Array grouping gets
easyyy
const itemsInInventory = [
{ name: "potato", type: "vegetables", quantity: 5 },
{ name: "banana", type: "fruit", quantity: 0 },
{ name: "chicken", type: "meat", quantity: 23 },
{ name: "apple", type: "fruit", quantity: 5 },
{ name: "beef", type: "meat", quantity: 22 },
];
// group items by type
const groupedItems = itemsInInventory.reduce((acc, item) => {
if (!acc[item.type]) {
acc[item.type] = [];
}
acc[item.type].push(item);
return acc;
}, {});
// group items in other way
const groupedItems2 = itemsInInventory.reduce((acc, item) => {
return {
...acc,
[item.type]: [
...(acc[item.type] ?? []),
item
],
};
}, {});
{
vegetable: [
{ name: 'potato', type: 'vegetable', quantity: 5 }
],
fruit: [
{ name: 'banana', type: 'fruit', quantity: 0 },
{ name: 'apple', type: 'fruit', quantity: 5 },
],
meat: [
{ name: 'chicken', type: 'meat', quantity: 23 },
{ name: 'beef', type: 'meat', quantity: 22 },
],
}
Result of grouping
Finally, it gets easyyyy
Thank you TC39 :)

const itemsInInventory = [
{ name: "potato", type: "vegetables", quantity: 5 },
{ name: "banana", type: "fruit", quantity: 0 },
{ name: "chicken", type: "meat", quantity: 23 },
{ name: "apple", type: "fruit", quantity: 5 },
{ name: "beef", type: "meat", quantity: 22 },
];
// group items by type
const groupedItems = itemsInInventory.group(item => item.type)
console.log(JSON.stringify(groupedItems,undefined, 4))
đ
Array.prototype.group()


Let's verify in....
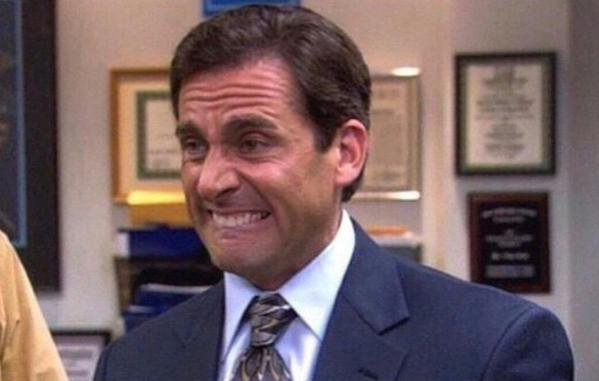




Lodash groupBy
Don't use shorthand

Radash group

Ramda groupBy

core-js polyfill

Make your own?
function groupArray(items, callback) {
return items.reduce((acc, item) => {
const key = callback(item);
if (!acc[key]) {
acc[key] = [];
}
acc[key].push(item);
return acc;
}, {});
}
const groupedItems = groupArray(itemsInInventory, item => item.type);

const itemsInInventory = [
{ name: "potato", type: "vegetables", quantity: 5 },
{ name: "banana", type: "fruit", quantity: 0 },
{ name: "chicken", type: "meat", quantity: 23 },
{ name: "apple", type: "fruit", quantity: 5 },
{ name: "beef", type: "meat", quantity: 22 },
];
// group items by type
const mapOfItems = itemsInInventory.groupToMap(item => item.type)
// Result
Map {
"vegetables" => [
{name: "potato", type: "vegetables", quantity: 5}
],
"fruit" => [
{name: "banana", type: "fruit", quantity: 0},
{name: "apple", type: "fruit", quantity: 5}
],
"meat" => [
{name: "chicken", type: "meat", quantity: 23},
{name: "beef", type: "meat", quantity: 22}
]} (3)
Array.prototype.groupToMap()
const itemsInInventory = [
{ name: "potato", type: "vegetables", quantity: 5 },
{ name: "banana", type: "fruit", quantity: 0 },
{ name: "chicken", type: "meat", quantity: 23 },
{ name: "apple", type: "fruit", quantity: 5 },
{ name: "beef", type: "meat", quantity: 22 },
];
const restock = { restock: true };
const sufficient = { restock: false };
// group items by quantity
const mapOfItems = itemsInInventory.groupToMap(
item => item.quantity < 6 ? restock : sufficient
)
console.log(mapOfItems.get(sufficient));
// Result
[
{name: "chicken", type: "meat", quantity: 23},
{name: "beef", type: "meat", quantity: 22}
]
Array.prototype.groupToMap() - by object
When in V8 engine?
(Chrome, Node.js, Deno)


Code
By MichaĆ Drobniak
Code
- 59