- Why should you care about security ?
- Who are you defending against ?
- What are the types of web attacks and vulnerabilities ?
- How do you defend against web attacks ?
Why should you care about security?
Who are you defending against ?
Motives
- Money
- Political or ideology
- Fun
What are the type of web attacks and vulnerabilities ?
- Broken Access Control
- Cryptographic Failures
- Injection
- Insecure Design
- Security Misconfiguration
- Vulnerable and Outdated Components
- Identification and Authentication Failures
- Software and Data Integrity Failures
- Security Logging and Monitoring Failures
- Server-Side Request Forgery

(Open Worldwide Application Security Project)
- Out-of-bounds Write
- Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
- Out-of-bounds Read
- Improper Input Validation
- Improper Neutralization of Special Elements used in an OS Command ('OS Command Injection')
- Improper Neutralization of Special Elements used in an SQL Command ('SQL Injection')
- Use After Free
- Improper Limitation of a Pathname to a Restricted Directory ('Path Traversal')
- Cross-Site Request Forgery (CSRF)
- Unrestricted Upload of File with Dangerous Type
- Missing Authentication for Critical Function
- Integer Overflow or Wraparound
- Deserialization of Untrusted Data
- Improper Authentication
- NULL Pointer Dereference
- Use of Hard-coded Credentials
- Improper Restriction of Operations within the Bounds of a Memory Buffer
- Missing Authorization
- Incorrect Default Permissions
- Exposure of Sensitive Information to an Unauthorized Actor
- Insufficiently Protected Credentials
- Incorrect Permission Assignment for Critical Resource
- Improper Restriction of XML External Entity Reference
- Server-Side Request Forgery (SSRF)
- Improper Neutralization of Special Elements used in a Command ('Command Injection')

(Common Weakness Enumeration)
How to defend from web attacks?
Defense
In
Depth
User input fields and output
Not validating user input fields for unsafe characters

Picture source: Web security for developers
User input fields and output
Not validating user input fields for unsafe characters

Picture source: Web security for developers
- Sanitize content received from any user input using a secure sanitizing library eg
-
sanitize-html
-
User input fields and output
import sanitizeHtml from 'sanitize-html';
const html = "<strong>hello world</strong>";
console.log(sanitizeHtml(html));
console.log(sanitizeHtml("<img src=x onerror=alert('img') />"));
console.log(sanitizeHtml("console.log('hello world')"));
console.log(sanitizeHtml("<script>alert('hello world')</script>"));
// Allow only a super restricted set of tags and attributes
const clean = sanitizeHtml(dirty, {
allowedTags: [ 'b', 'i', 'em', 'strong', 'a' ],
allowedAttributes: {
'a': [ 'href' ]
},
allowedIframeHostnames: ['www.youtube.com']
});
User input fields and output
var search = document.getElementById('search').value;
var results = document.getElementById('results');
//unsafe
results.innerHTML = 'You searched for: ' + search;
You searched for: <img src=1 onerror='/* Bad stuff here... */'>
//safe
results.innerText = 'You searched for: ' + search;
- Use innerText instead of innerHTML to display user input
User input fields and output
{{ data }}
//Vue
<div v-html="htmlData"></div>
////React
return <div dangerouslySetInnerHTML={createMarkup()} />;
//Angular
<div [innerHTML]='<a href="#">Unescaped link</a>'</div>
//Vue
{{constructor.constructor('alert(1)')()}}
<teleport to=script:nth-child(2)>alert(1)</teleport></div><script></script>
<component is=script text=alert(1)>
{{$el.ownerDocument.defaultView.alert(1)}}
- Use modern javascript frameworks (with caution)
User input fields and output
Content-Security-Policy: script-src 'self' https://api.foo.com
<meta http-equiv="Content-Security-Policy" content="script-src 'self' https://api.foo.com">
Real world story 📖
British Airways - 2018
https://www.dooble.com/search?q=tesla
https://www.dooble.com/search?q=<script>alert("hacked!")</script>
URL parameters
Not validating query and search params

//const queryString = window.location.search;
const queryString = sanitize(window.location.search);
const urlParams = new URLSearchParams(queryString);
//document.getElementById('searchValue').innerHTML = urlParams.get("q");
document.getElementById('searchValue').innerText = urlParams.get("q");
URL parameters
- Sanitize parameter key values
https://vulnerable-website.com/?__proto__[evilProperty]=payload
targetObject.__proto__.evilProperty = 'payload';
Object.freeze(Object.prototype);
URL parameters
-
Prevent prototype pollution for injection keys like __proto__
- Using an allowlist of permitted keys
- prevent prototype objects from being changed
https://example-website.com/login/home.html?admin=true
https://example-website.com/login/home.html?id=876
URL parameters
Exposure via URL redirect parameters
- Avoid exposure of roles in the the params
- Handle access control for every request of page resource
https://example-website.com/user
https://example-website.com/admin
https://example-website.com/admin_293399288
Page URL pathname
Exposing web page to non-admin
<script>
let isAdmin = false;
.....
if (isAdmin) {
redirectToPage('/admin_293399288');
setHeaderText("Welcome To Admin Page");
}
</script>
Page URL pathname
- Proper access control to restricted pages
- Access control check for every page resource
- Deny access by default
- Functional tests
- use non-disclosing description for variable and function names
Real world story 📖
U.S. Department of Defense website - Sept 2020
Hardcoding
Avoid exposure of secrets and credentials
- hardcoding secrets, passwords, API keys in source code, eg in HTML, Javascript, or any configuration file.
<!-- Use the DB administrator password for testing: f@keP@a$$w0rD -->
// or in script
const myS3Credentials = {
accessKeyId: config('AWSS3AccessKeyID'),
secretAcccessKey: config('AWSS3SecretAccessKey'),
};
Hardcoding
- Store the credentials in
- a configuration file that is not pushed to the code repository.
- in database or cloud service provider or repo hosting platform eg GitHub encrypted secrets, Azure key vaults
- Use scanning tools to scan for accidental exposure of sensitive info in your code , eg Github code scanning
- If a password has been disclosed through the source code: change it.
Error Message
Picture source: Microsoft learn
try {
//code
} catch(error) {
console.log(error);
}

Error Message

Picture source: tutorial.eyehunts.com
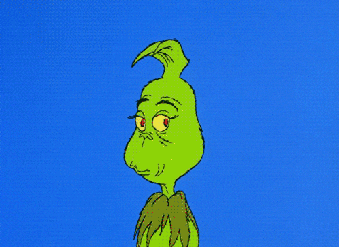
Error Message
- Log generic error message without giving specifics
- disable log visibility in production
- log using standard logging tools securely
- log just enough data to debug
- mask Personal Identifiable Information (PII)
- unit and functional tests
try {
//code
} catch(error) {
console.log("There seems to be an error!");
}
Real world story 📖
Node js libraries hiding crypto miners - Oct 2021
3rd party libraries & data
- Use trusted source of libraries
- use latest stable version
- https://github.com/advisories
- npm health checker https://snyk.io/advisor/
- Update patches libraries
- use repo dependabots to keep a check
-
npm audit
-
npm outdated
-
Sanitize data from 3rd party data or API's
Other areas
- Authentication
- Business logic
- File Uploads
- Iframes
- Network requests
- Local storage
Secure Development Cycle
- Secure coding with tests
- Review code for vulnerabilities
- Use Automated Security Scanning Tools
- Enable dependabots
- Security training and learning
- Spread security awareness
Free online learning resources
- OWASP Top 10
- CWE
- OWASP juice shop - gamified app to learn vulnerabilities
- Portswigger Web security academy
- OWASP Security knowledge framework
- Snyk learning courses
- OWASP Web security testing guide
Summary
- How do you defend against web attacks ?
- Defense In Depth
- Input fields and output
- URL parameters & pathname
- Hardcoding sensitive data
- 3rd party libraries & data
- Secure Development Cycle
- Defense In Depth
Thank you

Developer week: Securing Frontend Web App
By Mariam Reba Alexander
Developer week: Securing Frontend Web App
- 184