WHILE
Loops
WHILE Loops
As Python code:
again = 'yes'
while again == 'yes':
print('Hello')
again = input('Do you want to loop again? ')
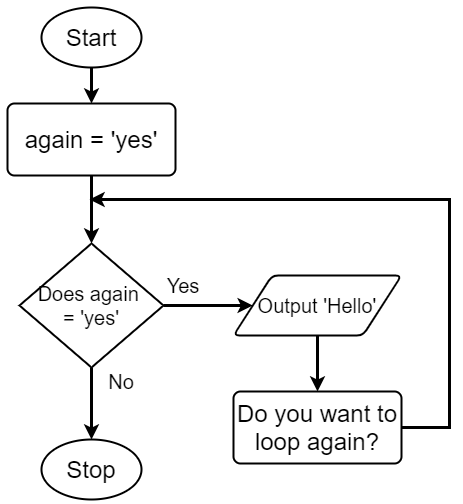
Comparison & Logic Operators
These are used all the time with WHILE loops
Operator | Description |
---|---|
== | equal to |
!= | not equal to |
> | greater than |
< | less than |
>= | greater or equal to |
<= | less or equal to |
Operator | Description |
---|---|
and | both conditions must be met |
or | either condition must be met |
Comparison operators
Logical operators
Example I
A WHILE loop to keep adding number until the value is 100 or more.
# 1 WHILE Loop to sum numbers up to 100
total = 0
while total < 100:
num = int(input('Enter a number: '))
total = total + num
print(f'The total is {total}')
Example II
Using a WHILE loop to verify user input
# 2 Enforcing correct user input
name = ''
while name != 'the greatest':
name = input('Who are you? ')
print(f'You are {name}!')
Tasks I
- Set the total to 0 to start with. While the total is 50 or less, ask the user to input a number. Add that number to the total and print the message "The total is... [total]". Stop the loop when the total is over 50.
- Ask the user to enter a number. Keep asking until they enter a value over 5 and then display the message "The last number you entered was a [number]" and stop the program.
- Ask the user to enter a number and then enter another number. Add these two numbers together and then ask if they want to add another number. If they enter "y", ask them to enter another number and keep adding numbers until they do not answer "y". Once the loop has stopped, display the total.
Tasks II
- Ask for the name of somebody the user wants to invite to a party. After this, display the message "[name] has now been invited" and add one to the count. Then ask if they want to invite somebody else. Keep repeating this until they no longer want to invite anyone else to the party and then display how many people they have coming to the party.
- Create a variable called compnum and set the value to 50. Ask the user to enter a number. While their guess is not the same as the compnum value, tell them if they guess is too low or too high and ask them to have another guess. If they enter the same value as compnum, display the message "Well done, you took [count] attempts".
Tasks III
- Ask the user to enter a number. If they enter a value under 10, display the message "Too low" and ask them to try again. If they enter a value above 20, display the message "Too high" and ask them to try again. Keep repeating this until they enter a value that is between 10 and 20 and then display the message "Thank you".
Tasks IV
- Using the song ten green bottles, display the lines "There are [ num] green bottles hanging on the wall, [num] green bottles hanging on the wall and if one green bottle should accidentally fall". Then ask the question "How many green bottles will be hanging on the wall?" If the user answers correctly, display the message "There will be [num] green bottles hanging on the wall." If they answer incorrectly, display the message "No, try again" until they get it right. When the number of green bottles gets down to 0, display the message "There are no more green bottles hanging on the wall.”
F WHILE Loops
By David James
F WHILE Loops
Python - WHILE Loops
- 1,057