Facade
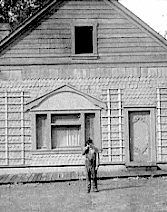
What? How? Why?
The facade is a class that provides a simple interface to a complex subsystem containing dozens of classes. The facade may have limited functionality in comparison to working with subsystem directly. However, it includes only those features that clients really care about.
Code

var Mortgage = function(name) {
this.name = name;
}
Mortgage.prototype = {
applyFor: function(amount) {
// access multiple subsystems...
var result = "approved";
if (!new Bank().verify(this.name, amount)) {
result = "denied";
} else if (!new Credit().get(this.name)) {
result = "denied";
} else if (!new Background().check(this.name)) {
result = "denied";
}
return this.name + " has been " + result +
" for a " + amount + " mortgage";
}
}
var Bank = function() {
this.verify = function(name, amount) {
// complex logic ...
return true;
}
}
var Credit = function() {
this.get = function(name) {
// complex logic ...
return true;
}
}
var Background = function() {
this.check = function(name) {
// complex logic ...
return true;
}
}
var mortgage = new Mortgage("Joan Templeton");
var result = mortgage.applyFor("$100,000");
console.log(result);
class CPU {
freeze() { /* code here */ }
jump(position) { /* code here */ }
execute() { /* code here */ }
}
class Memory {
load(position, data) { /* code here */ }
}
class HardDrive {
read(lba, size) { /* code here */ }
}
/* Facade */
class ComputerFacade {
constructor() {
this.processor = new CPU();
this.ram = new Memory();
this.hd = new HardDrive();
}
start() {
this.processor.freeze();
this.ram.load(this.BOOT_ADDRESS, this.hd.read(this.BOOT_SECTOR, this.SECTOR_SIZE));
this.processor.jump(this.BOOT_ADDRESS);
this.processor.execute();
}
}
/* Client */
let computer = new ComputerFacade();
computer.start();
THX
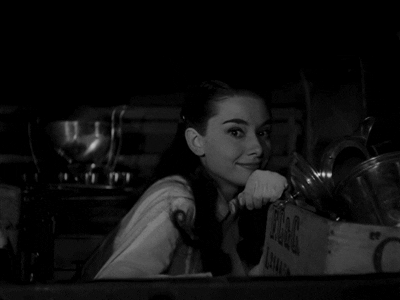
Facade
By just Just
Facade
- 24