Na froncie bez zmian?
Kuba Waliński
Kuba Waliński
- kubawalinski@gmail.com
- @kubawalinski
- github.com/kubawalinski
- blog.kubawalinski.com




JavaScript?!

But one day I got it...

Follow prophet Douglas
JavaScript: The Good Parts
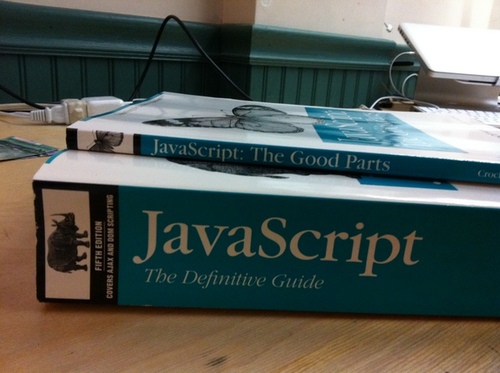
An inconvenient API
The Theory of the DOM
by Douglas Crockford

jQuery to the rescue
This is awesome!
I know css, so I can now program in JavaScript!
Today we already know that

node.js
JavaScript front and back
Knockout.js
promising beginnings
Angular.js
the elephant in the room
And then...
all hell broke loose
as JavaScript popularity went through the roof
React
Angular 2
Aurelia
etc. etc.
Yet Another JS Framework was released today
And I am OK with that
by Judah Gabriel Himango

The state of JavaScript
in 2015
by Jimmy Breck-Mckye
JavaScript a few years ago


JavaScript today
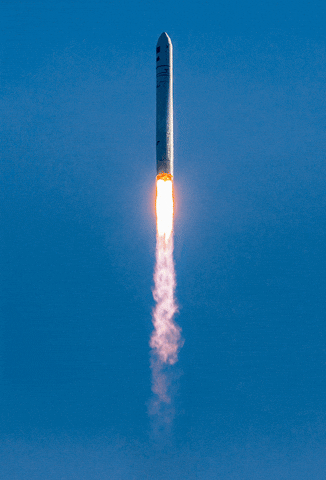
(Fat) Arrow Functions

Arrow Functions
console.log([1,2,3,4].map(x=>x*2)); //[2,4,6,8] var square = x => x*x; console.log(square); //[Function] console.log(square(4)); //16 console.log(square(3)); //9
console.log([1,2,3,4].map(function(x) { return x * 2 })); //[2,4,6,8] var square = function(x){ return x*x; }; console.log(square(4)); //16 console.log(square(3)); //9
Solving "this" problems

#1 reason for facepalms in JavaScript
Arrow Functions
var name = "Bob"; var bob = { name: name, friends: ["John", "Tom"], printFriends: function() { this.friends.forEach(function(f) { console.log(this.name + " knows " + f); }); } }; bob.printFriends(); //TypeError: Cannot read property 'name' of undefined
var name = "Bob"; var bob = { name, friends: ["John", "Tom"], printFriends() { this.friends.forEach(f => { console.log(this.name + " knows " + f); }); } }; bob.printFriends(); //Bob knows John //Bob knows Tom
var name = "Bob"; var bob = { name: name, friends: ["John", "Tom"], printFriends: function() { var self = this; this.friends.forEach(function(f) { console.log(self.name + " knows " + f); }); } }; bob.printFriends(); //Bob knows John //Bob knows Tom
Lexical this
Function scope and var

#2 reason for facepalms in JavaScript
let & const
function() { { console.log(x); //should throw a ReferenceError let x = "start"; y = "start"; console.log(x,y); // start start { const z = "permanent"; //z = "can't touch this"; x = "inner"; var y = "works everywhere"; console.log(x,y,z); // inner works everywhere permanent } //let x = "new value"; //illegal } //x = "end"; y = "end"; console.log(y); // end }();
Classes
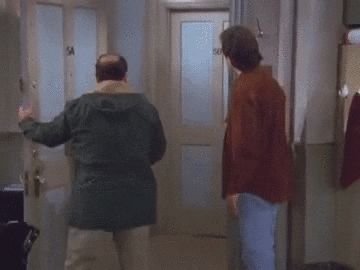
a welcome addition?
Classes
class Vehicle { constructor(name) { this.kind = 'Vehicle'; this.name = name; } printName() { console.log(this.name); } } class Car extends Vehicle { constructor(name) { super(name); this.kind = 'Car'; } }
Easier string manipulation

Template Strings
console.log(`In JavaScript '\t\t' is a couple of tabs.`); // In JavaScript ' ' is a couple of tabs. console.log(`In JavaScript this is not legal.`); // In JavaScript this is // not legal. var name = "Bob", time = "today"; console.log(`Hello ${name}, how are you ${time}?`); // Hello Bob, how are you today? var car = { make: "Mazda", model: "RX-8", production: [2007,2008,2009] }; console.log(`Production started in ${car.production[0]}.`); // Production started in 2007.
Destructuring

Destructuring
var [a, , b, c=4] = [1,2,3]; console.log(a,b,c); // 1 3 4 var {w,x,y,z:surprise} = {x:"this", y:"is", z:"amazing"} console.log(w,x,y,surprise); // undefined 'this' 'is' 'amazing' var getPerson = () => { return {name: "Kuba", company:"ABB"} }; var {name: myName} = getPerson(); console.log(myName); // Kuba function g({name: x, surname}) { console.log(x, surname); } g({name: "Tom", surname: "Jones"}); // Tom Jones
More syntactic sugar

Default / Rest / Spread
function f(x, y=12, z=2*y) { console.log(x,y,z); } f(1,2,3); // 1 2 3 f(5,8); // 5 8 16 f(4); // 4 12 24 function g(x, ...y) { console.log(y); } g(3, "hello", true); // ["hello", true] function h(x, y, z) { console.log(x + y + z); } h(...[1,2,3]); // 6
Modules
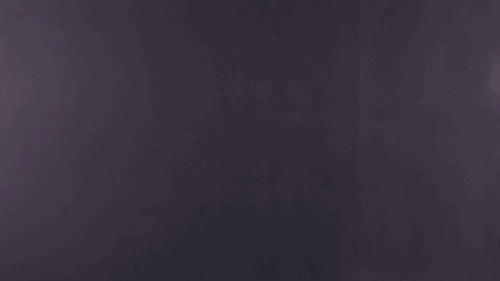
Modules
// lib/math.js export function sum(x, y) { return x + y; } export var pi = 3.141593; // app.js import * as math from "lib/math"; alert("2pi = " + math.sum(math.pi, math.pi)); // otherApp.js import {sum, pi} from "lib/math"; alert("2pi = " + sum(pi, pi));
Modules
// lib/math.js export function sum(x, y) { return x + y; } export var pi = 3.141593; // lib/mathplusplus.js export * from "lib/math"; export var e = 2.71828182846; export default function(x) { return Math.exp(x); } // app.js import exp, {pi, e} from "lib/mathplusplus"; alert("e**pi + e = " + (exp(pi) + e) );
Thanks!
questions + feedback

Na froncie bez zmian?
By Kuba Waliński
Na froncie bez zmian?
- 817