{Objects}
[Arrays]
Function(s){}
slides: slides.com/hrjr/obj-arr-func
exercises: https://github.com/kuychaco/JSFundamentals1
Credits
Bianca Gandolfo
Co-Founder of Telegraph Academy
(coding school for URMs)
http://www.telegraphacademy.com/

Intros
- Name
- Experience
- Motivation
Who is this for?
2-6mos experience learning JavaScript
Completed JS tutorial like Codecademy, CodeSchool, Khan Academy, or Bloc.io
Want to solidify fundamentals
Looking for more practice
Interested in getting into Hack Reactor

Part 1
Review and solidify core JS principles with project.
Part 2
Get started with functional methods.
After
Use foundation to grow as a JS engineer.



Class Format
- Silence your ringtones (please)
- Ask questions (between slides)
- Answer my questions
- Show thumbs
- Ask for help during exercises
- Lunch at some point (unless your phone rings during lecture)
Objects
{}
OVERVIEW
- Property Access
- Bracket Notation
- Dot Notation
- Dot vs Bracket
- Nested Objects
- Object Literals
- Iteration


Creating Objects
var box = {};

Assignment w/ Dots
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";

Access w/ Dots
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";
box.material; //??

Access w/ Dots
var box = {"material" : "cardboard"}
||
cb
var box = {};
box.material = "cardboard";
var cb = box.material;

Overwriting
box = {"material" : "cardboard"}
box = {"material" : "titanium"}
var box = {};
box.material = "cardboard";
box.material = "titanium";

Access before Assignment
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";
box.material; //"cardboard"
box.size; //??

Brackets - Assignment
var box = {"material" : "cardboard"}
var box = {};
box["material"] = "cardboard";
box.material; //??

Brackets - Access
var box = {"material" : "cardboard"}
var box = {};
box["material"] = "cardboard";
box["material"]; //??

Variables
var box = {};
box["material"] = "cardboard";
var key = "material";
box[key]; //??

Expressions
var box = {};
box["material"] = "cardboard";
var func = function(){
return "material";
};
box[func()]; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
box[key]; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
box[key]; //"cardboard"

Do's && Don'ts
Keys w/Special Chars
box = { "material" : "cardboard", "^&*" : "testing 123" }
var box = {};
box["material"] = "cardboard";
box["^&*"] = "testing 123";
var test = box["^&*"];
box.@#! //SYNTAX ERROR
Primitive Keys
box = { "material" : "cardboard", "0" : "meow", "3" : 3, "true" : "bark",
"null" : "hello", "undefined" : "goodbye" }
var box = {};
box["material"] = "cardboard";
box[0] = "meow";
box[1+2] = 3;
box[true] = "bark";
box[null] = "hello";
box[undefined] = "goodbye";
Bracket vs. Dots

THE RULES
DOTS
strings
Brackets
"strings
"quotes required
"weird characters
other primitives
variables
numbers
quotations
weird characters
expressions
expressions
Storing Other Objects
var box = {};
box["material"] = "cardboard";
box["size"] = {
"height": 2,
"width": 80
};
box.area = function(){
return box.size.height
* box.size.width;
};
box = { "material" : "cardboard", "size" : { "height": 2, "width": 80 }, "area" : fn() {...}
}
Object Literal
var box = {
"area" : function() {...},
"material" : "cardboard",
"size" : {
"height" : 2,
"width" : 80
}
};
var box = {
area : function() {...},
material : "cardboard",
size : {
height : 2,
width : 80
}
};
Iteration
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(box[key]); //??
}
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(key); //??
}
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(box.key); //??
}
What is an object?
What is the difference between dot and bracket notation?
When can you only use brackets and not dot?
When can you only use dot notation and not bracket?
How do you add a property with a key that contains special characters?
How do you add a property with a key that is stored in a variable?
How do you add a property whose key and value are stored in different variables?
How do you access an object that is inside another object?
How do you create an object that is nested inside another object?
How do we loop through objects to access the values?










Exercise Time

- What is the format for exercises?
- Pair programming?
- Can I use references?
- devdocs.io
- stack overflow
- Who are the TAs?
- When should I ask for help?
- How will they help?
Arrays
[]
Overview
- Arrays vs Objects
- Access && Assignment
- Native Properties
- Iteration

Arrays vs Objects

- JavaScript Arrays are Objects
- Arrays work best for storing lists of values
- Arrays store elements at numeric indices
- Arrays have built-in properties and methods to make it easier to work with lists of values
- Arrays can store any type of JavaScript value
Access and Assignment

box: [true, 'meow', {'hello' : 'goodbye'}]
index#: 0 1 2
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // ??
box[1]; // ??
box.pop() //??
box: [true]
box: [true, 'meow']
box: [true, 'meow', {'hello':'goodbye'}]
box: []
box: [true, 'meow']
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // ??
box.pop() //??
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // 'meow'
box.pop() //??
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // 'meow'
box.pop() // {'hello' : 'goodbye'}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // 9
box[0]; // 'meow'

Access and Assignment
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // ??
box[0]; // ??
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // 9
box[0]; // ??
{0 : 'meow', 'size' : 9 }
Access and Assignment

box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // ??
box[0]; // ??
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box[0]; // ??
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box[0]; // 'meow'
The Rules Don't Change!
Access and Assignment

box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // ??
box.0; // ??
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box.0; // ??
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box.0; // SyntaxError: Unexpected number
Native Properties

box: ['meow']
index #: 0
var box = [];
box['size'] = true;
box['0'] = 'meow';
box.length; //??
{0 : 'meow', 'size' : true }
var box = [];
box['size'] = true;
box['0'] = 'meow';
box.length; // 1

Native Properties
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box['length']; //??
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box['length']; // 4

Native Properties
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box[length]; //??
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box[length]; // undefined

Native Properties
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[3] = {'babyBox': true};
box[box.length]; //??
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[3] = {'babyBox': true};
box[box.length]; // undefined

Native Properties
box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; //??
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; // Whoohooo!

Native Properties
box: ['meow', 'Whoohooo!']
index #: 0 1
{0 : 'meow', 1 : 'Whoohooo!'}
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; //??
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; // Whoohooo!
Iteration

box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // ??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // 0
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // 0 size
}
Iteration

box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // ??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // undefined
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // undefined undefined
}
Iteration

box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); //??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); // meow
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); // meow 9
}
Iteration

box: ['meow', 'Whoohooo!']
index #: 0 1
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); //??
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); // 0
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); // 0 1
}
Iteration

box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); //??
}
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); // undefined
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); // undefined undefined
}
Iteration

box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); //??
}
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); // meow
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); // meow Whoohoo!
}
What do you use to loop through an array?
What are some different methods of adding values to an array?
What are some special properties about arrays that are different from objects?
What are some ways to access values in your array?
When would you use an array over an object?





Nesting
var box = {"innerBox" : {}}
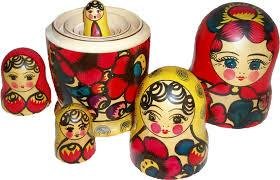
var box = {};
box.innerBox = {};
// or...
box['innerBox'] = {};
Nesting
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
// or...
var box = {
innerBox : {
innerInnerBox : {}
}
};
Nesting
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box['innerBox'].innerInnerBox = {};
box.innerBox //??
Nesting
var box = { "innerBox" : { "innerInnerBox" : { "full" : true } } }
var box = {};
box.innerBox = {};
box.innerBox['innerInnerBox'] = {};
var myInnerBox = box['innerBox'];
myInnerBox; //??
Exercise Time

Functions
function(s){}
Overview
- Anatomy
- Definition vs Invocation
- Named Functions vs Anonymous
- Parameters vs Arguments
- Return Values and Side Effects


Anatomy


Definition
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Body
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Named vs Anonymous
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Invocation/Call-time
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Arguments/Parameters
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Return/Side Effects
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
$('body').hide();
setTimeout(function() {
console.log("hello");
}, 1000);

Quick Review
var add = function(a, b){
return a + b;
};
add(3, 4); // ??

P.S. Functions are Objects!
var add = function(a, b){
return a + b;
};
add.example = 'testing 123!';
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
var farm = [];
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
var farm = [];
for(var i = 0; i < animalNames.length; i++){
var animalName = animalNames[i];
var animal = animalMaker(animalName);
farm.push(animal);
}
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
var farm = [];
for(var i = 0; i < animalNames.length; i++){
// condensed into one line
farm.push(animalMaker(animalNames[i]));
}
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
var farm = [];
for(var i = 0; i < animalNames.length; i++){
farm.push(animalMaker(animalNames[i]));
}
for(var j = 0; j < farm.length; j++){
var animal = farm[j];
animal.speak();
}
Looping

var animalMaker = function (name) {
return {
speak: function () {
console.log("my name is ", name);
}
};
};
var animalNames = ['Sheep', 'Liger', 'Big Bird'];
var farm = [];
for(var i = 0; i < animalNames.length; i++){
farm.push(animalMaker(animalNames[i]));
}
for(var j = 0; j < farm.length; j++){
farm[j].speak();
}
Exercises
Part 1
is
complete!
Live Classes Monthly
Check Dates here
Debrief
- Want to be a TA?
- Organize study groups amongst yourselves
-
Look out for a follow-up email
- Feedback (difficulty level, format, exercises, topics)
- Additional learning resources
- Please clean up after yourselves
Q & A
- Other JavaScript topics
- Mutability
- Anonymous Functions vs Named Functions
- Career stuff
- Daily life of a software engineer
- Roadmap / career path
- Hack Reactor
- Anything else?
JS Fundamentals 1: Objects, Arrays, Functions
By hrjr
JS Fundamentals 1: Objects, Arrays, Functions
4/12/2015, 6/14/2015
- 1,480