{Objects}
[Arrays]
Function(s){}
slides: slides.com/hrjr/telegraphPrepWeek1
exercises: https://github.com/telegraphPrep/week1
Credits
Bianca Gandolfo
Co-Founder of Telegraph Academy
(coding school for URMs)
http://www.telegraphacademy.com/

Intros
- Name
- Experience
- Motivation
Who is this for?
2-6mos experience learning JavaScript
Completed JS tutorial like Codecademy, CodeSchool, Khan Academy, or Bloc.io
Want to solidify fundamentals
Looking for more practice
Interested in getting into Telegraph Academy

In this class
Review and solidify core JS principles with project.
Get started with functional methods.
After
Use foundation to grow as a JS engineer.



Class Format
- Silence your ringtones (please)
- Ask questions (between slides)
- Answer my questions
- Show thumbs
- Ask for help during exercises
Let's start our journey!
Announcements
Space Tour
Water bottles
Join Slack!
TAs
Expectations
- We focus on pure JS here- no HTML/CSS/jQuery/Node/Angular, etc.
Expectations
- We're here to make you highly effective engineers, not to give you a comfortable experience
- A bumpy road will get you there more quickly
Google/
Stackoverflow
- Using Google and StackOverflow is a part of your job as an engineer. Get used to using these resources now.
Your Success Metrics
- The number of times you logged something to the console and got a different result.
- Your ability to explain exactly what every single word and piece of punctuation is doing once you have the expected output.
Success, reiterated
1. Experiment. Try new things like crazy. See what you get.
2. Sit down and explain things super methodically once you have the right answer.
let's get started!!
Objects
{}
OVERVIEW
- Property Access
- Bracket Notation
- Dot Notation
- Dot vs Bracket
- Nested Objects
- Object Literals
- Iteration

KEY TAKEAWAY:
DOT NOTATION WHEN YOU KNOW THE property NAME YOU'RE LOOKING UP
BRACKET NOTATION WITH VARIABLES OR NUMBERS

Creating Objects
var box = {};

Assignment w/ Dots
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";

Access w/ Dots
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";
box.material; //??

Access w/ Dots
var box = {"material" : "cardboard"}
||
cb
var box = {};
box.material = "cardboard";
var cb = box.material;

Overwriting
box = {"material" : "cardboard"}
box = {"material" : "titanium"}
var box = {};
box.material = "cardboard";
box.material = "titanium";

Access before Assignment
var box = {"material" : "cardboard"}
var box = {};
box.material = "cardboard";
box.material; //"cardboard"
box.size; //??

Brackets - Assignment
var box = {"material" : "cardboard"}
var box = {};
box["material"] = "cardboard";
box.material; //??

Brackets - Access
var box = {"material" : "cardboard"}
var box = {};
box["material"] = "cardboard";
box["material"]; //??

Variables
var box = {};
box["material"] = "cardboard";
var key = "material";
box[key]; //??

Expressions
var box = {};
box["material"] = "cardboard";
var func = function(){
return "material";
};
box[func()]; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
box[key]; //??
var box = {};
box['material'] = "cardboard";
var key = 'material';
box['key']; //undefined
box.key; //undefined
box[key]; //"cardboard"

Do's && Don'ts
Keys w/Special Chars
box = { "material" : "cardboard", "^&*" : "testing 123" }
var box = {};
box["material"] = "cardboard";
box["^&*"] = "testing 123";
var test = box["^&*"];
box.@#! //SYNTAX ERROR
Primitive Keys
box = { "material" : "cardboard", "0" : "meow", "3" : 3, "true" : "bark",
"null" : "hello", "undefined" : "goodbye" }
var box = {};
box["material"] = "cardboard";
box[0] = "meow";
box[1+2] = 3;
box[true] = "bark";
box[null] = "hello";
box[undefined] = "goodbye";
Bracket vs. Dots

THE RULES
DOTS
strings
Brackets
"strings
"quotes required
"weird characters
other primitives
variables
numbers
quotations
weird characters
expressions
expressions
KEY TAKEAWAY:
DOT NOTATION WHEN YOU KNOW THE NAME YOU'RE LOOKING UP
BRACKET NOTATION WITH VARIABLES OR NUMBERS
Storing Other Objects
var box = {};
box["material"] = "cardboard";
box["size"] = {
"height": 2,
"width": 80
};
box.area = function(){
return box.size.height
* box.size.width;
};
box = { "material" : "cardboard", "size" : { "height": 2, "width": 80 }, "area" : fn() {...}
}
Object Literal
var box = {
"area" : function() {...},
"material" : "cardboard",
"size" : {
"height" : 2,
"width" : 80
}
};
var box = {
area : function() {...},
material : "cardboard",
size : {
height : 2,
width : 80
}
};
Iteration
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(box[key]); //??
}
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(key); //??
}
var box = {};
box['material'] = "cardboard";
box[0] = 'meow';
box['^&*'] = "testing 123";
for(var key in box){
console.log(box.key); //??
}
What is an object?
What is the difference between dot and bracket notation?
When can you only use brackets and not dot?
When can you only use dot notation and not bracket?
How do you add a property with a key that contains special characters?
How do you add a property with a key that is stored in a variable?
How do you add a property whose key and value are stored in different variables?
How do you access an object that is inside another object?
How do you create an object that is nested inside another object?
How do we loop through objects to access the values?










Exercise Time

- What is the format for exercises?
- Pair programming?
- Can I use references?
- devdocs.io
- stack overflow
- Who are the TAs?
- When should I ask for help?
- How will they help?
Exercise Time

https://github.com/TelegraphPrep/week1
Arrays
[]
Overview
- Arrays vs Objects
- Access && Assignment
- Native Properties
- Iteration

Arrays vs Objects
- JavaScript Arrays are Objects
- Arrays work best for storing lists of values
- Arrays store elements at numeric indices
- Arrays have built-in properties and methods to make it easier to work with lists of values
- Arrays can store any type of JavaScript value
Access and Assignment
box: [true, 'meow', {'hello' : 'goodbye'}]
index#: 0 1 2
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // ??
box[1]; // ??
box.pop() //??
box: [true]
box: [true, 'meow']
box: [true, 'meow', {'hello':'goodbye'}]
box: []
box: [true, 'meow']
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // ??
box.pop() //??
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // 'meow'
box.pop() //??
var box = [];
box[0] = true;
box[1] = 'meow';
box.push({'hello' : 'goodbye'});
var i = 0;
box[i]; // true
box[1]; // 'meow'
box.pop() // {'hello' : 'goodbye'}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // 9
box[0]; // 'meow'
Access and Assignment
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // ??
box[0]; // ??
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box['size']; // 9
box[0]; // ??
{0 : 'meow', 'size' : 9 }
Access and Assignment
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // ??
box[0]; // ??
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box[0]; // ??
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box[0]; // 'meow'
The Rules Don't Change!
Access and Assignment
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // ??
box.0; // ??
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box.0; // ??
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.size; // 9
box.0; // SyntaxError: Unexpected number
Native Properties
box: ['meow']
index #: 0
var box = [];
box['size'] = true;
box['0'] = 'meow';
box.length; //??
{0 : 'meow', 'size' : true }
var box = [];
box['size'] = true;
box['0'] = 'meow';
box.length; // 1
Native Properties
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box['length']; //??
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box['length']; // 4
Native Properties
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box[length]; //??
var box = [];
box['0'] = 'meow';
box[3] = {'babyBox': true};
box[length]; // undefined
Native Properties
box: ['meow', undefined, undefined, {'babyBox': true}]
index #: 0 1 2 3
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[3] = {'babyBox': true};
box[box.length]; //??
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[3] = {'babyBox': true};
box[box.length]; // undefined
Native Properties
box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; //??
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; // Whoohooo!
Native Properties
box: ['meow', 'Whoohooo!']
index #: 0 1
{0 : 'meow', 1 : 'Whoohooo!'}
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; //??
var box = [];
box['0'] = 'meow';
box[1] = 'Whoohooo!';
box[box.length - 1]; // Whoohooo!
Iteration
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // ??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // 0
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(k); // 0 size
}
Iteration
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // ??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // undefined
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box.k); // undefined undefined
}
Iteration
box: ['meow']
index #: 0
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); //??
}
{0 : 'meow', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); // meow
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
for(var k in box){
console.log(box[k]); // meow 9
}
Iteration
box: ['meow', 'Whoohooo!']
index #: 0 1
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); //??
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); // 0
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var k in box){
console.log(box[k]);
}
for(var i = 0; i < box.length; i++){
console.log(i); // 0 1
}
Iteration
box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); //??
}
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); // undefined
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box.i); // undefined undefined
}
Iteration
box: ['meow', 'Whoohooo!']
index #: 0 1
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); //??
}
{0 : 'meow', 1 : 'Whoohooo!', 'size' : 9 }
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); // meow
}
var box = [];
box['size'] = 9;
box['0'] = 'meow';
box.push('Whoohoo!');
for(var i = 0; i < box.length; i++){
console.log(box[i]); // meow Whoohoo!
}
What do you use to loop through an array?
What are some different methods of adding values to an array?
What are some special properties about arrays that are different from objects?
What are some ways to access values in your array?
When would you use an array over an object?





Exercise Time

https://github.com/TelegraphPrep/week1
Control flow
For Loops & Conditionals (if/else)
What do we use looping for?

Accessing all the things inside a storage container
Doing something to each item in that storage container
Finding certain things in that storage container
Seeing if something is in that container
What do we use looping for?

Basically, whenever we want to access things inside an array or object, and we don't know exactly where it is beforehand
If we know where it is, we can grab it directly, like so:
hiddenCaveObject[topSecretTreasure]
//gives us the value stored
//at the topSecretTreasure property!
arrOfHumansSortedByHeight[0]
//gives us the tallest human
Types of For Loops

Arrays and Objects each get their own special for loop.
Occasionally you can get results that look somewhat correct when you use the wrong loop- DON'T EVER DO THIS!!!
They each have their own loop for a reason.
//ARRAYS
for( var i = 0; i < arr.length; i++) {
//do things...
}
//OBJECTS
for( var key in obj) {
//do things...
}
Array For loops

Arrays are numerically indexed.
This means you can access the values stored in an array by accessing a numbered position in that array.
var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//do things...
}
Array For loops

The code in between the curly braces is called the body of the for loop.
var arr = ['pirate','dragon','unicorn'];
for( var i = 0; i < arr.length; i++) {
//this is the body of the loop
//the code in here gets executed once
//for each iteration
}
Array For loops

Let's break down the signature of the for loop- the part in between the parens.
for( var i = 0; i < arr.length; i++) {
}
Array For loops

i is just a number, that we start at 0.
var i = 0;
//create a new variable called i
//set it equal to 0
Array For loops

Condition
i < arr.length;
//this is the ending condition
//as long as this is true,
//we will continue with the loop
//as soon as this is not true
//the loop ends
Array For loops

final expression
i++
//this says to increment i by 1
//this code gets run once for each iteration
//this is what changes the value of i
//this is evaluated after the body of the loop has run
Array For loops

All together: we instantiate a new variable i and set it equal to 0, while i is less than the length of the array, we execute the code in the loop body, and then increment i by 1.
for( var i = 0; i < arr.length; i++) {
//body
}
Array For loops

Each iteration of the for loop just executes the code inside the loop body, no matter what that code is.
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('hi from inside the for loop!');
}
//'hi from inside the for loop!'
//'hi from inside the for loop!'
//'hi from inside the for loop!'
Array For loops

i is just a number, starting at 0 and increasing up to one less than the length of the array.
Remember: arrays are 0 indexed, but the length property is not.
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('i: ' + i + ', arr.length: ' + arr.length);
}
//'i: 0, arr.length: 3'
//'i: 1, arr.length: 3'
//'i: 2, arr.length: 3'
Array For loops

How do we use the number stored in the variable i to access the values in the array?
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
//'pirate'
//'unicorn'
//'dragon'
Array For loops

How do we use the number stored in the variable i to access the values in the array?
var arr = ['pirate','unicorn','dragon'];
for( var i = 0; i < arr.length; i++) {
console.log('the valued stored at
the ' + i + 'th position of the array
is: ' + arr[i]
);
}
//'the value stored at the 0th position of the array is pirate'
//'the value stored at the 1th position of the array is unicorn'
//'the value stored at the 2th position of the array is dragon'
Yay for arrays!!
Object For loops

Objects are key indexed.
This means you can access the values stored in an object by accessing a key in that object.
var obj = {
occupation: 'pirate',
steed: 'dragon',
bestFriend: 'unicorn'
};
for( var key in obj ) {
//do things...
}
Object For loops

The syntax of object for loops isn't like anything else you'll see in JavaScript.
You just have to memorize it.
for( var key in obj ) {
//this is the loop body
}
Object For loops

Let's break down the pieces.
var key
//we create a new variable called key
Object For loops

This part is magic. You don't need to understand how it works. Just accept the fact that it does work (trust me, it does).
If you doubt it, test it out in your console!
var key
//we create a new variable called key
//JS does some magic on the back end
//and knows that this variable will get set
//to the string of each property name
Object For loops

in obj
//which object we're iterating through
Object For loops

for(var key in obj) {
//body
}
All together now: we declare a new variable called key, and tell it which object we're iterating through, then it runs the body of the loop once for each iteration.
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for(var key in obj) {
console.log('hi from inside obj loop!');
}
//'hi from inside obj loop!'
//'hi from inside obj loop!'
//'hi from inside obj loop!'
The body of the for loop is executed once for each of the keys in the object we're iterating through.
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log(key);
}
//'occupation'
//'steed'
//'bestFriend'
So what is key on each iteration?
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log(key);
}
//'bestFriend'
//'steed'
//'occupation'
Note: we can't assume that the keys in objects are in any certain order.
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var propertyName in obj ) {
console.log(propertyName);
}
//'bestFriend'
//'steed'
//'occupation'
Note: we can use any name we want for the variable that will get set equal to all the keys.
Object For loops

var unitedStatesObj = {rhodeIsland: 'tiny',
alaska: 'untamed', ohio: 'electorally relevant',
california: 'heck yes!'};
for( var state in unitedStateObj ) {
console.log(state);
}
//'rhodeIsland'
//'alaska'
//'ohio'
//'california'
You can use this to be clear about what each property name is.
Generally though, just stick with var key in obj. That's the default and is easy to understand until things get super complex.
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log(obj[key]);
}
//'pirate'
//'dragon'
//'unicorn'
So how do we use these property names to access the values in an object?
Object For loops

var obj = {occupation: 'pirate',
steed: 'dragon', bestFriend: 'unicorn'};
for( var key in obj ) {
console.log('the value stored at ' + key
+ ' is: ' + obj[key]);
}
//'the value stored at occupation is: pirate'
//'the value stored at steed is: dragon'
//'the value stored at bestFriend is: unicorn'
So how do we use these property names to access the values in an object?
Yay for Object loops!!
If statements

if('something that will evaluate to true or false') {
//body of the if statement
//this code is only executed
//if the condition is true
}
Also called conditional statements
If statements

var alwaysFalse = false;
if(alwaysFalse) {
//this code is never executed
}
Also called conditional statements
If statements

var alwaysFalse = false;
if(alwaysFalse) {
//this code is never executed
} else {
//this code is executed if the condition is false
}
if can be followed by else. This ensures that some block of code is always executed.
If statements

var alwaysFalse = false;
if(alwaysFalse) {
console.log('code was executed');
} else {
console.log('code was executed');
}
//'code was executed'
//clearly this is not a useful program
//but it shows that no matter what,
//one of the two blocks of code is executed
//because the thing inside the if parens
//will be evaluated to be either true or false
//and the if block will be executed if true
//and the else block will be executed if false
if can be followed by else. This ensures that some block of code is always executed.
If statements

var temperature = 79;
if(temperature > 80) {
console.log('too hot inside,
party in the streets!');
} else {
console.log('good biking temperature');
}
//'good biking temperature'
if can be followed by else. This ensures that some block of code is always executed.
If statements

var temperature = 79;
if(temperature > 80) {
console.log('too hot inside,
party in the streets!');
} else if(temperature < 32) {
console.log('stay indoors you fools!');
} else {
console.log('good biking temperature');
}
//'good biking temperature'
if can also be followed by else if. This lets you chain together multiple conditions.
If statements

//Things that evaluate to true or false:
'preston' === 'albrey'
8 > 5
6*2 === 12
var arr = [6,7,8,9,10];
3 < arr.length
arr[2] === 8
The thing inside the parentheses next to if must evaluate to true or false.
If statements

//always use the triple equals sign.
//double equals does some freaking weird stuff.
//please don't make me go there
//keep it easy and just use threequals
//always.
Threequals!!
If statements

//Other things that evaluate to true or false:
'hi there' //true
var arr = undefined;
arr //false
arr = [1,2,3];
arr //true
0 //false
-1 //true
"" //false
When evaluating a boolean expression (true or false), JavaScript converts everything to be a true or false value.
These are called 'truthy' and 'falsy'.
If statements

var alwaysTrue = true;
var alwaysFalse = false;
alwaysTrue && alwaysFalse //false
alwaysTrue || alwaysFalse //true
// && means both sides must be true
// || means just one side must be true
We can also chain boolean expressions together using && (and) as well as || (or).
If statements

var alwaysTrue = true;
var alwaysFalse = false;
!alwaysTrue //false
!alwaysFalse //true
//we can apply the negation operator
//directly to a truthy/falsy value
8 !== 5 //true
'preston' !== 'preston' //false
We can also negate an operator. We can use !== to mean "is not equal to".
time to practice!!
Exercises are available at:
https://github.com/telegraphPrep/week1
under day3LoopsAndIf.js
Functions
function(s){}
Overview
- Anatomy
- Definition vs Invocation
- Named Functions vs Anonymous
- Parameters vs Arguments
- Return Values and Side Effects


What is a function?
A function is nothing more than a set of instructions that we create.
Another way of phrasing this: A function is a block of code that we write that gets executed at some other point.

Why do we use functions?
1. Programmers are lazy. We don't like typing out the same lines of code over and over again. So instead, we write out a function that has those lines of code in it, and then we just invoke that function each time rather than writing out all the code again.
2. To control where the code gets executed. Functions let us write out a block of code but not have to run it right away. We can then choose when to run this code later on.

Anatomy


Definition
var nameImprover = function (name, adj) {
};

Body
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};

Named vs Anonymous
//Anonymous function saved into a variable
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//named function
function nameImprover(name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
}
//both work!

anonymous function
function(item) {
return item * 3;
}
//An anonymous function is just a set of instructions
//Since we're not invoking it yet, those instructions
//exist, but are not executed until later
//This becomes super useful in higher order functions
//REMEMBER THIS!!

Invocation/Call-time
Declaring a function is like creating a recipe: you decide what the steps are, but nothing actually gets made.
Invoking a function is like baking that recipe: now that you know what the steps are, you get to actually do them!
The cool part about this is that you can pass in different ingredients to that recipe each time! And each one is run totally independently of all other times that recipe has been created.

Invocation/Call-time
//Definition/Declaration:
var breadMaker = function(ingredient) {
return 'fresh baked ' + ingredient + 'bread';
}
//Invocation:
breadMaker('banana nut');
breadMaker('guacamole');
//Putting () next to a function name means
//that you are invoking it right then.

Arguments/Parameters
//When we define a function, what that function
// takes in (it's signature) are called parameters
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//When we invoke a function, what we pass in to
//that particular invocation are called arguments
nameImprover('preston','purple');

Return/Side Effects
//Returned value:
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
//Side Effects:
var instructorName = 'preston';
var sideEffectImprover = function(adj) {
instructorName = 'Col ' + instructorName +
' Mc' + adj + ' pants';
};

Return/Side Effects
//Returned value:
var nameImprover = function (name, adj) {
return 'Col ' + name + ' Mc' + adj + ' pants';
};
var returnResults = nameImprover('preston','purple');
returnResults; //'Col preston Mcpurple pants'
//Side Effects:
var instructorName = 'preston';
var sideEffectImprover = function(adj) {
instructorName = 'Col ' + instructorName +
' Mc' + adj + ' pants';
};
var sideEffectResults = sideEffectImprover('purple');
sideEffectResults; //undefined
instructorName; //'Col preston Mcpurple pants'

Why Use Side effects?
var logResults = console.log('side effects are useful');
logResults; //undefined
var addUserToDatabase = function(username, userObj) {
if(database[username] === undefined) {
database[username] = userObj;
}
};
//note that we are not returning anything here.
//we don't want to return the entire database
//and we already have the userObj since we had it
//to pass into addUserToDatabase.
//The function is clearly still useful, even though
//it doesn't return anything.

Return ends function
var totallyHarmless = function() {
return 'world peace';
launchAllNuclearMissiles();
};
totallyHarmless(); //returns 'world peace'
//does not invoke launchAllNuclearMissiles
//because that comes after the return statement
//and return statements immediately stop the
//function and end it.

tunnel- return
Since a function creates it's own local scope, the global* scope can't see anything that's going on inside that function body.
The return value is the one way for the outside world to 'tunnel into' the function and see something that's going on.
We can use side effects to save the work that a function that has done for us into a variable that's accessible in the global scope.
Or, we can return a value, directly communicating the results to whatever we have in the global scope that's listening for the results of the function.

tunnel- return
var testArr = [1,2,3,4];
var globalSum = 0;
var sumFunc = function(arr) {
for (var i = 0; i < arr.length; i++) {
globalSum += arr[i];
}
return 'string from sumFunc';
};
var returnVal = sumFunc(testArr);
console.log(globalSum); //10
console.log(returnVal); //'string from sumFunc'

Quick Review
var add = function(a, b){
return a + b;
};
add(3, 4); // ??

Quick Review
var add = function(a, b){
return a + b;
};
add(3, 4); // 7

P.S. Functions are Objects!
var treasureChest = function(){
return "you can't get nothin' from me!";
};
treasureChest.treasureMap = 'twirl three times';
console.log(treasureChest.treasureMap);
//'twirl three times'

P.S. Functions are Objects!
Anything we can do with an object, we can do with a function.
Examples:
Add a property to it (not super useful)
Assign a variable to point to it
Overwrite a variable with it
Pass it in as an argument to a function (super useful- that's what functional programming is!)
Return it as a value from a function (also pretty useful)
Practice TIME!!
https://github.com/telegraphPrep/week1
under day4Functions.js
Nesting
var box = {"innerBox" : {}}
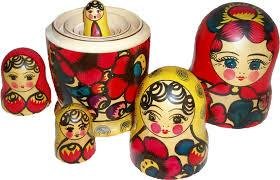
var box = {};
box.innerBox = {};
// or...
box['innerBox'] = {};
Nesting
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box['innerBox'].innerInnerBox = {};
box.innerBox //??
Nesting
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
var box = { "innerBox" : { "innerInnerBox" : {} } }
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
var box = {};
box.innerBox = {};
box.innerBox.innerInnerBox = {};
// or...
box['innerBox'].innerInnerBox = {};
// or...
box['innerBox']['innerInnerBox'] = {};
// or...
var box = {
innerBox : {
innerInnerBox : {}
}
};
Nesting
var box = { "innerBox" : { "innerInnerBox" : { "full" : true } } }
var box = {};
box.innerBox = {};
box.innerBox['innerInnerBox'] = {};
var myInnerBox = box['innerBox'];
myInnerBox; //??
Part 1
is
complete!
Live Classes Monthly
Check Dates here
Debrief
- Want to be a TA?
- Organize study groups amongst yourselves
-
Look out for a follow-up email
- Feedback (difficulty level, format, exercises, topics)
- Additional learning resources
- Please clean up after yourselves
Q & A
- Other JavaScript topics
- Mutability
- Anonymous Functions vs Named Functions
- Career stuff
- Daily life of a software engineer
- Roadmap / career path
- Hack Reactor
- Anything else?
Telegraph Prep Week1: Objects, Arrays, Loops, andFunctions
By hrjr
Telegraph Prep Week1: Objects, Arrays, Loops, andFunctions
A deep introduction to JS fundamentals
- 2,827