Trying out GSAP


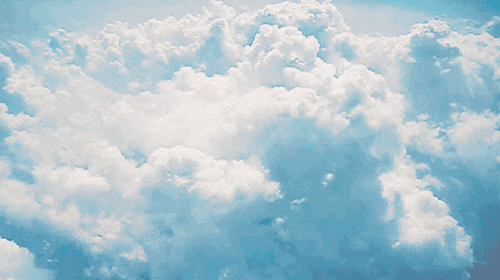
What is GSAP?
GreenSock Animation Platform is a set of small javascript files that helps creating animations between browsers.

Getting started
We need to include GSAP in our project, for this example, we will use the CDN.
This needs to be added in the HTML inside a script tag.
<script src=”https://cdnjs.cloudflare.com/ajax/libs/gsap/3.5.1/gsap.min.js"></script>
Or... using npm
npm install gsap
gsap.to()
GSAP is a properties manipulator. We can animate something from a state/position or take it to another one.
gsap.to(
'.box',
{
duration: 2,
x: 400
}
);
gsap.to()
We can animate something from a state/position or take it to another one.
gsap.from()
GSAP is a properties manipulator. We can animate something from a state/position or take it to another one.
gsap.from(
'.box',
{
x: 400
}
);
gsap.from()
We can animate something from a state/position or take it to another one.
gsap.to()
vs
gsap.from()
The only difference is the order in how the alteration to properties occurs.
Multiple properties
The properties object can alter can take multiple parameters at the same time.
gsap.fromTo()
We can control the initial and final positions of the elements you would like to animate.
gsap.fromTo(
'.box',
{
x: 400,
y: 10,
opacity: 0
},
{
y: 50,
opacity: 1,
duration: 10,
ease: 'elastic'
}
);
gsap.fromTo()
We can control the initial and final positions of the elements you would like to animate.
Easing
Let's take a look into the Ease Visualizer
Timelines
Every animation can be conceived as a node inside a timeline.
const timeline = gsap.timeline();
timeline.add(rotate('.ad'));
timeline.add(fadeIn('.title'));
Timelines
Every animation can be conceived as a node inside a timeline.
Exercise
Let's build a ball bouncing animation
Sources
GSAP
By Juan Sebastián
GSAP
- 137