Game development in React & ReactNative
- Jasbir Singh Shergill

Hey there!
My name is Jasbir
Freelancer, ex. Myntra, ex. GeekyAnts
Oh yea! 😎Â
Into Games & stuff
Some live demos

Pipers - svg based modern snake game
RocketGame - Endless Runner

Why use the web ?
All concepts of game development used here have counterparts in every platform.
Web makes your game cross-platform.
Easy to share / distribute game.
Easy to maintain / update.
Can build light-weight & high performance games.
Game Loop - the fundamental concept
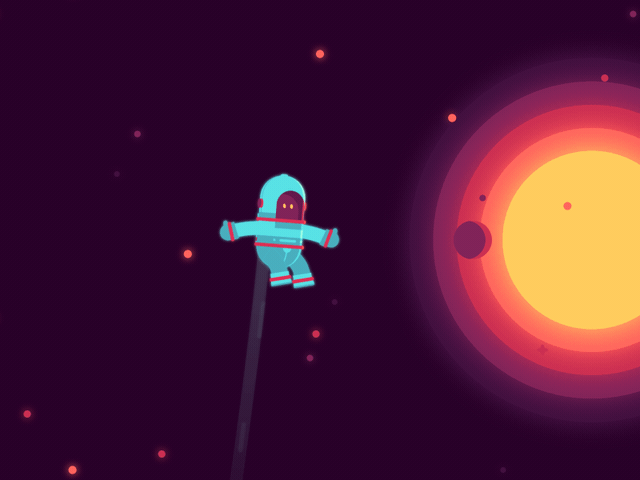
Simple game loop in React
runGameLoop() {
// update logic here
// collision detection here
this.gameLoop = requestAnimationFrame(this.runGameLoop);
}
// Start the loop
this.gameLoop = requestAnimationFrame(this.runGameLoop);
requestAnimationFrame vs setInterval
Collision detection
isColliding(a, b) {
return (
a.left <= b.right &&
b.left <= a.right &&
a.top <= b.bottom &&
b.top <= a.bottom
);
}
Bounding rectangle intersection check
inside(point, vs) {
var x = point[0], y = point[1];
var inside = false;
for (var i = 0, j = vs.length - 1; i < vs.length; j = i++) {
var xi = vs[i][0], yi = vs[i][1];
var xj = vs[j][0], yj = vs[j][1];
var intersect = ((yi > y) != (yj > y))
&& (x < (xj - xi) * (y - yi) / (yj - yi) + xi);
if (intersect) inside = !inside;
}
return inside;
}
// array of coordinates of each vertex of the polygon
var polygon = [ [ 1, 1 ], [ 1, 2 ], [ 2, 2 ], [ 2, 1 ] ];
inside([ 1.5, 1.5 ], polygon); // true
Ray casting algorithm to detect if a point lies inside a polygon
Using Lottie for modular animations

Using ThreeJS for 3D rendering
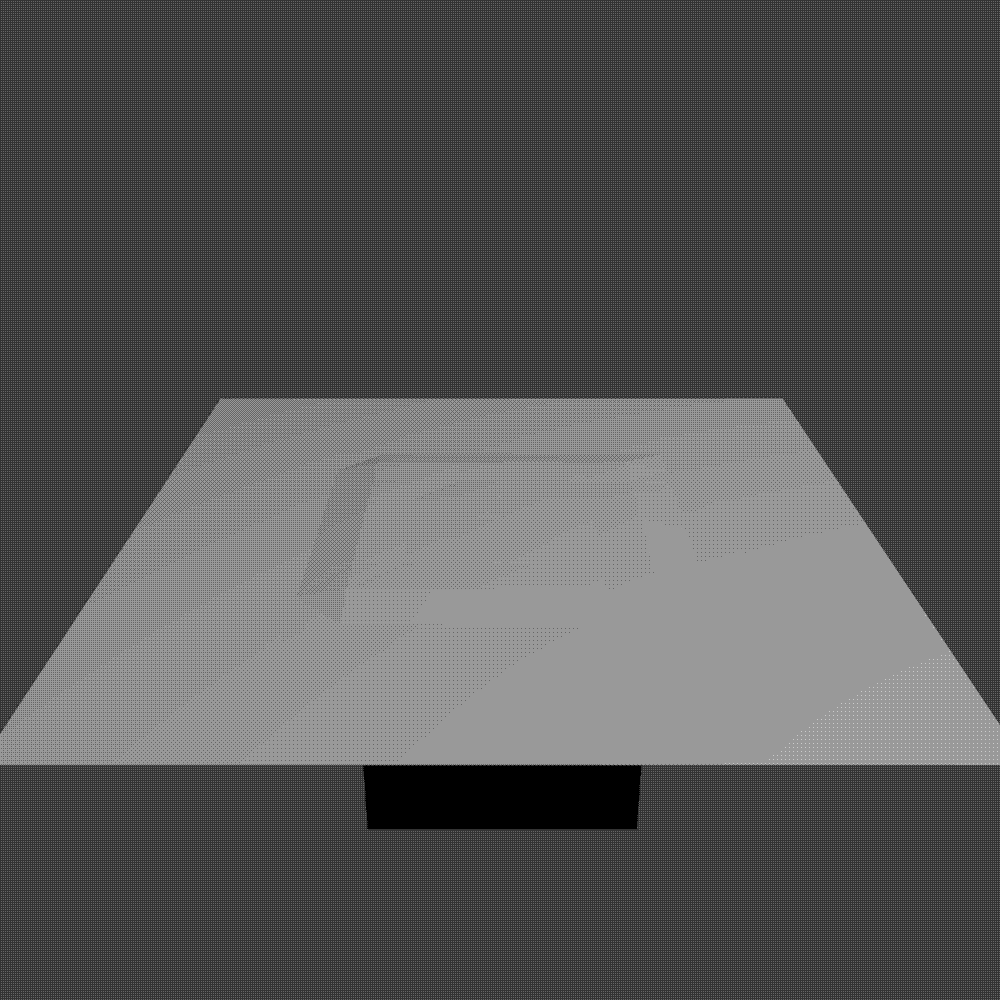
Key points ThreeJS
Much more powerful as uses GPU
Wherever actual 3D graphics is required ThreeJS becomes necessary :- changing perspectives, camera positions, change in object view angles
You need sooo... much math !
External 3D assets can get large and must be optimised
BookCricket with Myntra

The Hack! Making 2D lottie look like 3D
Be sure to use HTML renderer for 3D Lotties to preserve perspective transforms
Tips & Tricks for optimal performance
Single game loop, all update logic must go here itself
Keep re-renders as few as possible
Wherever possible, use CSS for animations
All computations inside game loop should be highly optimised. Keeping low time complexity should be the goal
Checkout my blog
Thank You!
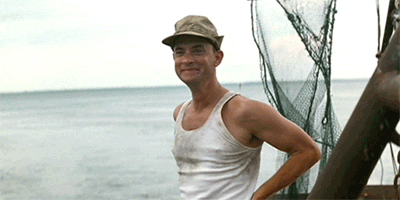
Game develop
By jasbir singh
Game develop
- 466