D3.js
data-driven documents
Intro
- Popular open-source project
- A JavaScript library with a focus on data visualizations
- Data-binding is a powerful method
- A combination of building blocks (modules)
- Used for mapping data to the DOM
- Smooth animation transformations
SVG's
- Scalable Vector Graphics
- XML file format for rendering graphics
- Can be rendered in modern browsers
JSON
- JavaScript Object Notation
- Syntax for storing and exchanging data
- Can present data by:
- keys/values (objects)
- array of values
- Is text only, therefore it can be read and used as a data format by any programming language
{
"data": [
{
"id": "X999_Y999",
"from": {
"name": "Tom Brady", "id": "X12"
},
"message": "Looking forward to 2010!",
"actions": [
{
"name": "Comment",
"link": "http://www.facebook.com/X999/posts/Y999"
},
{
"name": "Like",
"link": "http://www.facebook.com/X999/posts/Y999"
}
],
"type": "status",
"created_time": "2010-08-02T21:27:44+0000",
"updated_time": "2010-08-02T21:27:44+0000"
}
]
}
{
"red":"#f00",
"green":"#0f0",
"blue":"#00f",
"cyan":"#0ff",
"magenta":"#f0f",
"yellow":"#ff0",
"black":"#000"
}
{
"colorsArray":[{
"red":"#f00",
"green":"#0f0",
"blue":"#00f",
"cyan":"#0ff",
"magenta":"#f0f",
"yellow":"#ff0",
"black":"#000"
}
]
}
Built-in Request methods
Getting data from another file into an application
- d3.csv (most used)
- d3.json (most used)
- d3.html
- d3.tsv
- d3.text
- d3.xml
Example of methods
// JSON method
d3.json('path-to-file.json', function(error, data){
});
// CSV method
d3.csv('path-to-file.csv', function(error, data){
});
// HTML method
d3.html('path-to-file.html', function(error, data){
});
// text method
d3.text('path-to-file.txt', function(error, data){
});
// TSV method
d3.tsv('path-to-file.tsv', function(error, data){
});
// XML method
d3.xml('path-to-file.xml', function(error, data){
});
d3.json method
// D3 JSON method
d3.json('path-to-file.json', function(error, data){
if ( error ) {
// handle the error
}
// do something with the loaded data
});
- First argument is a callback to a file or data object
- Second argument is a callback function and is only executed after the request returns data or fails
Data-binding
- D3 does not store data within variables
- D3 stores data within the DOM selectors by calling the selection.data() method
- You can create elements in the DOM based on data
Simple data-binding
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://d3js.org/d3.v4.min.js"></script>
</head>
<body>
<ul>
<li>Lorem ipsum</li>
<li>Lorem ipsum</li>
<li>Lorem ipsum</li>
<li>Lorem ipsum</li>
</ul>
</body>
</html>
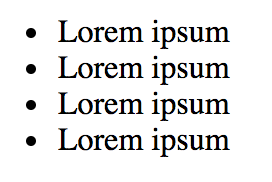
// Data to be bind
var dummyData = [2, 30, 45, 11];
// Select all <li>
d3.selectAll('li')
// Bind data from Array with the data method
.data(dummyData)
// Pass the data to <li> and concat px to the data to change styling
.style('margin-left', function(data) {
return data + 'px';
});
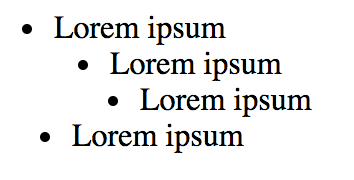
Selections
-
You first need to select an element because you can change or modify it
- Takes valid CSS selectors as parameters
- Uses 2 primary methods
// Returns the first element matching the CSS selector given.
d3.select('div')
d3.select('#name')
// Returns a selection in an array that contains
// all elements matching the CSS selector given.
d3.selectAll('div')
d3.selectAll('#name')
Changing attributes
- Can be used to adjust attributes for SVG's and non-SVG's such as size or position
- Can be combined with data-binding and mouse events
// method
.attr()
// Needs 2 values: attribute that needs to be changed and the new value
selection.attr("attribute string", new value);
// Example
circle.attr("r", 55);
Changing styles
- Can be used to adjust styling for SVG's and non-SVG's such as size or position
- D3 can utilize these new changes without the need of a patch
- Can be combined with data-binding and mouse events
// method
.style()
// Needs 2 values: CSS style that needs to be changed and the new value
selection.style("CSS Style string", new value);
// Example
circle.style("fill", "#FF0000");
circle.style("stroke", "#000000");
Transitions
- Used on a selection to allow it's operators to apply smoothly over time
- By default it starts and last for a duration of 250ms
// Select an element and chain transition to it
d3.select('div').transition();
// Select a div element and give it the ease transition
d3.select('div')
.transition()
.ease('bounce');
D3.js
By Kim Massaro
D3.js
A simple introduction to D3.js
- 744