TypeScript
TypeScript = types + ECMAScript
Why JavsScript is bad?
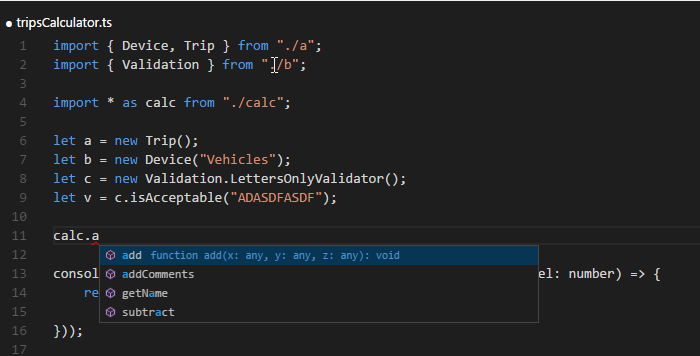
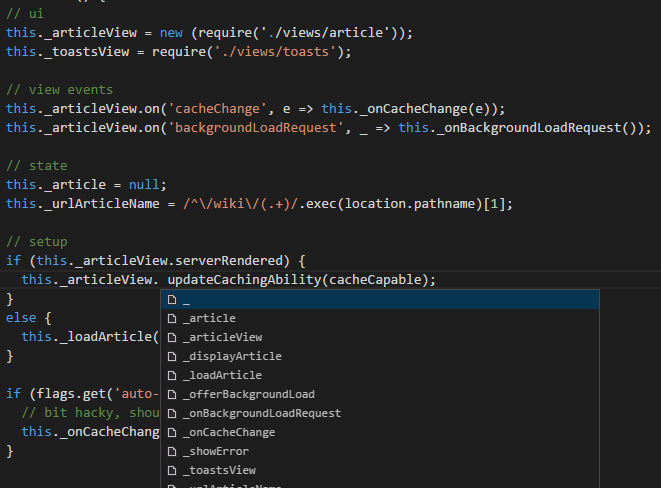
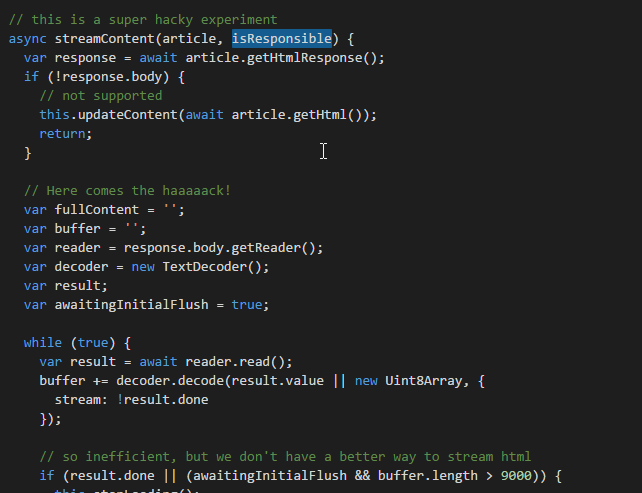
Questions for developer of the module
- What arguments are important
- What types are valid
- What options are valid
- What settings are optional
Questions for user of the module
- Is it save to add one more argument
- Can i remove argument
- What if I return something else
TypeScript saves your live
ES2015 + Types
Types
- Boolean
- Number
- String
- Symbol
- Array
- Tuple
- Void
- Any
- Enum
- Interface
Types
var a: number = 3;
var b: number[] = [1,2,3];
var a: number = <number>b;
function (a?: number, isTrue: boolean = false) { ... }
function (a?: number|string, isTrue: boolean = false) { ... }
Types
var a: any = {};
any is a lie)))
Types

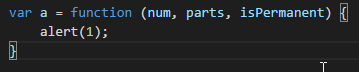
ES2015 transpiler
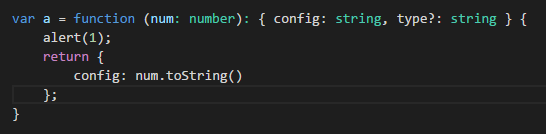
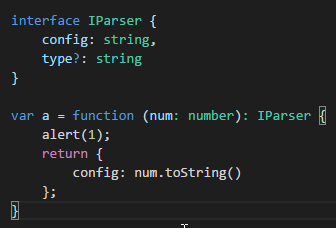
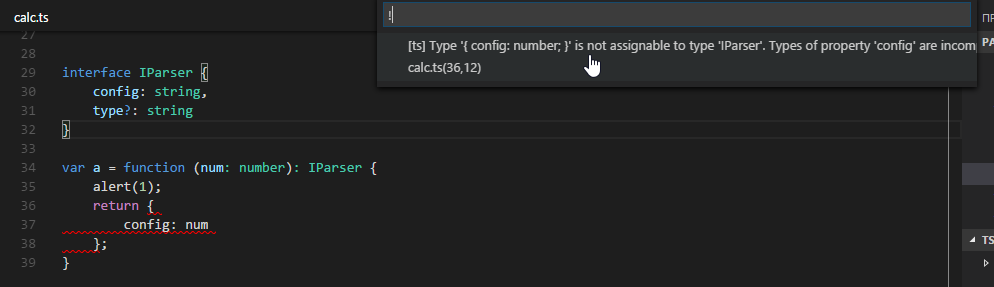
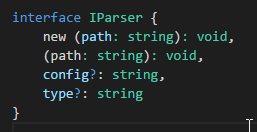
Classes
- private, protected, public
- abstract
- implements, extends
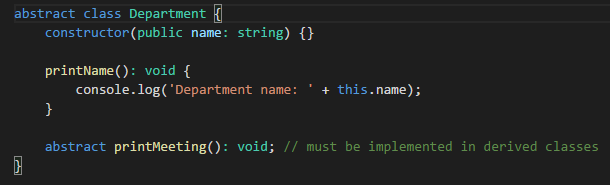
Generics
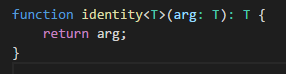

namespace
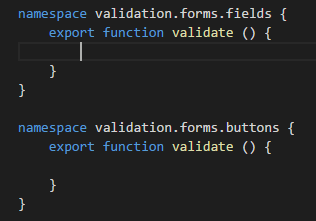
namespace
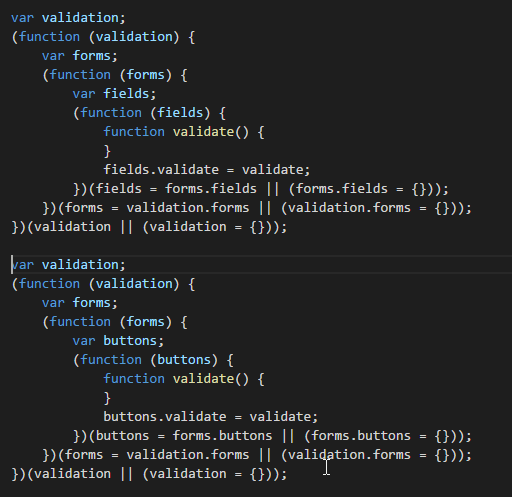
Future
- Mixins
- Decorators
Demo
Libs
JQuery
Webix
window
console
definition files
http://definitelytyped.org/tsd/
lib.d.ts
new Date
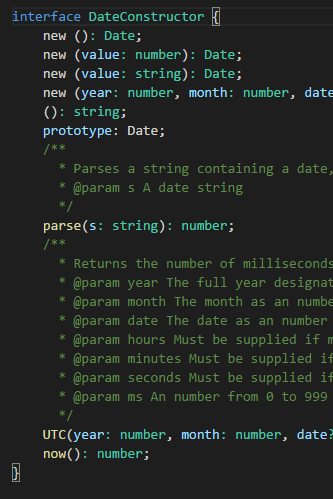
How can I start working with ts
WebStorm
VS + NuGet
VS Code
Compile options
http://www.typescriptlang.org/docs/handbook/tsconfig.json.html
Flags
http://www.typescriptlang.org/docs/handbook/compiler-options.html
Experience...
Performance
ES2015 vs ES5
https://kpdecker.github.io/six-speed/
Modules
Rules
- local scope
- importing
- exporting
- export by reference
- accessing by path
Local scope
var a = 2;
(function(){
var a = 2;
return {
getName: function(){
return "vlad";
}
}
})()
Importing
import defaultMember from "module-name"; import * as name from "module-name"; import { member } from "module-name"; import { member as alias } from "module-name"; import { member1 , member2 } from "module-name"; import { member1 , member2 as alias2 , [...] } from "module-name"; import defaultMember, { member [ , [...] ] } from "module-name"; import defaultMember, * as name from "module-name"; import "module-name";
Exporting
export { name1, name2, …, nameN }; export { variable1 as name1, variable2 as name2, …, nameN }; export let name1, name2, …, nameN; export let name1 = …, name2 = …, …, nameN; export default expression; export default function (…) { … } export default function name1(…) { … } export { name1 as default, … };
Exporting
export function a(){}
export class a { }
export let a = 2;
let a = 3;
export a;
let a = 3;
export { a };
let a = 3;
export { a as num };
export default let a = 5;
Importing
import a from "";
What is in "a"?
export default let a = 6
import b from "";
b.a === 6;
import * as b from "";
b.a === 6;
import { a, num as b } from ""
export let a;
export let function num(){}
by reference
import {a} from "";
a = 2
export let a = 4;
import {a} from "";
a === 2
Loading
it's ES2015, baby
module tag + HTTP2
AMD
CommonJS
UMD
ES2015
My Solution + commonjs
(fucntion (require, exports) {
//code
})( getRequire(path), getExports(path))
Questions?
TypeScript
TypeScript
By Vladimir
TypeScript
- 124