webpack (4)
1-0-1
Why ?

Gulp and Grunt are JavaScript task runners, while Webpack is a bundler.
Webpack takes all the code from your application and makes it usable in a web browser.
webpack


Agenda
- Entry
- Output
- Loaders
- Plugins
- Mode
- Dev-Server
- Zero-config
Entry
An entry point indicates which module webpack should use to begin building out its internal dependency graph.

Single Entry

As a rule of thumb: Use exactly one entry point for each HTML document.
Multi Page Application
Output
Configuring the output configuration options tells webpack how to write the compiled files to disk. Note that, while there can be multiple entry points, only one output configuration is specified.

Usage

Multiple Entry Points
Custom functions
//src/client/index.js
import { handleSubmit } from './js/formHandler'
import { urlChecker } from './js/urlChecker'
export {
handleSubmit,
urlChecker
}
//webpack.[ENV].js
entry: './src/client/index.js',
output: {
libraryTarget: 'var',
library: 'Client'
},
<form class="" onsubmit="return Client.handleSubmit(event)">
Loaders
What are loaders?
- Loaders are kind of like “tasks” in other build tools and provide a powerful way to handle front-end build steps.
- Loaders can transform files from a different language (like TypeScript) to JavaScript or load inline images as data URLs.
- Loaders even allow you to do things like import CSS files directly from your JavaScript modules!
Install & use
npm install --save-dev css-loader ts-loader

Multiple loaders
Loaders are evaluated/executed from right to left (or from bottom to top).

Loader Features
- Loaders can be chained. Each loader in the chain applies transformations to the processed resource.
- Loaders can be synchronous or asynchronous.
- Loaders run in Node.js and can do everything that’s possible there.
- Loaders can be configured with an options object.


Loaders
Plugins
What are plugins?
While loaders are used to transform certain types of modules, plugins can be leveraged to perform a wider range of tasks like bundle optimization, asset management and injection of environment variables.
Install & use

npm install --save-dev html-webpack-plugin

Plugins
Mode
What is Mode ?
Mode enables webpack's built-in optimizations that correspond to each environment. The default value is production.

Supported values

Dev Server
What is dev-server ?
The webpack-dev-server is a little Node.js Express server, which uses the webpack-dev-middleware to serve a webpack bundle. It also has a little runtime which is connected to the server via Sock.js.
Install & Use
npm install webpack-dev-server.
//package.json
{
"scripts":{
//.....
"build-dev": "webpack-dev-server --config webpack.dev.js --open",
}
}
Zero config
What is zero config ?
no webpack.config.js
Install & Use
npm install webpack webpack-cli
<!DOCTYPE html>
<html>
<body>
<script src="main.js"></script>
</body>
</html>
//src/index.js
document.write("From inside webpack zero config!")
webpack //generates dist/index.html
Production configuration
"build-prod": "webpack --config webpack.prod.js",
webpack.prod.js
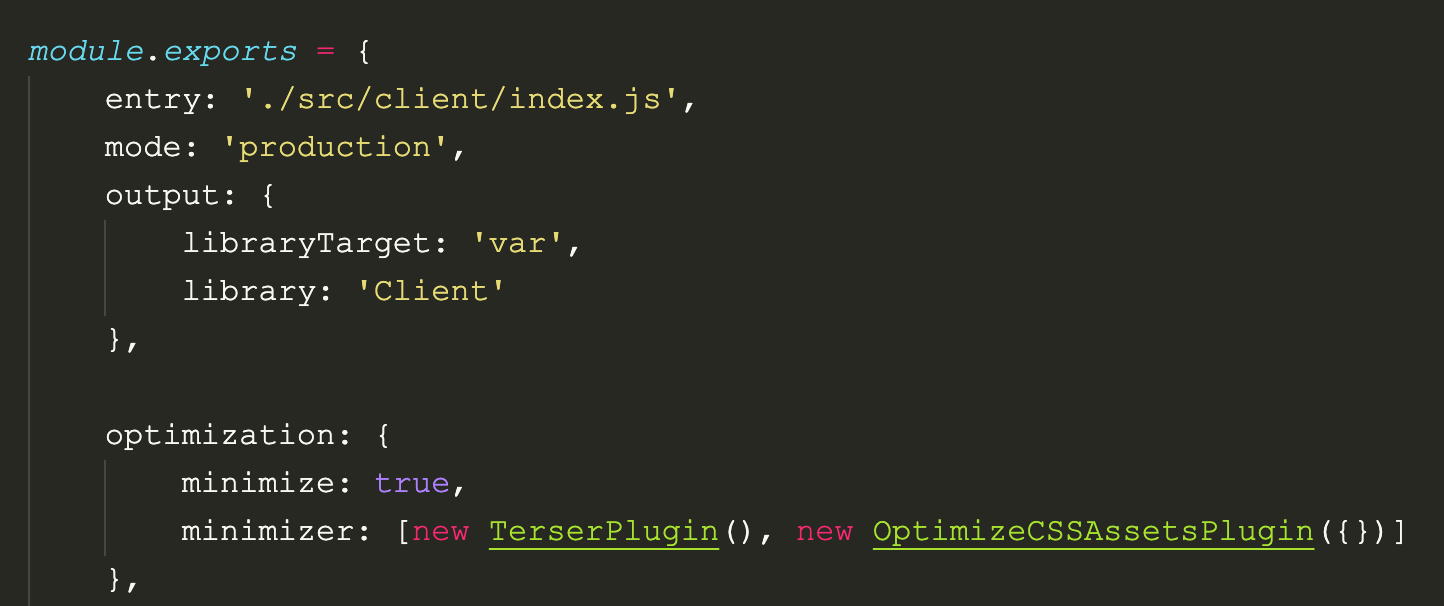
Terser: JavaScript parser, mangler and compressor toolkit
OptimizeCSS: webpack plugin to optimize \ minimize CSS assets.

webpack.prod.js

webpack.prod.js
Browser & Node compatibility
Browser Compatibility
- webpack supports all browsers that are ES5-compliant (IE8 and below are not supported).
- webpack needs Promise for import().
- If you want to support older browsers, you will need to load a polyfill before using these expressions.
Node Environment
webpack runs on Node.js version 8.x and higher.
DEMO
Thank you!
Webpack 101
By Dragosh
Webpack 101
Smooth introduction into webpack tool.
- 222