Getting Started

Exploring Node.JS

Prerequisites
-
JavaScript
-
ES6 concepts
-
Command Line
This class is for:
-
Server-side engineers
-
Developers experienced with browser-side JavaScript
-
Anyone who wants to learn Node.js
WHAT WE WILL LEARN
What Node is
What you can do with Node
How To Install Node
Node REPL and how to execute Node
How Node Works
Browser vs. Node
Event loop and concurrency
Advantages and drawbacks of Node
Chrome V8 Engine
Node Architecture
Client vs. Server
Single Thread
Non-Blocking
Node Binary
Libuv
BONUS
EXERCISE
- Install Node.js on your machine
- Verify your installation with node -v
- Use the Node REPL and write some Javascript (2 + 2)
- Use the Node CLI executable against a .js file
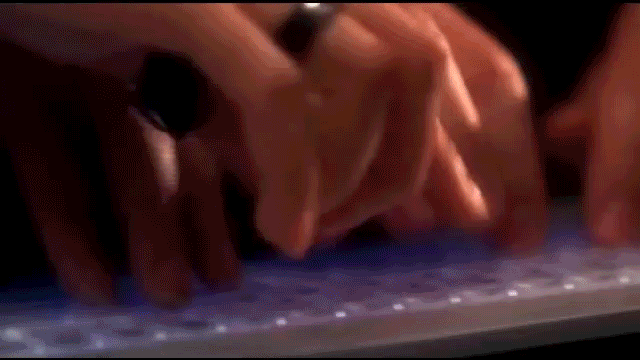
WHAT IS NODE.JS?
-
Javascript Runtime Environment
-
Built on Chrome's V8 Javascript Engine
-
Created by Ryan Dahl in 2009
-
Open source
-
Server-side scripting
-
Cross-platform
-
Persistence
Google Chrome’s V8 JavaScript Engine
The V8 engine is the open-source JavaScript engine that runs in Google Chrome and other Chromium-based web browsers, including Brave, Opera, and Vivaldi. It was designed with performance in mind and is responsible for compiling JavaScript directly to native machine code that your computer can execute.




JIT
Client Side
- Frontend Code
- Code that results in something the user sees in their web browser.
Server Side
- Backend Code
- Code used for application logic (how data is organized and saved to the database).
- Server-side code is responsible for authenticating users on a login page, running scheduled tasks, and even ensuring that the client-side code reaches the client.
LG’s InstaView Fridge Come with Windows 10 Tablet on the Door
Google Servers
How Not To Install Node.js?
-
Using a Node installer is the least recommended way of installing Node
- Package managers fall behind Node.js release cycle
- Installing global modules with Node's module installer (npm) can require the use of sudo (a command which grants root privileges). And it's not a good security practice.
Installing Node.js on macOS and Linux
The recommended way to install Node.js on macOS and Linux is by using a Node version manager, in particular nvm. See https://github.com/nvm-sh/nvm for full details.
INSTALL NVM
The way to install nvm is via the install script at https://github.com/nvm-sh/nvm. If curl is installed (it usually is) a single command can be used to install and setup nvm:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.37.2/install.sh | bash
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.37.2/install.sh | zsh
cat install.sh | bash
cat install.sh | zsh
Verify The Installation of NVM
command -v nvm
If this fails on Linux, close and reopen the terminal (or SSH session) and try running the command again. On macOS see https://github.com/nvm-sh/nvm#troubleshooting-on-macos for in depth troubleshooting.
Install Latest Version of Node
nvm install node # "node" is an alias for the latest version
nvm install 6.14.4 # install a specific version
node --version
node -v
Verify Node is Installed:
Installing Node.js on Windows 10 and Up
Node Version Switcher(nvs) is the recommended version manager for Windows. The nvs version manager is actually cross-platform so can be used on macOS and Linux but nvm is a lot more popular.
To install nvs on Windows go to the release page, https://github.com/jasongin/nvs/releases,
download the MSI installer file of the latest release:

After it's installed open a cmd.exe or powershell prompt and run the following:
nvs add latest # latest version of node
nvs add lts # add the latest LTS version of node
nvs use lts # activate the newly installed version
node -v # verify that Node is installed
How To Know which node is installed?
which node

Running Node.js Code
-
REPL
-
Execute Files
REPL (Read, Eval, Print, Loop)
-
Great for trying things out, think console on the browser, playground
-
It allows easy execution of any JavaScript code in a virtual environment
-
Just type the node command with no args and begin
Text
2 + 2
let name = 'Node'
5 === 5
const hello = () => {
return 'world'
}
.editor
function myFunction(a, b) {
return a * b;
}
function myAnotherFunction(a, b) {
return a + b;
}
myFunction(3,6)
myAnotherFunction(3,6)
.help
Number.
Array.
console.log(global)
.break
Math.random()
const num = _
num
Text
TAB AND UNDERSCORE
EXECUTE JAVASCRIPT CODE
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Browser
- Build interactive apps for the web
- DOM
- Window
- Fragmentation
- Optional modules (es6)
- Cannot access filesystem
- Async
- Browser APIs
Node
- Build server side apps and scripts
- No GUI
- Global
- Versioned
- Required modules (commonjs +)
- Can access filesystem
- Async
- No browser based APIs
What Is Node.JS NOT?
-
a web framework
-
multi-threaded, think of it as a single threaded server (EVENT LOOP)
-
beginner friendly
Node.js is an event-based, non-blocking, asynchronous I/O runtime that uses Google’s V8 JavaScript engine and libuv library.
WHY JAVASCRIPT?
“JavaScript has certain characteristics that make it very different than other dynamic languages, namely that it has no concept of threads. Its model of concurrency is completely based around events.”
- Ryan Dahl

"Any application that can be written in JavaScript, will eventually be written in JavaScript."
Jeff Atwood, Author, Entrepreneur, Cofounder of StackOverflow.


Client/browser
Server
2. The server responds to the client by loading a page or sending data.
1. The client makes a request to the server.








SINGLE THREADED
PHP
NODE
- The server requests a file from the file system.
- The server waits until the system opens and reads the file.
- The server returns the data to the client.
- The server handles the next request.
- The server requests a file from the file system.
- The server handles the next request.
- When the system opens and reads the file, the server will return the data to the client.
NON-BLOCKING I/0
Asynchronous Programming


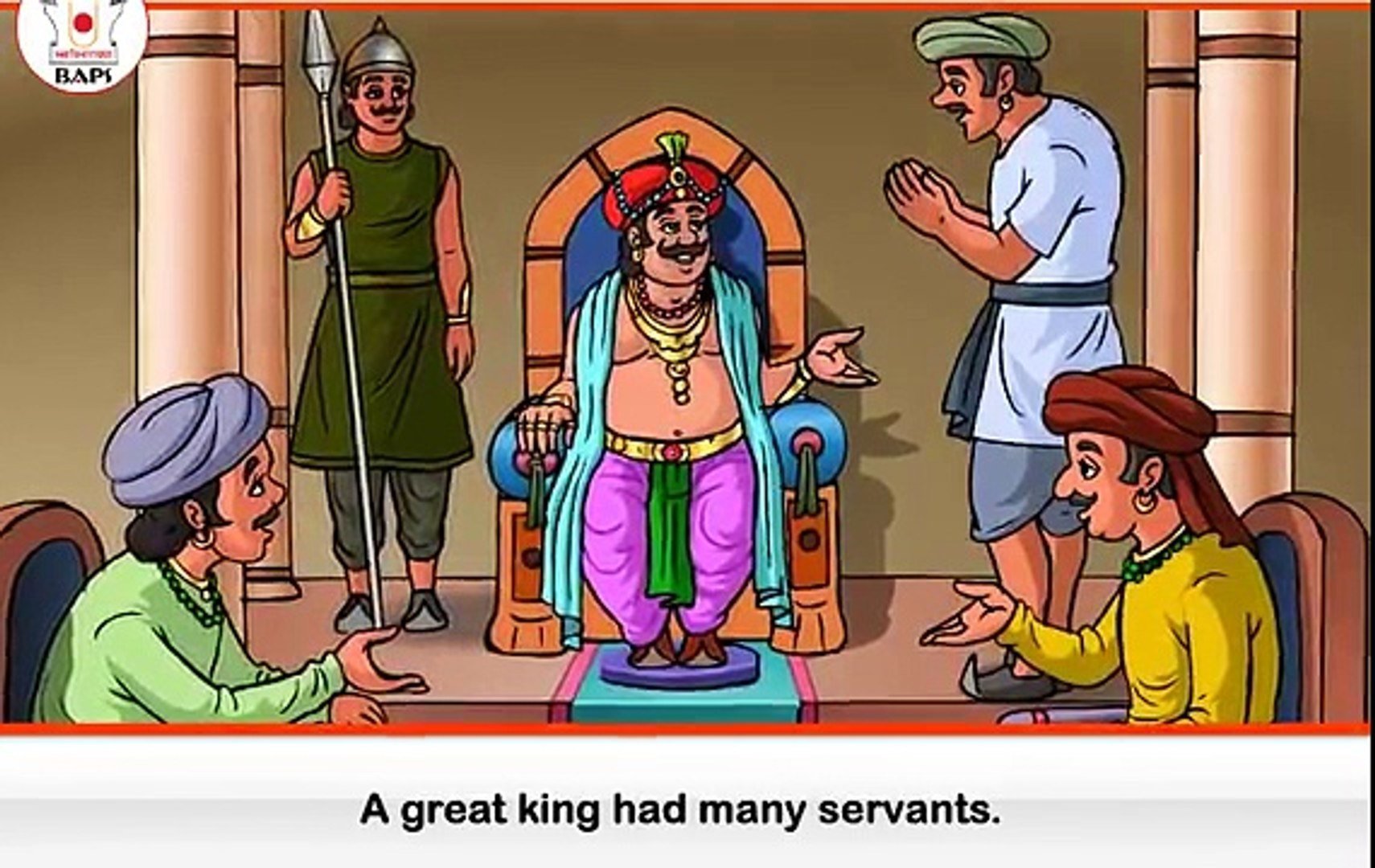
What can you do with Node?
- Shell scripts for file manipulation or network access
- Interactive command-line interfaces
- Build web servers (http, express)
- Powerful APIs & back-end interfaces, full-stack apps
- WebSocket Server like a Chat Server
- File Upload
- Desktop Applications (Electron)
Electron, the Node.js-based wrapper around the Chrome engine, lets Node.js developers create desktop GUI applications and is the foundation on which many popular applications have been built, including the Atom and Visual Studio Code editors, GitKraken, Postman, Etcher, and the desktop Slack client.






What can you do with Node?
-
CDN's
-
Shareable libraries
-
IOT
- Microservices or serverless API backends
- Query databases (MongoDB, MYSQL, Postgres, Redis)
Additional Resources
The following applications are built with Node.js:
-
CLI tools such as hexa.run and Azure Functions CLI
-
Back-end servers and API services such as Express.js and NestJS
-
Desktop apps such as Slack (using Electron)
-
IoT libraries such as Johnny-Five, Puck-js, and Tessel
-
Plug-ins for SketchApp and Adobe XD
-
Code editors such as Visual Studio Code and Atom
-
Native mobile development with NativeScript
Advantages of Node
- It's fast because it's mostly C code
- It's simple to use
- It's JavaScript
- Fast to deploy
- Scalability
- Community
- No more browser incompatibilities or polyfills
Companies that
use Node.js














What Node.js Brings
-
Built-in module system(fs, http,https crypto,zip,...)
- C++, JavaScript add-ons build packages
-
Debugger and other utilities
-
NPM
-
Command Line Interface
-
Module dependency manager
Drawbacks
-
Node’s asynchronous non-blocking nature
-
Having a big package registry
-
Security risks
-
CPU intensive tasks



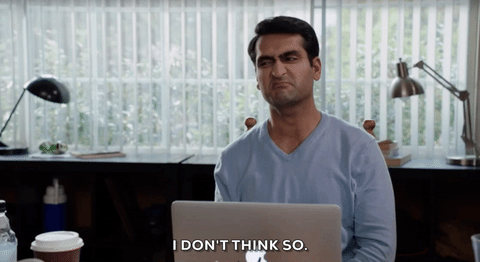
BONUS INTERVIEW QUESTIONS !!!!!
1. In a Node.js environment, who executes the JavaScript code that you write?
A VM, like V8 or Chakra
2. In which programming languages can you use to create Node.js Addons?
C++ and JavaScript
3. Why is Node.js Single-threaded?
Node.js is single-threaded for async processing. By doing async processing on a single-thread under typical web loads, more performance and scalability can be achieved instead of the typical thread-based implementation.
4. How is Node.js most frequently used?
-
Real-time chats
-
Internet of Things
-
Complex SPAs (Single-Page Applications)
-
Real-time collaboration tools
-
Streaming applications
-
Microservices architecture
5.WHAT IS NPM?
NPM stands for Node Package Manager, responsible for managing all the packages and modules for Node.js.
-
Provides online repositories for node.js packages/modules, which are searchable on https://www.npmjs.com/
-
Provides command-line utility to install Node.js packages and also manages Node.js versions and dependencies
6. WHAT IS A CALLBACK IN NODE.JS?
A callback function is called after a given task. It allows other code to be run in the meantime and prevents any blocking. Being an asynchronous platform, Node.js heavily relies on callback. All APIs of Node are written to support callbacks.
7. WHAT IS I/O ?
-
The term I/O is used to describe any program, operation, or device that transfers data to or from a medium and to or from another medium
-
Every transfer is an output from one medium and an input into another. The medium can be a physical device, network, or files within a system
8. What is the command used to import external libraries?
The “require” command is used for importing external libraries.
const http = require ('http')
This will load the HTTP library and the single exported object through the HTTP variable.
9. What are the two types of API functions in Node.js?
The two types of API functions in Node.js are:
-
Asynchronous, non-blocking functions
-
Synchronous, blocking functions
10. What is LIBUV?
libuv is a multi-platform support library with a focus on asynchronous I/O. It was primarily developed for use by Node.js, but it’s also used by Luvit, Julia, pyuv, and others.
Some of the features of libuv are:
-
Full-featured event loop backed by epoll, kqueue, IOCP, event ports.
-
Asynchronous TCP and UDP sockets
-
Asynchronous file and file system operations
-
Child processes
-
File system events
LINKS
-
Node.js Design Patterns(book)
-
Node Cookbook(book)
-
Node.js Web Development:5th Edition(book)
-
Node.js The Right Way (book)
-
Awesome-Nodejs(github)
-
Stream Handbook (github)
-
Awesome Nodejs Learning (github)
-
Node Best Practices (github)
-
Node School (website)
-
30 Days of Node (website)
- Web Development with Node and Express (book)

node.js
By Hulya Karakaya
node.js
course slides
- 1,689