0;
}
}
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.
For example,
given nums = [0, 1, 0, 3, 9], after calling your function,
nums should be [1, 3, 9, 0, 0].
Move Zeros
null & 0
Two pointers
Clean up
Try it out
Copyright © 直通硅谷
http://www.zhitongguigu.com/
public void moveZeroes(int[] nums) {
if (nums == null || nums.length == 0) {
return;
}
int p = 0;
for (int i = 0; i < nums.length; i++) {
if (nums[i] != 0) {
nums[p++] = nums[i];
}
}
while (p < nums.length) {
nums[p++] = 0;
}
}
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.
For example,
given nums = [0, 1, 0, 3, 9], after calling your function,
nums should be [1, 3, 9, 0, 0].
Move Zeros
null & 0
Two pointers
Clean up
Try it out
Copyright © 直通硅谷
http://www.zhitongguigu.com/
public void moveZeroes(int[] nums) {
if (nums == null || nums.length == 0) return;
int p = 0;
for (int i = 0; i < nums.length; i++)
if (nums[i] != 0) nums[p++] = nums[i];
while (p < nums.length) nums[p++] = 0;
}
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.
For example,
given nums = [0, 1, 0, 3, 9], after calling your function,
nums should be [1, 3, 9, 0, 0].
Move Zeros
Please Don't
Two-Pointer
Use TWO pointers, instead of one, to traverse the array in the same/opposite direction.
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Two-Pointer
-
Mostly, SORTED.
- in number order.
- in sequence order.
- linked list order.
- To find two numbers, or two set of numbers, which are subject to some conditions.
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java
Variables
&
Data Types
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
Copyright © 直通硅谷
http://www.zhitongguigu.com/
- 8 Primitive Types
- byte
- short
- int
- long
- float
- double
- char
- boolean
- Reference Types
- Object obj = new Object();
- int[] array = {1,2,3};
- Interface
- Enum
Java Variables & Data Types
Copyright © 直通硅谷
http://www.zhitongguigu.com/
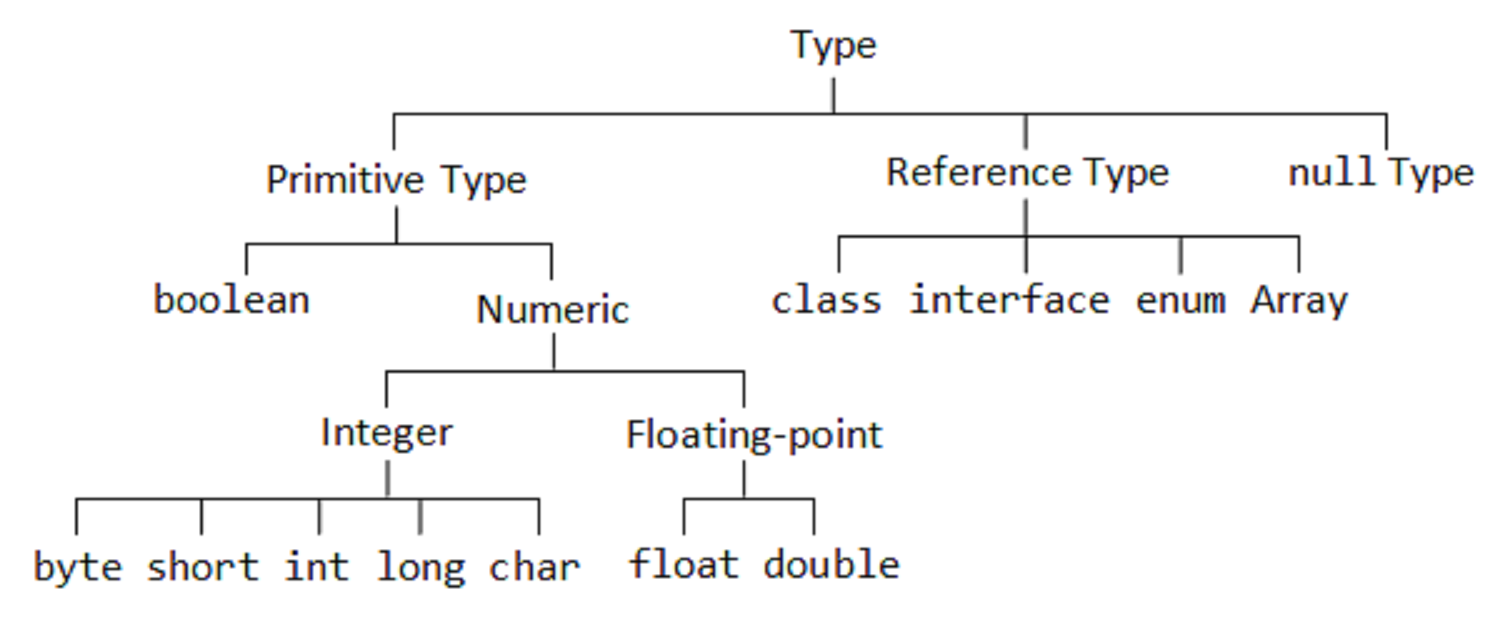
char
Copyright © 直通硅谷
http://www.zhitongguigu.com/
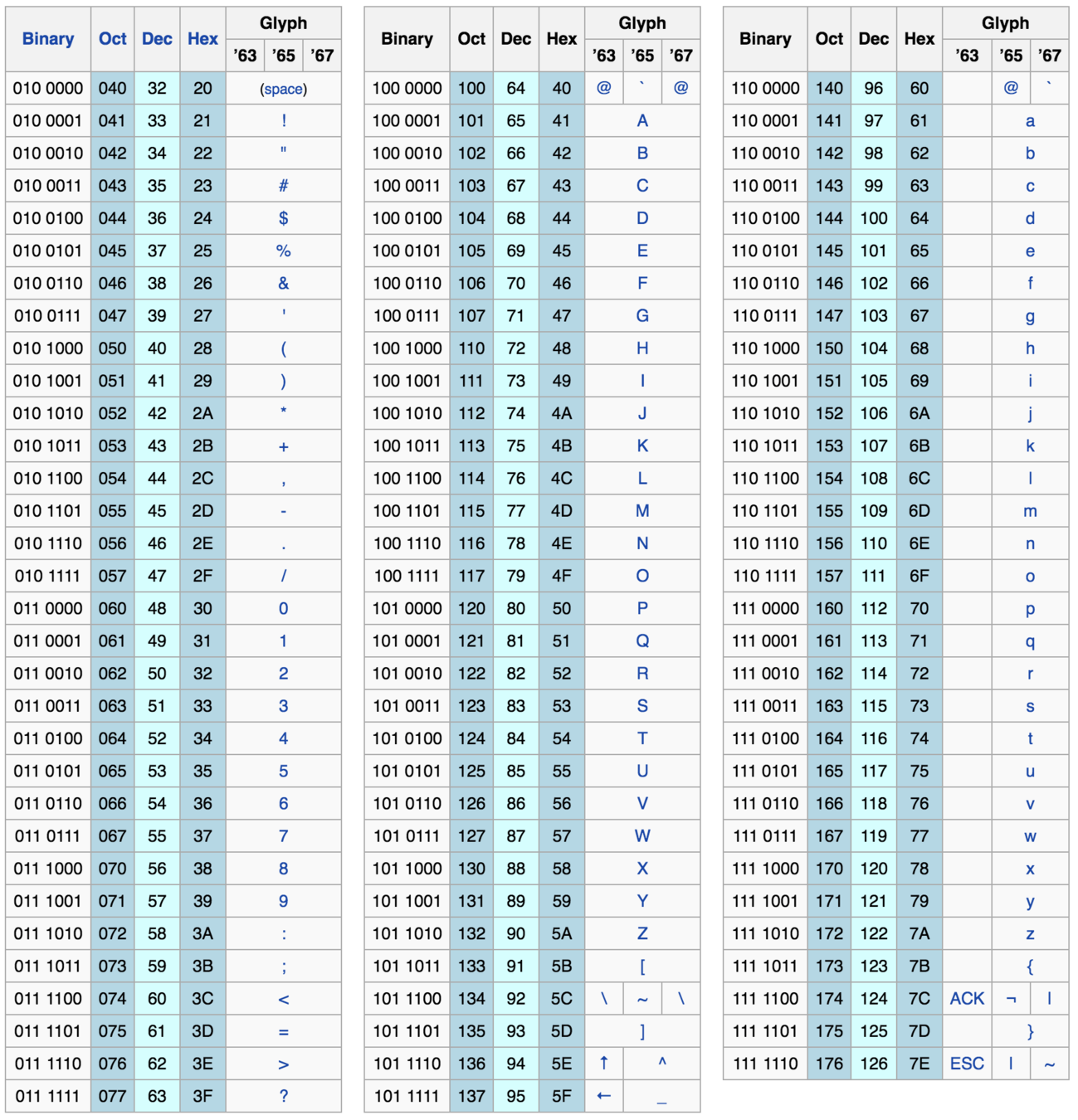
char c = 'a';
System.out.println(c);
System.out.println(c + 1);
48 = 32 + 16
65 = 64 + 1
97 = 64 + 32 + 1
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
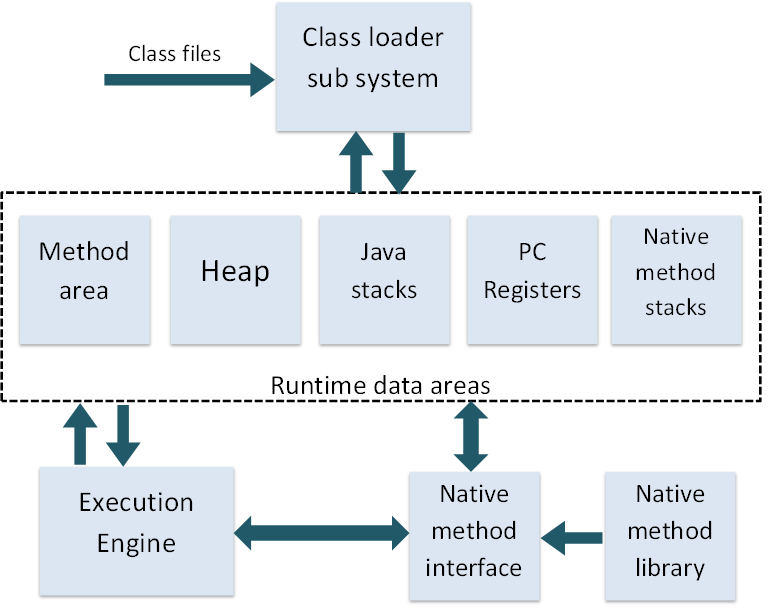
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
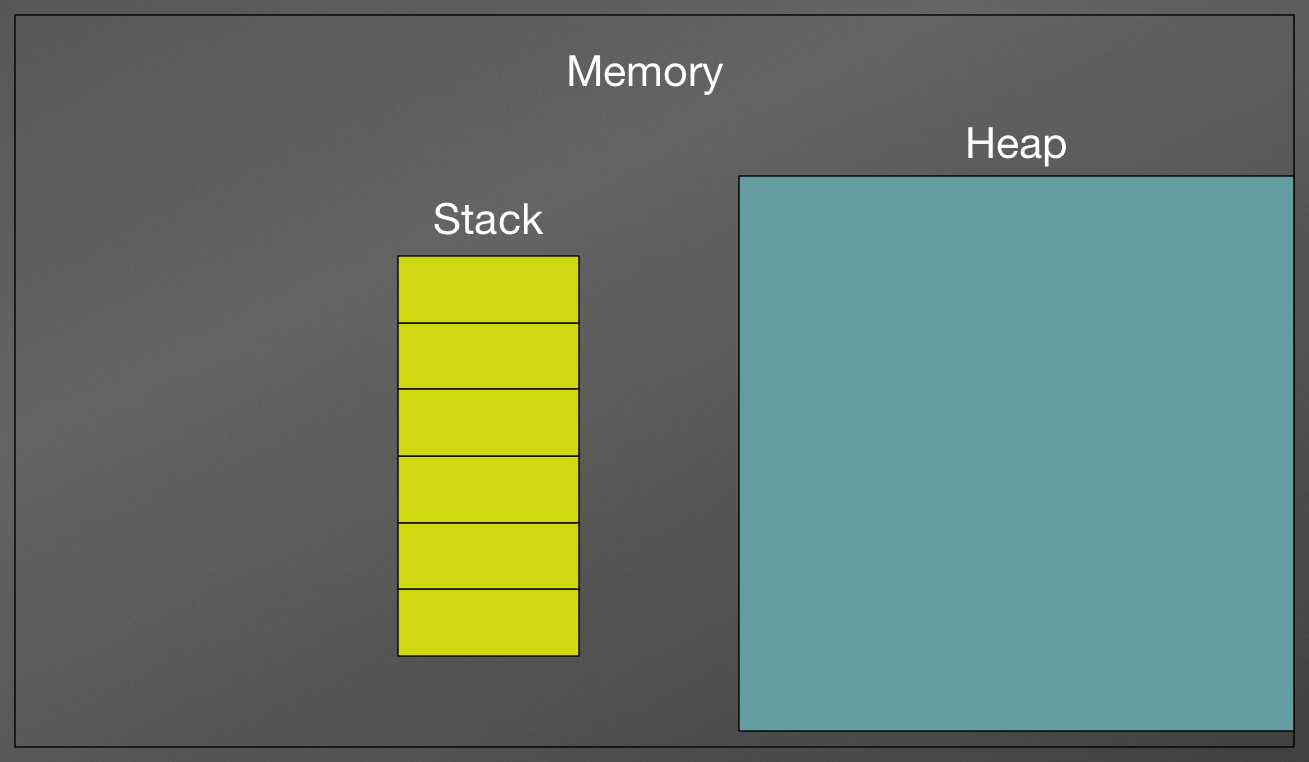
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
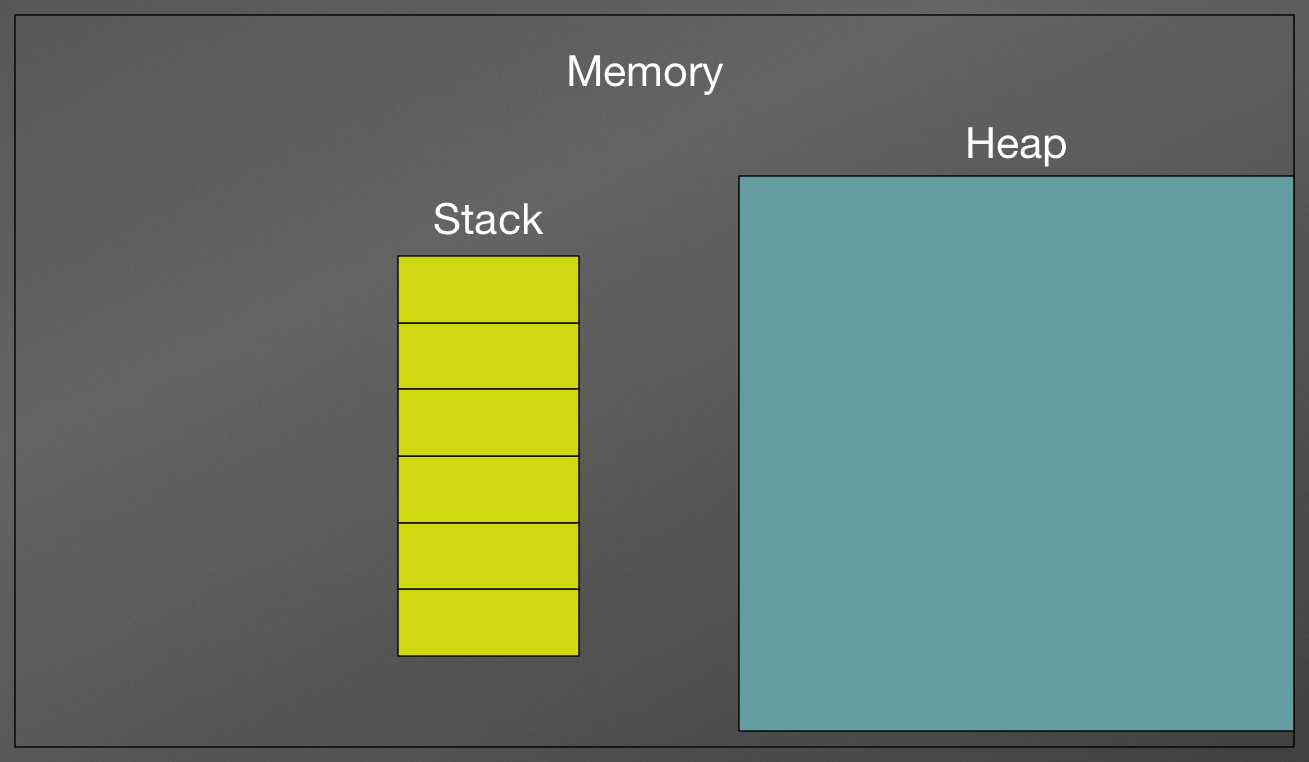
int a = 5;
int[] arr = new int[6];
Object obj = new Object();
a = 5
arr = @4e25154f
@4e25154f
obj
int b = a;
int[] arr2 = arr;
Object obj2 = obj;
@9d1c81a8
0 1 2 3 4 5
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
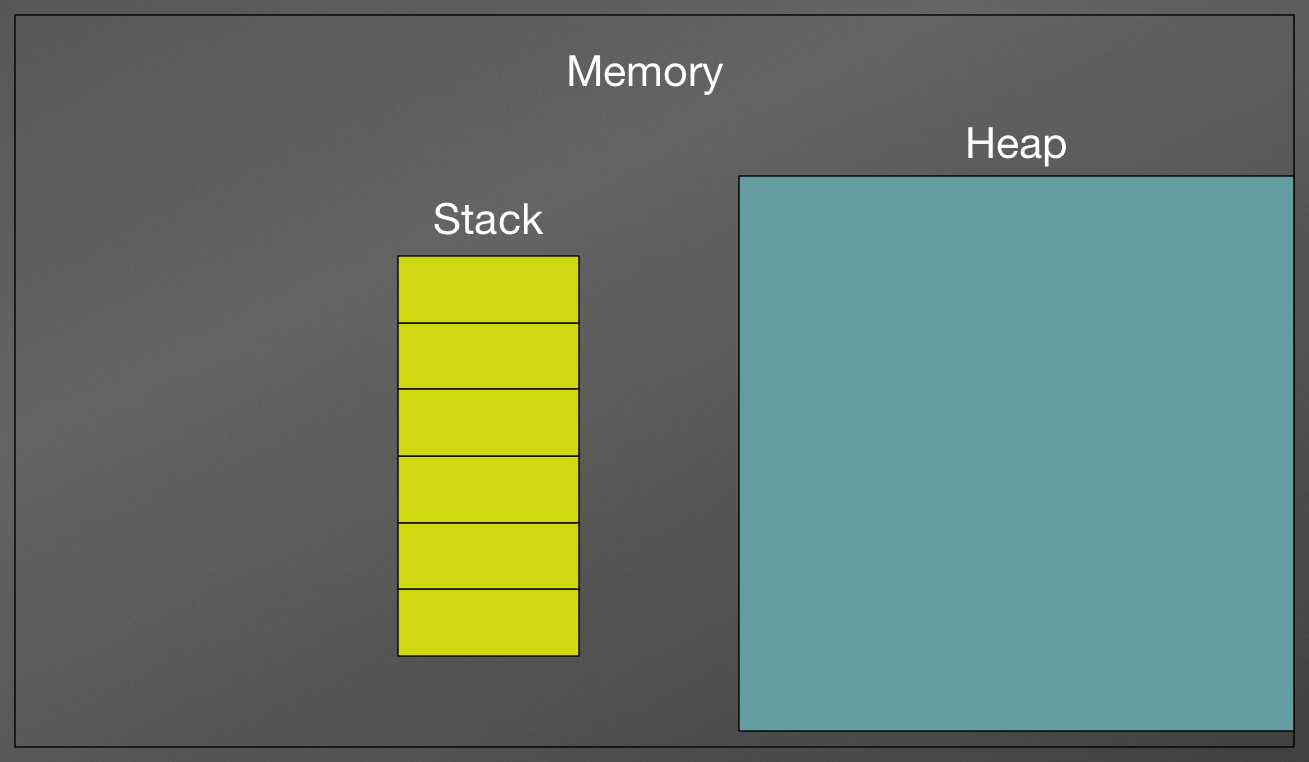
int a = 5;
int[] arr = new int[6];
Object obj = new Object();
a = 5
arr = @4e25154f
@4e25154f
obj = @9d1c81a8
@9d1c81a8
int b = a;
int[] arr2 = arr;
Object obj2 = obj;
b = 5
arr2 = @4e25154f
obj2 = @9d1c81a8
THINK
a = 10;
// what about b?
arr[0] = 8;
// what about arr1[1]
obj = null;
// what about obj2?
0 1 2 3 4 5
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
void caller() {
int a = 5;
int[] arr = new int[6];
Human hu = new Human(15);
change(a, arr, hu);
System.out.println(a);
System.out.println(arr[1]);
System.out.println(hu.age);
}
void change(int b, int[] a_, Human animal) {
b = 10;
a[1] = animal.age - 4;
animal.age = 22;
}
class Human {
int age;
public Human(int age) {
this.age = age;
}
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
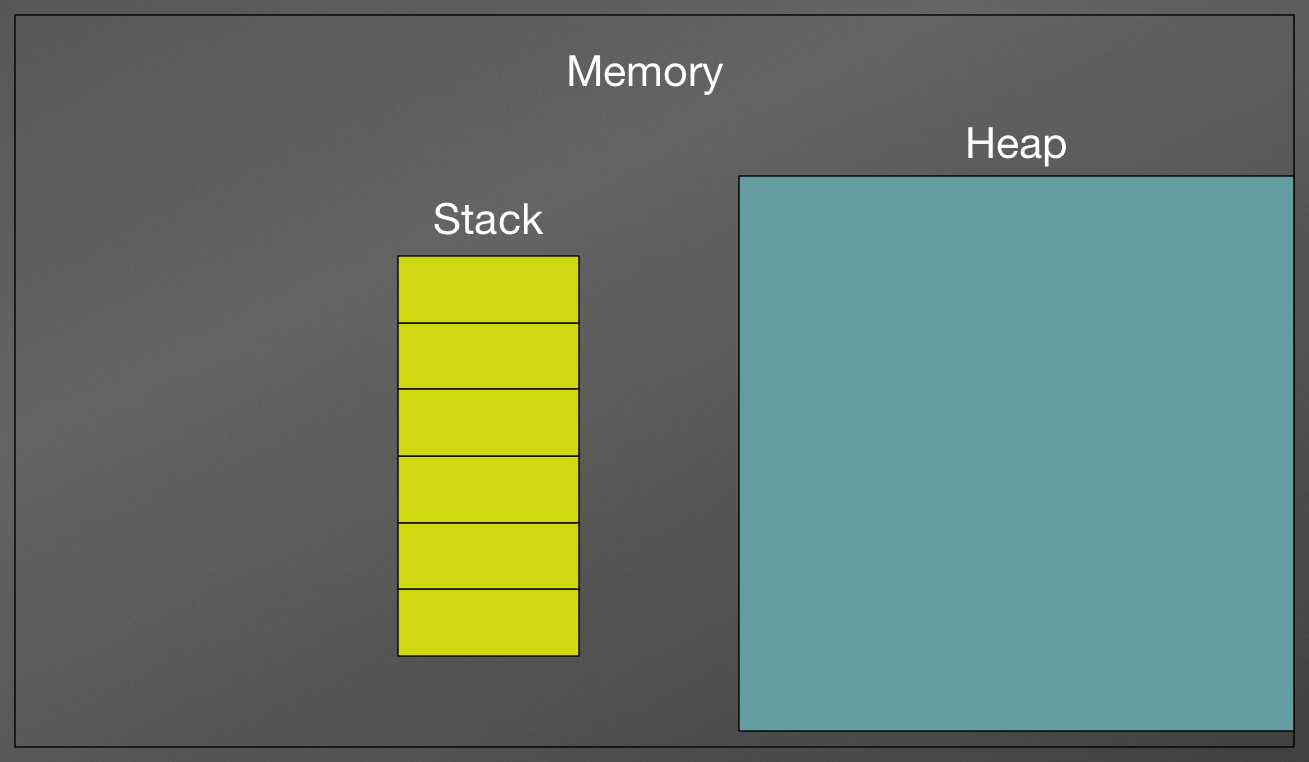
void caller() {
int a = 5;
int[] arr = new int[6];
Human hu = new Human(15);
change(a, arr, hu);
System.out.println(a);
System.out.println(arr[1]);
System.out.println(hu.age);
}
void change(int b, int[] a_, Human animal) {
b = 10;
a[1] = animal.age - 4;
animal.age = 22;
}
class Human {
int age;
public Human(int age) {
this.age = age;
}
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
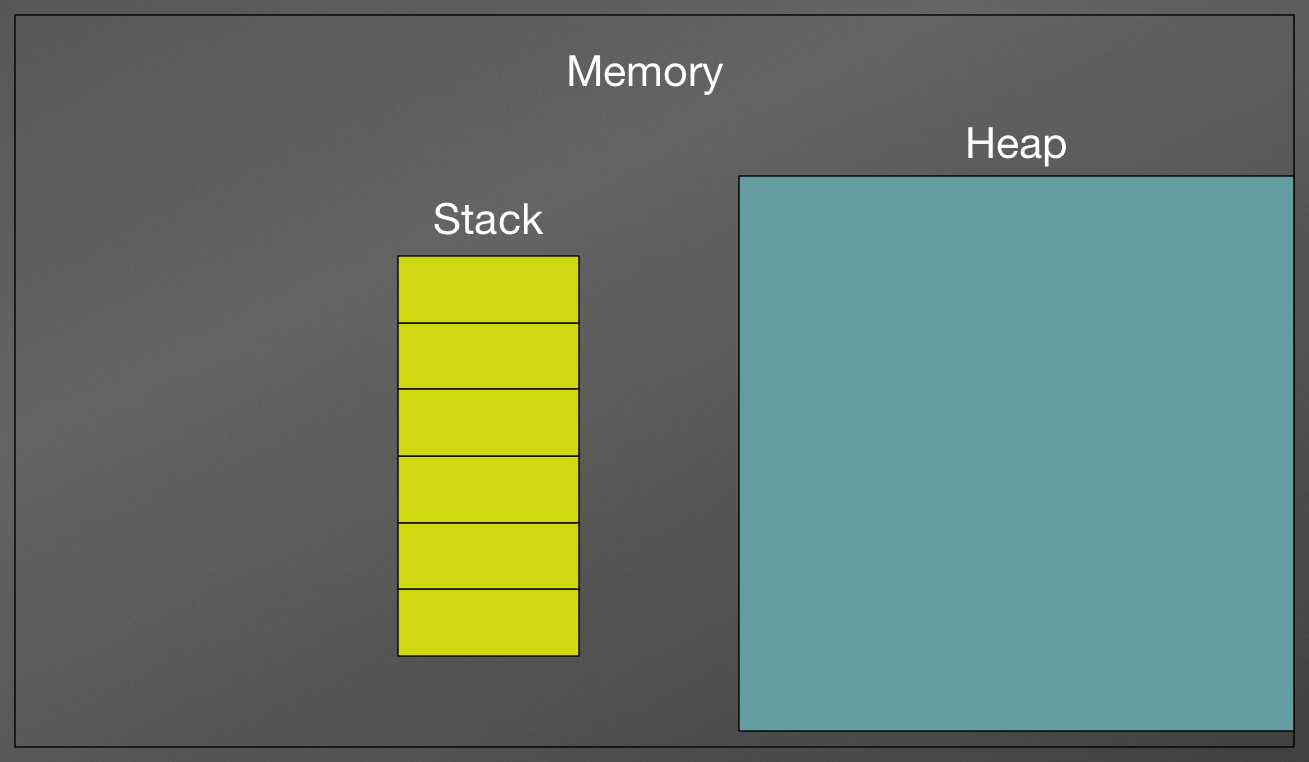
arr
a = 5
hu
a_
b
animal
age = 15
0
0
0
0
0
0
void caller() {
int a = 5;
int[] arr = new int[6];
Human hu = new Human(15);
change(a, arr, hu);
System.out.println(a);
System.out.println(arr[1]);
System.out.println(hu.age);
}
void change(int b, int[] a_, Human animal) {
b = 10;
a[1] = animal.age - 4;
animal.age = 22;
}
class Human {
int age;
public Human(int age) {
this.age = age;
}
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
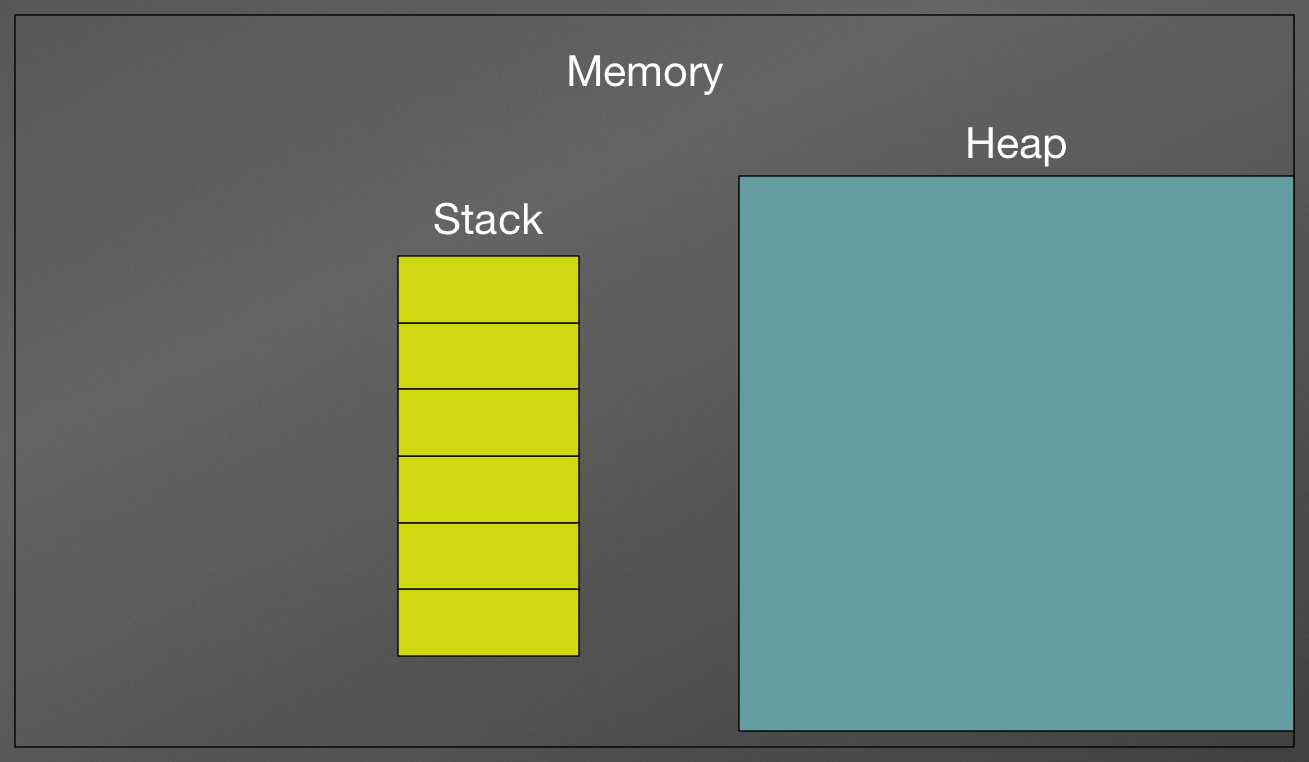
arr
a = 5
hu
a_
b = 10
animal
age = 22
11
0
0
0
0
0
Primitive Type
Passing the COPY of primitive value.
Reference Type
Passing the COPY of object address.
void caller() {
int a = 5;
int[] arr = new int[6];
Human hu = new Human(15);
change(a, arr, hu);
System.out.println(a);
System.out.println(arr[1]);
System.out.println(hu.age);
}
void change(int b, int[] a_, Human animal) {
b = 10;
a[1] = animal.age - 4;
animal.age = 22;
}
class Human {
int age;
public Human(int age) {
this.age = age;
}
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Variables & Data Types
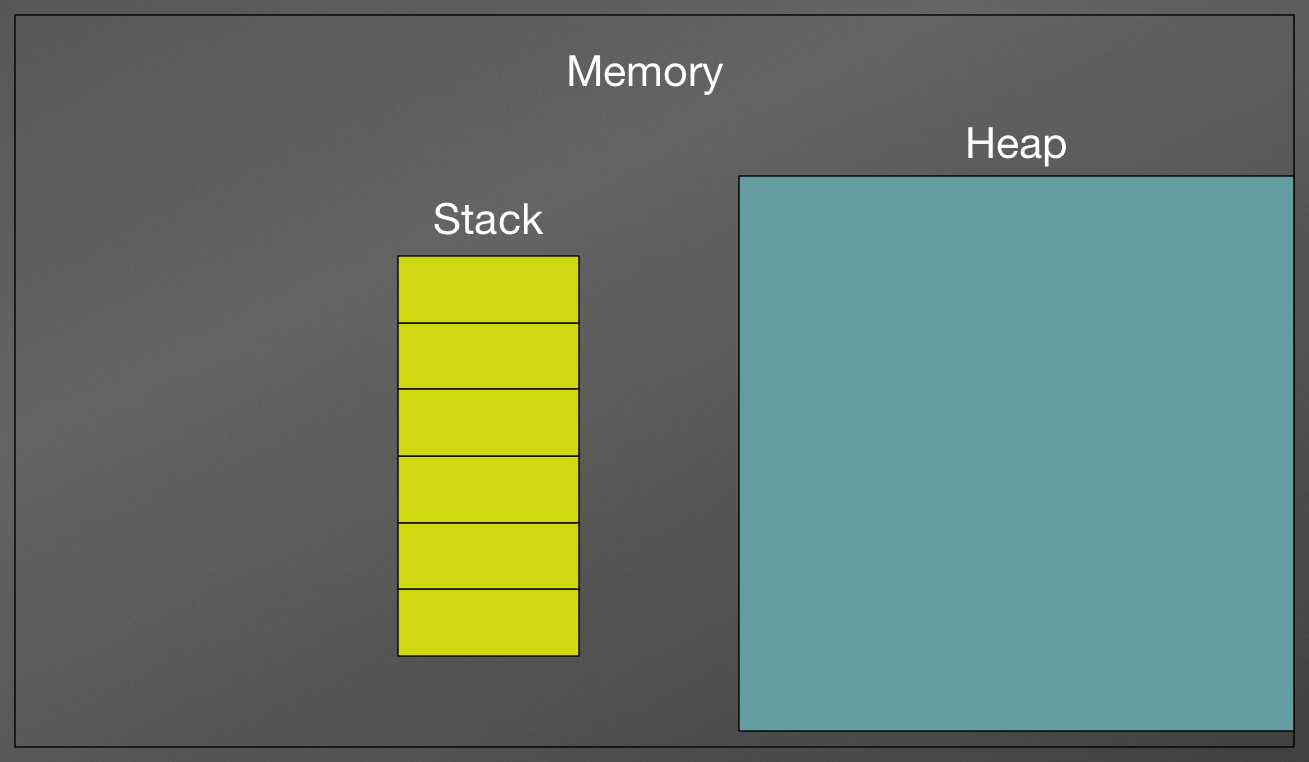
arr
a = 5
hu
age = 22
11
0
0
0
0
0
Primitive Type
Passing the COPY of primitive value.
Reference Type
Passing the COPY of object address.
void caller() {
int a = 5;
int[] arr = new int[6];
Human hu = new Human(15);
change(a, arr, hu);
System.out.println(a);
System.out.println(arr[1]);
System.out.println(hu.age);
}
void change(int b, int[] a_, Human animal) {
b = 10;
a[1] = animal.age - 4;
animal.age = 22;
}
class Human {
int age;
public Human(int age) {
this.age = age;
}
}
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Variable Comparison
-
Primitive Type
-
Compare the value of the variable.
- int a = 5;
- int b = 5; // a == b ? True
-
Compare the value of the variable.
-
Reference Type
-
Compare the address of the object.
- MyObject obj1 = new MyObject();
- MyObject obj2 = obj1; // obj1 == obj2 ? True
- obj2 = new MyObject(); // obj1 == obj2 ? False
-
Compare the address of the object.
Copyright © 直通硅谷
http://www.zhitongguigu.com/
String a = "abc";
String b = "abc";
System.out.println(a == b); // true
String c = new String("abc");
System.out.println(a == c); // false
System.out.println(a.equals(c)); // true
String d = new String("abc");
System.out.println(c == d); // false
System.out.println(d.equals(c)); // true
Variable Comparison
Copyright © 直通硅谷
http://www.zhitongguigu.com/
Java Data Type Summary
-
Primitive Type
-
Always means a literal value
- 5
- 's'
- true
-
Always means a literal value
-
Reference Type
-
Always means an address.
- Object obj = new Object();
- obj = obj2;
- int[] array = new int[3];
- myFunction(array);
-
Always means an address.
Address of the real object.
Let's talk about ArrayList
Array
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
ArrayList
Basic Operations
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Operation | Input | Output | Time |
---|---|---|---|
Get | <Index> | value | O(1) |
Set | <index, value> | void/ArrayList | O(1) |
Add | <[index], value> | void/ArrayList | O(n) |
Remove | <index/value> | void/ArrayList | O(n) |
Find | <value> | boolean/index | O(n) |
How to implement Java ArrayList
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
// TODO: implement this class.
public int get(int index) {
// TODO: implement this method.
}
public void set(int index, int value) {
// TODO: implement this method.
}
public void add(int value) {
// TODO: implement this method.
}
public void add(int index, int value) {
// TODO: implement this method.
}
public void remove(int index) {
// TODO: implement this method.
}
public void remove(int value) {
// TODO: implement this method.
}
}
ArrayList - Fields
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
private int capacity;
private int size;
private int[] data;
public ArrayList(int capacity_) {
capacity = capacity_;
size = 0;
data = new int[capacity];
}
}
ArrayList - Get
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public int get(int index) {
return data[index];
}
}
ArrayList - Get
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public int get(int index) {
if (index < 0 || index >= size) {
// throw Exception
}
return data[index];
}
}
ArrayList - Set
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public void set(int index, int value) {
if (index < 0 || index >= size) {
// throw Exception
}
data[index] = value;
}
}
ArrayList - Add
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public void add(int index, int value) {
if (index < 0 || index > size) {
// throw Exception
}
size++;
for (int i = size-1; i >= index+1; i--) {
data[i] = data[i-1];
}
data[index] = value;
}
}
ArrayList - Add
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public void add(int index, int value) {
if (index < 0 || index > size) {
// throw Exception
}
if (size == capacity) {
resize();
}
size++;
for (int i = size-1; i >= index+1; i--) {
data[i] = data[i-1];
}
data[index] = value;
}
private void resize() {
capacity *= 2;
int[] temp_data = new int[size];
for (int i = 0; i < size; i++) {
temp_data[i] = data[i];
}
data = new int[capacity];
for (int i = 0; i < size; i++) {
data[i] = temp_data[i];
}
}
}
ArrayList - Add
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public void add(int index, int value) {
if (index < 0 || index >= size) {
// throw Exception
}
if (size == capacity) {
resize();
}
size++;
for (int i = size-1; i >= index+1; i--) {
data[i] = data[i-1];
}
data[index] = value;
}
private void resize() {
capacity *= 2;
int[] new_data = new int[capacity];
for (int i = 0; i < size; i++) {
new_data[i] = data[i];
}
data = new_data;
}
}
ArrayList - Remove
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public class ArrayList{
public void remove(int index) {
if (index < 0 || index >= size) {
// throw Exception
}
size--;
for (int i = index; i < size; i++) {
data[i] = data[i+1];
}
}
}
ArrayList - Key Points
- Key data storage, data[]
- Initialize with capacity
- Alway Check Bound
- Resize
very very very important!!!
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Warmup Questions
- Sum of the array numbers
- Minimum element of the array
- Second minimum element of the array
- kth min/max elem of the array
- Swap every two elements in an array
- [1,2,3,4] -> [2,1,4,3]
-
Mirror k elems in a group for an array. k <= n
- [1,2,3,4,5,6], k = 4 -> [4,3,2,1,5,6]
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Two Sum
Given an array of integers (no duplicate), find two numbers such that they add up to a specific target number.
The function twoSum should return the two numbers such that they add up to the target, where number1 must be less than number2.
You may assume that each input would have exactly one solution.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: numbers={2, 7, 11, 15}, target=9
Output: {2, 7}
public int[] twoSum(int[] nums, int target) {
// TODO: implement this function.
}
Always ASK before you write code.
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Two Sum
Given an array of integers (no duplicate), find two numbers such that they add up to a specific target number.
The function twoSum should return the two numbers such that they add up to the target, where number1 must be less than number2.
You may assume that each input would have exactly one solution.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: numbers={2, 7, 11, 15}, target=9
Output: {2, 7}
public int[] twoSum(int[] nums, int target) {
// TODO: implement this function.
}
Solution-1
- Try one number nums[i], check all other numbers to see if their sum is expected.
- After trying all i, we can certainly find the pair.
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
// sudo code
for i from 0 to n - 1
for j from i + 1 to n - 1
if nums[i] + nums[j] == target
return
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution-1
public int[] twoSum(int[] numbers, int target) {
int[] result = new int[2];
if (numbers.length < 2) {
return result;
}
for (int i = 0; i < numbers.length-1; i++) {
for (int j = i+1; j < numbers.length; j++) {
if (numbers[i] + numbers[j] == target) {
if (numbers[i] < numbers[j]) {
result[0] = numbers[i];
result[1] = numbers[j];
} else {
result[0] = numbers[j];
result[1] = numbers[i];
}
return result;
}
}
}
return result;
}
Big O?
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution-1
public int[] twoSum(int[] nums, int target) {
int[] result = new int[2];
for (int i = 0; i < nums.length-1; i++) {
for (int j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] == target) {
return (nums[i] < nums[j]) ? new int[] {nums[i], nums[j]} : new int[] {nums[j], nums[i]};
}
}
}
return result;
}
Solution-1 Summary
- Direct, Easy
- Time Complexity, O(n^2).
- Inefficient
- Numbers : {1, 4, 20, 15, 8, 6, 3 }, Target = 10.
- First loop, 1 + 20 > 10
- Second loop, 4 + 20, NO NEED!
- Numbers : {1, 4, 20, 15, 8, 6, 3 }, Target = 10.
- Brute Force
- Sort
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Why sort helps?
- J > 10 => pass
- {1, 3, 4, 6, 8, 15, 20}, 10
- 1+20>10, 1+15>10
- for 3, start from 8.
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution-2
- Sort the array
- Try one number (i) from the beginning, check the other one (j) from the end.
- if sum == target, RESOLVED.
- if sum > target, j--.
- if sum < target, i++.
-
Numbers : {1, 4, 20, 15, 8, 6, 3 }, Target = 10.
- After sorting, {1, 3, 4, 6, 8, 15, 20}.
- There won't even be comparisons like 4+20 ? 10
- Time Complexity: O(nlogn) + O(n) = O(nlogn).
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution-2
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public int[] twoSum(int[] numbers, int target) {
int[] result = new int[2];
Arrays.sort(numbers);
int first = 0, second = numbers.length - 1;
while (first < second) {
if (numbers[first] + numbers[second] == target) {
result[0] = numbers[first];
result[1] = numbers[second];
return result;
}
if (numbers[first] + numbers[second] > target) {
second--;
}
if (numbers[first] + numbers[second] < target) {
first++;
}
}
return result;
}
Three Sum
Given an array of integers (no duplicate), find THREE numbers such that they add up to a specific target number.
The function threeSum should return the three numbers such that they add up to the target, where three numbers are in increasing order.
You may assume that each input would have exactly one solution.
Examples:
- COMMUNICATION!!!
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: numbers={-1, 0, 1, 2, -4}, target=0
Output: {-1, 0, 1}
public int[] threeSum(int[] nums, int target) {
// TODO: implement this function.
}
Solution
- Try first two numbers,
- check the third number in all other numbers to see if their sum are expected (target-num1-num2).
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution
-
Try first two numbers,check the third number in all other numbers to see if their sum are expected (target-num1-num2).
- Try the first number,
- check the other two in all other numbers to see if their sum are expected (target-num1).
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution
-
Try first two numbers,check the third number in all other numbers to see if their sum are expected (target-num1-num2).
- Try the first number,
- check the other two in all other numbers to see if their sum are expected (target-num1).
-
Time Complexity
- Sort + twoSum for n different first number.
- O(nlogn) + n*O(n) = O(n^2)
twoSum
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Solution
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public int[] threeSum(int[] nums, int target) {
// Sudo code
int[] result = new int[3];
if (nums.length < 3) {
return nums;
}
Arrays.sort(nums);
for (int i = 0; i < nums.length-2; i++) {
twoSum(nums[i+1..], target-nums[i]);
if (twoSum has results) {
result = {nums[i], (twoSum result)}
}
}
return result;
}
method {
m1();
m2();
}
Solution
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public int[] threeSum(int[] nums, int target) {
int[] result = new int[3];
if (nums.length < 3) {
return nums;
}
Arrays.sort(nums);
for (int i = 0; i < nums.length-2; i++) {
int first = i+1, second = nums.length-1, new_target = target-nums[i];
while (first < second) {
if (nums[first] + nums[second] == new_target) {
result[0] = nums[i];
result[1] = nums[first];
result[2] = nums[second];
return result;
}
if (nums[first] + nums[second] > new_target) {
second--;
}
if (nums[first] + nums[second] < new_target) {
first++;
}
}
}
return result;
}
Summary for k-Sum
- Sort.
- Try first number, use (k-1)-Sum.
- Time Complexity
- 2-Sum: O(nlogn) + O(n) = O(nlogn)
- 3-Sum: O(nlogn) + O(n^2) = O(n^2)
- 4-Sum: O(nlogn) + O(n^3) = O(n^3)
- k-Sum: O(nlogn) + O(n^(k-1)) = O(n^(k-1))
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Reverse Array
Given an array, reverse all the numbers in the array.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {1, 2, 3, 4, 5, 6, 7}
Output: {7, 6, 5, 4, 3, 2, 1}
public void reverseArray(int[] nums) {
// TODO: implement this function.
}
Inplace?
What is Inplace?
Reverse Array
Given an array, reverse all the numbers in the array.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {1, 2, 3, 4, 5, 6, 7}
Output: {7, 6, 5, 4, 3, 2, 1}
public void reverseArray(int[] nums) {
int first = 0, end = nums.length - 1;
while (first < end) {
swap(nums, first++, end--);
}
}
private void swap(int[] nums, int first, int second) {
int temp = nums[first];
nums[first] = nums[second];
nums[second] = temp;
}
Swap without third var?
Reverse Array
Given an array, reverse all the numbers in the array.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {1, 2, 3, 4, 5, 6, 7}
Output: {7, 6, 5, 4, 3, 2, 1}
public void reverseArray(int[] nums) {
// TODO: implement this function.
int first = 0, end = nums.length - 1;
while (first < end) {
swap(nums, first++, end--);
}
}
private void swap(int[] nums, int first, int second) {
int temp = nums[first];
nums[first] = nums[second];
nums[second] = temp;
}
Follow up:
- Reverse Number (1234 -> 4321)
-
Palindrome Number/String
- ('abcd' -> false; 343 -> true)
- Odd Even Sort, Pivot Sort
Odd Even Sort
Given an array of integers, sort them so that all odd integers come before even integers.
The order of elements can be changed. The order of sorted odd numbers and even numbers doesn't matter.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 3, 5, 2, 1, 11, 0, 8, 6, 9}
Output: {9, 3, 5, 11, 1, 2, 0, 8 , 6, 4}
public void oddEvenSort(int[] nums) {
// TODO: implement this function. After sorting,
// nums should start with odd numbers and then
// even numbers.
}
Odd Even Sort
Given an array of integers, sort them so that all odd integers come before even integers.
The order of elements can be changed. The order of sorted odd numbers and even numbers doesn't matter.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 3, 5, 2, 1, 11, 0, 8, 6, 9}
Output: {9, 3, 5, 11, 1, 2, 0, 8 , 6, 4}
public void oddEvenSort(int[] nums) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (nums[first] % 2 == 1) {
first++;
}
while (nums[second] % 2 == 0) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
}
Odd Even Sort
Given an array of integers, sort them so that all odd integers come before even integers.
The order of elements can be changed. The order of sorted odd numbers and even numbers doesn't matter.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 3, 5, 2, 1, 11, 0, 8, 6, 9}
Output: {9, 3, 5, 11, 1, 2, 0, 8 , 6, 4}
public void oddEvenSort(int[] nums) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] % 2 == 1) {
first++;
}
while (first < second && nums[second] % 2 == 0) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
}
No inplace
Traverse 1 time
Pivot Sort
Given an array of integers and a target number, sort them so that all numbers that are smaller than the target always come before the numbers that are larger than the target. The order of elements can be changed.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 9, 5, 2, 1, 11, 0, 8, 6, 3}, 7
Output: {4, 3, 5, 2, 1, 6, 0, 8, 11, 9}
public void pivotSort(int[] nums, int target) {
// TODO: implement this function.
}
Pivot Sort
Given an array of integers and a target number, sort them so that all numbers that are smaller than the target always come before the numbers that are larger than the target. The order of elements can be changed.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 9, 5, 2, 1, 11, 0, 8, 6, 3}, 7
Output: {4, 3, 5, 2, 1, 6, 0, 8, 11, 9}
public static void pivotSort(int[] nums, int target) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] <= target) {
first++;
}
while (first < second && nums[second] > target) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
}
public static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
Stability
Pivot Sort
Given an array of integers and a target number, sort them so that all numbers that are smaller than the target always come before the numbers that are larger than the target. The order of elements can be changed.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {4, 9, 5, 2, 1, 11, 0, 8, 6, 3}, 7
Output: {4, 3, 5, 2, 1, 6, 0, 8, 11, 9}
public static void pivotSort(int[] nums, int target) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] <= target) {
first++;
}
while (first < second && nums[second] > target) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
}
public static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
QuickSort
[Homewrok]
Follow up
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
// TODO: implement this function. After removing,
// nums should contain only instances which
// is not equal to val (from 0 to new length).
}
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] != val) {
first++;
}
while (first < second && nums[second] == val) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
return first;
}
[3, 2, 2, 3]
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] != val) {
first++;
}
while (first < second && nums[second] == val) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
return first;
}
[2, 2, 3, 3]
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
if (nums.length == 0) {
return 0;
}
int first = 0, second = nums.length - 1;
while (first < second) {
while (first < second && nums[first] != val) {
first++;
}
while (first < second && nums[second] == val) {
second--;
}
if (first < second) {
swap(nums, first++, second--);
}
}
return nums[first] != val ? first+1 : first;
}
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
int index = 0, len = nums.length;
// len is the valid length of remaining array.
while (index < len) {
if (nums[index] == val) {
len--; // remove one element.
nums[index] = nums[len]; // Keep the possible valid element.
} else {
index++;
}
}
return len;
}
Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.
The order of elements can be changed. It doesn't matter what you leave beyond the new length.
Example:
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Input: {10, 9, 5, 3, 9, 9, 8, 6, 7}, 9
Output: {10, 5, 3, 8, 6, 7, X, X, X}, 6
public int removeElement(int[] nums, int val) {
int index = 0, len = nums.length;
while (index < len) {
nums[index] = nums[index] == val ? nums[--len] : nums[index++];
}
return len;
}
Merge Two Sorted Array
Given two sorted arrays of integer, both with increasing order. Please merge them into one sorted array, with increasing order.
Examples:
Input: {1, 3, 5}, {2, 4, 6}
Output: {1, 2, 3, 4, 5, 6}
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Merge Two Sorted Array
Given two sorted arrays of integer, both with increasing order. Please merge them into one sorted array, with increasing order.
Examples:
Input: {1, 3, 5}, {2, 4, 6}
Output: {1, 2, 3, 4, 5, 6}
Solution:
Iterate two arrays at the same time, and always pick the smaller element from two numbers to put into the result array.
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
public int[] removeElement(int[] arr1, int[] arr2) {
int[] result = new int[arr1.length + arr2.length];
int index = 0, index1 = 0, index2 = 0;
while (index1 < arr1.length && index2 < arr2.length) {
if (arr1[index1] < arr2[index2]) {
result[index++] = arr1[index1++];
} else {
result[index++] = arr2[index2++];
}
}
for (int i = index1; i < arr1.length; i++) {
result[index++] = arr1[i];
}
for (int i = index2; i < arr2.length; i++) {
result[index++] = arr2[i];
}
}
Merge Two Sorted Array
Given two sorted arrays of integer, both with increasing order. Please merge them into one sorted array, with increasing order.
Examples:
Input: {1, 3, 5}, {2, 4, 6}
Output: {1, 2, 3, 4, 5, 6}
Follow up: Homework
- Merge Two Sorted Linked List
- Merge K Sorted Array
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Homework
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Two Sum - follow up
Given an array of integers (no duplicate), find two numbers such that they add up to a specific target number.
The function twoSum should return the two numbers such that they add up to the target, where number1 must be less than number2.
You may assume that each input would have exactly one solution.
Java:
public ArrayList<ArrayList<Integer>> twoSum(int[] nums, int target);
C++:
vector<vector<int>> twoSum(vector<int>& nums, int target);
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Partition Array
Given an array nums of integers and an int k, partition the array (i.e move the elements in "nums") such that:
- All elements < k are moved to the left
- All elements >= k are moved to the right
Return the partitioning index, i.e the first index i nums[i] >= k.
Java:
public int partitionArray(int[] nums, int k);
C++:
int partitionArray(vector<int> nums, int k);
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
Optional Homework
- Copyright © 直通硅谷
- http://www.zhitongguigu.com/
[GoValley-201612] Array, ArrayList
By govalley201612
[GoValley-201612] Array, ArrayList
Week 1 Lec 1&2
- 1,192