Bridge
Problem

Solution

Code
function Circle(color) {
this.color = color;
this.toString = function () {
return `${this.color.getColorName()} Circle`;
};
}
function Rectangle(color) {
this.color = color;
this.toString = function () {
return `${this.color.getColorName()} Rectangle`;
};
}
function Triangle(color) {
this.color = color;
this.toString = function () {
return `${this.color.getColorName()} Triangle`;
};
}
function Red() {
this.getColorName = function () {
return 'Red';
}
}
function Blue() {
this.getColorName = function () {
return 'Blue';
}
}
function Green() {
this.getColorName = function () {
return 'Green';
}
}
const redColor = new Red();
const blueColor = new Blue();
const greenColor = new Green();
const redCircle = new Circle(redColor);
const blueCircle = new Circle(blueColor);
const greenRectangle = new Rectangle(greenColor);
var RemoteControl = function(tv) {
this.tv = tv;
this.on = function() {
this.tv.on();
};
this.off = function() {
this.tv.off();
};
this.setChannel = function(ch) {
this.tv.tuneChannel(ch);
};
};
/* Newer, Better Remote Control */
var PowerRemote = function(tv) {
this.tv = tv;
this.currChannel = 0;
this.setChannel = function(ch) {
this.currChannel = ch;
this.tv.tuneChannel(ch);
};
this.nextChannel = function() {
this.setChannel(this.currChannel + 1);
};
this.prevChannel = function() {
this.setChannel(this.currChannel - 1);
};
};
PowerRemote.prototype = new RemoteControl();
/** TV Interface
Since there are no Interfaces in JavaScript I am just
going to use comments to define what the implementors
should implement
function on
function off
function tuneChannel(channel)
*/
/* Sony TV */
var SonyTV = function() {
this.on = function() {
console.log('Sony TV is on');
};
this.off = function() {
console.log('Sony TV is off');
};
this.tuneChannel = function(ch) {
console.log('Sony TV tuned to channel ' + ch);
};
}
/* Toshiba TV */
var ToshibaTV = function() {
this.on = function() {
console.log('Welcome to Toshiba entertainment');
};
this.off = function() {
console.log('Goodbye Toshiba user');
};
this.tuneChannel = function(ch) {
console.log('Channel ' + ch + ' is set on your Toshiba television');
};
}
/* Let's see it in action */
var sony = new SonyTV(),
toshiba = new ToshibaTV(),
std_remote = new RemoteControl(sony),
pwr_remote = new PowerRemote(toshiba);
THX
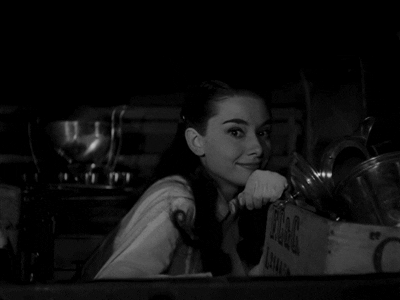
deck
By just Just
deck
- 23