Monads for JavaScript developers
with practical use cases
@MichalZalecki
Let's define a "Monad"!
MONAD
/ˈməʊnad/ or /ˈmɒnad/
In functional programming, monads are a way to build computer programs by joining simple components in robust ways.
‒ wikipedia.org
What is a "MONAD"?
- Design Pattern
- Specification
- Algebraic Structure
- Functor
- Object
Fantasy Land Specification
Specification for interoperability of common algebraic structures in JavaScript
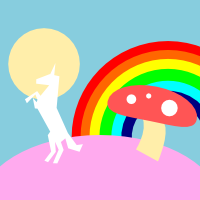
I"M SO PROUD OF MYSELF 💪
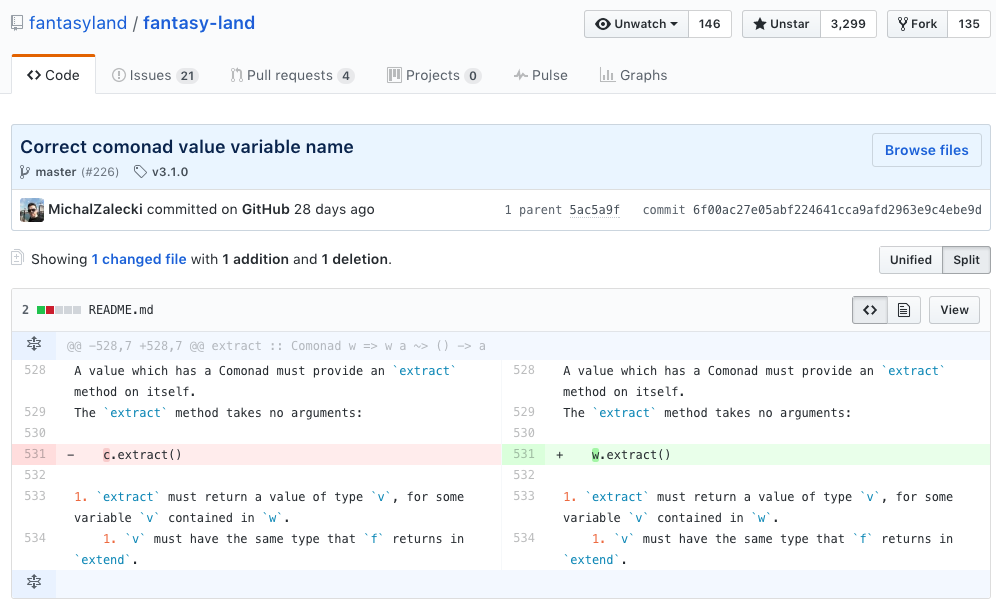
1 commit, 1 character change
Identity
class Identity { static of(value) { return new Identity(value); } constructor(public value) {} chain(fn) { return fn(this.value); }
ap(m) { return m.map(x => x(this.value)); }
map(fn) { return Identity.of(fn(this.value)); } }
Either
class Left { constructor(public value) {} map(_fn) { return this; } } class Right { map(fn) { try { return Right.of(fn(this.value)); } catch (err) { return Left.of(err); } } } type Either = Left | Right;
Maybe
class Nothing { map(_fn) { return this; } } class Just { map(fn) { try { return Just.of(fn(this.value)); } catch (err) { return new Nothing(); } } } type Maybe = Nothing | Just;
IO
class IO { static of(value, ...args) { return new IO(value, ...args); } map(fn) { return IO.of(fn(this.value)); } run() { this.value(...this.args); } equals(m) { return deepEqual(this, m); } }
LIST
function* lazyMap(iterable, fn) { for (let elem of iterable) { yield fn(elem); } }
export class List { map(fn) { return List.of(lazyMap(this.value, fn)); } *[Symbol.iterator]() { yield* this.value; } }
Continuation
Promise.resolve(URL) .then(axios.get) .then(appendPosts) .catch((err) => { ... });
Continuation.of(URL) .chain(axios.get) .chain(appendPosts) .chain(m => if (m instanceof Error) {})
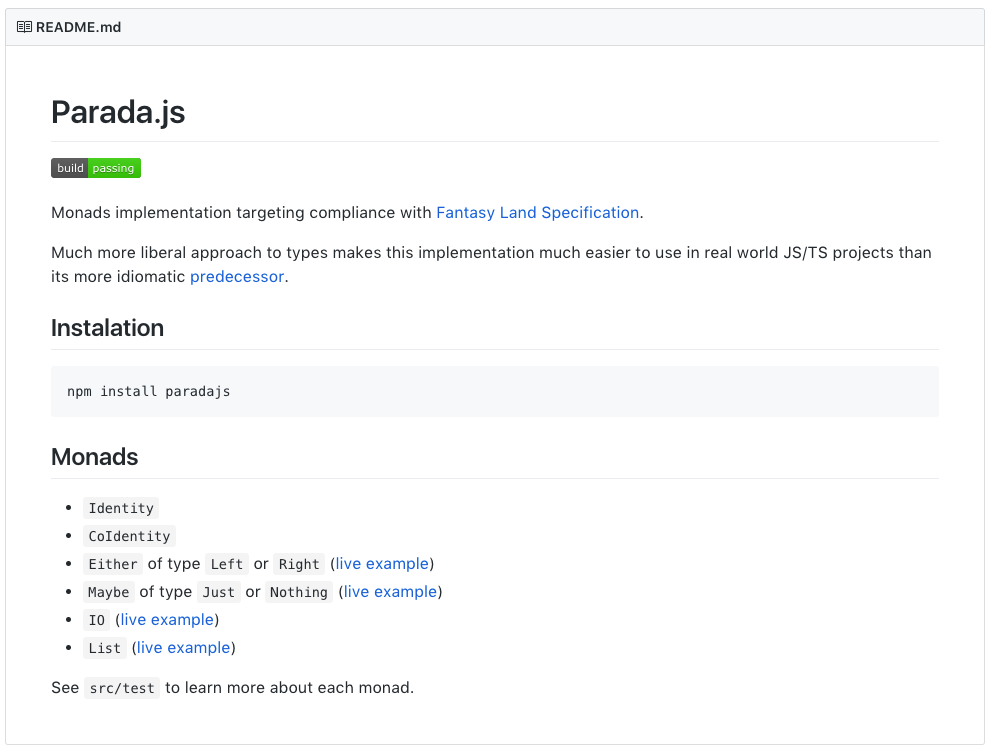
Thank YOu!
Questions?
@MichalZalecki
Monads for JavaScript developers with practical use cases @MichalZalecki
MONADS FOR JAVASCRIPT DEVELOPERS
By Michał Załęcki
MONADS FOR JAVASCRIPT DEVELOPERS
- 1,741