The reasons
why I like
It is free and open source
- cost
+ contributors
Good documentation
and
lot of good articles
and many others...
Frameworks & Tools
and many, many, many others...just look at Ruby Gems or The Ruby Tool Box
Elegant syntax
AND
Great features
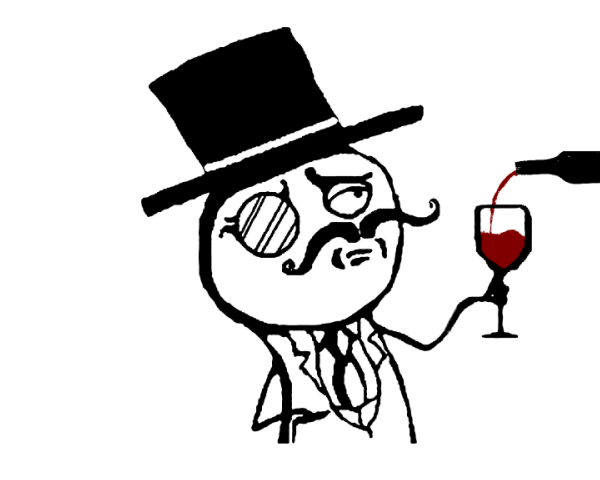
Underscore in numbers
# bad - how many 0s are there?
num = 1000000
# good - much easier to parse for the human brain
num = 1_000_000
String Interpolation
# concatenation ;(
"My name is " + user.name + " and I have " + user.age + " years old."
# interpolation :)
"My name is #{user.name} and I have #{user.age} years old."
# even it works
"You will have #{user.age + 1} years old next year."
Access modifiers
class User
def yell_hello
puts "hello"
end
protected
def say_hello
puts "hello"
end
private
def whisper_hello
puts "hello"
end
end
Symbol
# It's just a name and an internal ID
:open
:closed
puts "hello".object_id
puts "hello".object_id
puts :hello.object_id
puts :hello.object_id
# 70347643412820
# 70347646952580
# 923848
# 923848
Double Pipes or Equals
a = nil
b = 20
c = 30
a ||= b
puts a #=> 20
a ||= c
puts a #=> 20
# daily usage
class User
define initialize(name)
@name ||= name
end
end
Question Marks
"hello".empty? #=> false
" ".empty? #=> false
"".empty? #=> true
Exclamation Points
"hello".delete "l" #=> heo
"hello".delete "x" #=> hello
"hello".delete! "x" #=> nil
Dynamic Typing
(Duck Typing)
# define the type in runtime
x = 100
puts x.class
# type is dinamically changed
x = "hello"
puts x.class
# String
# Fixnum
Strongly Typing
n = "10"
# yeap
puts n + " days"
# nope
puts n + 1
Blocks
# Monkeypatching
class Array
def double!
self.each_with_index do |n, i|
self[i] = yield(n)
end
end
end
array = [1, 2, 3, 4]
array.double! do |n|
n * 2
end
puts array.inspect #=>[2, 4, 6, 8]
Metaprogramming
my_object = Object.new
# define a set method
def my_object.set_my_variable=(var)
@my_instance_variable = var
end
# define a get method
def my_object.get_my_variable
@my_instance_variable
end
my_object.set_my_variable = "Hello"
my_object.get_my_variable # => Hello
Metaprogramming
class Module
def attribute(*attribs)
attribs.each do |a|
define_method(a) { instance_variable_get("@#{a}") }
define_method("#{a}=") { |val| instance_variable_set("@#{a}", val)}
end
end
end
class Person
attribute :name, :email
end
person = Person.new
person.name = "Max"
p person.name
Hash
status = { "open" => "Open", "close" => "Close" }
status = { :open => "Open", :close => "Close" }
status = { open: "Open", close: "Close" }
status = { 0 => "Open", 1 => "Close" }
# used a lot
Person.create(name: "John Doe", age: 27)
def self.create(params)
@name = params[:name]
@age = params[:age]
end
Iterators
# times
100.times do
puts "\o/"
end
# each
users.each do |user|
puts user.name
end
# each_with_index
users.each_with_index do |user, index|
puts "#{index} - #{user.name}"
end
Enumerators
# all?
%w[ant bear cat].all? { |word| word.length >= 3 } #=> true
# any?
%w[ant bear cat].any? { |word| word.length >= 3 } #=> true
# none?
%w{ant bear cat}.none? { |word| word.length == 5 } #=> true
# map
(1..4).map { |i| i*i } #=> [1, 4, 9, 16]
# collect
(1..4).collect { "cat" } #=> ["cat", "cat", "cat", "cat"]
# find_all
(1..10).find_all { |i| i % 3 == 0 } #=> [3, 6, 9]
# select
[1,2,3,4,5].select { |num| num.even? } #=> [2, 4]
# group_by
(1..6).group_by { |i| i%3 } #=> {0=>[3, 6], 1=>[1, 4], 2=>[2, 5]}
# reject
(1..10).reject { |i| i % 3 == 0 } #=> [1, 2, 4, 5, 7, 8, 10]
# sort
%w(rhea kea flea).sort #=> ["flea", "kea", "rhea"]
(scroll down)
Unless statement
# negative if ;(
if !is_true
do_something
end
# unless :)
unless is_true
do_something
end
Single lines
# if
do_something if some_condition
# until
do_something until some_condition
# while
do_something while some_condition
# blocks
names.select { |name| name.start_with?('S') }.map { |name| name.upcase }
Questions?

The Reasons Why I like Ruby
By Max Nunes
The Reasons Why I like Ruby
Some of the best parts of Ruby that I most like
- 3,064