Python: INTRO
Iain Nash
Apr 22nd
IEEE Tech Talk
Why Python?
- intuitive
- dynamic
- easy to get started
- little/no boilerplate
- "batteries included"
- consistent
- simple tools
simple "hello world"
In C:
#include >stdio.h<
int main(int argc, char** argv)
{
printf("Hello World");
return 0;
}
In Java:
class HelloWorldApp {
public static void main(String[] args) {
System.out.println("Hello World!"); // Display the string.
}
}
In Python:
print('hello world!')
Step -1:00
Why is python called "python"?
On 2/27/07, Doug Stone <dejstone at pacbell.net> wrote: > Hi. I just started investigating Python and was wondering about the origin > of Python's name. > What did van Rossum have in-mind when he named Python? http://en.wikipedia.org/wiki/Guido_van_Rossum About the origin of Python, Van Rossum wrote in 1996: Over six years ago, in December 1989, I was looking for a "hobby" programming project that would keep me occupied during the week around Christmas. My office ... would be closed, but I had a home computer, and not much else on my hands. I decided to write an interpreter for the new scripting language I had been thinking about lately: a descendant of ABC that would appeal to Unix/C hackers. I chose Python as a working title for the project, being in a slightly irreverent mood (and a big fan of Monty Python's Flying Circus).
Step 00: GEtting started
If you're on:
Mac: just open the terminal!
Windows: install activestate.com/activepython/
Linux: you should know what you're doing
All else fails? Go to http://python.org/'s interactive shell
Step 01: DAtatypes
# string / text
"im a string!"
# int / number
24
# float / number with decimal
24.4
# list / a list of somethings (numbers etc)
[12, 14, 4, 524, 5, -240, 50] or ["asdf", "52jgar", "afwefw4"]
# dict / a list of something to something
{'city': 'la', 'state': 'california'}
# function
help() # does something
# Try entering a few strings in your console!
# type print("im a string!") to print a string to the screen?
Step 01.00:
Datatypes can be manipulated intuitively
How to use datatypes:
{'number_of_people_here': 50, 'number_of_people_paying_attention': 20}
vs
number_of_people_here = 50
number_of_people_paying_attention = 20
number_of_people_not_paying_attention = number_of_people_here - number_of_people_paying_attention
vs
people_count = 50
people_showing_attention = 20
people_not_showing_attention = people_count - people_showing_attention
Step 01:01
& python has builtin functions to help you out
int(10.0) <- force integer
float(10) <- force float
str(10) <- force string
(int)10.0
Step 02: functions
def imafunction():
print 'hi world!'
imafunction()
# calls the function imafunction
# prints hi world.
why use functions?
1: to allow code reuse
2: to make it easy to
separate
code
functions "transform" and act on data types
(note: that colon after the function is REALLY important!, it tells python the next
lines are part of the function.)
Step 02:01
Functions Transform Data:
def add(num1, num2):
return num1 + num2
def max(num1, num2):
if (num1 > num2):
return num1
else:
return num2
def min(num1, num2):
if (num1 < num2):
return num1
else:
return num2
def sub(num1, num2):
return num1 - num2
def lowest_diff(num1, num2):
return sub(max(num1, num2), min(num1, num2))
print lowest_diff(20, 90)
Step 02.02
You can import collections of functions
(classes) to use in your own programs
import random
# random from 10 to 100
print random.randrange(10, 100)
# random from 0 - 1
print random.random()
words = ['this', 'makes', 'sentence', 'sense', 'what']
print words
random.shuffle(words)
print words
Step 02:03
Python has "batteries included", by including many libraries.

Step 02:04
Such as:
os: os specific information / operations
sys: system-level operations
time: time math and manipulation, tracking
urllib2: download urls from the internet
HTMLParser: parse html
smtplib: send emails with smtplib
SimpleHTTPServer: build a basic webserver
pythonistic gotchyas
White space MATTERS
def eat(food):
print 'eating ' + food
def eat(food):
print 'eating ' + food
def eat(food):
print 'eating ' + food
def eat(food):
print 'eating ' + food
Which works, and why?
>>> def eat(food):
... print 'eating'
... print food
File "<stdin>", line 3
print food
^
IndentationError: unexpected indent
>>> def eat(food):
... print 'eating ' + food
File "<stdin>", line 2
print 'eating ' + food
^
IndentationError: expected an indented block
Step 04:01
How can I make a program to print:
"it's hot" if the temperature is more than 70
"it's cold" if the temperature is less than 50
"nice and ca" if the temp. is more than 50, less than 70
you need control statements!
Control statements tell the program how to flow,
ie. what is executed next.
They're pretty simple:
if (temperature > 50):
print "it's hot!"
Finished program:
if (temperature > 80):
print "it's hot!" elif (temperature < 60): print "it's cold!" else: print "nice in ca!"
Can we make improvements?
Any ideas given what you know about python?
improvements!
def analyze_temperature(temperature):
if (temperature > 80):
print "it's hot!"
elif (temperature < 60):
print "it's cold!"
else:
print "nice in ca!"
analyze_temperature(int(raw_input('What temperature is it?')))
Step 05:00
Let's supercharge our last example:
Given magical functions to get your location and the temp,
how would you use the improved program to automatically print the weather like:
python print_the_weather.py
it's a nice ca day!
bye!
Let's see:
# file: print_weather_now.py
location = find_my_location()
weather = download_weather_now()
temp = weather.temperature
analyst_string = analyze_temperature(temp)
print 'It's %i today, %s' % (temp, anaylst_string)
print 'bye!'
imports!
import antigravity
-
imports let you import libraries, functions of collections that can do exciting things easily.
-
you can install your own libraries, and use the ones included as well
-
the best way to install new libraries is to use the `pip` tool included with most pythons.
-
if you don't have pip, use easy_install to install pip `sudo easy_install pip`
-
then you're on your way!
import urllib2
import json
req = urllib2.urlopen('http://freegeoip.net/json/')
req_str = req.read()
req_json = json.loads(req_str)
my_zip = req_json['zipcode']
print 'Your zipcode is ' + my_zip + ' in ' + req_json['city'] + ' ' + req_json['region_name'] + '.'
# or:
print('Your zipcode is %s in %s %s' % (my_zip, req_json['city'], req_json['region_name']))
Full result:
import urllib2
import json
def get_location():
req = urllib2.urlopen('http://freegeoip.net/json/')
req_str = req.read()
return json.loads(req_str)
def get_todays_weather(coords):
req = urllib2.urlopen('http://api.openweathermap.org/data/2.5/weather?lat=%s&lon=%s' % (coords['latitude'], coords['longitude']))
req_weather_str = req.read()
weather_data = json.loads(req_weather_str)
return weather_data
def convert_to_farenheit(kelvin):
#(ºK- 273.15)* 1.8000 + 32.00
return (float(kelvin) - 273.15) * 1.8000 + 32.00
def analyze_weather(temp): print "It's %.2f today" % temp
todays_weather = get_todays_weather(get_location())
temp_in_kelvin = todays_weather['main']['temp']
weather_anaylsis = analyze_weather(convert_to_farenheit(temp_in_kelvin))
Step 06:00
Let's make a GUI!
ps, first download magic.py (`wget http://ow.ly/w2i2f`)
import magic
manager = magic.WindowManager()
manager.add_title('Your current location:')
loc = magic.get_location()
manager.add_dict(loc)
# >>> loc
# {u'city': u'Los Angeles', u'region_code': u'CA', u'region_name': u'California', u'ip': u'68.181.240.25', u'area_code': u'213', u'zipcode': u'90089', u'longitude': -118.2987, u'metro_code': u'803', u'latitude': 33.7866, u'country_code': u'US', u'country_name': u'United States'}
What just happened?!?
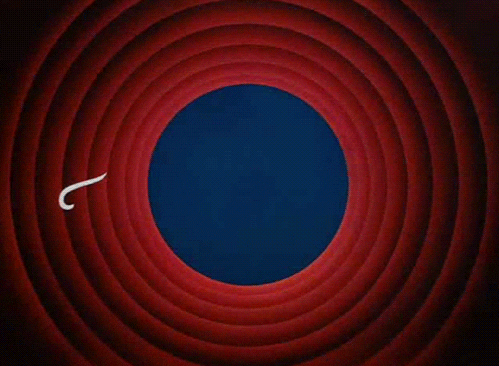
Questions?
iain.nash@usc.edu
Python: INTRO
By Iain Nash
Python: INTRO
- 1,525