Unit Testing
By: Manjunath C B
with

Contents
1. Introduction
2. Necessary of testing
3. Types of testing
4. Thinking perspective on tests
5. Introduction to Junit with examples
6. Unit testing at ShieldSquare
Introduction
- A unit test is a piece of code written by a developer that exercises a very small, specific area of functionality of the code being tested. .
- Unit tests are performed to prove that a piece of code does what the developer thinks it should do.

"We debug
why to test?"

Debugging is not Testing
Necessity of Testing
- Unit testing makes life easier. It makes designs better .
- Reduces the amount of time spend on debugging.
- A unit test behaves as executable documentation,
- Team members can look at the tests for examples of how to use your code.
- It’s not polite to expect others to clean up our own messes, and in extreme cases submitting large volumes of buggy code can become a “career limiting” move.
- Benefits QA group .

Generic cost model
Different testing levels.
- Functional testing
- Unit testing
- Acceptance testing,
- Integration Testing
- validation and verification etc.
Unit testing is lowest-level testing that’s designed for programmers.
"Developers have to perform Unit Testing"
Who does testing?
When to perform testing ?
- “Test now” versus “Test later.”
- Parallel testing is best practiced for unit testing
- “Pay-as-you-go”
- It takes too much time to write the tests if Test later is considered.

Thinking perspective on tests
- Test cases have to be planned for "likely to break" scenarios.
- With enough experience, you start to get a feel for those things that are “likely to break,”.
- Check for Boundary conditions, Force error conditions to happen, Performance characteristics within bounds.
Example:
[7, 8, 9] → 9 , [8, 9, 7] → 9 , [9, 7, 8] → 9 , [1] → 1
Check for duplicate numbers?
• [7, 9, 8, 9] → 9
Check for negative numbers:
• [-9, -8, -7] → -7
static int largest(int[] list);
[ 7, 8 , 9 ]
Function to test
Tests

JUnit is an open source framework that has been designed for the purpose of writing and running tests in the Java programming language.
JUnit is a regression-testing framework that developers can use to write unit tests as they develop systems.
Unit provides also a graphical user interface ( GUI ) which makes it possible to write and test source code quickly and easily.

Installation


Example



Test Practices on Naming convention and Folder struucture


Test Practices on Naming convention and Folder struucture



Example demonstrating Junit test and folder structure

Annotations
@Test
@Before
@After
@BeforeClass
@AfterClass
@Mock

Example demonstrating Junit Annotations
Assertion methods
- assertEquals()
- assertTrue()
- assertFalse()
- assertNull()
- assertArrayEquals()
- assertSame()
- etc....

Example demonstrating Junit Assert, Exception Handling
What if the method doesn't return a value.
It must have some other side effect such as,
- creating a file,
- modifying a member variable,
- modifying the state
- etc...
Testing void methods
You need to test whether that side effect has taken place
Testing dependent methods


Testing dependent methods
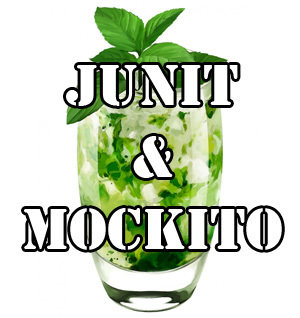
Mockito
- Mockito is a mocking framework
- JAVA-based library that is used for effective unit testing of JAVA applications.
- Mockito is used to mock interfaces so that a dummy functionality can be added to a mock interface that can be used in unit testing.
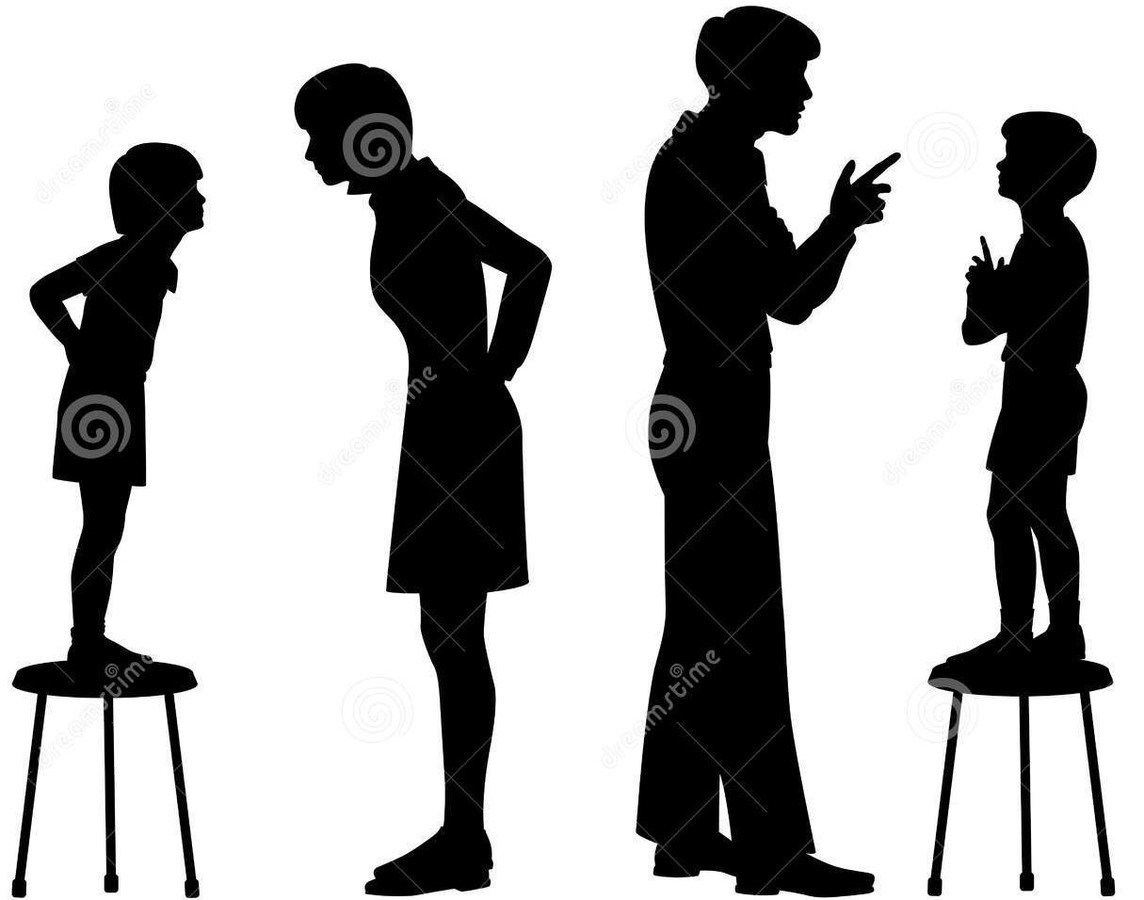
Installing Mockito:


Mock Test Example :
Testing place order()

Mock Test Example :
Testing place order() by mocking order_DAO()

Mock Test Example
Test coverage
-
Test coverage measures the amount of testing performed by a set of test.
-
which parts of a program are actually executed when running the test
-
Its good to have atleast 80% test coverage
Eclipse test coverage plugin :

Test Coverage and Test Suit Example

Unit Testing @
Most of the ShieldSquare modules interact with Redis and Mongo. So there is a need for Mocking jedis and mongo.
Fongo : Library for mocking mongo(fake mongo)
https://github.com/fakemongo/fongo
Mock Jedis : Library for mocking redis
Refactoring at shieldsquare: Often changes in the module code.
Conclusion
Unit testing is low level testing. It has to be performed by developers.Unit testing is to be done in parallel to development. It reduces the cost in long run. Junit api is used for unit testing in java development. Its syntax and usage is easy to understand and implement. Mockito is used for mocking objects. Fongo and Mock jedis are used to mock mongo and redis. Which are well suited for shield square development. Testing void methods has to be done considering its side effects. Good to have more than 80% test coverage. EclEmma plugin is best known for calculating test coverages.


Questions ?



Test Practices on Naming convention and Folder struucture
Junit Presentation
By MANJUNATH BAGEWADI
Junit Presentation
- 1,128