Agile
Learning Objectives
Software Development Cycle
Agile Methodologies
User Stories
Trello
Minimal Viable Product
Learn and Understand:
The Software Development Cycle
The SDLC is the software development life cycle, also referred to as the application development life-cycle. It is a term used to describe a process for planning, creating, testing, and deploying an information system.
The Software Development Cycle
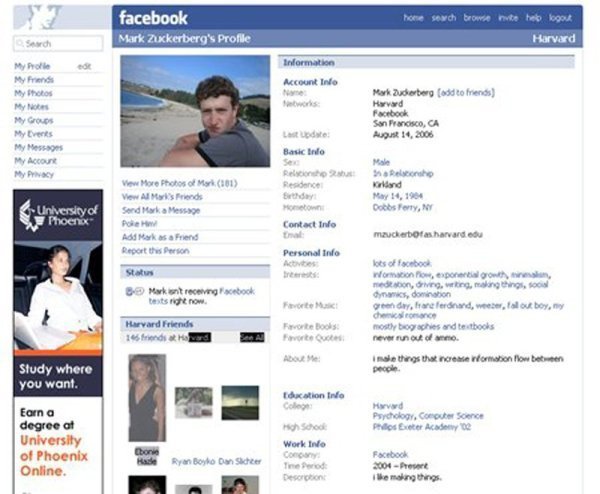
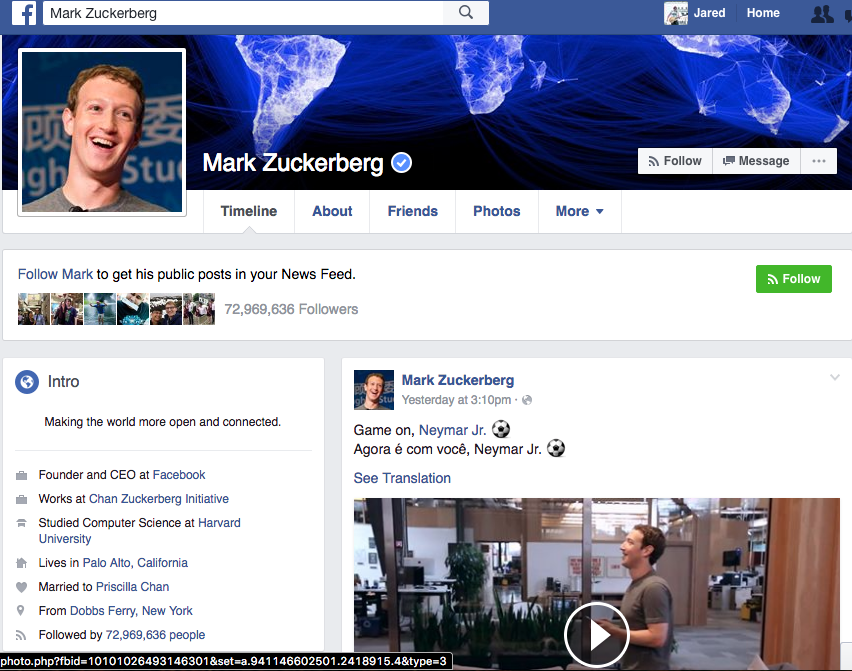
The Software Development Cycle
All of these changes to the User Interface as well as the functionality of the site did not happen overnight.
Development is not just about coding. Planning, preparing, discussion, and iteration is in the lifeblood of web developers. It's integral to how effective development happens.
We can strategize and describe the SDLC with Software Development Processes, such as Agile.
A (really short) History of Software Development Processes
Waterfall Development
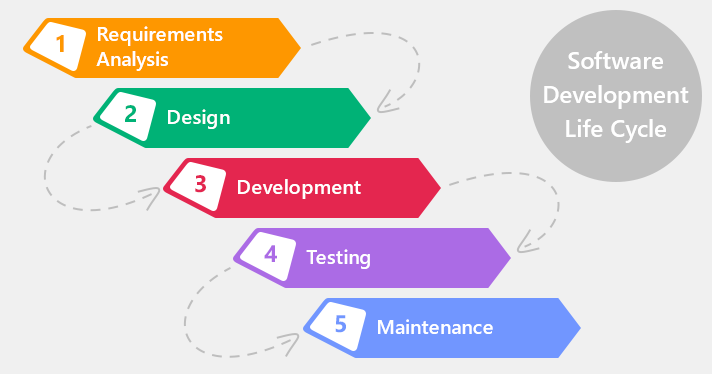
(Traditional Approach)
Waterfall Development
(continued)
Complete phase 1 ( ex. design) BEFORE moving on to phase 2 ( ex. development)
Rarely revisit a phase once it is completed, which means - you better get it right the first time!
Highly risky, less efficient, and often more costly than Agile approaches
Waterfall Development
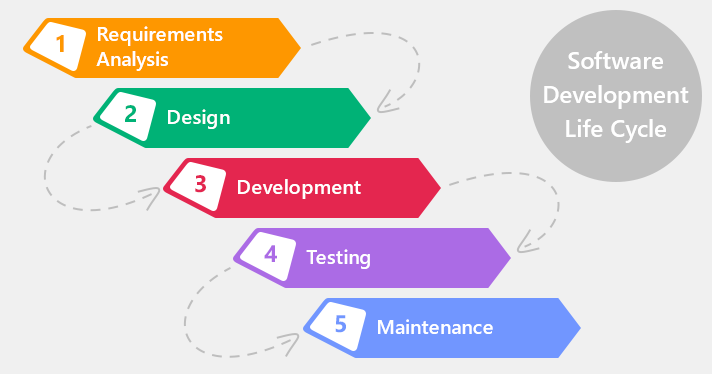
Problems!
Changes
Takes too long
Skipped
AGILE
Rapid
Quality-Driven
Not quite a process, more like a philosophy or a set of values
Adaptable
Iterative
Cooperative
Agile Development
Agile software development is a group of software development methods in which requirements and solutions evolve through collaboration between self-organizing, cross-functional teams.
"The Agile movement is not an anti-methodology, in fact many of us want to restore credibility to the word methodology. We want to restore balance."
- Agile Manifesto
Agile Development
- Self-organization, motivation, and interactions like pair programming are important
- Working software is more useful and welcome than just presenting documents to clients in meetings
- Requirements cannot be fully collected at the beginning of the SDLC, therefore continuous customer involvement is key
- Agile methods are focused on quick responses to change and continuous development
Agile Development
The Basic Implementation of Agile workflow is rapid development based on quick iterations known as sprints. Traditionally sprints can last anywhere from 1-4 weeks. Development teams build trust in their clientele/customer base by delivering early and often.
This also provides the additional benefit of allowing for steering. Changing the direction of the project on a per iteration basis if need be.
What Does This Mean for You?
For your projects you can structure your own sprints in even smaller increments. Maybe even 1 or 2 days. Perhaps shorter!
When planning sprints for your project, set concrete goals for yourself. "I am going to write out the logic for selecting cards by 12:30 so that I can get started on changing the styling of selected cards after lunch."
Traditionally during project weeks you'll have time to work from home. It is VITAL that you guys schedule yourselves well.
Exercise
You are tasked with setting goals for the Tic-Tac-Toe game we made yesterday in class. Write out your goals in sprints, with specific deadlines and with a total timeframe of 2 days.
Consider each part of making the game including writing pseudocode, styling, all parts of the game logic, animations, and anything else you would include in your game.
Work with a partner to plan out your sprints. What should you start with? How long will you need to spend on each part?
Minimal Viable Product
Minimal Viable Product, or MVP, is essentially the bare bones of a project. It is all of the features and functionality that are required in order to deliver a product that meets its purpose.
Think of a bronze, silver and gold medals. Silver is what you're trying to make, but if you fall short on time you have your bronze you can fall back on. If you have enough time, you could go for the gold medal. Your project MVP should be that silver medal.
Minimal Viable Product
SLACK-
A site that allows users to sign in and create private chat channels, with both group and private messaging.
SPOTIFY-
An app that allows users to sign in, search for music, maintain playlists, and play music.
User Stories
In order to work towards our MVP, we write user stories.
User stories are essentially small granular features for your application. They have:
- a role
- a goal
- a reason
As a <role> I should be able to <goal> so that <reason>
User Stories
User stories should be granular and succinct
Good User Story:
As a student, I should be able to send private messages so I can let my instructors know how awesome they are
Bad User Story:
This app should have a really good social networking engine
User Stories
To create an analogy, let's look at some user stories that would make sense for a movie theater:
As a customer, I should be able to see the list of movies and the times they are playing so I can decide on a showing.
As an employee, I should be located near the list of movies so I can assist customers purchase tickets
As a projectionist, I should be able to access the movie reels and the projection booth so I can start the movie
Exercise
In groups, discuss as many user stories for your application as you can. Think about how users interact with each function of the app separately ( ex: group messages are a different user story than private ones). Write out the MVP's for your app. What are its most essential features? Whiteboard at least 10 user stories, which ones are MVP features?
Facebook - Table 1
LinkedIn - Table 2
Gmail - Table 3
Github - Table 4
Trello
User stories are great, but wouldn't it be nice if there was a great place to store and track them all? There is! It's called trello. There are other tools out there, but we recommend this one. I've used it in production, and we are using it to plan this class!
Quickly sign up for a trello account if you don't have one. Then:
- Create a Trello Board for Project 1
- Create cards to keep track of user stories for your game
Wireframes
A wireframe is a simple blueprint/template/sketch/visual outline of the components of your website. There are great digital wireframing tools out there, but all you need really is a piece of paper and something to write with.
Creating a wireframe can be very useful for planning your project, as it allows you to sketch a rough design before you start coding. This removes the burden of coming up with big picture styling as you code
Backlog and Iceboxes
A backlog is a list of features which the team maintains and at the moment are known to be necessary and sufficient to complete a project.
If an item does not contribute to the project's goal, it should be removed. If a feature becomes known that turns out to be necessary to the project, it should be added go the backlog
The icebox is where all your ideas go that you don't currently plan to build. Does'nt mean you can't thaw them out later but they are not current necessities for your project
Coding Best Practices
Indentation
Proper Indentation is crucial for debugging and thinking about how to complete your next step.
It is also very helpful for 'seeing' the scope of a variable and organizing functions.
Coding Best Practices
Bad Indentation
var arr = [1,3,5];
if (arr[0] % 2 !== 0) {
console.log(arr[0]);
} else if (arr[1] % 2 !== 0)
{console.log(arr[1]);} else if (arr[2] % 2 !== 0) {
console.log(arr[2]);}
Glorious, Wondrous, Friendly
var arr = [1,3,5];
if (arr[0] % 2 !== 0) {
console.log(arr[0]);
} else if (arr[1] % 2 !== 0) {
console.log(arr[1]);
} else if (arr[2] % 2 !== 0) {
console.log(arr[2]);
}
Coding Best Practices
D.R.Y. Code
DRY (do not repeat yourself) is a way developers describe code that is reusable and is no more repetitive than it needs to be.
Writing Dry code is tremendously helpful for reading, writing, and debugging your code. It also makes things much simpler, and makes changes much easier to implement.
Coding Best Practices
Not D.R.Y.
var arr = [1,3,5];
if (arr[0] % 2 !== 0) {
console.log(arr[0]);
}
if (arr[1] % 2 !== 0) {
console.log(arr[1]);
}
if (arr]2] % 2 !== 0) {
console.log(arr[2]);
}
DRY
var arr = [1,3,5];
arr.forEach(function(number) {
if (number % 2 !== 0) {
console.log(number);
}
});
Coding Best Practices
Appropriate Variable Names
Vague or nonsensical variable names can make writing and debugging your code extremely difficult.
Yes, sometimes it will take a few extra characters. But, having appropriate and descriptive variable names will always save you time and headaches.
Coding Best Practices
Bad Variable Names
var x = "Flannel";
var arr = "Flannel";
var person = "Flannel";
var Bryan = "Flannel";
Magical, Benevolent, Soulful
var shirtType = "Flannel";
var str = "Flannel"
var person.top = "Flannel";
var Bryan = "Flannel";
Conclusion
We have just had a crash course in some of the basics involved in the web development process.The important thing for this course is how we use these principles and methodologies to help us plan for, and work efficiently on our projects
Take Aways:
Agile
MVP
User Stories
Wireframming
Coding Best Practices
Agile
By Jared Murphy
Agile
- 527