Coding Style
蔡銘軒 @ Sprout 2021 C/C++語法班
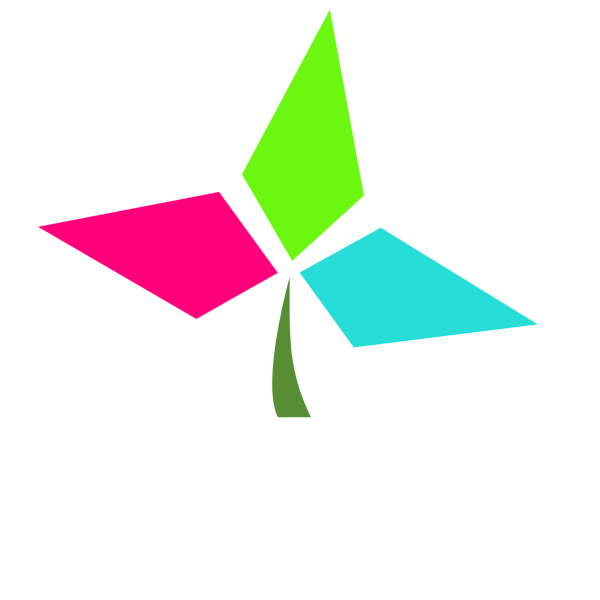
Coding Style?
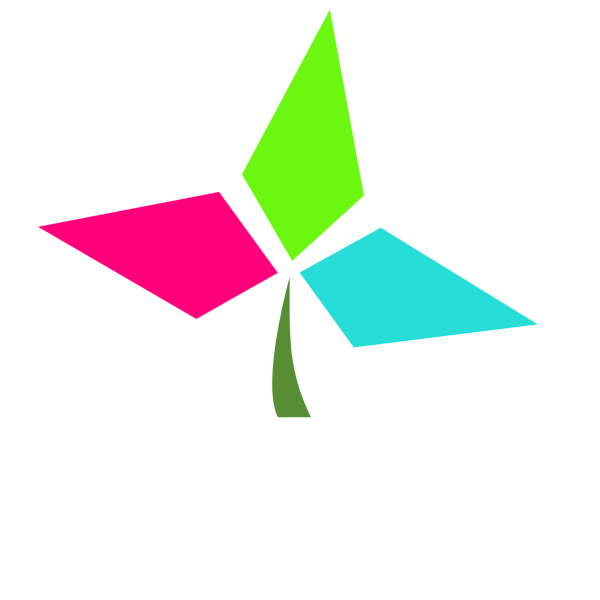
這是一堂輕鬆的課
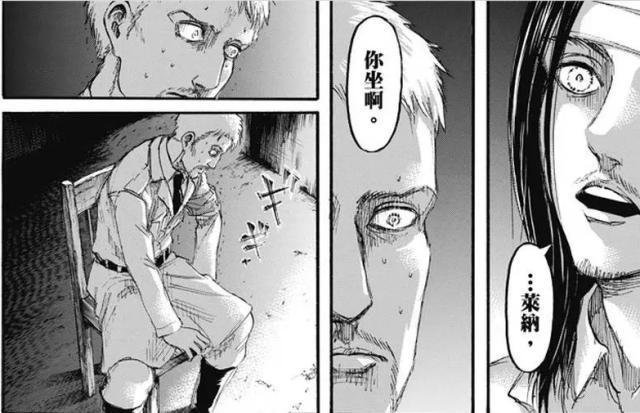
Coding Style?
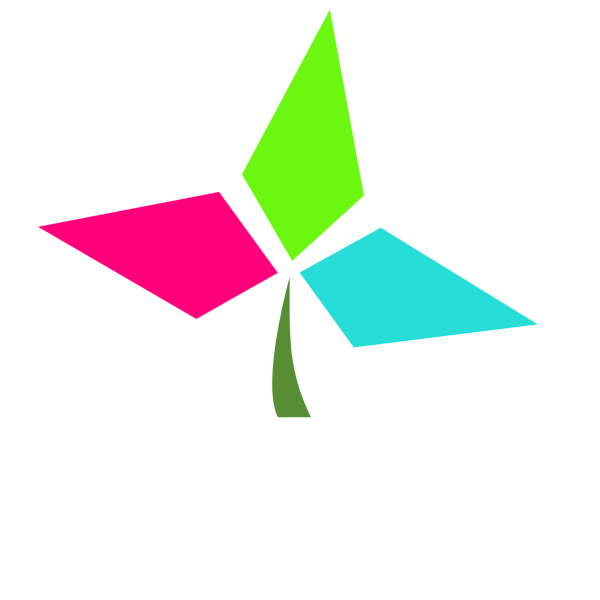
這是一堂教你寫出更好的Code的課
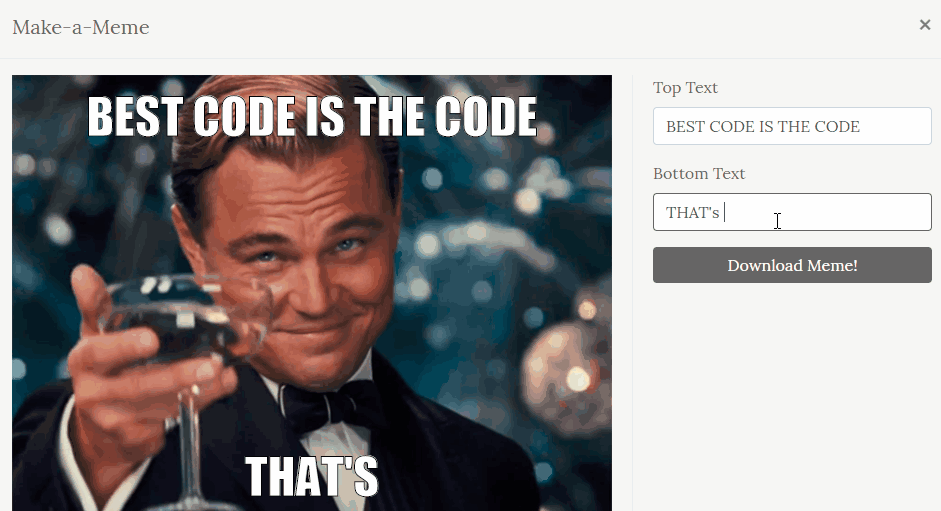
Coding Style?
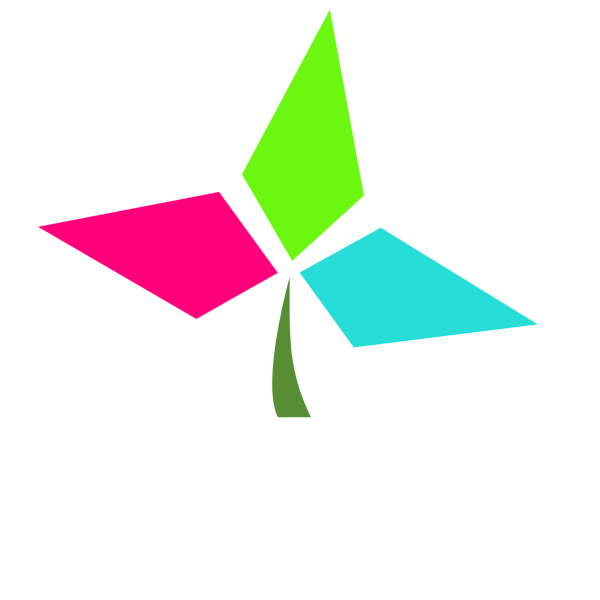
這是一場宗教戰爭
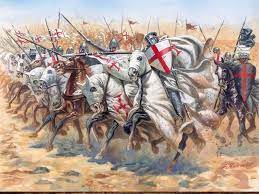
Naming Convention
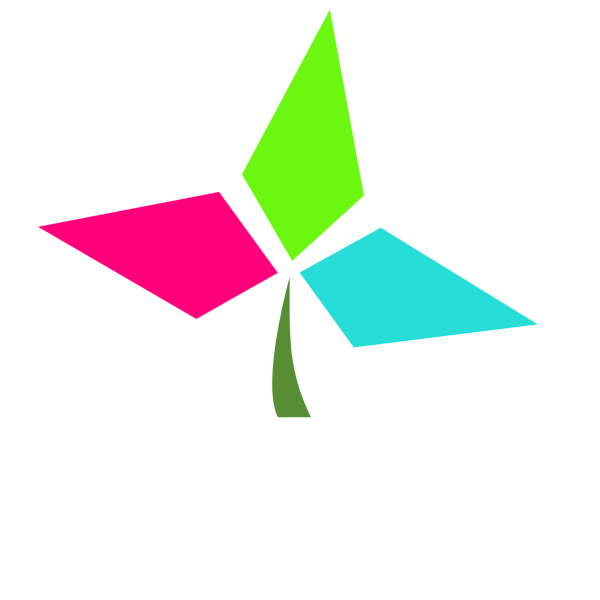
為變數與函式取有意義的名字
double func(double a) {
const double b = 3.14;
return a * a * b;
}
double get_circle_area(double radius) {
const double PI = 3.14;
return radius * radius * PI;
}
Naming Convention
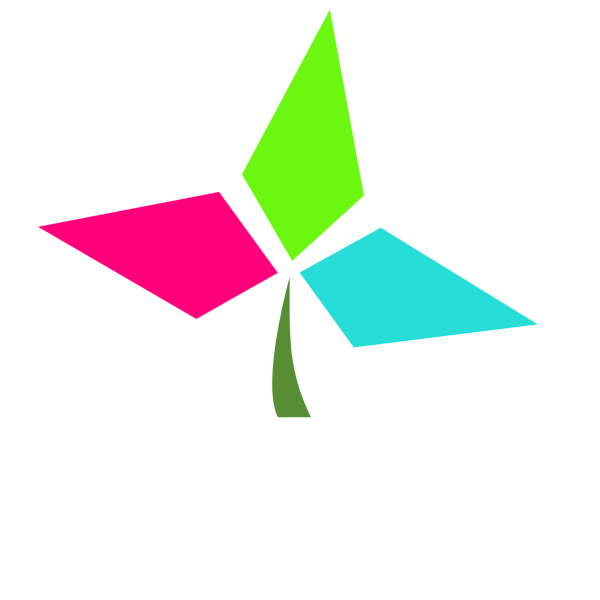
同時遵守一些潛規則
for (int i = 0; i < n; i++) {
std::cout << "this is ok\n";
}
例:在 for loop 裡使用 i, j, k
Naming Convention
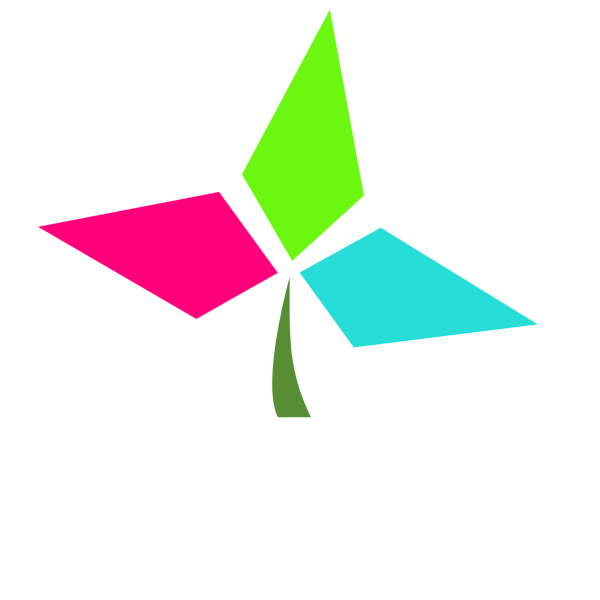
兩大教派
under_score
camelCase
int get_area();
int getArea();
int student_count;
int studentCount;
Naming Convention
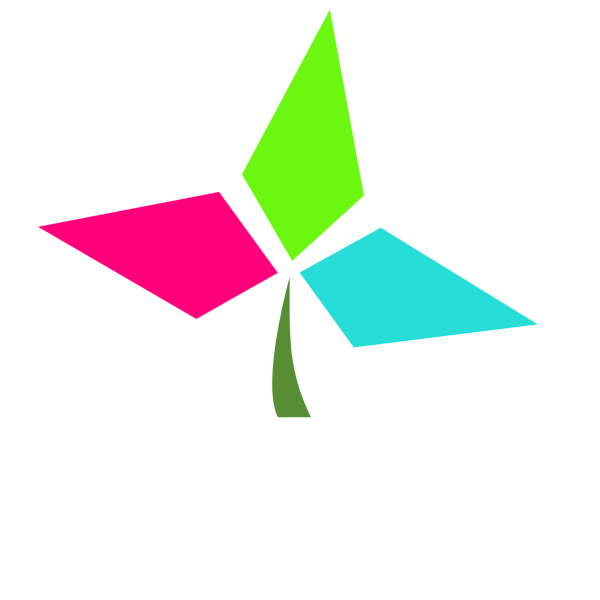
只有差異,沒有優劣
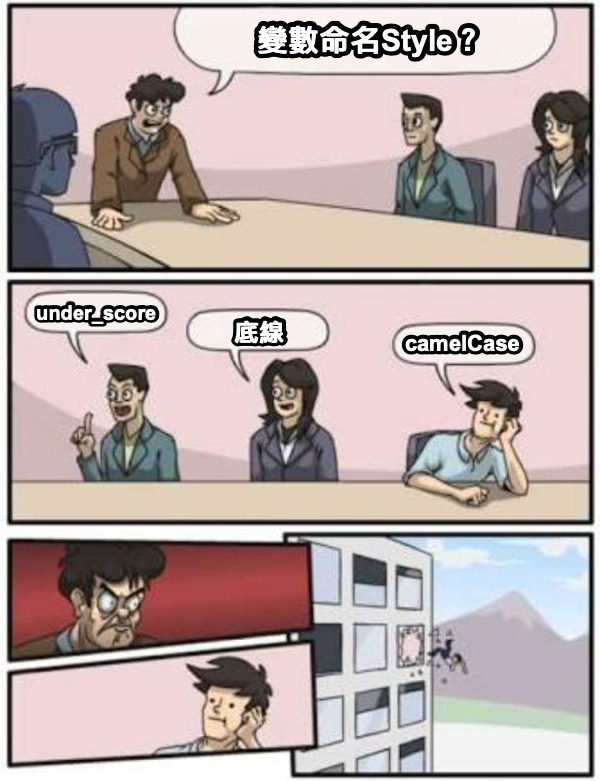
Naming Convention
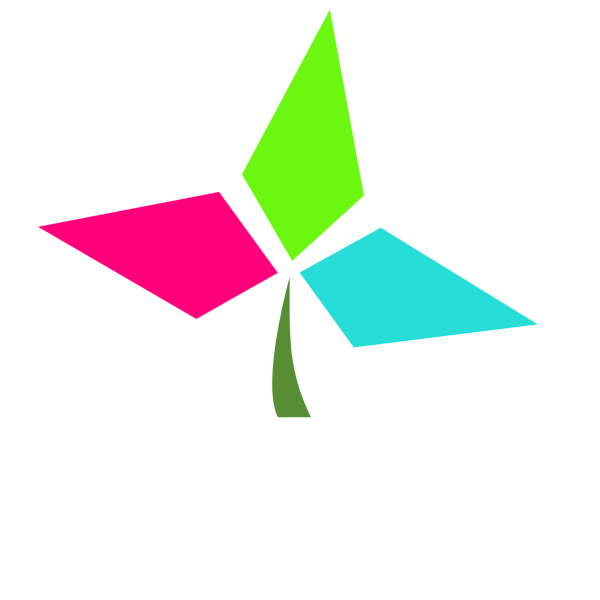
只有差異,沒有優劣
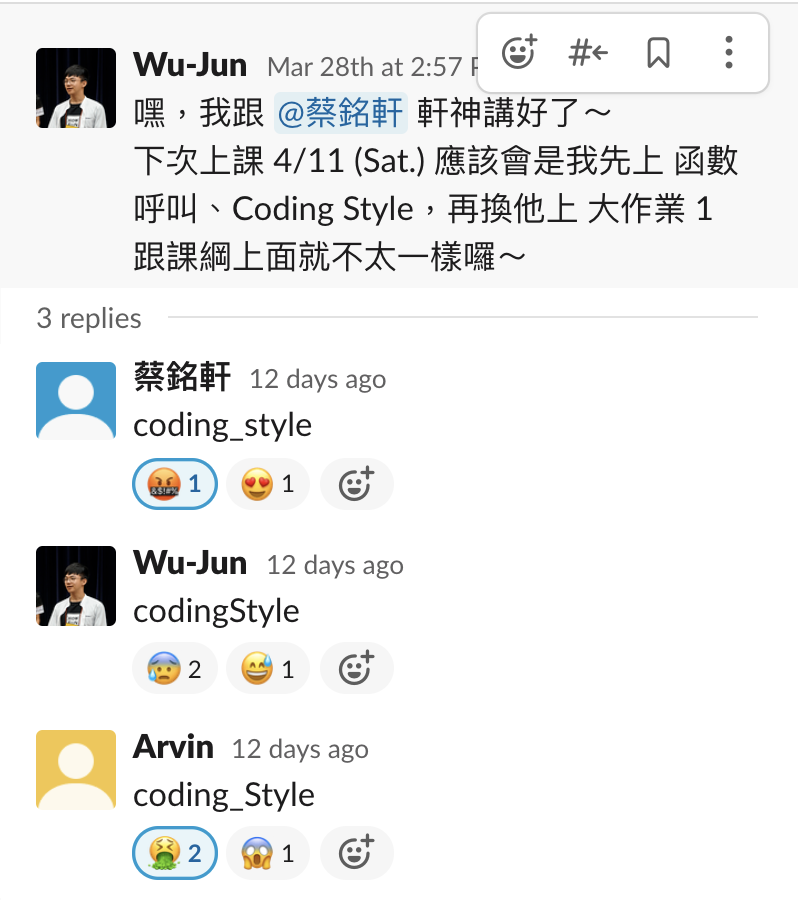
2020/3/28
2021/3/6
裴裴(Wu-Jun):
我現在好像覺得底線比較好
Naming Convention
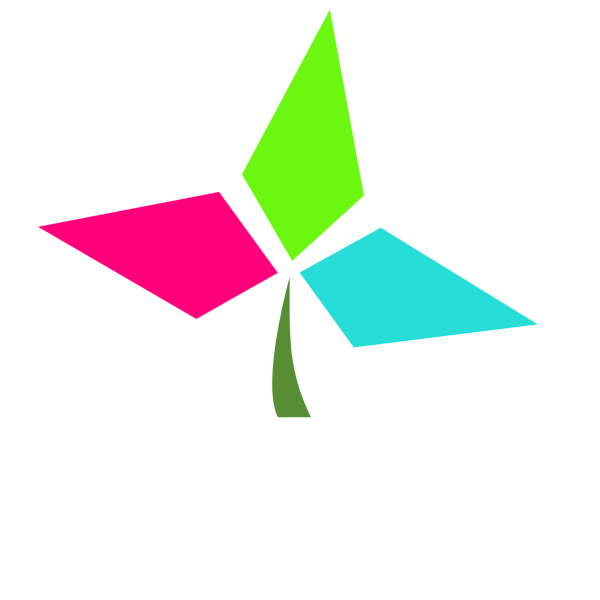
言歸正傳
根據 Google Coding Style Guide
函式名稱用camelCase
變數名稱用under_score
void demoFunction(int demo_variable) {
int other_variable;
/* do something */
return;
}
Naming Convention
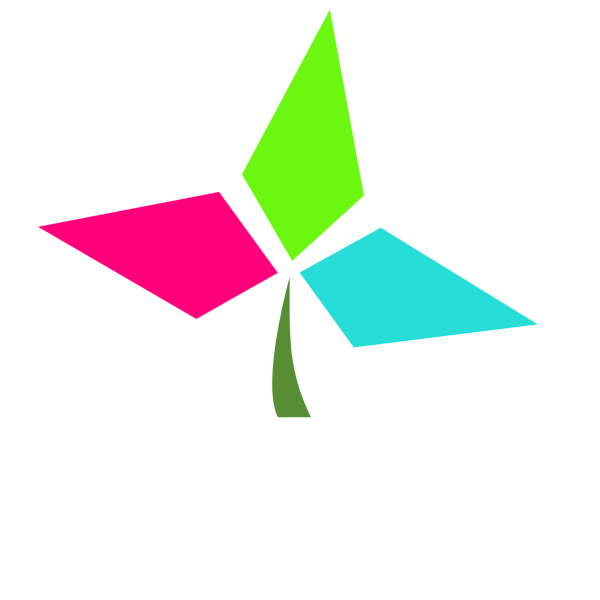
關於camelCase
camelCase還可以分成大/小駝峰
大駝峰: CamelCase
小駝峰: camelCase
根據 Google Coding Style Guide
class 的名稱使用大駝峰
Naming Convention
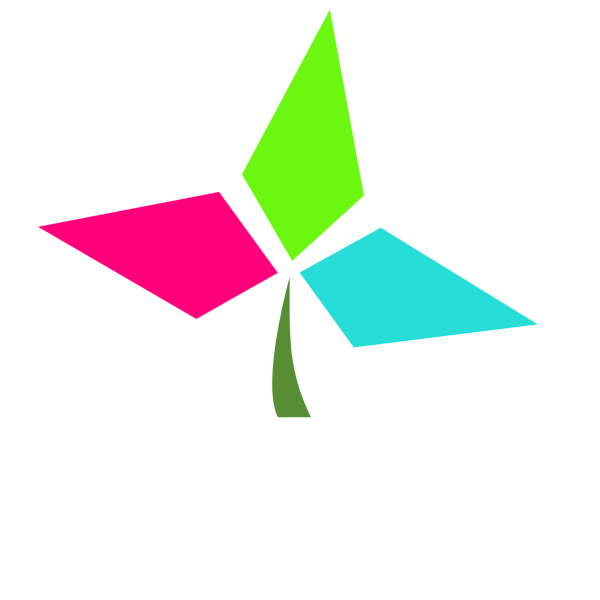
原則
一致性
整份程式使用相同的命名方式
可讀性
int thisIs_aJoke();
Spacing
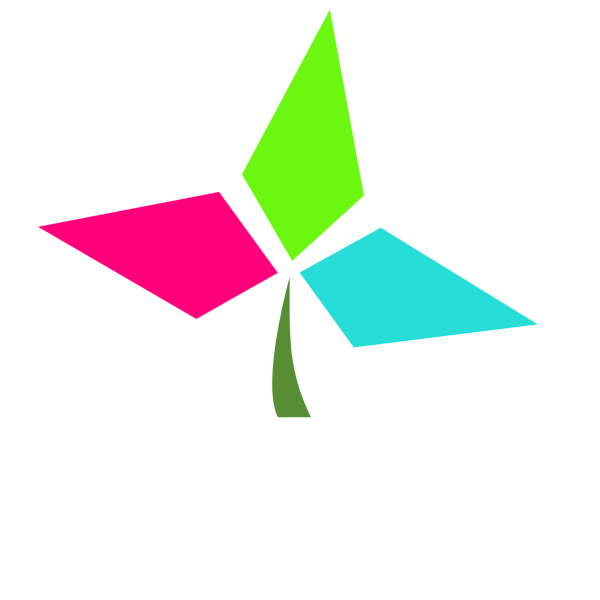
善用空白可以大大增加可讀性
double get_area(double radius) {
const double PI=3.14;
return radius*radius*PI;
}
double get_area(double radius) {
const double PI = 3.14;
return radius * radius * PI;
}
Spacing
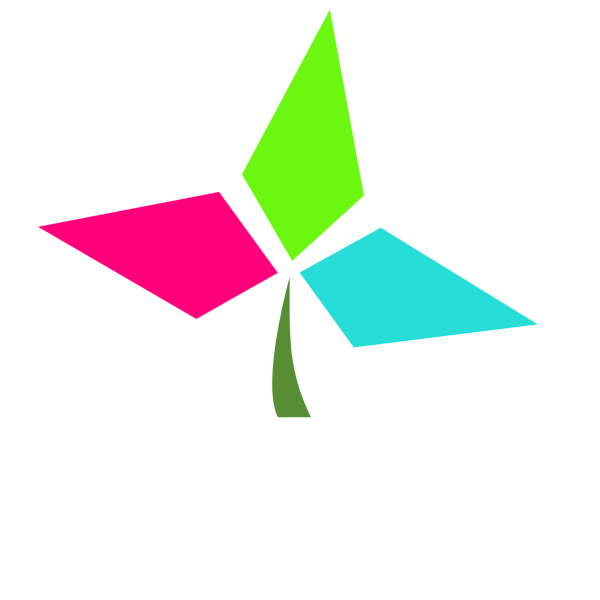
indent
for (int i = 0; i < n; i++)
for (int j = 0; j < m; j++)
if (i > j)
std::cout << "bigger\n";
for (int i = 0; i < n; i++)
for (int j = 0; j < m; j++)
if (i > j)
std::cout << "bigger\n";
Spacing
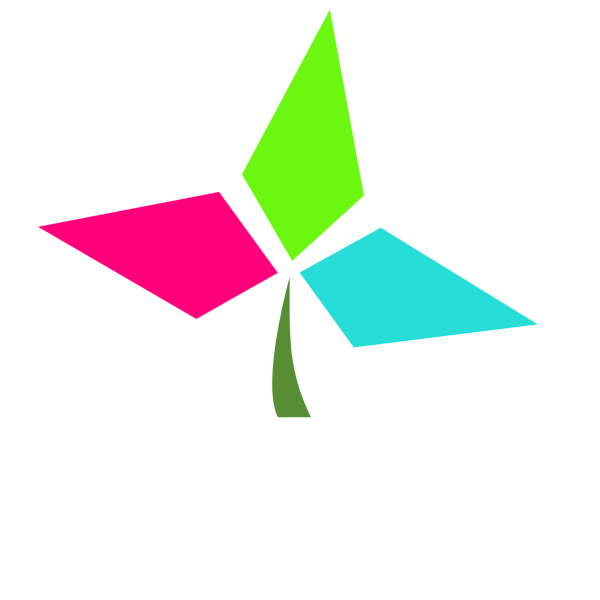
indent
用空白代替tab
通常可以在編輯器設定按tab時代表幾個空白
Google: 使用2個空白
個人偏好: 使用4個空白
Spacing
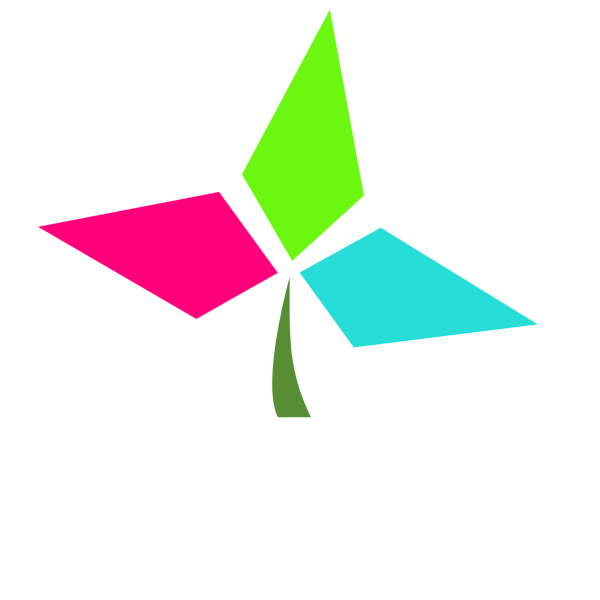
適度放入一些空白的行
double get_circle_area(double radius) {
const double PI = 3.14;
return radius * radius * PI;
}
int get_rectangle_area(int height, int width) {
return height * width;
}
int main() {
double radius = 1.23;
double circle_area = get_circle_area(radius);
int height = 3;
int widht = 4;
int rectangle_area = get_rectangle_area(height, width);
return 0;
}
Spacing
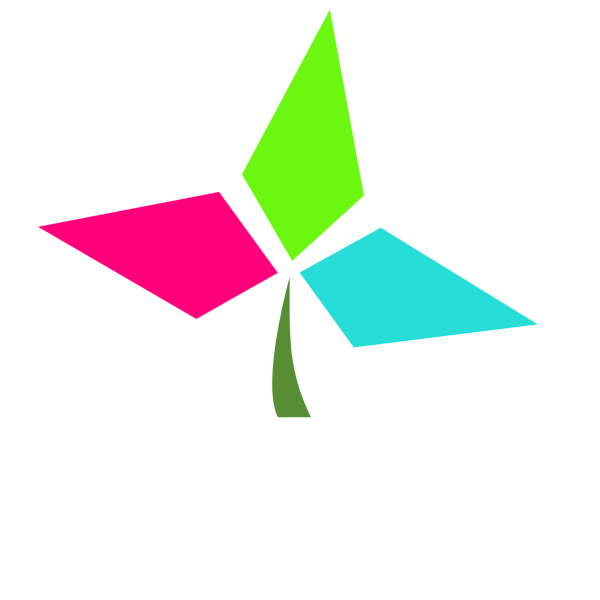
傳教時間
if (a + b > c) {
// good
}
if( a + b > c ){
// not so good
}
if(a+b>c){
// you wanna fight?
}
Curly Braces
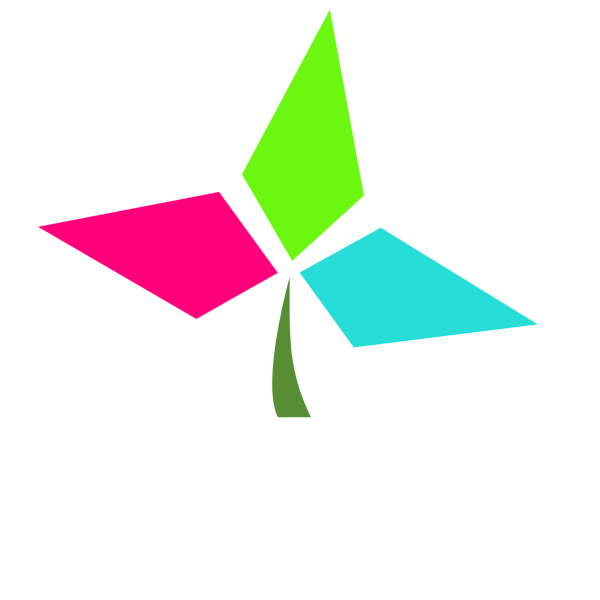
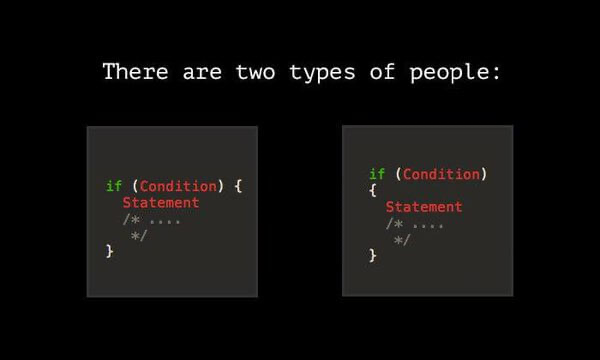
Comments
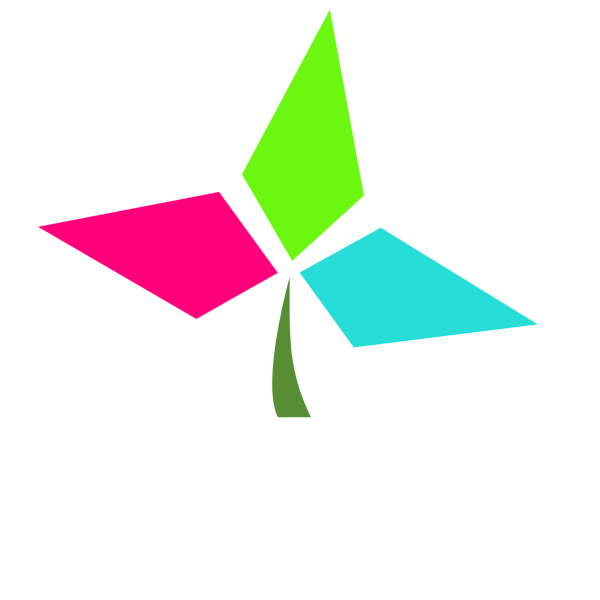
適度加入註解,增加可讀性
char* get_student_class(char* student_name) {
// return one of "C++", "Python", "algo"
// or "none" if student is not in any class
...
}
善用 // 或是 /* */
保持一致性
Misc
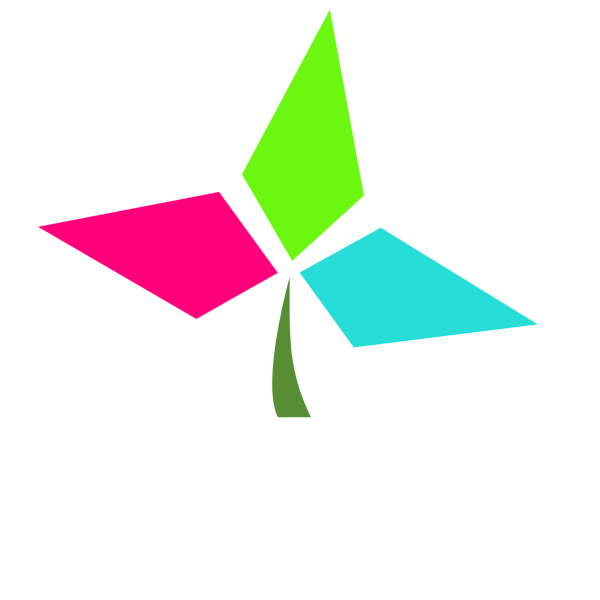
1. 在宣告變數時,宣告在需要這個變數的附近地方
2. 盡量不要使用全域變數
3. 初始化剛宣告的變數
4. 盡量不要 using namespace std;
5. 編輯器使用dark theme (不可靠研究顯示coding速度+37%)
Conclusion
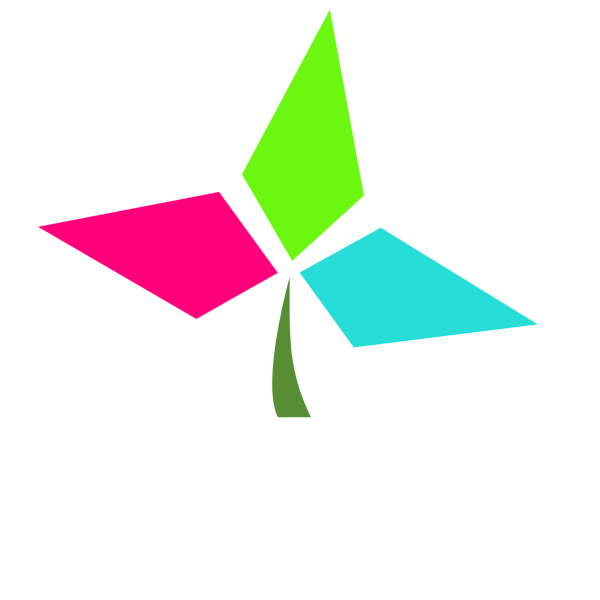
這是一堂最簡單的課
也是一堂最困難的課
沒有新的語法、技術、邏輯、算數....
如何把程式寫的簡單易懂是長期的課題,沒有捷徑
Conclusion
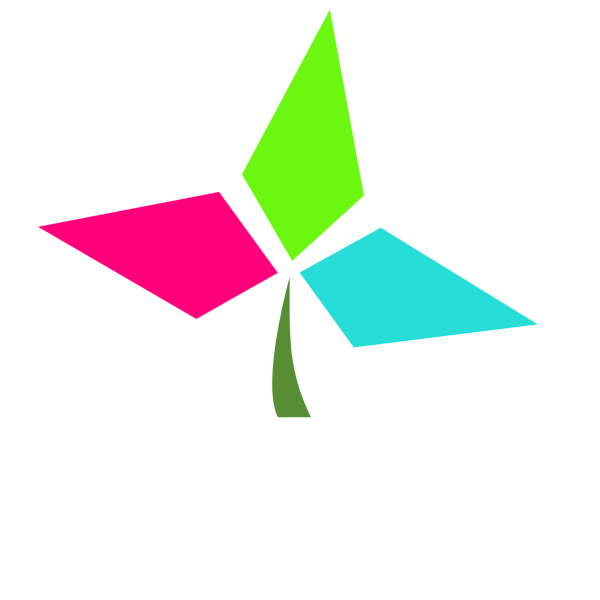
一個會寫程式的人,不只是可以寫出功能,還要寫的漂亮
Sprout 2021 Coding Style
By JT
Sprout 2021 Coding Style
- 1,252