Making Websites
with JavaScript
Katja Durrani
@kdurrani
mail@kdurrani.co.uk
Women Who Code
Bristol
27 Jan 2015
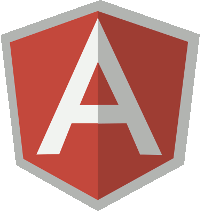
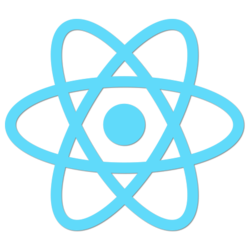
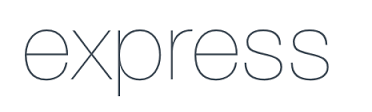
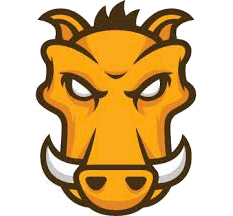

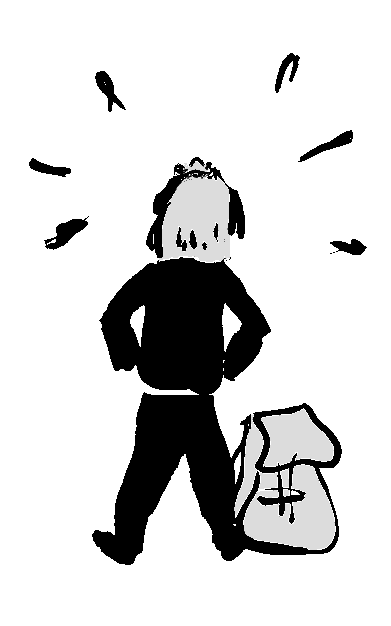
Key Features
- JavaScript outside the browser
- Contains a library for creating a web server
- 'Single-threaded, event-based, non-blocking I/O'
- Suited for real-time applications
- Modular (CommonJS style)
- Can be extended via npm package manager
"A development platform built on top of Google's V8 JavaScript virtual machine"
What is NodeJS?
What Node is used for
- Building APIs
- Real-time web applications, streaming
- Building command-line tools
- Hard-ware hacking
Node is popular
About 90 NodeSchools world-wide!
- Setting up a server
- Routing
- Providing APIs
Main uses for Express
There are some alternative frameworks (Hapi, Koa), but so far Express is the most widely used
The "Hello World" of Node and Express:
Writing a server
var express = require('express')
var app = express()
app.get('/', function (req, res) {
res.send('Hello World!')
})
var server = app.listen(3000, function () {
var host = server.address().address
var port = server.address().port
console.log('Example app listening at http://%s:%s', host, port)
})
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
}).listen(8124, "127.0.0.1");
console.log('Server running at http://127.0.0.1:8124/');
Node
Express
JS App Workflow Considerations
Structure
Sub-directories for static files, views, routes, models etc.
Dependency Management
Usually via package manager (e.g. npm, bower, composer)
For npm: Dependencies listed in package.json file
npm install // installs dependencies listed in package.json
npm install <module> --save // installs new package, saves reference in package.json
JS App Workflow ctd.
Task Automation
Grunt, Gulp
Modularisation
Node uses CommonJS syntax
var React = require('react');
var App = {
[...]
}
module.exports = App
You can write node-style modules for the browser, using tools like browserify which compile them for that use
Hosting
You might have to add code to your app for it to run on specific platforms (e.g. OpenShift, AWS, Heroku)
Looking at an example
Real-time Polls App built on MEAN stack
Key Features
- Markup and display logic are combined inside nestable components
- 'Virtual DOM and synthetic events'
- High performance
- One-way reactive data flow
- Re-renders whole component on every change
- JSX preprocessor lets you use HTML-like syntax inside JS
"A JavaScript library for building user interfaces -
the V in MVC"
What is ReactJS?
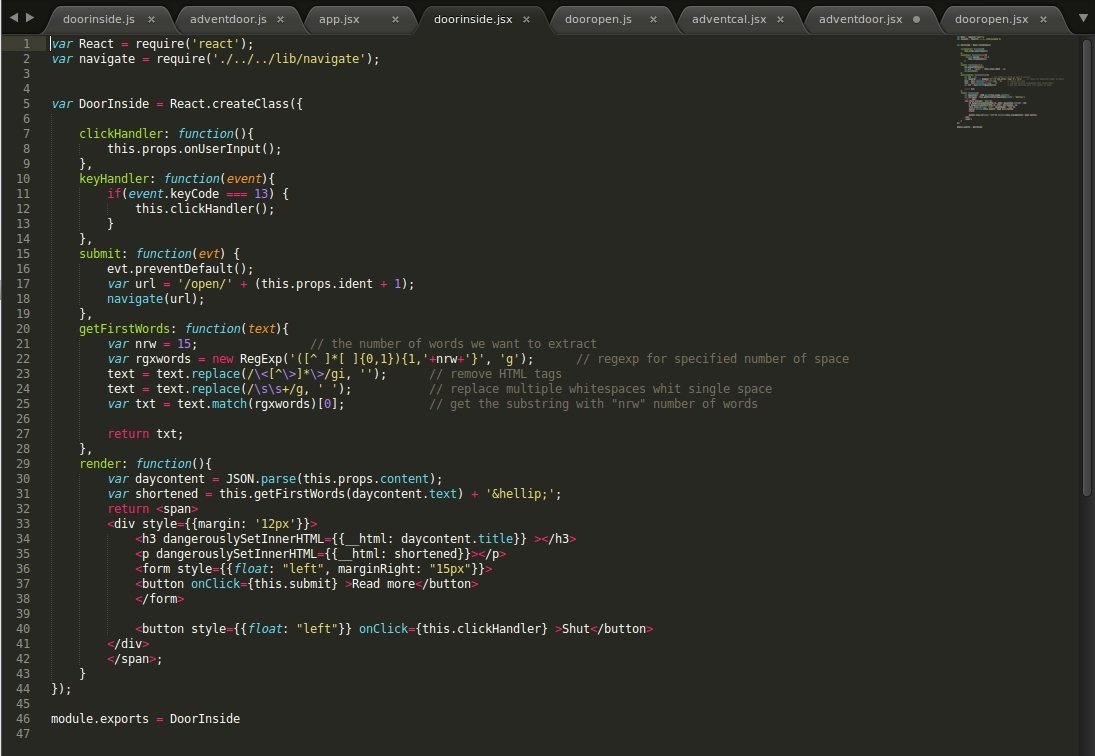
Example of React Component (before and after compilation to JS)
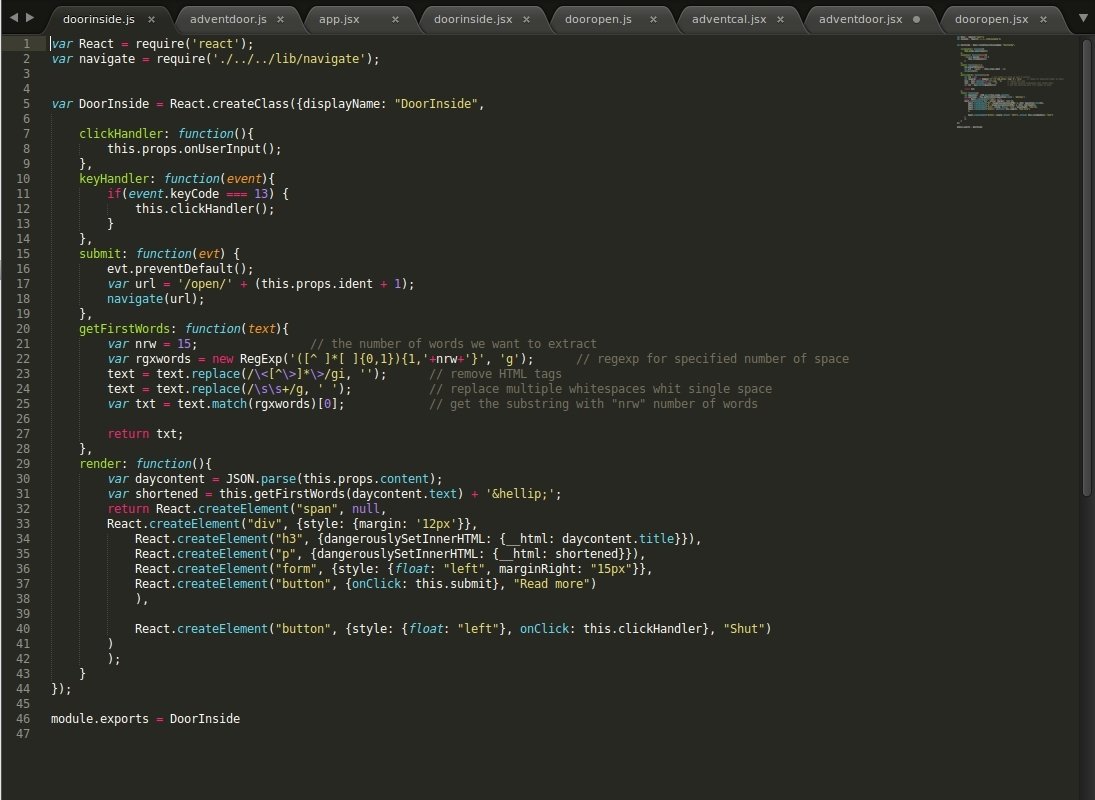
Playing with ReactJS
Click to get through to the sites
Thank you!
Making websites with JavaScript
By Katja Durrani
Making websites with JavaScript
- 1,258