Node 4
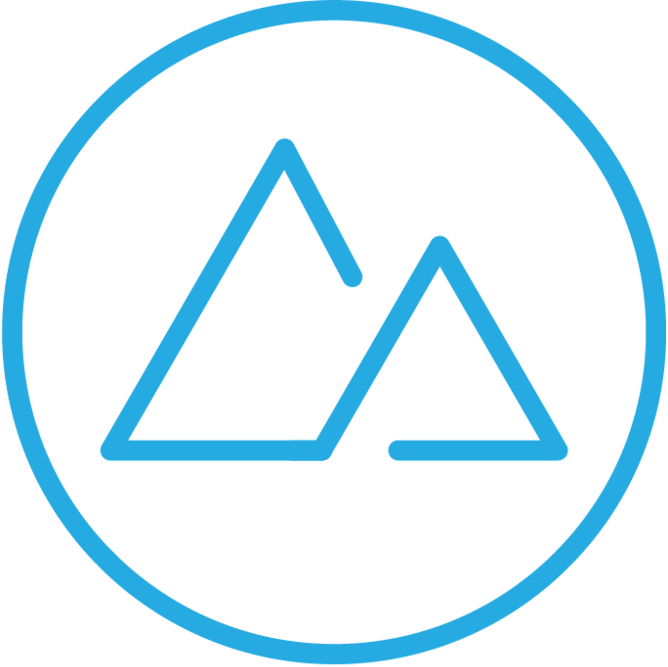
Sessions, Cookies, and Authentication
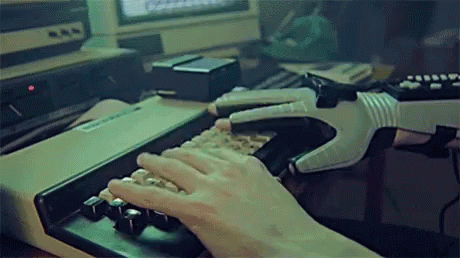
Middleware Review
A middleware function is a function that fires between a request being received by the server and the handler function of an endpoint.
There are two types of middleware:
1. top-level (application level) middleware
2. request-level middleware
Top level middleware fires for every request, or a group of requests.
app.use((req, res, next) => {
console.log('custom top level middleware hit!')
next();
});
Request-level Middleware
Request-level middleware fires before a specific endpoints handler function.
app.get('/api/test', function(req, res, next){
console.log('request level one')
next();
}, function(req, res, next){
console.log('request level two')
next();
}, function(req, res){
console.log('handler function')
res.send('Send a response!');
})
What is a Cookie?
Cookies are small files that are stored by a browser for different webpages.
A common use for cookies is to allow a user to access their profile on a website, without having to login every time they visit the website.
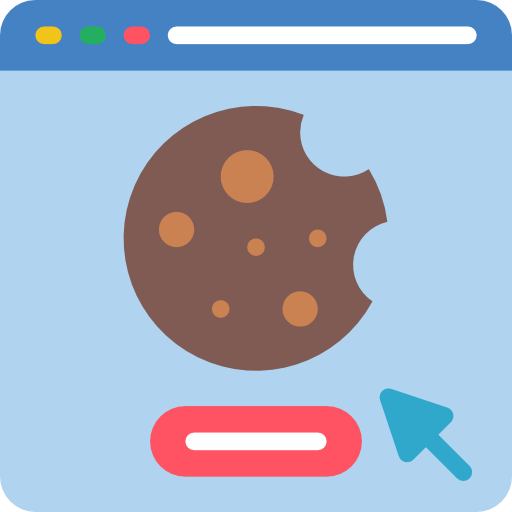
What is a Session?
A session is information keeping track of users cookies and other data.
Sessions are kept on servers, and they correspond to a client's cookies.
Cookies and sessions are NOT the same thing.
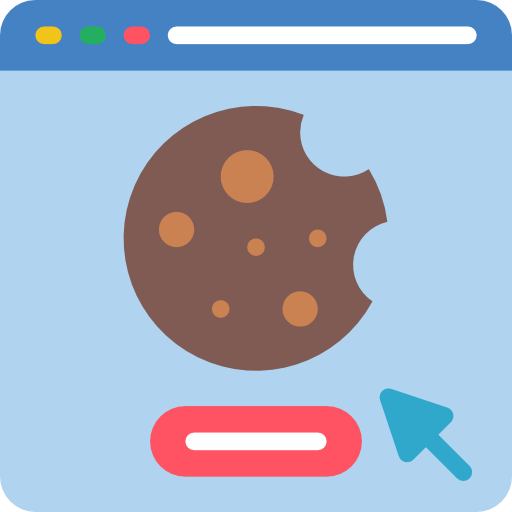
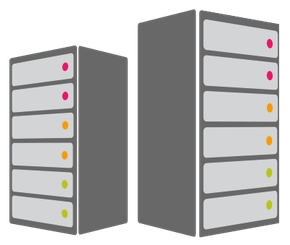
Cookie
Info from Session
Client
Server
Checks if cookies match a session
What is a Session?
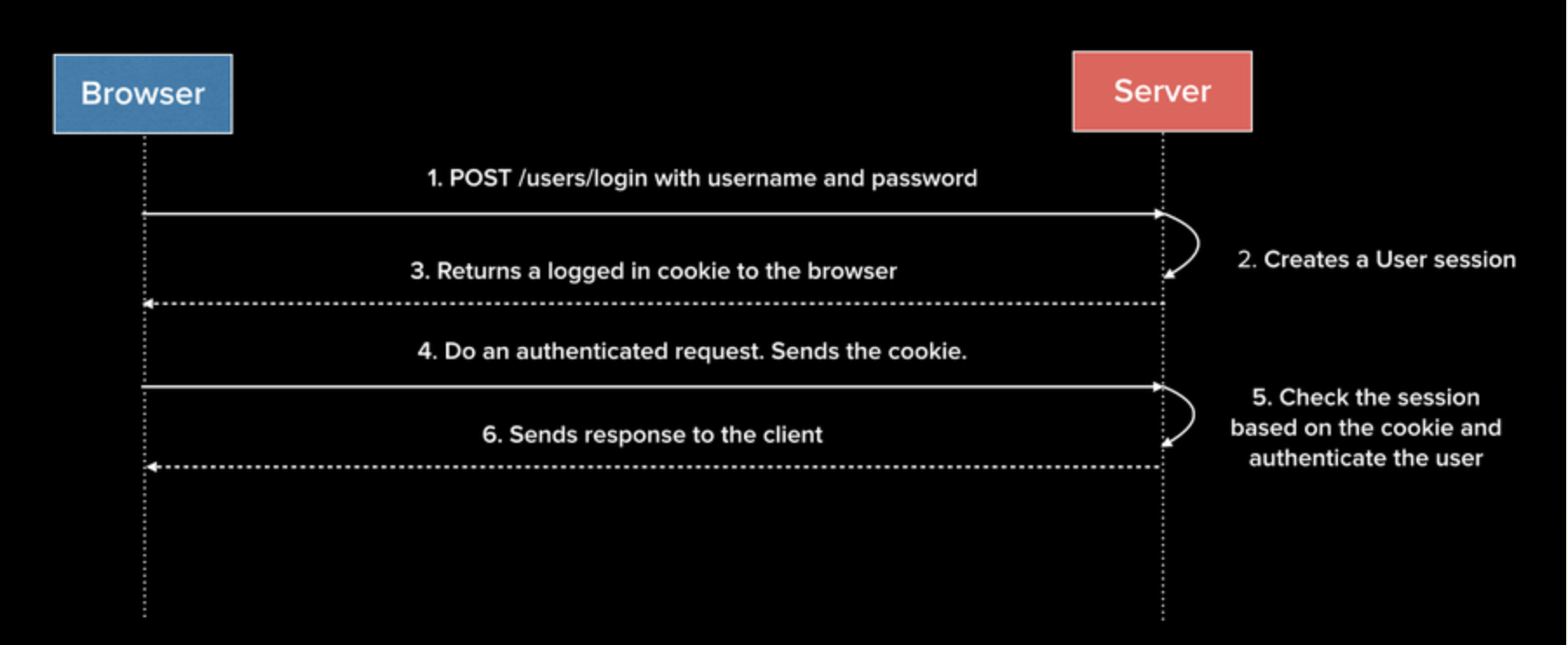
express-session
express-session is a package we can use to create sessions.
It uses middleware to create a session and send back a cookie to the users browser to be stored.
To setup express-session, install it from NPM:
npm install express-session
Then require it to your main server file:
const session = require('express-session')
express-session
Once required, we will use Top-level middleware with express-session:
app.use(session({
resave: false,
saveUninitialized: true,
secret: 'asdfjkl;',
cookie: {maxAge: 1000 * 60}
}));
should active sessions save if no changes made to them?
should a new session save if no data was added?
random string to protect the cookie, typically stored in .env file
object to add settings to the cookie, including the max age of the cookie in milliseconds
Authentication
Authentication is the process of verifying a client is who they say they are.
This is often done through login credentials (username and password, email and password, etc.).
Authentication can be done through different methods such as encoding (HTTP does this) or encryption (HTTPS does this).
Authentication
When working with user passwords, we should follow some simple rules:
Do NOT store plain passwords in a database
Do NOT store encoded passwords in a database
Do NOT store encrypted passwords in a database
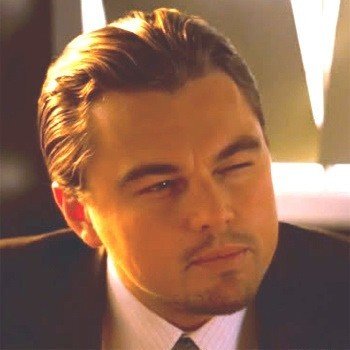
To ensure passwords are stored safely, we need to hash our passwords before storing them in the database.
This is like making a password for your password.
What is Hashing?
Hashing is a process of scrambling a password into a random string of characters, known as a hash.
Hashes are stored to a database in place of the plain-text password.
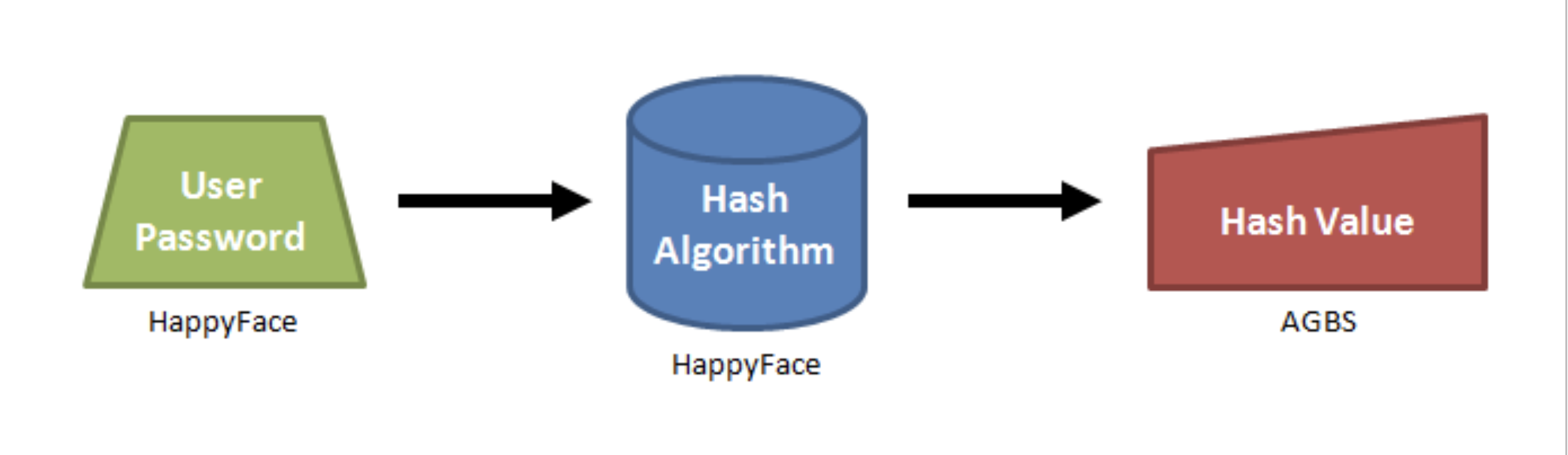
Getting Salty
Salting is adding an extra set of random characters to a hash.
This creates an extra level of security for a users password.
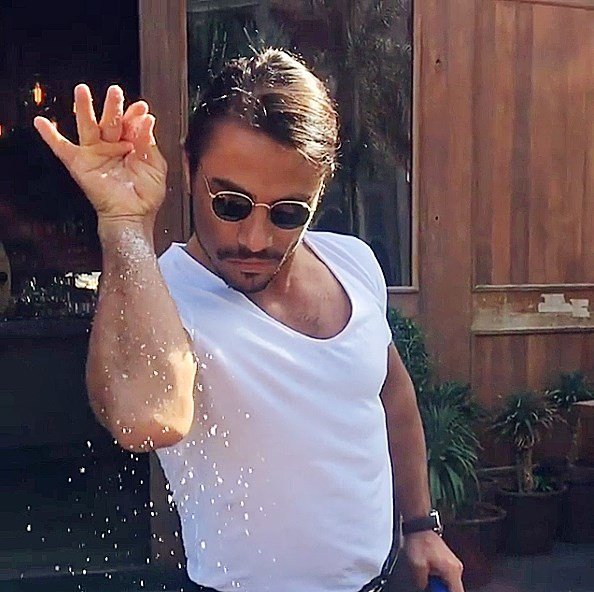
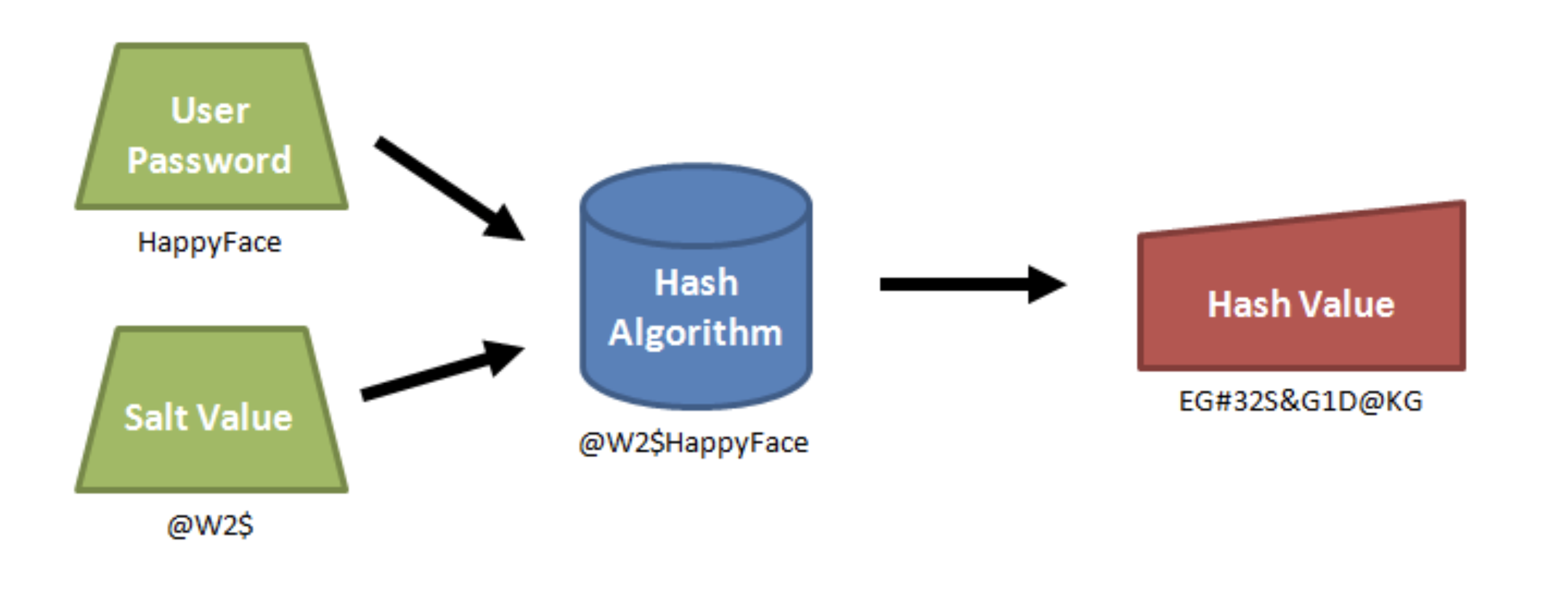
Bcrypt
bcrypt.js is a package that handles hashing and salting passwords.
We can use bcrypt, paired with sessions, to help create authentication handler functions.
To use bcrypt, first install it from NPM:
npm install bcryptjs
Then require it to the top of the controller containing your authentication handler functions:
const bcrypt = require('bcryptjs');
Using Bcrypt
To use hashed passwords, we need the following bcrypt methods:
let password = '12345';
let user = 'Andrew'
let salt = bcrypt.genSaltSync(10);
let hash = bcrypt.hashSync(password, salt)
let hashedPassword = db.get_password([user]);
let authenticated = bcrypt.compareSync(password, hashedPassword);
creates a salt
mixes the password and salt, then creates a hash
compares the password with the hash found in the db
Authentication Endpoints
We will now build authentication endpoints, using bcrypt, and storing authenticated users on sessions.
Example code can be found below:
Node 4
By matias_perez
Node 4
Sessions, Cookies, Authentication
- 404