Frontend with Vue.js
Kongkow IT Meetup #2
Introduction
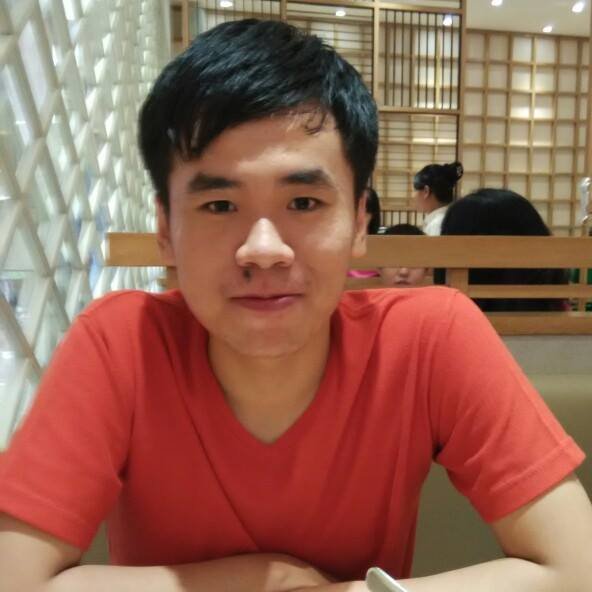
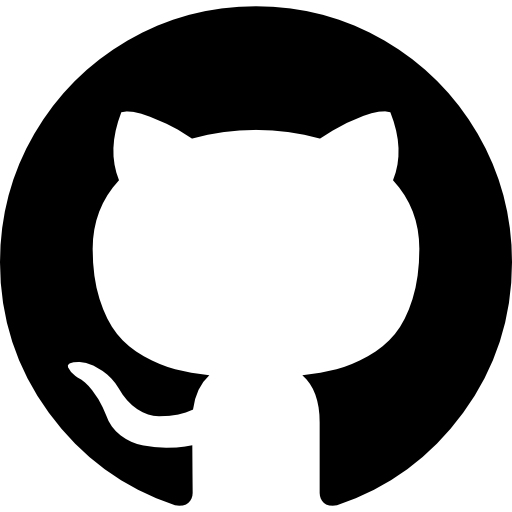
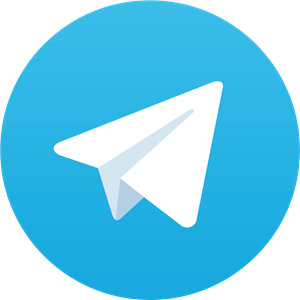
@kevinongko
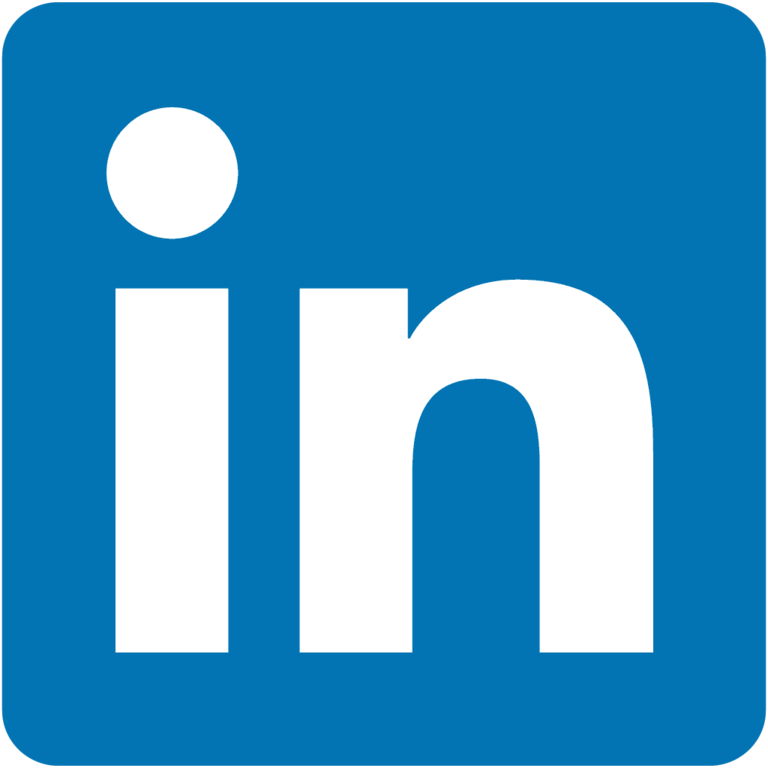
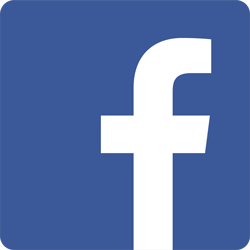
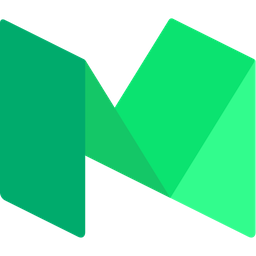
What is Vue.js ?
- It's a Javascript framework.
- Progressive, small core with official library for routing & global state.
- Virtual DOM and reactive components.
- Performant only 20 kb min+gzip.
- Easy to learn.
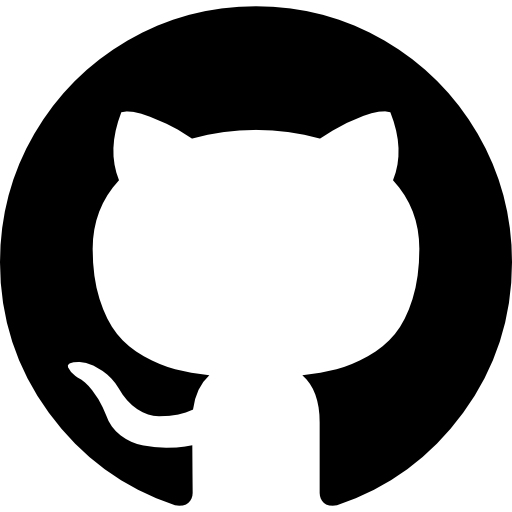
64,500+ stars
top 10 all time
2nd most-starred Javascript framework
672k+ monthly NPM downloads
excluding mirrors from china
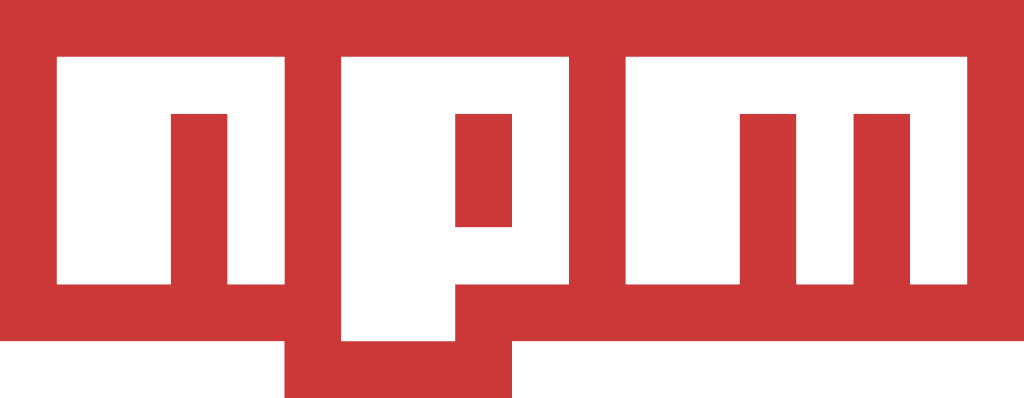
is Using Vue.js
- Otten Coffee
- Waruna group
- Jakmall
- Blibli
- Bukalapak
- Alibaba
- Tencent
- Baidu
- Adobe
- Gitlab
- Codeship
Comparing Vue with other JS frameworks
vuejs.org/v2/guide/comparison.html
Installation
- Include <script> tag in .html file
- Install via NPM (Node Package Manager)
- Automatic setup via vue-cli
Hello world
<div id="app">
{{ message }}
</div>
<script src="https://unpkg.com/vue"></script>
<script>
new Vue({
el: '#app',
data: {
message: 'Hello World!'
}
})
</script>
index.html
Template Syntax
Interpolation
<p> {{ message }} </p>
Basic form of data binding is text interpolation using "mustache" syntax
data: {
message: 'Hello world'
}
Directive
Special attribute with v- prefix
<!-- v-model directive -->
<input type="text" v-model="username">
<!-- v-if directive -->
<p v-if="visible">I'm visible</p>
<!-- v-for directive -->
<li v-for="dog in dogs">{{ dog.name }}</li>
Input binding with v-model
<div id="app">
<input type="text" v-model="message">
<p> My message is {{ message }} </p>
</div>
<script>
new Vue({
el: '#app',
data: {
message: ''
}
})
</script>
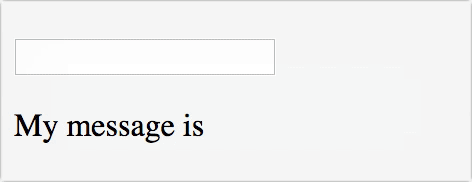
Conditional Rendering
<div id="app">
<p v-if="sent"> Message sent </p>
<p v-else> Message pending </p>
</div>
<script>
new Vue({
el: '#app',
data: {
sent: false
}
})
</script>
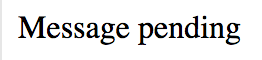
List Rendering
<div id="app">
<ul>
<li v-for="student in students">
{{ student.name }} - {{ student.age }}
</li>
</ul>
</div>
<script>
new Vue({
el: '#app',
data: {
students: [
{ name: 'John', age: 18 },
{ name: 'Steve', age: 17 },
{ name: 'Sarah', age: 19 }
]
}
})
</script>
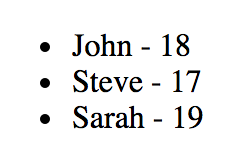
Event Handling
<div id="app">
<button v-on:click="counter++">Click me</button>
<p>Button clicked {{ counter }} times.</p>
</div>
<script>
new Vue({
el: '#app',
data: {
counter: 0
}
})
</script>
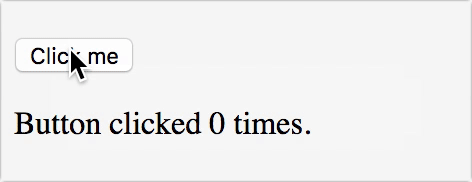
Method Event Handler
<div id="app">
<button v-on:click="say('hi')">Say hi</button>
<button v-on:click="say('what')">Say what</button>
</div>
<script>
new Vue({
el: '#app',
methods: {
say: function (message) {
alert(message)
}
}
})
</script>
- Re-useable
- Multiple action
Computed Properties
<div id="app">
<input type="text" v-model="firstName">
<input type="text" v-model="lastName">
<p>My name is {{ fullName }}</p>
</div>
<script>
new Vue({
el: '#app',
data: {
firstName: '',
lastName: ''
},
computed: {
fullName: function () {
return this.firstName + ' ' + this.lastName
}
}
})
</script>
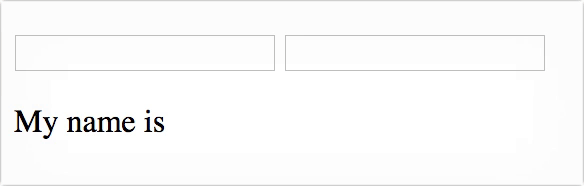
Component
What is component?
<house>
<room>
<clock timezone="UTC"></clock>
<desk></desk>
</room>
<garage>
<car color="red"></car>
</garage>
</house>
- Extend basic HTML elements
- Reusable code
- Can be separated in .vue files
Register component
<div id="app">
<hello-component></hello-component>
<hello-component></hello-component>
<hello-component></hello-component>
</div>
<script>
Vue.component('hello-component', {
template: '<p> {{ message }} </p>',
data: function () {
return {
message: 'hello from component'
}
}
})
new Vue({
el: '#app'
})
</script>
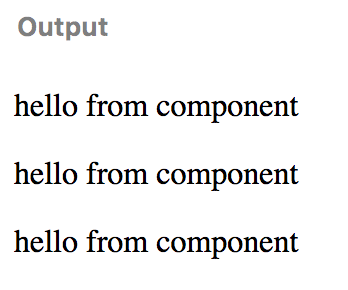
Register component (2)
var helloComponent = {
template: '<p> {{ message }} </p>',
data: function () {
return {
message: 'hello from component'
}
}
}
new Vue({
el: '#app',
components: {
'hello-component': helloComponent
}
})
Component relationship
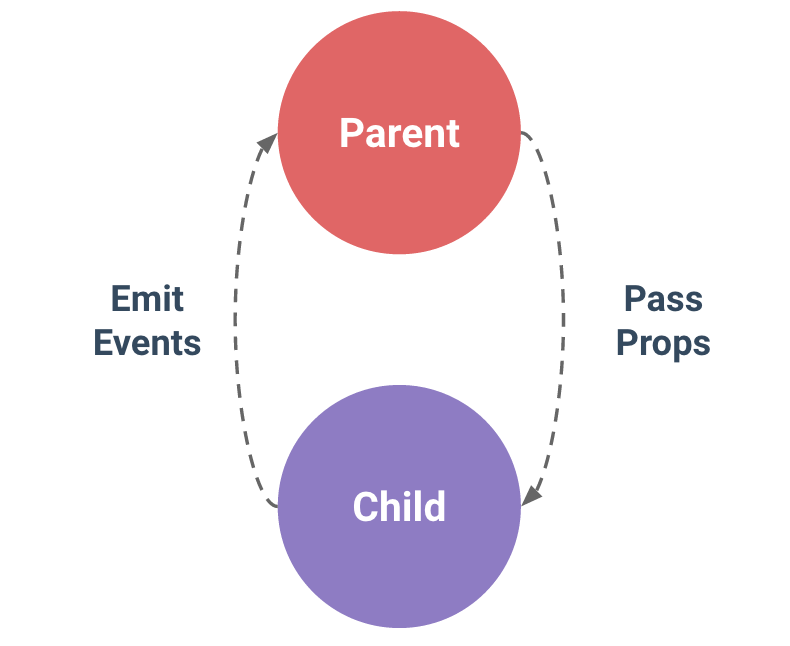
Parent to Child: Props
<div id="app">
<child message="hello from parent"></child>
</div>
<script>
Vue.component('child', {
props: ['message'],
template: '<p>{{ message }}</p>'
})
new Vue({
el: '#app'
})
</script>
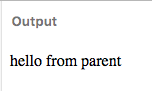
Child to Parent: Custom event
<div id="app">
<p>{{ total }}</p>
<child v-on:child-clicked="incrementTotal"></child>
</div>
new Vue({
el: '#app',
data: {
total: 0
},
methods: {
incrementTotal: function () {
this.total += 1
}
}
})
Vue.component('child', {
template: '<button v-on:click="handleClick">click</button>',
methods: {
handleClick: function () {
this.$emit('child-clicked')
}
},
})
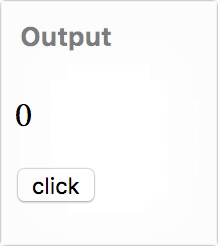
Single File Component
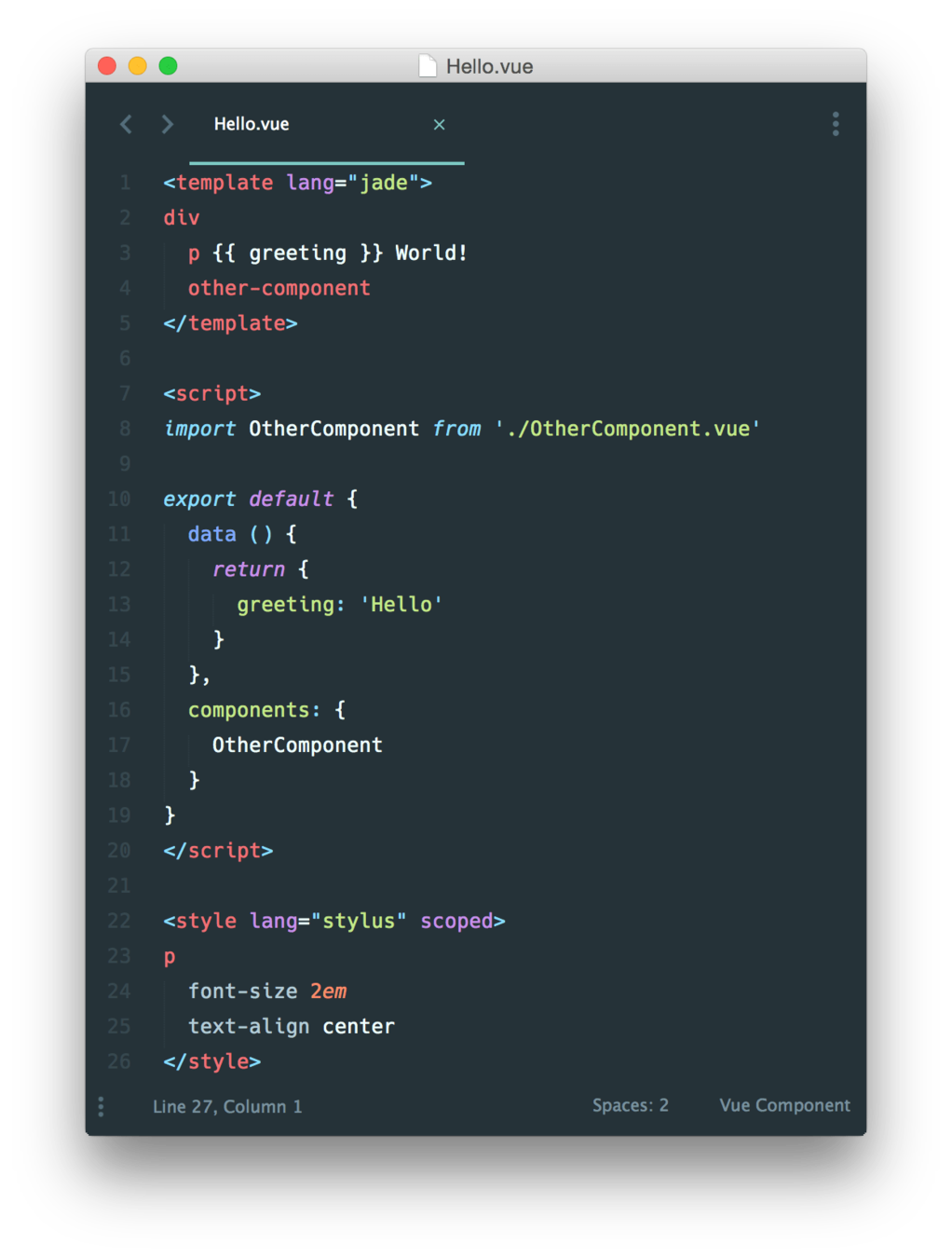
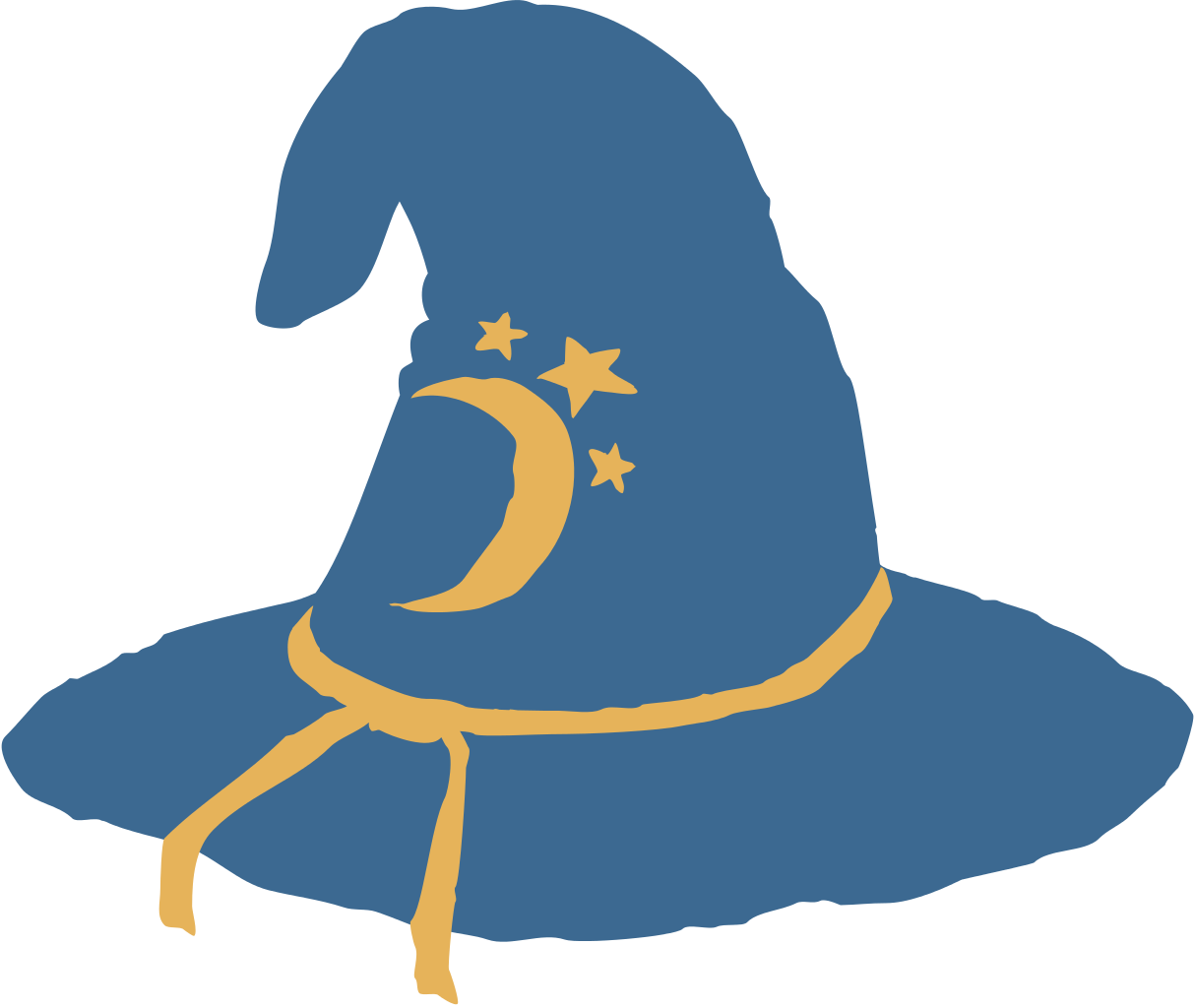
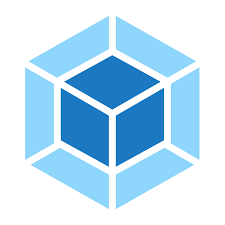
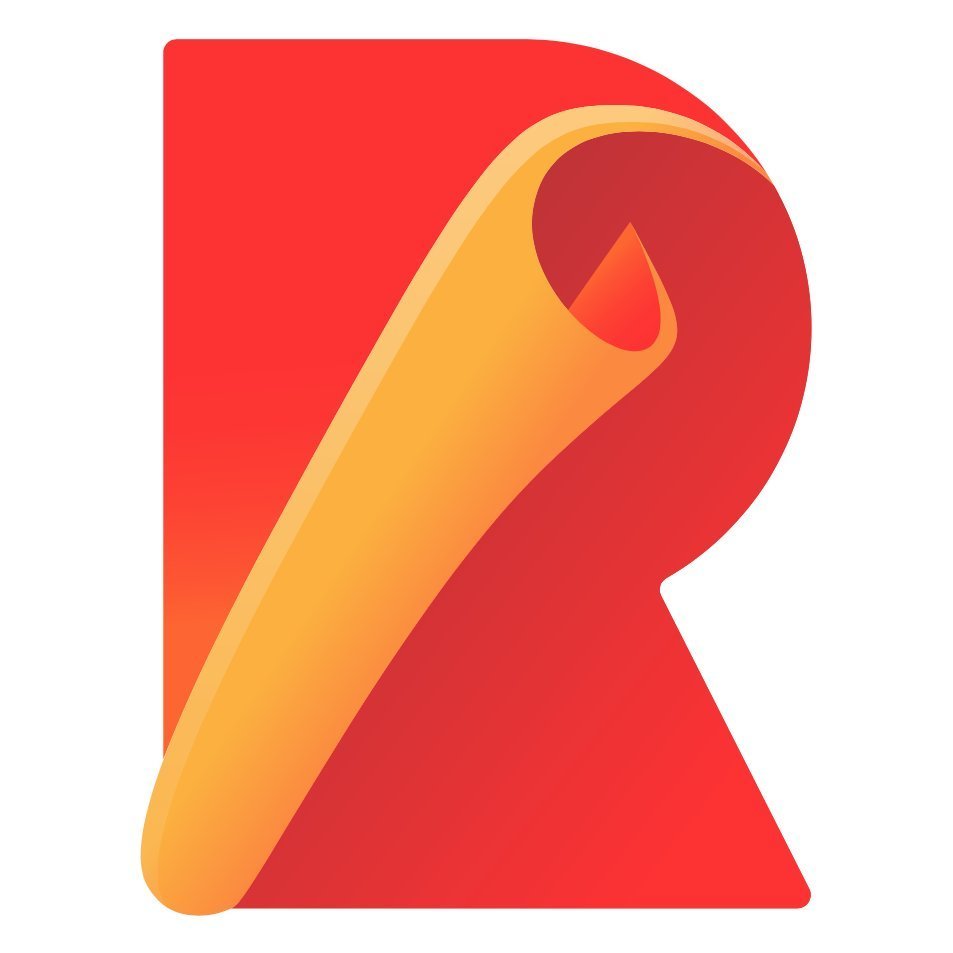
Vue CLI
A simple CLI for scaffolding Vue.js projects.
Template:
- Webpack
- Browserify
- Simple
- PWA
-
npm install --global vue-cli
-
vue init <template> <project-name>
Routing
router.vuejs.org
State management
vuex.vuejs.org
Core plugins
Development
Linter
github.com/vuejs/eslint-plugin-vue
Test
github.com/vuejs/vue-test-utils
Browser Devtool (Chrome/Firefox/Safari)
github.com/vuejs/vue-devtools
Mobile application
Hybrid
Native
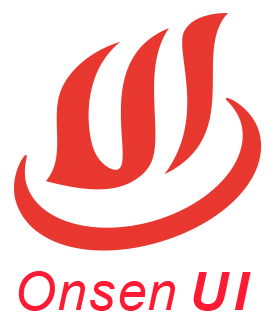
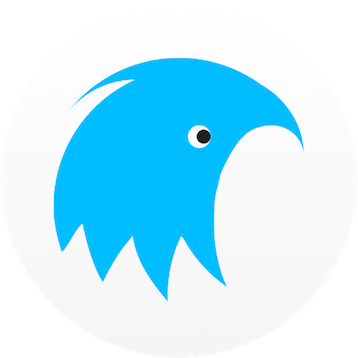
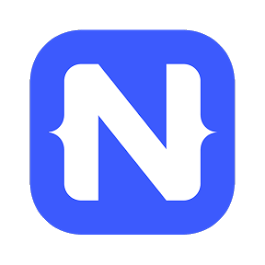
weex
nativescript
Not covered yet...
- Class & style binding
- Custom directive
- Filter
- Mixin
- Plugin
- Transition
- Server side rendering
- Typescript support
- Unit testing
- Too much to list here :|
Check out my repo
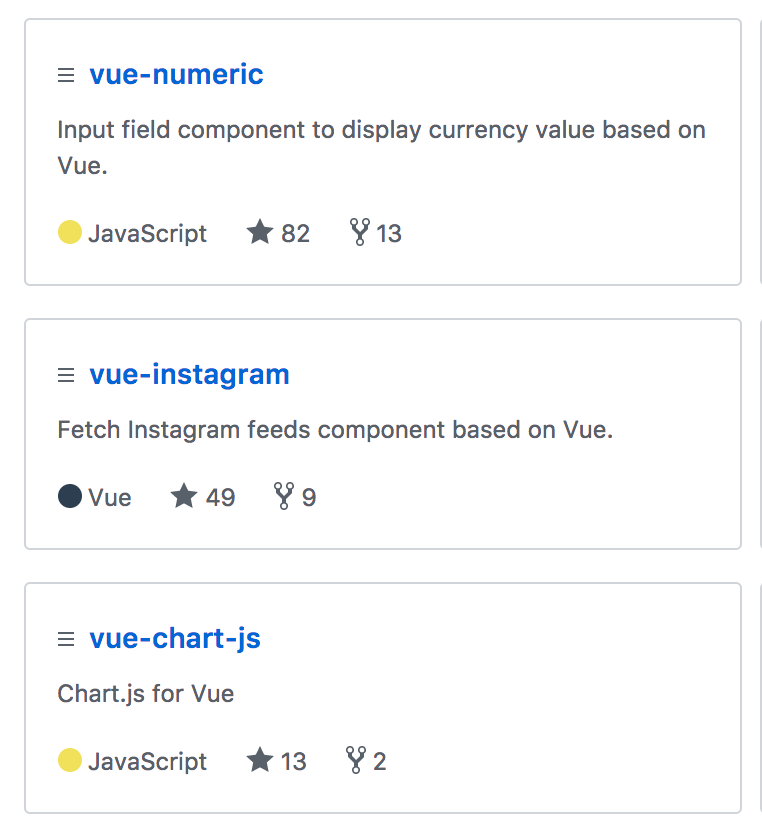
Thank you =)
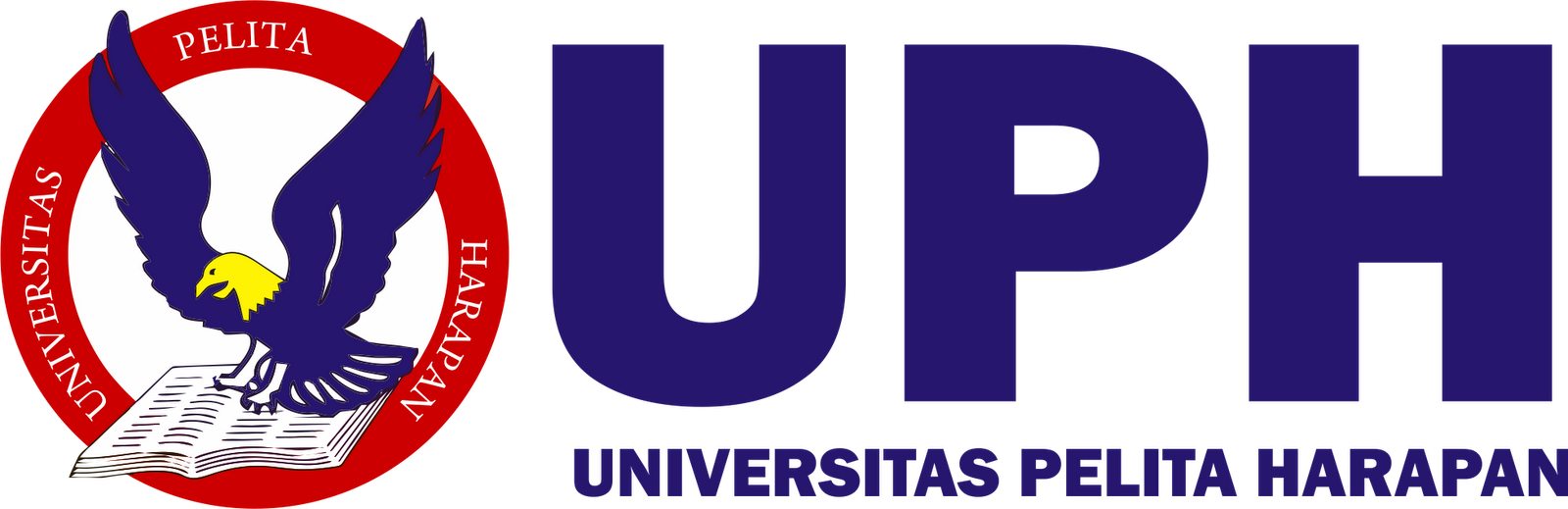
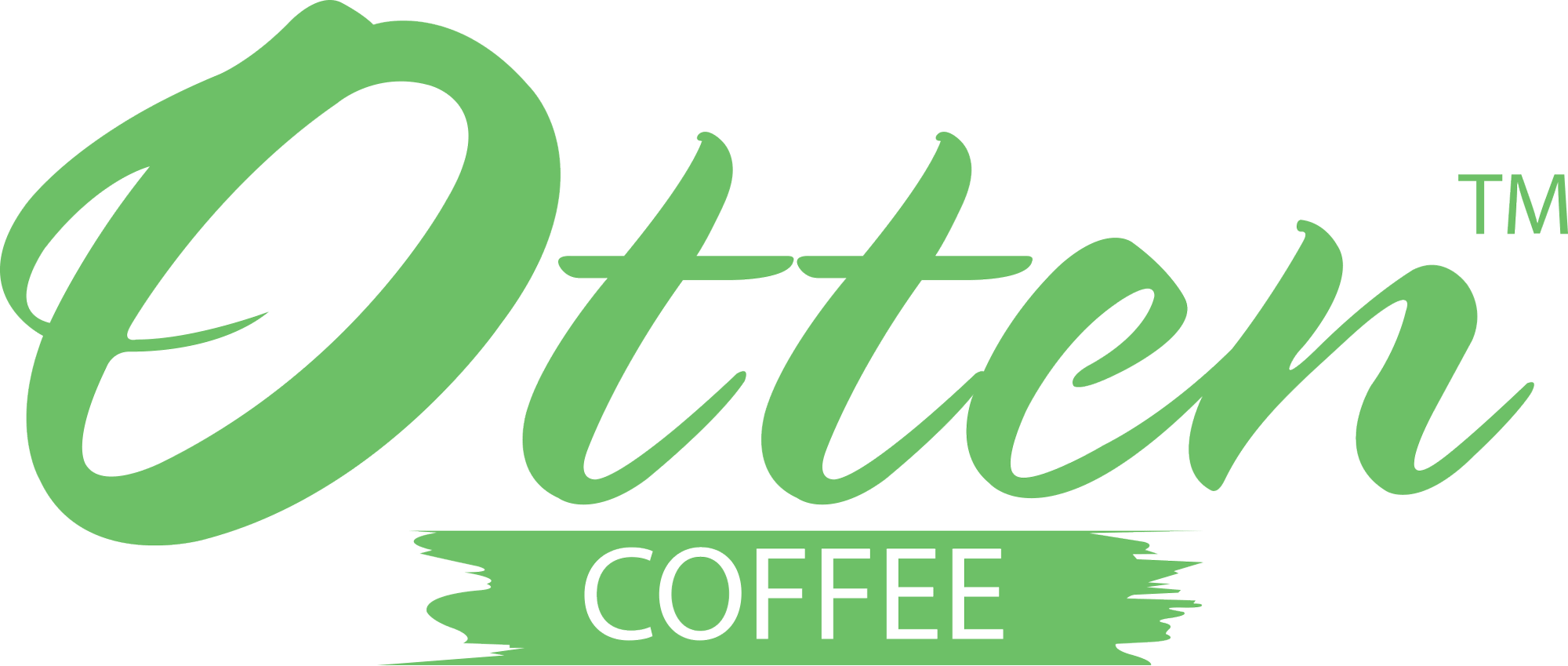
end of slide
Frontend with Vue.js
By Kevin Ongko
Frontend with Vue.js
Kongkow IT Meetup #2
- 1,239