A Gentle Intro
to Coding
slides available here: https://goo.gl/5HKE2J
Introductions
Who am i
Maëlys

(mah
-eh
-liss)
she/her pronouns
10 years professional programming experience
I like corgis
Who am i
Quinn

he/him pronouns
loves ice cream
LGBTQ+ organizer, tech inept
Who am i
Mado

they/them
Planning on making disability/activism-related software once I am powerful enough
Programmer in training
Who am i
Serena

loves going out for ice cream
has been in tech a long time and is pretty much a tech granny by now
former pet photographer
territorial acknowledgement
We are meeting on aboriginal land that has been inhabited by Indigenous peoples from the beginning. This particular event is taking place on the traditional unceded territory of the Algonquin Anishnaabeg people.
I recognize and deeply appreciate their historic connection to this place. I also recognize the contributions that Métis, Inuit, and other Indigenous peoples have made, both in shaping and strengthening this community, and our province and country as a whole.
As a settler, I am grateful for the opportunity to meet here and thank all the generations of people who have taken care of this land for thousands of years.
I would encourage you not to dismiss this territorial acknowledgement, but understand it as part of a larger responsibility we all share not to be silent, for silence only protects the status quo.
And the status quo just won't do.

- Read the Truth and Reconciliation Final Report
what you can do
Source: Ontario Federation of Labour Aboriginal Circle
- Sponsor and promote Indigenous events and advocacy
- Support the calls to action
- Acknowledge Indigenous territory at all of your meetings
overview of today's content
the theme for this workshop is Dipping Feet in code
We will cover
what software developers do
Programming languages
A BIT OF Coding!
software development
Let's talk perception

Reality





Coding is HARD at first BECAUSE IT'S UNFAMILIAR
Pro developers find doing UNFAMILIAR Program-my things hard All the time
This is expected!
we're all at various levels of familiarity
in this workshop
the point isn't to keep up with the person next to you
It's to be a little ahead of where you were
there is no shame being at whatever point you are at
You're here and that's really great
We are here to help you at the point you are at

I know it can be RLY intimidating to ask questions
try to remind yourself
All questions are good questions
if you're thinking it so is someone else
And you'll be helping them too

~please turn on your Laptop~
Programming Languages
programming languages are for humans
to tell computers What to do
sometimes they look like this

or this

but they more or less work the same
you write instructions for the computer in a language for humans (programming language)
then a program turns it into something the computer understands (Machine Code)


Programming Language
Readable by Humans
Machine Code
Readable by Computers
Software
Today we will learn a programming language called python
We're using it because it's
Easier to learn
Used in academia
Loved by Pro Developers
Used in Web development
Questions?
Getting set-up to code
Make sure tools are installed
Python:
Visual Studio Code:
Install the latest version of Python & Visual Studio Code!
please Launch visual studio code
visual studio code is what we use to write code
it's Sort of like the microsoft word of writing code
We're going to do two things
Create a project in visual studio code
make sure we can use python

Dismiss errors related to git.
Click open folder

Dismiss errors related to git.

Create a folder called pythonworkshop

click to select the folder

our project is now created
now to make sure python works
Click View Then integrated terminal


TYPE "PYTHON" IN THE TERMINAL
TYPE "py.exe" IN THE TERMINAL
Mac/
Linux
Windows

Does anyone not see this?
write " " in the terminal and press enter
exit()

creating our first program
we will create a file
that contains our code
and then have the computer run THAT CODE

click on the new file icon
write "hello.py"
And press enter

we now have a file for our code
This file is called "hello.py"
files that end in .py contain code in the python programming language
The file doesn't have anything in it right now


print("hello")
write AND SAVE
print() tells the computer to show text on the screen
this is our code
it was written in python, a language for humans
now we will run it in a program so the computer will understand it
RUN "PYTHON hello.py" IN THE TERMINAL
Mac/
Linux
Windows
RUN "py.exe hello.py" IN THE TERMINAL

does anyone not see "hello" In the terminal
Now put something else inside Print() other than "Hello"
IDEAS
print("hi there!")
print(1234)
print(1 + 1)
print(4.3 - 2.1)
You just wrote a program!
next up are Three topics
these are fundamental almost all programming languages
functions
variables
comments
Using functions
functions are a shortcut to code written elsewhere
print("hi there!")
print(1234)
print(1 + 1)
print(4.3 - 2.1)
remember this?
print() is a function
Print shows things on the screen
you didn't tell the computer how to show something on the screen
someone else wrote that code and it exists elsewhere
you use a shortcut to USE that function
Python comes with a bunch of functions you can use
Create a new file called "functions.py"


abs(-5)
write AND SAVE
We'LL explain what this function does in a bit
just know that abs() outputs a number
Let's run this program
RUN "PYTHON functions.py" IN THE TERMINAL
Mac/
Linux
Windows
RUN "py.exe functions.py" IN THE TERMINAL
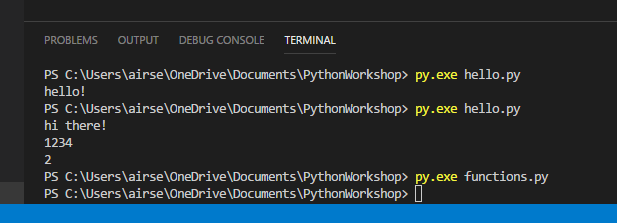
There's nothing shown!
How could we show something on the screen?
print(abs(-5))

Can anyone say what abs() does?
now it's your turn
you now know the functions
abs() and print()
See what these ones do
len("the dog")
round(5.3)
max(1, 2, 3, 4)
variables
sometimes it's useful for the computer to remember things like numbers and text
That's what variables are for
message = "my name is Maëlys"
print(message)
For example
"Message" Is a variable
a = 2
b = 6
c = a + b
print(c)
For example
"a", "B" & "C" ARE variableS
Let's play with this a bit

Create a new file called "variables.py"
a = 2
b = 3
print(a * b)
put this code in the file

RUN "PYTHON variables.py" IN THE TERMINAL
Mac/
Linux
Windows
RUN "py.exe variables.py"
IN THE TERMINAL
What does "*" do?

It multiplies
now it's your turn
See what "**", "/", "%" DOES
a = 10
b = 7
print(a + b)
print(a - b)
print(a * b)
print(a / b)
print(a ** b)
print(a % b)
comments
sometimes programmers want to leave notes for themselves in code
To help them remember what things do
Because it's not always obvious

comments are a way to add notes in code
Remember this?
a = 10
b = 7
print(a + b)
print(a - b)
print(a * b)
print(a / b)
print(a ** b)
print(a % b)
We can add comments
a = 10
b = 7
# Adds two numbers
print(a + b)
# Subtracts two numbers
print(a - b)
# Multiplies two numbers
print(a * b)
much more clear!
in python, comments are added by starting a line with "#"
now it's your turn
add comments To Your "Variables.Py" file
a = 10
b = 7
# Adds two numbers
print(a + b)
# Subtracts two numbers
print(a - b)
# Multiplies two numbers
print(a * b)

creating functions
Earlier We looked at using functions other people wrote
functions like print() and len()
We can also create functions too
first though let's understand what a functions does
Input:
1
3
Output:
4
Function to add numbers
+
functions take input, do something to it, then have output
here's a function to add two numbers In python
def add_numbers(a, b):
# add numbers
c = a + b
# put the result in the output
return c
# Use the function
result = add_numbers(10, 2)
print(result)
Let's look at the parts of a function
def add_numbers(a, b):
# add numbers
c = a + b
# put the result in the output
return c
input
output
indentation
name of function
each line of code in functions start with indentation
why would we need indentation?
because python can't tell where the function ends
def add_numbers(a, b):
# add numbers
c = a + b
# put the result in the output
return c
# Use the function
result = add_numbers(10, 2)
print(result)
use the tab key to add indentation
python also can't tell what the output should be
def add_numbers(a, b):
# add numbers
c = a + b
# put the result in the output
return c
return
So "return" is put to say to output a variable
Let's create a function
this function will multiply three numbers
Create a new file called "createfunctions.py"

write this function in your file
def multiply(a, b, c):
# multiply numbers
output = a * b * c
return output
We're just missing one thing to make it work
a line of code to use the function
because functions don't do anything until they're used
ADD thIS code to use the function
def multiply(a, b, c):
# multiply numbers
output = a * b * c
return output
# use the function
print(multiply(2, 3, 4))

now run the program
does it do what you expect?
now it's your turn
Create your own function
ideas
dividing two numbers
giving the square of a number
that's it for this week!
next week
reading files
user input
learning resources
Questions?
Thank you to Serena & Mado
And to quinn and the Algoquin pride centre for this space
slides available here: https://goo.gl/5HKE2J
thank you!
my website:
www.maelys.bio
A Gentle Intro to Coding
By Maëlys
A Gentle Intro to Coding
This workshop is intended to help non-programmers gain a bit of familiarity with what software development is and learn a bit of coding.
- 2,190