
Introduction to
@kajetansw
kajetan.dev
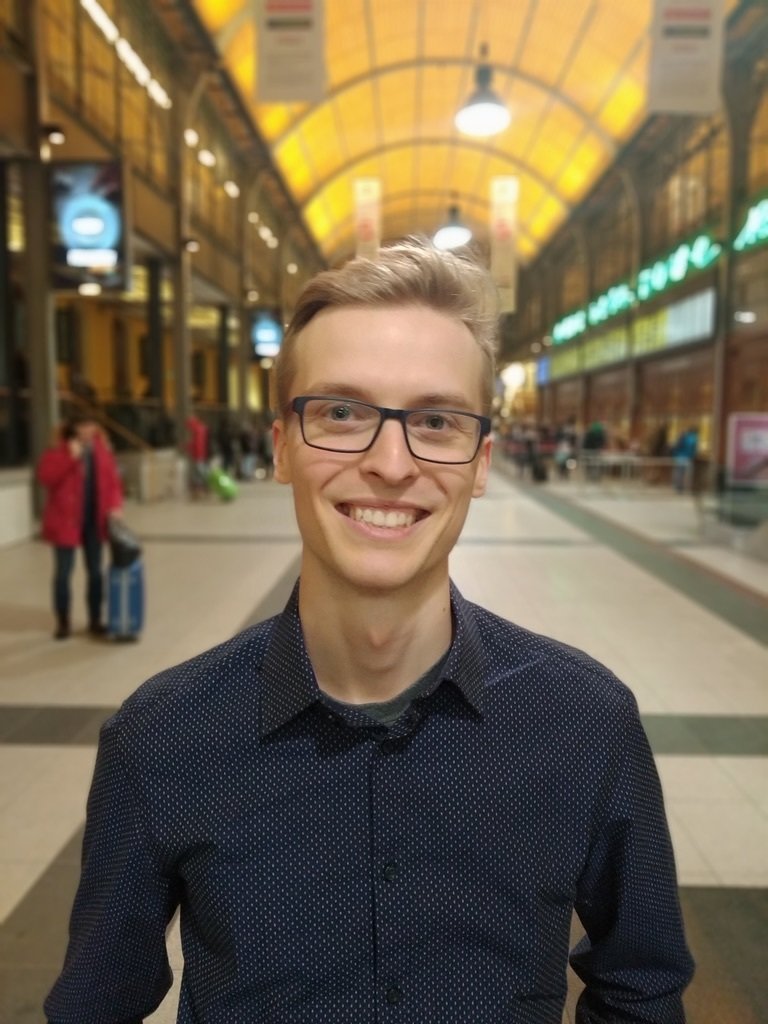
Kajetan Świątek
👨🎨 Front-end developer
🇵🇱 Wrocław
Interesting facts
It had 90% satisfaction score
in the State of JS 2021 survey.
Front-end library made
by Ryan Carniato
First public version in 2018,
v1.0 in 2021
Selling points
🔁 Reactivity 🔁
⚡️ Performance ⚡️
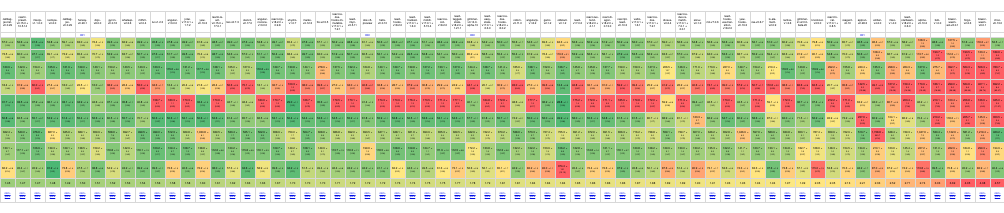

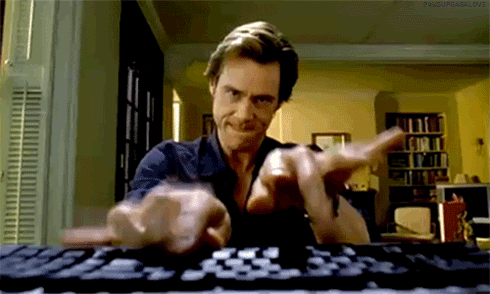
Let's jump to the code!
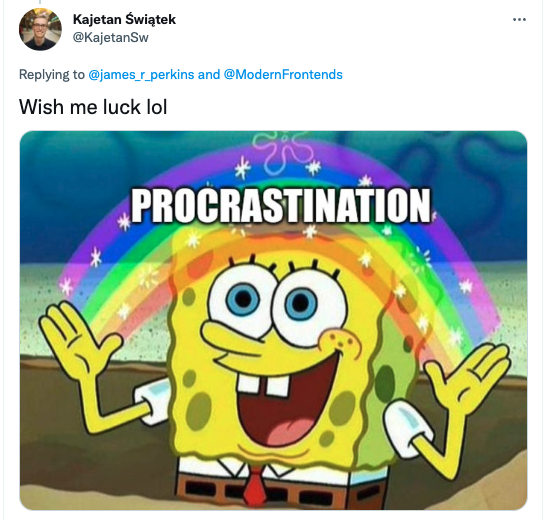
Components and JSX
import { Component } from 'solid-js';
const App: Component = () => {
return <p>Hello world!</p>;
};
Reactive primitives
import { Component, createSignal, createEffect } from 'solid-js';
const App: Component = () => {
const [password, setPassword] = createSignal('');
createEffect(() => {
console.log(password());
});
return (
<>
Password: {password()}
<input
type="text"
onInput={(e) => setPassword(e.currentTarget.value)}
/>
</>
);
};
Derived signals
const [count, setCount] = createSignal(0);
const doubleCount = () => count() * 2;
Async resources
const fetchUser = async (id) =>
(await fetch(`https://swapi.dev/api/people/${id}/`)).json();
const App = () => {
const [userId, setUserId] = createSignal();
const [user] = createResource(userId, fetchUser);
return (
<>
<input
type="number"
onInput={(e) => setUserId(e.currentTarget.value)}
/>
<span>{user.loading && "Loading..."}</span>
<span>Username: {user()?.name}</span>
</>
);
};
Control flow
const App = () => {
const [loggedIn, setLoggedIn] = createSignal(false);
const toggle = () => setLoggedIn(!loggedIn())
return (
<Show
when={loggedIn()}
fallback={
<button onClick={toggle}>Log in</button>
}
>
<button onClick={toggle}>Log out</button>
</Show>
);
}
-
Show
-
For
-
Switch
Further reading
📚
Thank you!
✋😎
@kajetansw
kajetan.dev
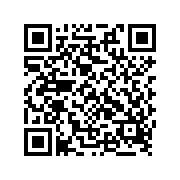
Intro to SolidJS
By Kajetan Świątek
Intro to SolidJS
- 504