Building JavaScript with JavaScript
or
"Drinking your way to sane automation"
TWeet: @_joelgriffith_
e-mail: joel@joelgriffith.net
GITHUB: joelgriffith
The Master Plan
JS
Pacakge all scripts into a single file. Build a DEV and PROD version
Run unit tests in all relevant browsers when code changes
See changes in brower w/o reloading
Resolve requires() to node_modules, bower_components, or locally. Support ES6
BIG GULPS, HUH?

Webpack Module Management
- Can compile just about any file/JS type
- Ability to write custom loaders
- Why YES you can use ES6, NPM, Bower, coffe...
// WOW, ES6!
export default class Person {
constructor(name) {
this.name = name;
}
getName() {
let name = this.name;
console.log(name);
}
}
import Person from 'person';
var joel = new Person('Joel');
joel.sayName() // Prints: 'My Name is Joel'
var webpackConfig = {
resolve: {
modulesDirectories: ['node_modules', 'bower_components', 'yourJsFile']
},
module: {
loaders: [{
test: /\.js$/,
loader: 'es6-loader'
}]
}
};
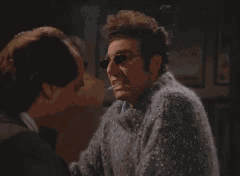
Karma test-runner
- Can use preprocessors for compiling before tests
- Awesome CI via file watch
- Really just a http/websocket server, easy to test external browsers (virtual, emulated, whatevs).
// Build the codez before you runs it!
var karmaConfig = {
preprocessors: {
'**/*.js': ['webpack']
},
// SauceLabs Browsers!
customLaunchers: {
ie6: {
base: 'SauceLabs',
browserName: 'internet explorer',
platform: 'Windows XP',
version: '6'
},
android4: {
base: 'SauceLabs',
browserName: 'android',
platform: 'linux',
version: "4.0"
},
},
// Test framworks
frameworks: ['mocha', 'chai'],
// list of files / patterns to load in the browser
files: [
"*.spec.js"
],
}

Gulp for task running
- Tasks are functions
- A lot less object literals
- Doesn't need to be a gulp plugin to work
- Streams are fast, and drinkable.
- It's just JavaScript!
// Compile dat JS, push to LiveReload
gulp.task('scripts', ['clean'], function() {
return gulp.src(['index.js'])
.pipe(changed())
.pipe(webpack(webPackConfig))
.pipe(gulp.dest('dev/js'))
.pipe(connect.reload())
.pipe(uglify())
.pipe(gulp.dest('build/js'));
});
// CI Testing Task
gulp.task('test', function() {
return gulp.src(karmaConfig)
.pipe(karma({
browsers: ['Chrome', 'Firefox', 'ie6', 'android4'],
configFile: 'karma.conf.js',
action: 'watch'
}));
});
// Watch file changes
gulp.task('watch', function() {
gulp.watch(['**/*.js'], ['scripts']);
});
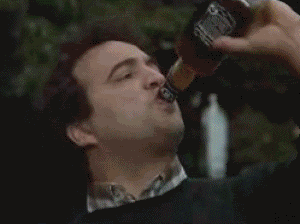
But wait, I want moar
- Image compression?
- CSS building/prefixin'/manglin'?
- Some testing sugar (mocks, DI, proxies)?
- Externalization w/o deploying?

to be continued
Get more in early October @ AppNexus
Building JavaScript with JavaScript
By Joel Griffith
Building JavaScript with JavaScript
Having recently built some automated tools for a work project, I found that you *can* have beer and drink it too! This deck explore some of the technologies used to get me to build nirvana.
- 1,231