Rust intro
(for Java Developers)
Devoxx US 2017 - #devoxx
Hi!
- Computer Engineer
- Programming
- Electronics
- Math <3 <3
- Physics
- Lego
- Meetups
- Animals
- Coffee
- Pokémon
- GIFs

#devoxx @hannelita
OSS Projects:
- https://github.com/hannelita/neo4j-cassandra-connector
- https://github.com/hannelita/neo4j_doc_manager
Disclaimer
This is a session about Rust Features :)
Disclaimer
This is not a Rust intro tutorial
Some theory
Some subjects that may cause discussion. Views are on my own.
GIFs :)
Disclaimer
There are some references for introductory Rust Content
Language peace <3
Agenda
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
#devoxx @hannelita
What is Rust?
#devoxx @hannelita
'Rust is a general purpose programming language, compiled, strong and static typed, sponsored by Mozilla Research. It is designed to be a "safe, concurrent, practical language", supporting functional and imperative-procedural paradigms.'
https://en.wikipedia.org/wiki/Rust_(programming_language)#cite_note-FAQ_-_The_Rust_Project-10
Is it yet another language that runs on top of the JVM?

#devoxx @hannelita
No. Rust is not 'yet another language that runs on top of the JVM'.
#devoxx @hannelita
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Embedded systems
#devoxx @hannelita
Sometimes they are so restrictive that you can't use Java.
Which language do you choose?
Source - http://www.diva-portal.org/smash/get/diva2:215157/FULLTEXT01
C and C++
#devoxx @hannelita

C and C++
#devoxx @hannelita
- It's hard to debug
- It can be difficult to maintain the code
- Manual memory allocation
It may be inconvenient.
What are we looking for in terms of language?
#devoxx @hannelita
Good choices
- No manual memory management
- Strong and Static Typed
- Compiled
- Fast
- Reduce number of runtime errors
- Active community
- Good amount of frameworks and libraries
- Open Source
#devoxx @hannelita
Meet Rust!
#devoxx @hannelita

Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Quick view at Rust
fn main() {
}
source: http://rustbyexample.com/fn.html
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fizzbuzz_to(n
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
}
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
}
}
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fizzbuzz(n
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
Macros
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
fn is_divisible_by
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
fn is_divisible_by(lhs: u32,
rhs: u32)
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
fn is_divisible_by(lhs: u32,
rhs: u32) -> bool {
}
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
source: http://rustbyexample.com/fn.html
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
fn fizzbuzz(n: u32){
}
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
fn is_divisible_by(lhs: u32,
rhs: u32) -> bool {
if rhs == 0 {
return false;
}
lhs % rhs == 0
}
Limited type inference. Explicit type declaration for function parameters and return.
(same as in Java)
Quick view at Rust
fn main() {
fizzbuzz_to(100);
}
fn is_divisible_by(lhs: u32, rhs: u32) -> bool {
if rhs == 0 {
return false;
}
lhs % rhs == 0
}
fn fizzbuzz(n: u32) -> () {
if is_divisible_by(n, 15) {
println!("fizzbuzz");
} else if is_divisible_by(n, 3) {
println!("fizz");
} else if is_divisible_by(n, 5) {
println!("buzz");
} else {
println!("{}", n);
}
}
fn fizzbuzz_to(n: u32) {
for n in 1..n + 1 {
fizzbuzz(n);
}
}
source: http://rustbyexample.com/fn.html
Quick view at Rust
fn main() {
let my_binding = 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
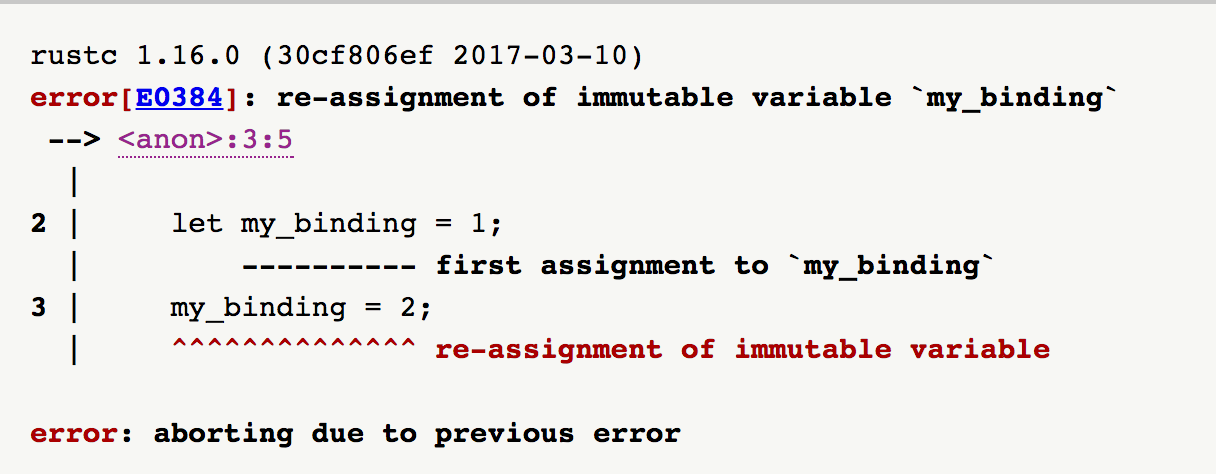
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
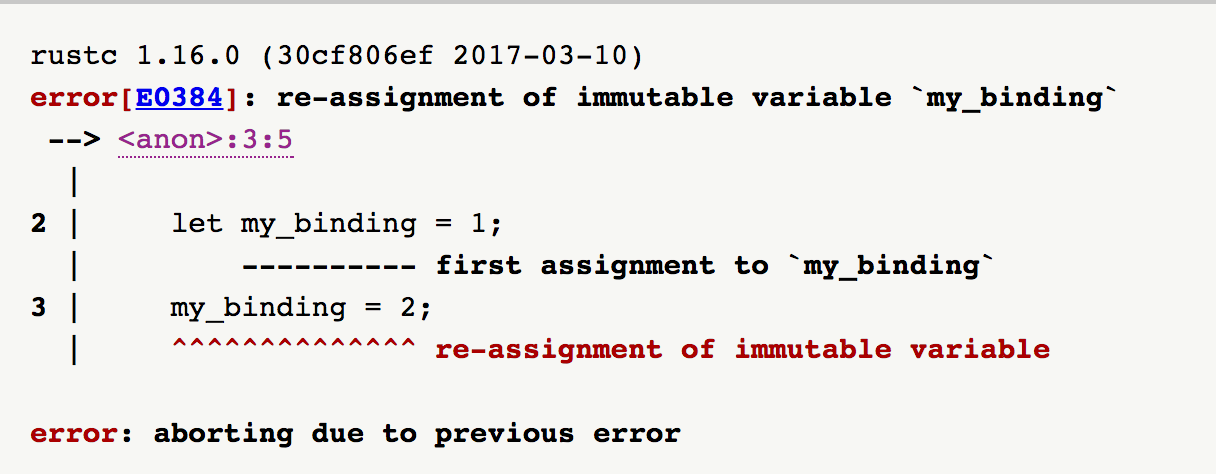
Immutability by default
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
let mut mutable_binding = 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
let mut mutable_binding = 1;
mutable_binding += 1;
}
source: http://rustbyexample.com/variable_bindings/mut.html
Quick view at Rust
fn main() {
let my_binding = 1;
my_binding += 1;
let mut mutable_binding = 1;
mutable_binding += 1;
//^now it works!
}
source: http://rustbyexample.com/variable_bindings/mut.html
Quick view at Rust
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers:
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
(0..).map(|n| n * n) // All natural numbers squared
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
(0..).map(|n| n * n) // All natural numbers squared
.take_while(|&n| n < upper) // Below upper limit
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
(0..).map(|n| n * n) // All natural numbers squared
.take_while(|&n| n < upper) // Below upper limit
.filter(|n| is_odd(*n)) // That are odd
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
(0..).map(|n| n * n) // All natural numbers squared
.take_while(|&n| n < upper) // Below upper limit
.filter(|n| is_odd(*n)) // That are odd
.fold(0, |sum, i| sum + i); // Sum them
println!("result: {}",
sum_of_squared_odd_numbers);
}
source: http://rustbyexample.com/fn/hof.html
Quick view at Rust
fn is_odd(n: u32) -> bool {
n % 2 == 1
}
fn main() {
println!("Find the sum of all the
squared odd numbers under 1000");
let upper = 1000;
let sum_of_squared_odd_numbers: u32 =
(0..).map(|n| n * n) // All natural numbers squared
.take_while(|&n| n < upper) // Below upper limit
.filter(|n| is_odd(*n)) // That are odd
.fold(0, |sum, i| sum + i); // Sum them
println!("result: {}",
sum_of_squared_odd_numbers);
}
source: http://rustbyexample.com/fn/hof.html
High Order Functions
Other features - Tuples, Enums, Structs, Traits.
#devoxx @hannelita
Rust - features
- Memory safe, data race free
- A compiler that blocks lots of runtime errors
- Interface with C/C++
- Generics
- Polymorphism
- By Mozilla and an amazing community
#devoxx @hannelita
Rust - it meets the requirements
#devoxx @hannelita
- No manual memory management √
- Strong and Static Typed √
- Compiled √
- Fast √
- Reduce number of runtime errors √
- Active community √
- Good amount of frameworks and libraries √
- Open Source √
Bonus
- About the same level of verbosity as Java :)
- Rust Compiler is also verbose to explain the errors to you
More about Rust
- No VM
- No GC
- No manual malloc/free
- No seg faults

#devoxx @hannelita
#devoxx @hannelita

How do we achieve the 'No Seg Faults' feature?
#devoxx @hannelita
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Variable bindings own the values in Rust
fn foo() {
let v = vec![1, 2, 3];
}
#devoxx @hannelita
Variable bindings own the values in Rust
fn foo() {
let v = vec![1, 2, 3];
let v2 = v;
}
#devoxx @hannelita
Variable bindings own the values in Rust
fn foo() {
let v = vec![1, 2, 3];
let v2 = v;
}

Rust compiler says:"error: use of moved value: `v` println!("v[0] is: {}", v[0]);"
What?

#devoxx @hannelita
It may sound unpractical, but by having this model, Rust prevents several memory errors.
#devoxx @hannelita
What happens if I REALLY want to share a reference?
fn main() {
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn vect_reduce
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) {
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn sum_vec(v: Vec<i32>) -> i32 {
return v.iter().fold(0, |a, &b| a + b);
}
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
fn main() {
fn sum_vec(v: Vec<i32>) -> i32 {
return v.iter().fold(0, |a, &b| a + b);
}
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce( v1, v2);
}
Borrowing
#devoxx @hannelita
I need to borrow the vectors to the function!
fn main() {
fn sum_vec(v: Vec<i32>) -> i32 {
return v.iter().fold(0, |a, &b| a + b);
}
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce(&v1, &v2);
}
Borrowing
#devoxx @hannelita
I need to borrow the vectors to the function!
fn main() {
fn sum_vec(v: Vec<i32>) -> i32 {
return v.iter().fold(0, |a, &b| a + b);
}
fn vect_reduce(v1: Vec<i32>, v2: Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce(&v1, &v2);
}
Borrowing
#devoxx @hannelita
Also on the function
args:
fn main() {
fn sum_vec(v: &Vec<i32>) -> i32 {
return v.iter().fold(0, |a, &b| a + b);
}
fn vect_reduce(v1: &Vec<i32>, v2: &Vec<i32>) -> i32 {
let s1 = sum_vec(v1);
let s2 = sum_vec(v2);
s1 + s2
}
let v1 = vec![1, 2, 3];
let v2 = vec![4, 5, 6];
let answer = vect_reduce(&v1, &v2);
}
Borrowing
#devoxx @hannelita
Also on the function
args:
Rust allows you to share some references with a feature called 'borrowing'
#devoxx @hannelita
It is similar to Read-Writers lock
let v1 = vec![1, 2, 3];
Reader
Reader
Reader
v1, what is
your value?
v1, what is
your value?
v1, what is
your value?
It is similar to Read-Writers lock
let v1 = vec![1, 2, 3];
Reader
Reader
Reader
v1, what is
your value?
v1, what is
your value?
v1, what is
your value?
Writer
It is similar to Read-Writers lock
let v1 = vec![1, 2, 3];
Reader
Reader
Reader
v1, what is
your value?
v1, what is
your value?
v1, what is
your value?
Writer
Writers, GET OUT OF HERE.
It is similar to Read-Writers lock
let mut v1 = vec![1, 2, 3];
Writer
Writers, GET OUT OF HERE.
It is similar to Read-Writers lock
let mut v1 = vec![1, 2, 3];
Writer
Writers, GET OUT OF HERE.
If v1 is mut, the writer can change the vector value.
It is similar to Read-Writers lock
- Many readers at once OR a single writer with exclusive access
- Read only does not require exclusive access
- Exclusive access does not allow other readers
(More info: https://users.cs.duke.edu/~chase/cps210-archive/slides/moresync6.pdf )
Rust uses a similar model at compile time.
It is similar to Read-Writers lock
- Many readers at once OR a single writer with exclusive access
- Read only do not require exclusive access
- Exclusive access do not allow other readers
Rust uses a similar model at compile time.
T: Base type; owns a value
&T: Shared reader
&mut T: Exclusive writer
(Note: I am not considering another Rust feature called Copy)
It is similar to Read-Writers lock
- Many readers at once OR a single writer with exclusive access
- Read only do not require exclusive access
- Exclusive access do not allow other readers
Rust uses a similar model at compile time.
T: Base type; owns a value
&T: Shared reader
&mut T: Exclusive writer
(Note: I am not considering another Rust feature called Copy)
Immutable reference
Mutable reference
About exclusive writers
fn main() {
let mut x = 5;
}
About exclusive writers
fn main() {
let mut x = 5;
let y = &mut x;
}
About exclusive writers
fn main() {
let mut x = 5;
let y = &mut x;
*y += 1;
}
About exclusive writers
fn main() {
let mut x = 5;
let y = &mut x;
*y += 1;
}
Rust compiler says: "error: cannot borrow `x` as immutable because it is also borrowed as mutable println!("{}", x);"
Top issues that borrowing prevents:
- Iterator invalidation
- Data race problems
- Use after free
#devoxx @hannelita
BTW, how do I free a variable in Rust? Since there is no GC, how should I clean up the memory?
#devoxx @hannelita
Also, I could easily mess up with borrowing by freeing a variable that I lent to a function.
#devoxx @hannelita

#devoxx @hannelita
You don't have to handle that manually. At least, not explicitly.
#devoxx @hannelita

#devoxx @hannelita
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
In Rust, every reference has some lifetime associated with it.
fn lifetimes() {
let a1 = vec![1, 2, 3]; // +
|
|
|
|
|
'a1
|
}
#devoxx @hannelita
In Rust, every reference has some lifetime associated with it.
fn lifetimes() {
let a1 = vec![1, 2, 3]; // +
|
|
|
|
|
'a1
|
}
#devoxx @hannelita
In Rust, every reference has some lifetime associated with it.
fn lifetimes() {
let a1 = vec![1, 2, 3]; // +
let a2 = vec![4, 5, 6]; // + |
// | |
| |
| |
| |
'a2 'a1
}
#devoxx @hannelita
In Rust, every reference has some lifetime associated with it.
fn lifetimes() {
let a1 = vec![1, 2, 3]; // +
let a2 = vec![4, 5, 6]; // + |
// | |
let b1 = &a1; // + | |
let b2 = &a2; // + | | |
foo(b1); // | | | |
foo(b2); // 'b2 'b1 'a2 'a1
// | | | |
}
#devoxx @hannelita
You can explicit lifetimes in Rust
fn explicit_lifetime<'a>(x: &'a i32) {
}
Or even multiple lifetimes:
fn multiple_lifetimes<'a, 'b>(x: &'a str, y: &'b str) -> &'a str {
}
#devoxx @hannelita
By the end of a lifetime, a variable is free.
#devoxx @hannelita
- GC is not necessary
- Another mechanism to avoid dangling pointers
- No manual malloc nor free
Top issues that lifetime system prevents:
#devoxx @hannelita
Okay, so is Rust always safe?
#devoxx @hannelita
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Rust has a good Generics resource, with Traits and Closures
http://huonw.github.io/blog/2015/05/finding-closure-in-rust/
#devoxx @hannelita
Comparison - Java and Rust Features
#devoxx @hannelita


Classes
#devoxx @hannelita


public class MyClass {
private in number = 42;
private MyOtherClass c =
new MyOtherClass();
public int count() {
..
}
}
struct MyClass {
number: i32,
other: MyOtherClass,
}
impl MyClass {
fn myMethodCountHere(&self) -> i32 {
...
}
}
Primitive types
Primitive types
Interfaces
#devoxx @hannelita


public interface MyInterface {
void someMethod();
default void someDefault(String str){
//implementation
}
}
trait Animal {
fn noise(&self) -> &'static str;
fn talk(&self) {
println!("I do not talk to humans");
}
}
struct Horse { breed: &'static str }
impl Animal for Horse {
fn noise(&self) -> &'static str {
"neigh!"
// I can't mimic horse sounds
}
fn talk(&self) {
println!("{}!!!!", self.noise());
}
}
impl Horse {
fn move(&self) {
//impl
}
}
Generics
#devoxx @hannelita


public class MyGeneric<T> {
//impl
}
public class NotGeneric {
public static <T extends Comparable<T>> T maximum(T x, T y) {
//awesome
}
}
trait Traverse<I> {
// methods
}
struct Bag<T> {
//struct
}
impl<T> Bag<T> {
//impl
}
Rust Generics
#devoxx @hannelita

fn general_method<T: MyTrait>(t: T) { ... }
fn general_method<T: MyTrait + AnotherTrait + SomeRandomTrait>(t: T)
(Trait bounds: use it for the good and for the evil)
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Rust is pretty safe not only because of borrowing and lifetimes
#devoxx @hannelita
You can call C/C++ functions from Rust. But C/C++ is not safe.
#devoxx @hannelita
unsafe
fn main() {
let u: &[u8] = &[49, 50, 51];
unsafe {
assert!(u == std::mem::transmute::<&str, &[u8]>("123"));
}
}
#devoxx @hannelita
Encapsulate unsafe calls inside safe functions.
#devoxx @hannelita
So, is Rust perfect?
#devoxx @hannelita
Agenda
#devoxx @hannelita
- What is Rust?
- Why is Rust interesting?
- Rust structure quick overview
- Borrow
- Lifetime
- Feature Comparison
- The sense of safety
- Rust downsides
Top Rust complaints
- Learning curve is not too fast
- Lots of new concepts
- Pretty new language
#devoxx @hannelita
Top Rust complaints
- Learning curve is not too fast
- Lots of new concepts
- Pretty new language
Top Rust responses to these problems
- Great docs and learning resources
- The community is active and willing to help
- The community is building lots of tools and libraries
#devoxx @hannelita
Bonus #1:
How can you describe Rust type system?
#devoxx @hannelita
Answer: Somewhat static, strongly typed. There is a huge research project to describe Rust type system
https://www.ralfj.de/blog/2015/10/12/formalizing-rust.html
#devoxx @hannelita
Bonus #2: Performance
#devoxx @hannelita


source: https://benchmarksgame.alioth.debian.org/u64q/compare.php?lang=rust&lang2=java
Bonus #3:
Free GIF!

References
- https://www.youtube.com/watch?v=Q7lQCgnNWU0
- https://www.quora.com/Why-do-programming-languages-use-type-systems
- http://blogs.perl.org/users/ovid/2010/08/what-to-know-before-debating-type-systems.html
- http://lucacardelli.name/papers/typesystems.pdf
- https://www.ralfj.de/blog/2015/10/12/formalizing-rust.html
- http://jadpole.github.io/rust/type-system
- https://wiki.haskell.org/Typing
- https://gist.github.com/Kimundi/8391398
- https://www.smashingmagazine.com/2013/04/introduction-to-programming-type-systems/
- http://pcwalton.github.io/blog/2012/08/08/a-gentle-introduction-to-traits-in-rust/
- https://llogiq.github.io/2016/02/28/java-rust.html
References - Rust Intro
- https://doc.rust-lang.org/book/
- https://doc.rust-lang.org/reference.html
- https://doc.rust-lang.org/nomicon/
- Rust And Pokémons - http://slides.com/hannelitavante-hannelita/rust-and-pokmons-en#/
- Rust Type System - http://slides.com/hannelitavante-hannelita/rust-type-system-pybr12#/ (Python Brasil 2016 closing keynote)
Special Thanks
- Rust Community - https://www.meetup.com/Rust-Sao-Paulo-Meetup/ and @bltavares
- B.C., for the constant review
- Devoxx Team
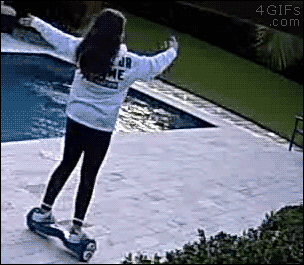
Thank you :)
Questions?
hannelita@gmail.com
@hannelita

Rust Intro (for Java developers) - Devoxx US 2017
By Hanneli Tavante (hannelita)
Rust Intro (for Java developers) - Devoxx US 2017
- 2,398