Currying and Decorator examples
by: Grecia

What is CurrYing
A curried function is a function that takes multiple arguments one at a time.
what is closure
A closure gives you access to an outer function’s scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures
https://medium.com/javascript-scene/curry-and-function-composition-2c208d774983

this is a closure
this function refers to the closure above
// Normal Function
function sum(a,b,c){
return a+b+c
}
//Function with clousure
function sum(a){
return function(b){
return function(c){
return a+b+c
}
}
}
//Function with clousure with arrow function
let currySum = (a)=>(b)=>(c)=> a+b+c;
// Normal console.log of a function
console.log(sum(1,2,3)) // 6
// console.log of a Function with a clousure
let sumWithClousure= currySum(1)(2)(3)
console.log(sumWithClousure)
function discount(discount, price) {
return discount*price
}
const discount10per = discount(0.1,500);
const discount20per = discount(0.2,500);
const discount30per = discount(0.3,500);
const discount40per = discount(0.4,500);
const discount50per = discount(0.5,500);
console.log(discount10per)
console.log(discount20per)
console.log(discount30per)
console.log(discount40per)
console.log(discount50per)
function discount(discount){
return function(price){
return price*discount
}
}
let discount10per= discount(0.1)
let discount20per= discount(0.2)
let discount30per= discount(0.3)
let discount40per= discount(0.4)
let discount50per= discount(0.5)
console.log(discount10per(500))
console.log(discount20per(500))
console.log(discount30per(500))
console.log(discount40per(500))
console.log(discount50per(500))
CurRyng and inheritance
const createPerson = (type)=>{
let person={
name:'Person',
age: 0,
isWoman: true,
type:type
};
return (name, age , isWoman)=>{
person.name = name || person.name
person.age = age || person.age
person.isWoman = isWoman
return person
}
}
let createaMom = createPerson('Mom')('Carmen',45,true)
console.log(createaMom)
let createaDad = createPerson('Dad')('Mauricio',53,false)
console.log(createaDad)
//{ name: 'Carmen', age: 45, isWoman: true, type: 'Mom' }
//{ name: 'Mauricio', age: 53, isWoman: false, type: 'Dad' }
Conclusions
- Closure makes currying possible in JavaScript
- It’s ability to retain the state of functions already executed, gives us the ability to create factory🏭
DEcorators
What is decorator
A decorator is simply a way of wrapping a function with another function to extend its existing capabilities. You “decorate” your existing code by wrapping it with another piece of code
https://dev.to/anuradha9712/call-apply-bind-methods-in-javascript-4pfn
let person = {
name: 'Grecia',
sayName(age, city, pet) {
return console.log(`Hi, ${this.name}. You are ${age} and live in ${city} and you have a ${pet}`)
}
}
function decorator(fn, context) {
let count = 0;
return function(...args) {
count ++;
console.log(`Function ${fn.name} has been called ${count} time(s)`)
return fn.call(context, ...args)
}
}
decorator(person.sayName, person)(22, 'Lima','parrot')

Thanks!
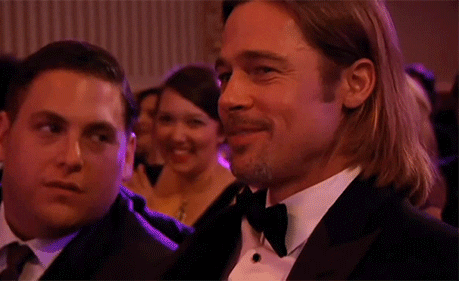
Currying and Decoratos
By Grecia Delgado
Currying and Decoratos
- 75