All Aboard the Type Train
Kadi Kraman
@kadikraman
π

Hi, my name is
Kadi
I work at
ormidable
... and I write a lot of JavaScript
@kadikraman

@kadikraman
@kadikraman
π
π
π
π



Flow
TypeScript
Since PEP 484
PHP 7.4
@kadikraman
But why?
Frontend
Backend
@kadikraman
"The Stack"
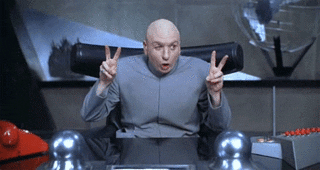

Frontend
Backend

π







@kadikraman
"The Stack"
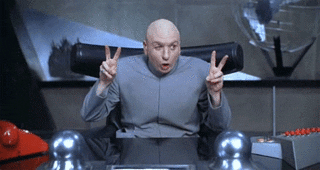

Frontend
Backend
π
@kadikraman
@kadikraman

Let's talk about JavaScript
@kadikraman
function addOne(array) {
return array.map(val => val + 1);
}
addOne([1, 2, 3])
// [2, 3, 4]
addOne({})

@kadikraman
function addOne(array) {
return array.map(val => val + 1);
}
/**
* Adds 1 to each element in the array
* @param array (array of numbers): array to increment
*/
function addOne(array) {
return array.map(val => val + 1);
}
@kadikraman
I could add comments?
Just kidding - no one reads comments
function addOne(array) {
if (!Array.isArray(array) || !array.every(val => typeof val === "number")) {
return [];
}
return array.map(val => val + 1);
}
@kadikraman
I could add a fallback?
But this function is only meant to be used on a number array
function addOne(array: number[]): number[] {
return array.map(val => val + 1);
}
@kadikraman
... or I could add explicit type information?
* not valid in plain JavaScript
@kadikraman
β‘οΈNews Flash!β‘οΈ
@kadikraman
All programming languages have a type system
The difference is when the type checking is done!
@kadikraman
Compile time
Run time
@kadikraman
Static vs Dynamic
Compile time vs run time type checking
Strong vs Weak
Typed vs Untyped
@kadikraman
Run time

π€·ββοΈ Β π€·ββοΈ Β π€·ββοΈ

Compile time
@kadikraman
Run time
Compile time




Type errors?Β
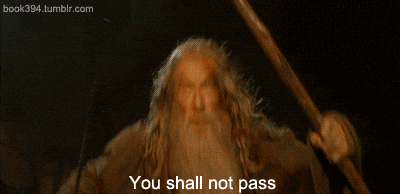
Errors can still occur, but not type errors
@kadikraman
Static Typing - the downsides
@kadikraman

( source: https://xkcd.com/303/Β )
It takes time
@kadikraman
It adds verbosity
vs
function addOne(array: number[]): number[] {
return array.map(val => val + 1);
}
function addOne(array) {
return array.map(val => val + 1);
}
@kadikraman
Static Typing - the upsides
@kadikraman
Fewer runtime exceptions
(no type errors)
@kadikraman
Fewer tests
(you don't need to test for type correctness)
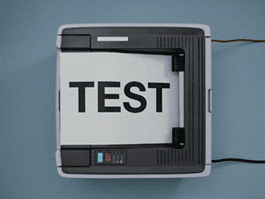
@kadikraman
Dynamic Typing - the upsides
@kadikraman
Faster
(no compile step)
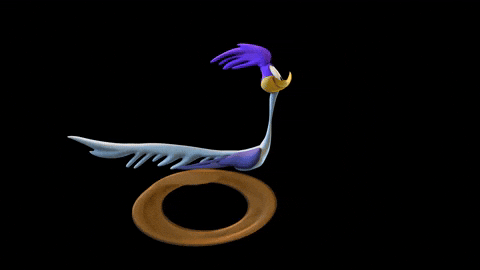
@kadikraman
More Flexible
var myValue = 1;
myValue = "potato";
@kadikraman
Dynamic Typing - the downsides
@kadikraman
Runtime Type Errors

@kadikraman
Harder to Refactor with ConfidenceΒ
π€
@kadikraman
Dynamic
Static
β Faster
β More flexible
Β
β Runtime type errors
β Harder to refactor
β Fewer runtime exceptions
β Fewer tests
Β
β Takes longer
β Adds verbosity
Could we borrow concepts from statically typed languages?
@kadikraman
How to reduce Type Errors
in a Dynamically Typed language?
@kadikraman

ESLint for type checking in JavaScript

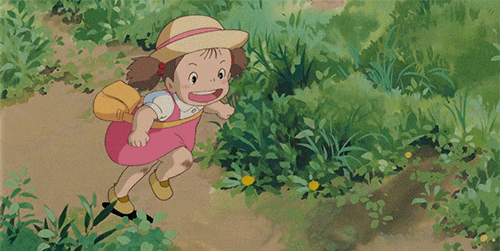
@kadikraman
React Community
2 years ago

Infers type information from existing code
@kadikraman
You can enforce types

@kadikraman
There's a community for typing node_modules
@kadikraman

@kadikraman
Downsides?

Configuration π±

Memory π
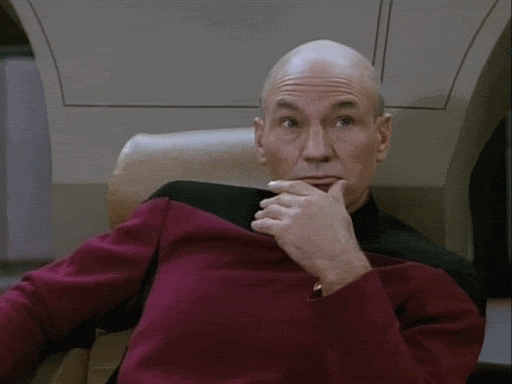
You can easily ignore it π³

@kadikraman


React Community
now
@kadikraman
Static type checker
Language


@kadikraman


@kadikraman

You can still...
Infer type information from existing code
@kadikraman
You can still...
Enforce types

@kadikraman
The node_modules community is bigger
Flow
TypeScript


@kadikraman

Configuration

Flow π±Β
TypeScript β
@kadikraman
Memory

Flow π±Β
TypeScript β
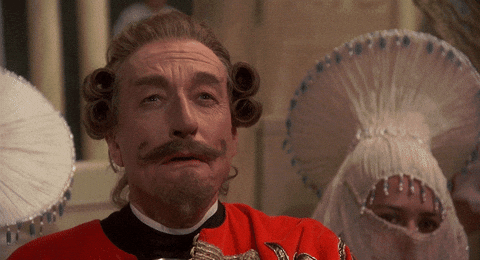
@kadikraman
In summary...
β has a larger community
β has a faster release cycle
β is more reliable
β is easier to set up
β has an ability to enforce type-checking
Β
β although you can still ignore type checking
TypeScript...
But I don't want to use TypeScript!
@kadikraman
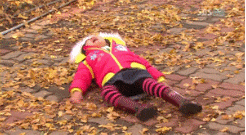
Do you even care about static type checking?
NO
YES

Use plain JavaScript!
@kadikraman
Why can't we just use a "proper" typed language?
@kadikraman
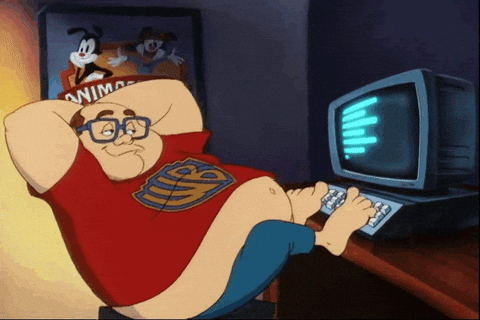
@kadikraman

Frontend
Backend

π







@kadikraman

Frontend
Backend














ReasonML (2016 by Jordan Walke)
(Transpiles to OCaml which compiles to JS)
let add = (x: int, y: int) : int => x + y;
@kadikraman
@kadikraman

type user = { firstName: string, lastName: string };
let user: user = {
firstName: "Harper",
lastName: "Perez"
};
let formatName user =>
user.firstName ^ " " ^ user.lastName;
let hello =
(ReactRe.stringToElement ("Hello, " ^ formatName user));
let header =
<h1 title=(formatName user)>
hello
</h1>;
ReactDOMRe.renderToElementWithId header "root";

Elm (2012 by Evan Czaplicki)
add : Number -> Number -> Number
add a b =
a + b
"no runtime exceptions"
(compiles to JS)
@kadikraman
import Browser
import Html exposing (Html, button, div, text)
import Html.Events exposing (onClick)
main =
Browser.sandbox { init = 0, update = update, view = view }
type Msg = Increment | Decrement
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (String.fromInt model) ]
, button [ onClick Increment ] [ text "+" ]
]

@kadikraman
@kadikraman
1996
2014
2016
2012

JavaScript

Elm

TypeScript

Flow

Reason
(3 years)
(5 years)
(7 years)
(23 years)
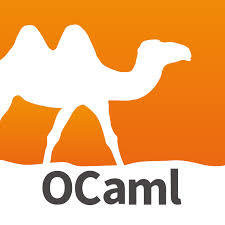
OCaml
2017
(4 years)

Web Assembly
New project, who dis?
Reliability in prod
Effort to learn




JavaScript
Flow
Reason
Elm

TypeScript
@kadikraman
New project, who dis?
Reliability in prod
Effort to learn




JavaScript
Flow
Reason
Elm

TypeScript
@kadikraman
Server Fetched Partials?
π»
Add types to your code
Stay Safe
@kadikraman
For riding the Type Train π
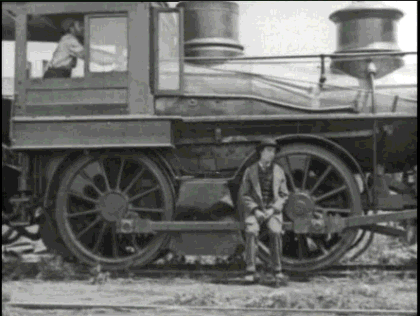
Thank you!
@kadikraman
All Aboard the Type Train (FSEU2019)
By Kadi Kraman
All Aboard the Type Train (FSEU2019)
JavaScript is a language of add-ons, and one of its more recent trends is typing. There are obvious benefits to typing, but what are the drawbacks? Should you choose Flow or TypeScript? Why use types at all? And why not just go straight to Reason?
- 1,268