TypeScript:
How to type a talk




@bloo_df
/bloodf
/heitorramon
Heitor Ramon
- HTML "Developer"
- *.IE6{_content:"Hacker";}
- Table time survivor
- Ex Advertising Worker
- UX
- Frontend Developer
- On the Hype Train
- Author of Vue.js 3 Cookbook
Buy it (amzn.to/32KEQw8 | http://bit.ly/2QEUofl)





@bloo_df
/bloodf
/heitorramon
Agenda




@bloo_df
/bloodf
/heitorramon
Migration Stories
AirBnb: Brie Bunge at Airbnb gave a talk at JSConf Hawaiʻi on how Airbnb adopted TypeScript at Scale
(https://www.youtube.com/watch?v=P-J9Eg7hJwE)
Google: Rodoslav Kirov and Bowen Ni covered how TypeScript became one of the five languages available at Google at TSConf 2018
(https://www.youtube.com/watch?v=sjov1k5jexA)
Slack: Felix Rieseberg at Slack covered the transition of their desktop app from JavaScript to TypeScript in their blog
(https://slack.engineering/typescript-at-slack/)



@bloo_df
/bloodf
/heitorramon
Who use it?








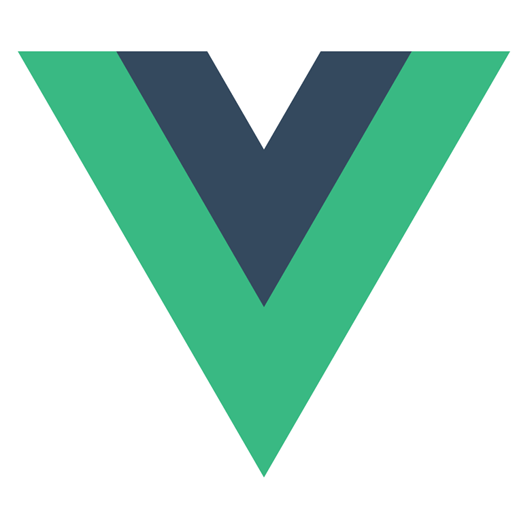




@bloo_df
/bloodf
/heitorramon
Why
Types are
Fundamental
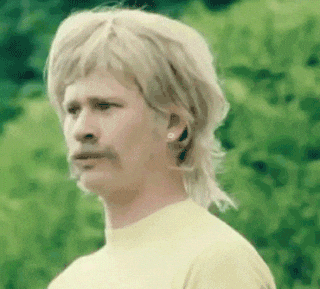



@bloo_df
/bloodf
/heitorramon
Pros and Cons of using it
Do I need to say anything more?

Pros
- Maintainability
- Refactor
- Easy reading and understanding
- @types and .d.ts
Cons
- It's not truly typed
- Adds a new layer of complexibility on JavaScript
- Learning curve for newcomers



@bloo_df
/bloodf
/heitorramon
Back to the Basic
In TypeScript, there are some basic types that are used by the variables and return types.
Those types are:
- String
- Number
- Boolean
- Array
- Unknown
- Enum
- Any
- Void
- Object
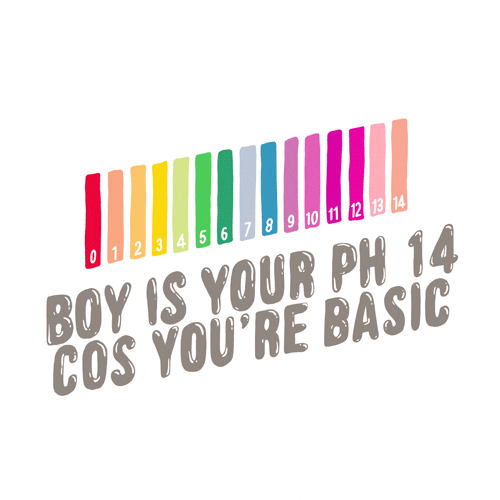



@bloo_df
/bloodf
/heitorramon
Interfaces
Using interfaces in Typescript is a good way to create a contract between classes, objects, or even complex types.
An interface can be manipulated and extended to reach its final form.
interface Shape {
area: number;
}
interface Square extends Shape {
width: number;
height?: number;
}
interface Circle extends Share {
radius: number;
}



@bloo_df
/bloodf
/heitorramon
Custom Types
With custom, types bring the possibility to create new frontiers to the code, where you can bring to the game new comments that are not implemented in the core compiler and re-use codes.
Custom types can be inferred from new types, interfaces, mixed of new methods or special joiners.
type RetroGames = 'Atari' | 'Pong' | 'Commodore';
type EightBitConsole = 'Famicon' | 'MasterSystem';
type VideoGame = RetroGames | EightBitConsole;
interface Shape {
area: number;
}
interface Square extends Shape {
width: number;
height?: number;
}
interface Circle extends Shape {
radius: number;
}
type GeometricForm = Square | Circle



@bloo_df
/bloodf
/heitorramon
Advanced Types
TypeScript provides some advanced types that can improve the code, where you can take advantage within as a type guard or as a new type on the compiler.
The type guards provide security on compiling and development.
const pet = getSmallPet();
const fishPet = pet as Fish;
const birdPet = pet as Bird;
if (fishPet.swim) {
fishPet.swim();
} else if (birdPet.fly) {
birdPet.fly();
}
function f<T extends boolean>(x: T): T extends true
? string
: number;
function foo(x: string | number): string | number;
type T1 = ReturnType<typeof foo>;



@bloo_df
/bloodf
/heitorramon
Utility Types
TypeScript provides some utility types with helper interfaces that use Generics to get the types and interfaces.
Those helpers can be imported into the code and used everywhere you could use an interface or a type.
interface Todo {
title: string;
description: string;
completed?: boolean;
}
function updateTodo(fieldsToUpdate: Partial<Todo>) {
return { ...todo, ...fieldsToUpdate };
}
type TodoPreview = Omit<Todo, "description">;
const todo: TodoPreview = {
title: "Clean room",
completed: false,
};
const todo2: Required<Todo> = {
title: "Clean House",
description: "Clear the House",
completed: true,
};



@bloo_df
/bloodf
/heitorramon
Decorators
With ES6 bringing classes and the possibility to create an annotation and modifying classes.
A Decorator can be attached to a class declaration, method, accessor, property, or parameter.
function f() {
console.log("f(): evaluated");
return function () {
console.log("f(): called");
};
}
function g() {
console.log("g(): evaluated");
return function (
target,
propertyKey: string,
descriptor: PropertyDescriptor
) {
console.log("g(): called");
};
}
class C {
@f()
@g()
method() {}
}



@bloo_df
/bloodf
/heitorramon
Iterators and Generators
JavaScript with its new versions brought the possibility of Generators functions.
Those functions can be exited and later re-entered. Their context (variable bindings) will be saved across re-entrances.
Iterators are functions that define a sequence and potentially a return value upon its termination.
function* generator(i) {
yield i;
yield i + 10;
}
const gen = generator(10);
console.log(gen.next().value); // 10
console.log(gen.next().value); // 20
const it = makeRangeIterator(1, 10, 2);
let result = it.next();
while (!result.done) {
console.log(result.value); // 1 3 5 7 9
result = it.next();
}



@bloo_df
/bloodf
/heitorramon
Where I can learn?
- TypeScript HandBook - typescriptlang.org/docs/handbook
- TS DeepDive - basarat.gitbook.io/typescript
- TS CheetSheet - rmolinamir.github.io/typescript-cheatsheet
- EggHead Courses - egghead.io/search?query=typescript
- Understanding TypeScript - udemy.com/course/understanding-typescript/
- Effective TypeScript: 62 Specific Ways to Improve Your TypeScript
- Programming TypeScript: Making Your JavaScript Applications Scale
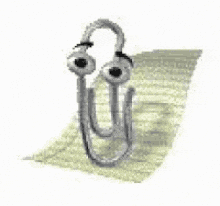



@bloo_df
/bloodf
/heitorramon
Thank You
Typescript: How to type a talk
By Heitor Ramon Ribeiro
Typescript: How to type a talk
- 178