

A brief introduction to:
Python

History
-
Guido van Rossum in early 90s
-
Named after the comedy group Monty Python
-
Official release 1991
-
Manged by Python Software Fundation
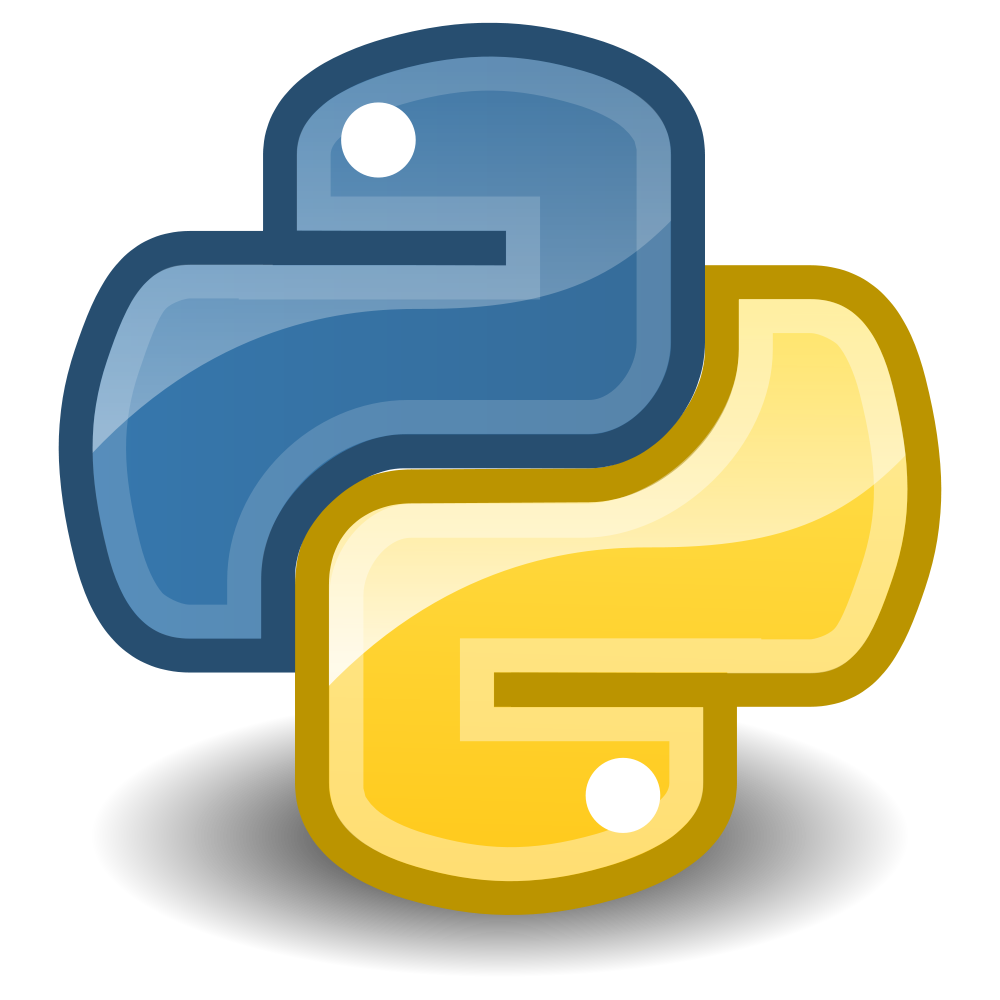

Features
Easy-to-Learn
Python has surpassed Java as the top language used to introduce U.S. students to programming and computer science


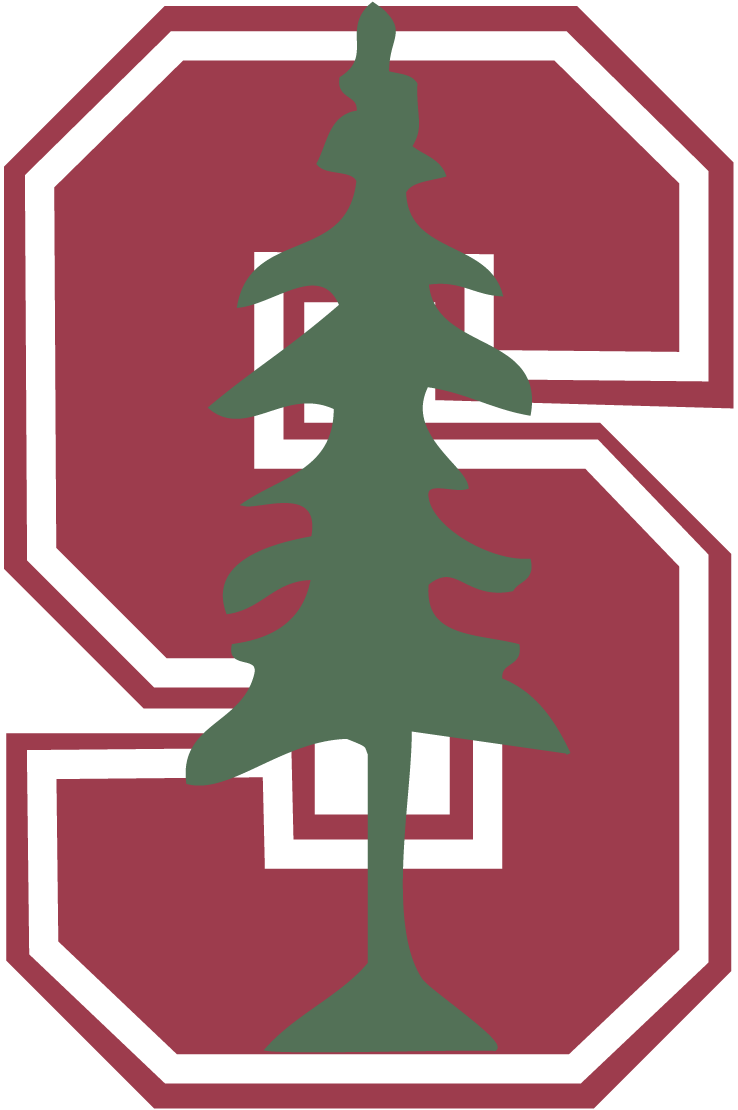

Rapid Development
Python let's you get more done with less code and less time.


Who is using python...
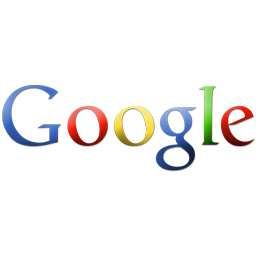
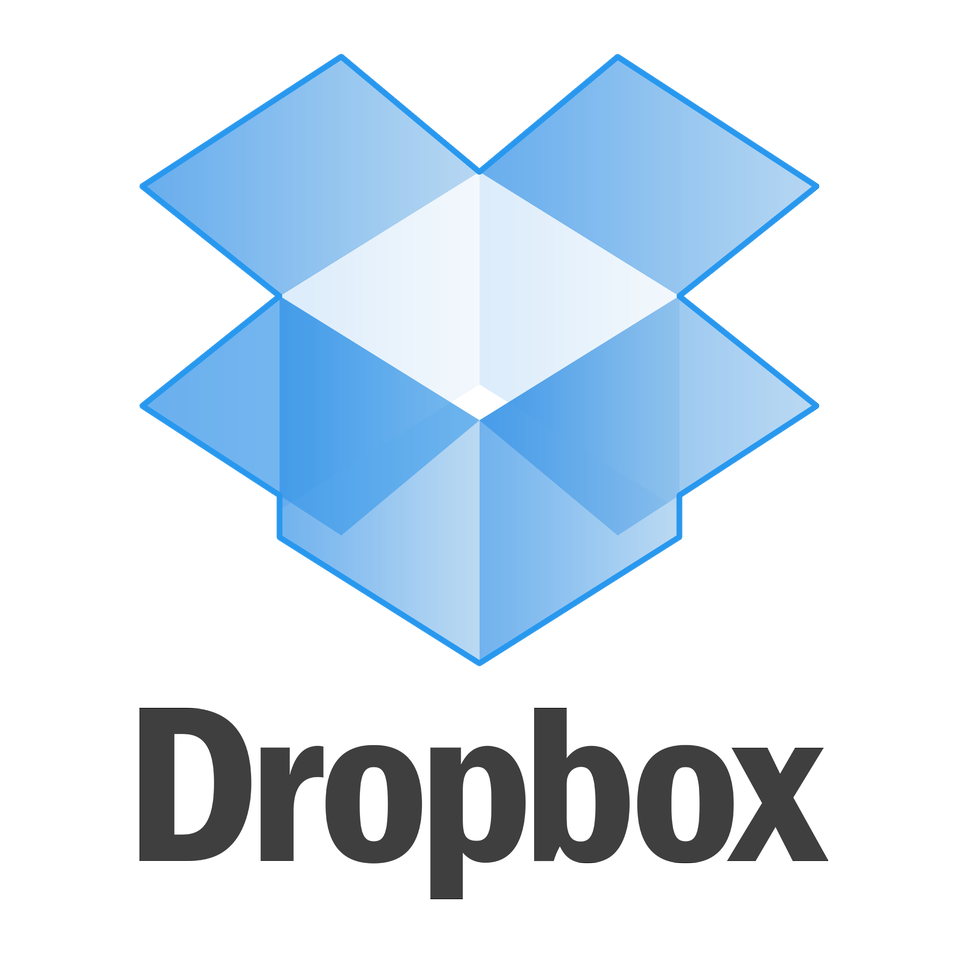

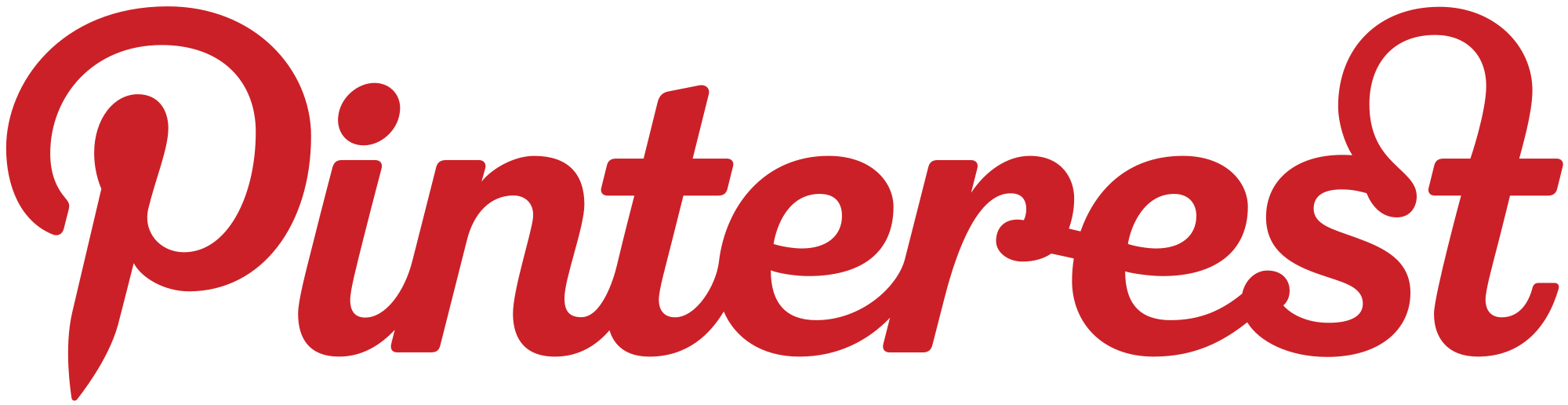



Functionality of Python
Web Development
Desktop GUIs
Scientific and Numeric Programming
Networking Programming
Databases Access

Projects through python






Python 2.x or 3.x ?...
Python 2.x is legacy, Python 3.x is the present and future of the language
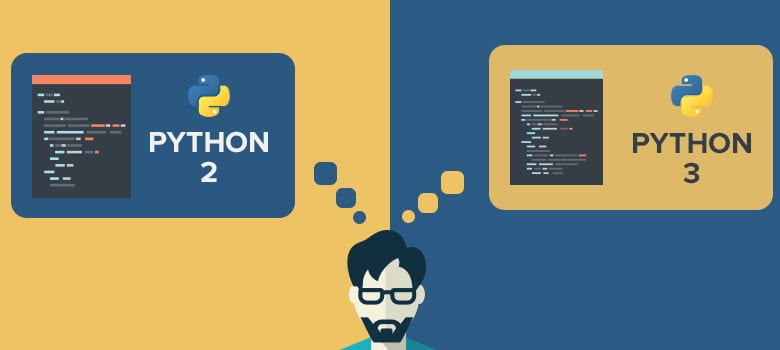

The print function
Very trivial, and the change in the print-syntax is probably the most widely known change
print 'Python', python_version()
print 'Hello, World!'
print('Hello, World!')
print "text", ; print 'print more text on the same line'
Python 2
Python 3
print('Python', python_version())
print('Hello, World!')
print("some text,", end="")
print(' print more text on the same line')

For-loop variables and the global namespace leak
print 'Python', python_version()
i = 1
print 'before: i =', i
print 'comprehension: ', [i for i in range(5)]
print 'after: i =', i
print('Python', python_version())
i = 1
print('before: i =', i)
print('comprehension:', [i for i in range(5)])
print('after: i =', i)
Conclusion...
Python 3.4.1
before: i = 1
comprehension: [0, 1, 2, 3, 4]
after: i = 1
Python 2.7.6
before: i = 1
comprehension: [0, 1, 2, 3, 4]
after: i = 4

Basic syntax
Variables
int_example = 3
string_example = 'Holis'
list_ example = [1,2,3]
list_ example = [1,2,3,4,5,6,7]
print(list_example[2:4]))
Using a list.
Output: [ 3, 4 ,5 ]

Loop
list_example = [1,2,3,4,5,6,7,8,9]
for i in range(0, len(list_example)):
print(list_example[i])
for number in list_example:
print(number)
day_of_the_week = 'Monday'
if(day_of_the_week == 'Monday'):
print('Today are Monday talks!')
elif(day_of_the_week == 'Wednesdays'):
print('Today are Coding_Wednesdays!')
else:
print(':(')
If-Statements

methods
classes
def method(x,y):
sum = x + y
print(sum)
method(4,2)
class Employee:
'Common base class for all employees'
empCount = 0
def __init__(self, name, salary):
self.name = name
self.salary = salary
#This would create first object of Employee class
emp1 = Employee("Zara", 2000)
#This would create second object of Employee class
emp2 = Employee("Manni", 5000)
creating a class
instance

Code Style
Python Enhancement Proposals (PEPs)
PEP 8 is the de-facto code style guide for Python.
One reason for Python code to be easily read and understood is its relatively complete set of Code Style guidelines and “Pythonic” idioms.
python
Give a try to


Thanks for coming!
Monday Talk 04.06.15
By Luis Hernandez
Monday Talk 04.06.15
- 513