

Rita Krutikova
* Crash course
Graph what?
QL - query language for API
/api/v1/users
/graphql/v1
{
users: [
{
id: 1234
name: ...
email: ...
fullName: ...
}
]
}
query {
users {
id
fullName
}
}
GraphQL
Fetch related data
query {
users {
id
fullName
todos {
id
text
}
}
projects {
name
}
}
GraphQL Schema
type Todo {
id: Int!
text: String!
}
type Query {
users: [User!]
todo(id: String!): Todo
}
type Mutation {
addTodo(text: String!): Todo
}
- Server defines its capabilities
- Client applications fetch them
type User {
id: Int!
email: String!
todos: [Todo!]
}
Type system for the API
Introspection query


Client
Introspection query
Schema in json
- Generate reason types & decoders
- Validate queries at compile time
(meta API call 😉)
Why GraphQL?
-
Response shape is flexible
-
Client fetches only data it needs
-
Smaller payload
-
Strongly typed vs JSON in REST
-
Tooling/discoverability
-
Developer experience




Rita Krutikova

Agenda
- Apollo & Apollo-client
- GraphQL ppx
- Reason apollo hooks
- DEMO
Apollo
-
Apollo is an implementation of GraphQL
- Server in NodeJs
- Clients for Android (Java), iOS (Swift), Web (Js)
-
Tooling - both server & client
- Types and schema generators
- Community driven (OSS)
React apollo
State management library
- Supports any GraphQL API
-
Keep track of the query state
- loading ⏳, error ⚠️, data 💾
- Update UI when state changes
- Keeps data in a normalized cache
- Local state (alt to Redux)
- *No compiler
React-apollo
@apollo/react-hooks
reason-apollo
reason-apollo-hooks
GraphQL ppx
graphql_ppx_re
+

Client setup
GraphQL Query
module Query = [%graphql
{|
query {
todos {
id
text
completed
}
}
|}];
const query = gql`
query {
todos {
id
text
completed
}
}`


GraphQL PPX
- Parses GraphQL json schema (introspection query)
- Compile time validation against schema
- Generates response types & decoders
- Not specific to Apollo
Type-safe GraphQL queries
module Todos = [%graphql{|
query {
todos {
id
text
}
}
|}];
- Response type is a Js.t object from the schema
- Directives modify how the result of a query is parsed
{
.
"todos":
Js.Array.t({
.
"id": string,
"text": string,
}),
};
Response
Fragments
module Fragment = [%graphql {|
fragment TodoItem on TodoItem {
id
text
}
|}];
module TodosQuery = [%graphql {|
query {
todos {
...Fragment.TodoItem
}
}
|}];
Apollo Hooks
- useQuery
- useLazyQuery
- useMutation
- useSubscription (web sockets)
- useApolloClient

** Examples show soon-to-be released new amazing API of reason-apollo-hooks 😅
useQuery
module Todos = [%graphql {|...|}];

switch (simple) {
| Loading => <Spinner />
| Data(data) => <TodoList data />
| NoData => React.null
| Error(_) => <Error />
}
* simple
let (simple, full) = useQuery((module Todos));
module Todo = [%graphql {|
query ($id: Int!) ...
} |}];
let variables = Todo.make(~id=42, ())##variables;
let (s, _) = useQuery(~variables, (module Todo));

useQuery
* variables
useQuery
let (_, full) = useQuery((module Todos));
full.fetchMore(...);
full.refetch();

* advanced
// pagination
// imperative refresh
DEMO
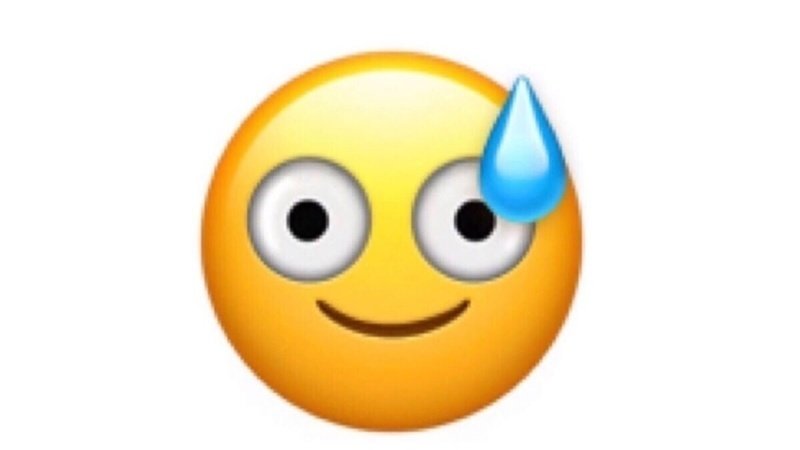
* mild panic
Summary
- GraphQL ppx for type-safe queries
- Validates queries & mutations
- Generates response types & decoders
- Reason apollo hooks
- State management & GraphQL client
- Unopinionated & flexible
- Easy to get started with
- No compiler optimizations
Thanks!
ReasonML 4ever
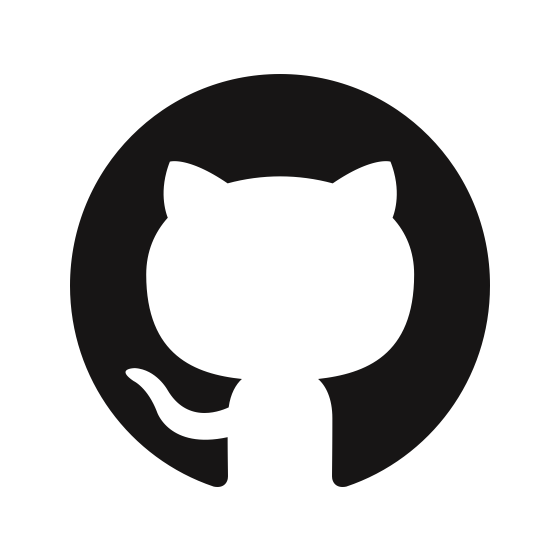


Questions? 🤔
GraphQL: Apollo + Reason
By margaretkru
GraphQL: Apollo + Reason
- 206