Frontend for the dark side (aka Backend Team)
Contents
- grunt, bower, npm...
- Angular basics
- Factories
- Promises
- Code Reuse
- Angular intermediate
- One time binding
- The problem with scopes
Grunt, bower, npm...
Grunt, npm, bower...
- Packages defined in: bower.json
- Packages used by the client's browser
- Back-end look-alike: pip
- Package examples: angularjs, bootstrap
Bower
Package manager
Grunt, npm, bower...
- Packages defined in: package.json
- Packages used by the server
- Back-end look-alike: pip (also)
- Package examples: uglifyjs, jasmine, karma
npm
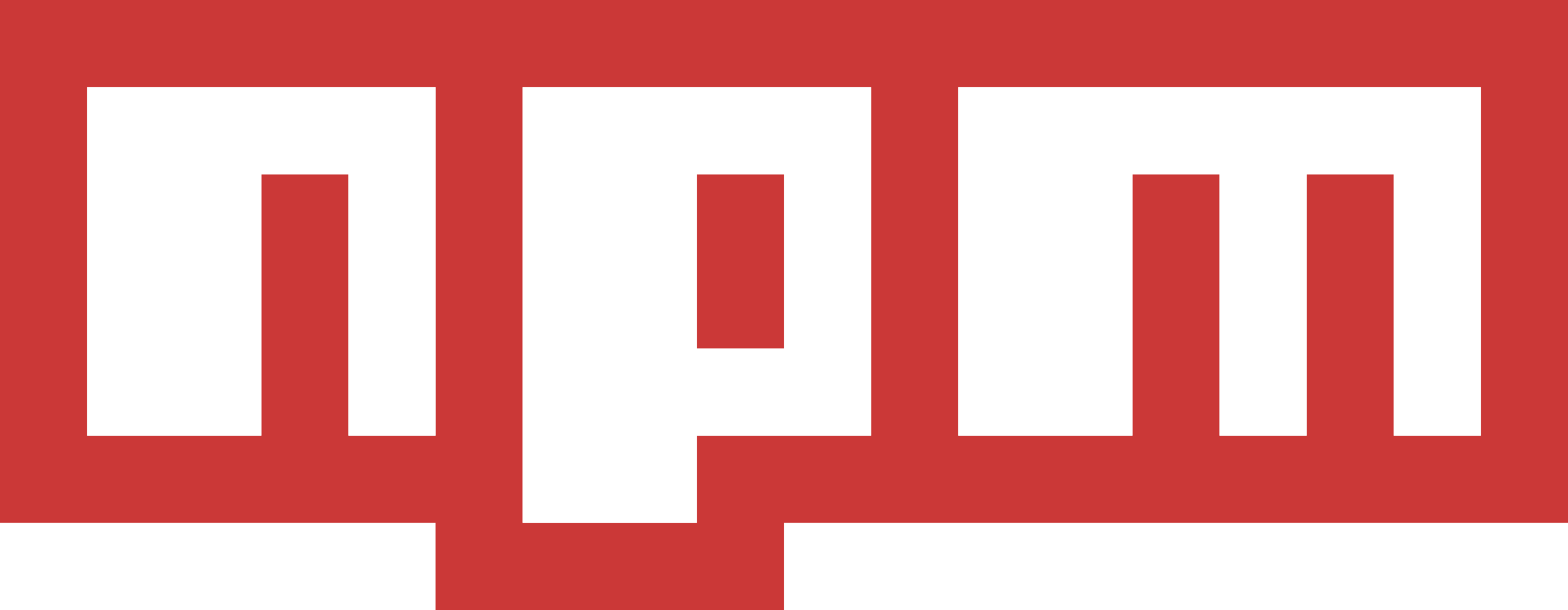
Package manager
Grunt, npm, bower...
Main file | Used by | Common Packages | |
---|---|---|---|
bower |
bower.json | client | angularjs bootstrap |
npm |
package.json | server | uglifyjs jasmine |
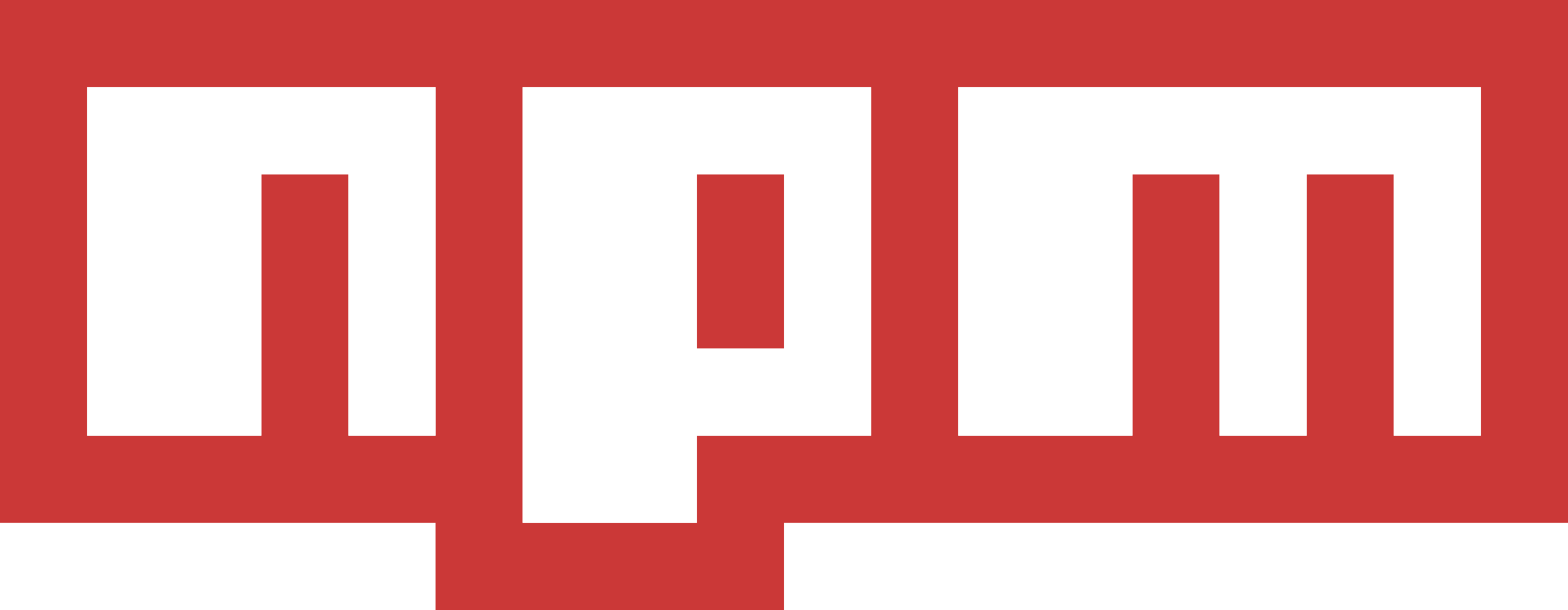
Grunt, npm, bower...
- Used by the server to build F.E. app
- Back-end look-alike: Fabric? (http://www.fabfile.org/)
Grunt
Build manager
Grunt, npm, bower...
Bower packages
Frontend code
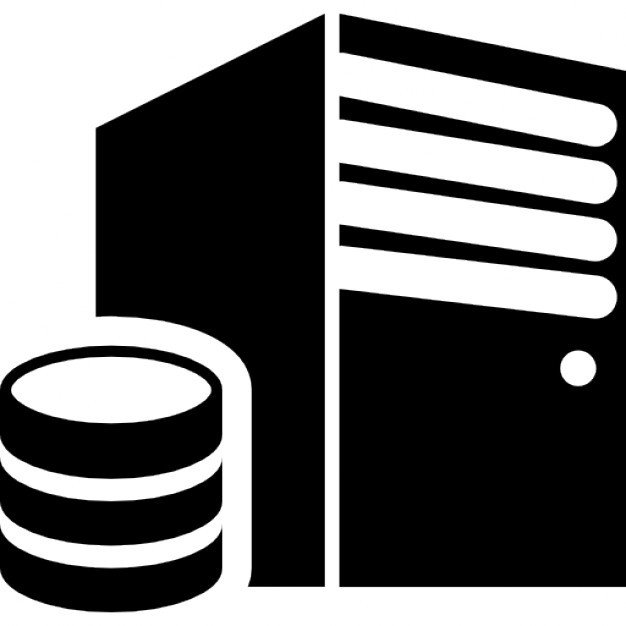
npm packages
download
download
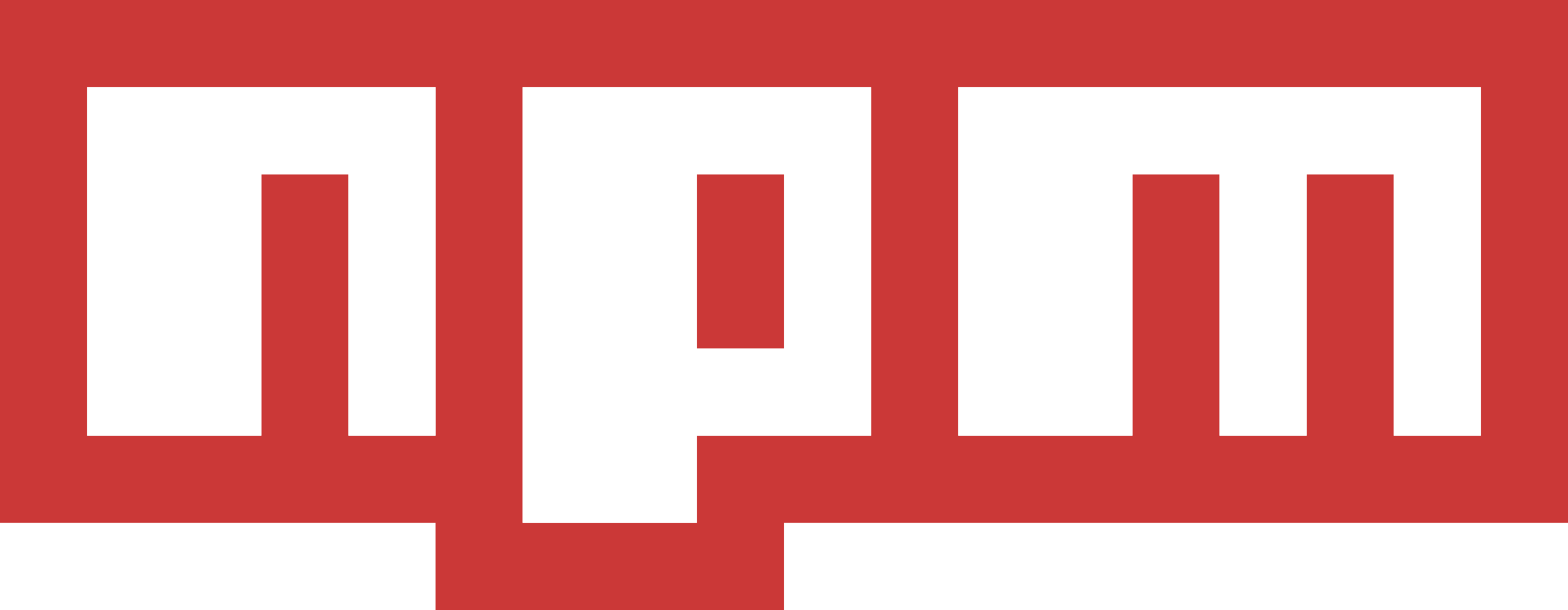
1
2
run
3
uses
to pack / treat
generates
4
frontend app
3a
3b
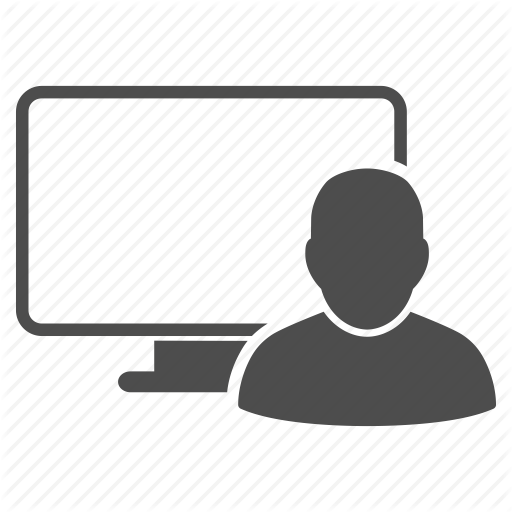
uses
5
Grunt, npm, bower...
Bower packages
Frontend code
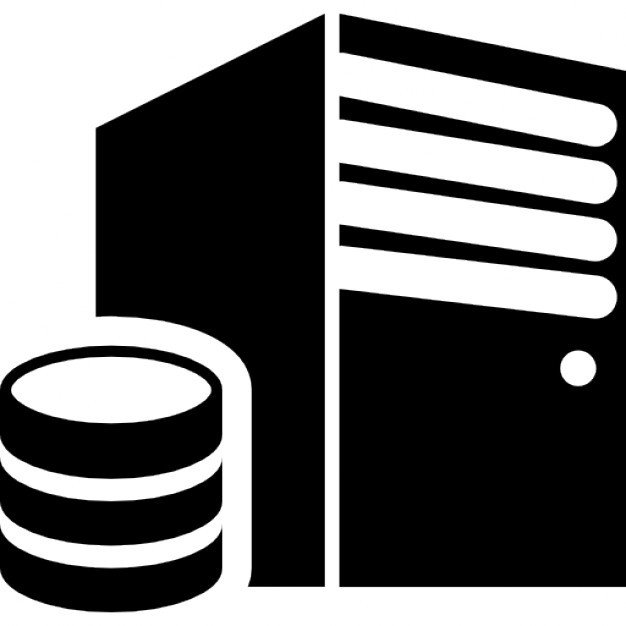
npm packages
download
download
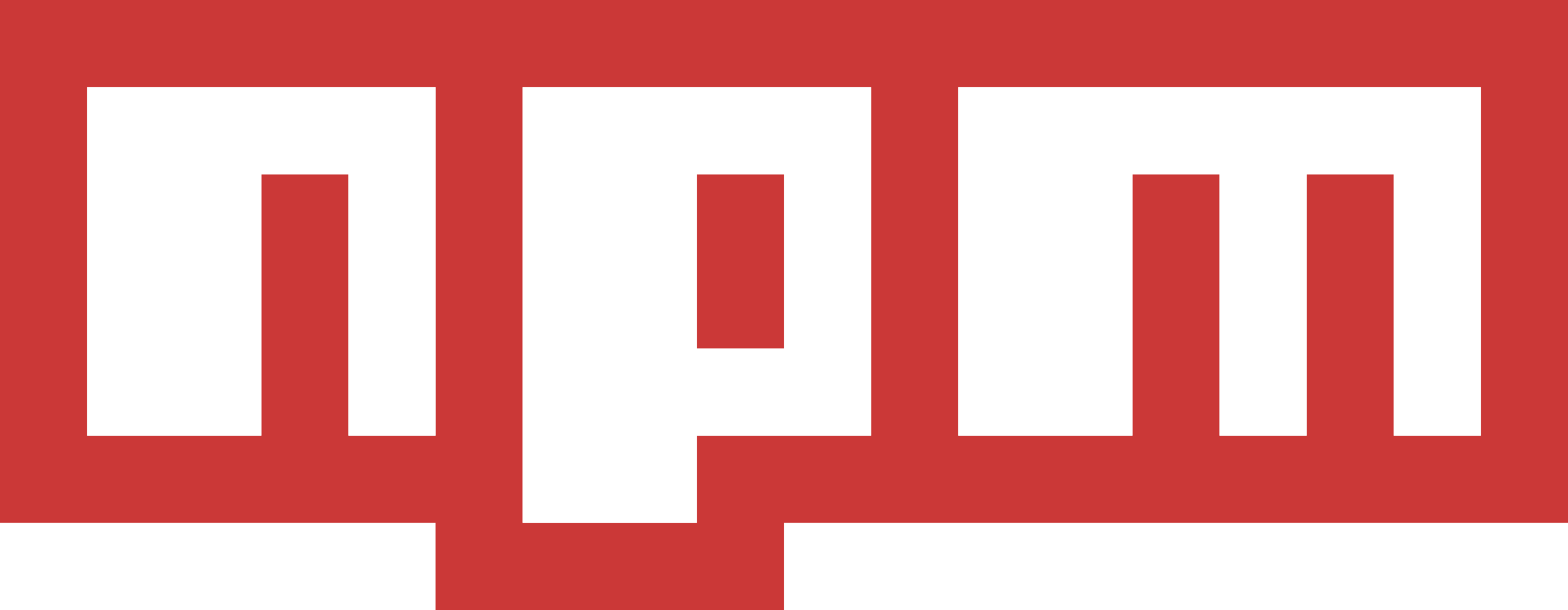
1
2
run
3
uses
to pack / treat
generates
4
frontend app
3a
3b
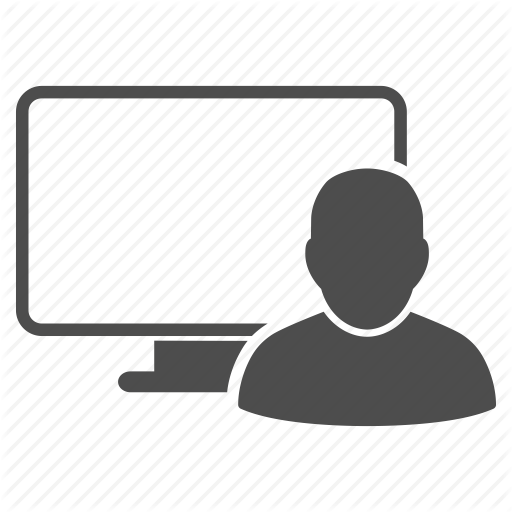
uses
5
Angular basics
Angular basics
Factories
- Also called services
- Maintain app state
Frontend app
loaded
service
service.setSomething(value)
service.getSomething()
view 1
view 2
controller 1 created
controller 2 created
controller 1 destroyed
controller 2 destroyed
Angular basics
Promises
Objects representing asynchronous calls
// some code
var promise = $http.get(...)
// more code, not waiting for $http.get() to finish
promise
.then(...)
.catch(...)
Code executed when the request is finished successfully
Code executed when the request is finished with an error
Angular basics
Code reuse
Providers: Services, Factories, Values
Host state and common logic through all the app
Directives
Reusable UI Components (with their own logic and template)
ngInclude (reusable views)
ngInclude to insert some html into another, combine with stand-alone less files.
Combine with $templateRequest and cache mechanisms to improve performance.
Other advanced javascript techniques
Adding common logic to javascript object prototypes...
Angular intermediate
Angular intermediate
One time bindings
::someVariable
When?
Values that, once loaded, won't change not dinamyc values)
Why?
Improve performance by reducing CPU and memory usage in client
Typical examples
- {{ ::currentUser.userName }}
- ng-repeat="::listOfCurrencies"
- ng-show="::retailer.disabled"
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
JavaScript prototypical inheritance
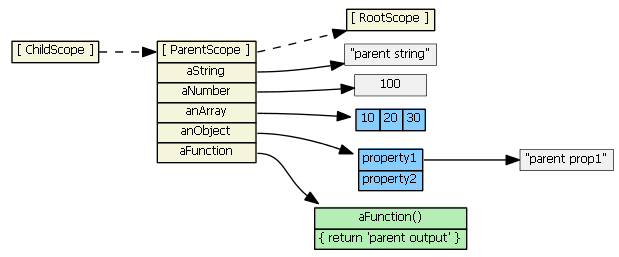
aString visible for [ ChildScope ]
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
JavaScript prototypical inheritance
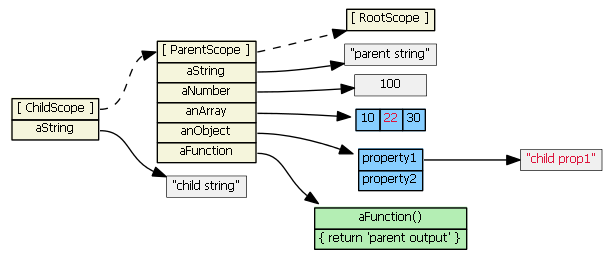
aString in [ ChildScope ] masks the one in [ ParentScope ]
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
JavaScript prototypical inheritance
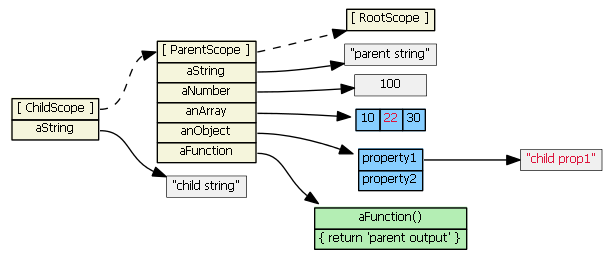
aString in [ ChildScope ] masks the one in [ ParentScope ]
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
Why is this important for AngularJS?
- The following create new scopes, and inherit prototypically: ng-repeat, ng-include, ng-switch, ng-view, ng-controller, directive with scope: true, directive with transclude: true.
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
Why is this important for AngularJS?
Having an ngModel inside an ngRepeat (or other directives creating child scopes) is, then, useless
Solution
Storing models in JS objects, not directly in the scope
ng-model="user.name"
Angular intermediate
The scopes problem
Basically: https://github.com/angular/angular.js/wiki/Understanding-Scopes
Why is this important for AngularJS?
When making write operations on a model inside a child scope
$scope.someVar = "Some value"
Javascript is creating a new someVar in the child $scope instead of modifying the one in the parent scope
End. Questions?
Angular JS for the dark side (aka Backend Team)
By Hector Pablos
Angular JS for the dark side (aka Backend Team)
- 1,730