Programming Languages
M. Rocha
ROP Exploring Computer Science
Low level
High level
Programing Language Levels
Script
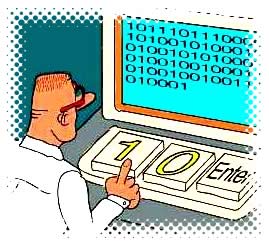

Interpreted vs. Compiled

In most cases the interpreter compiles the source code each time you run it. This is slow relative to the speed of compiled code.
Interpreted vs. Compiled
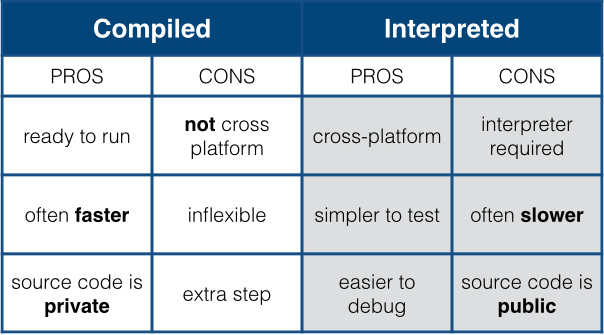
Development times are significantly faster with interpreted programs
Execution times are usually faster for compiled programs
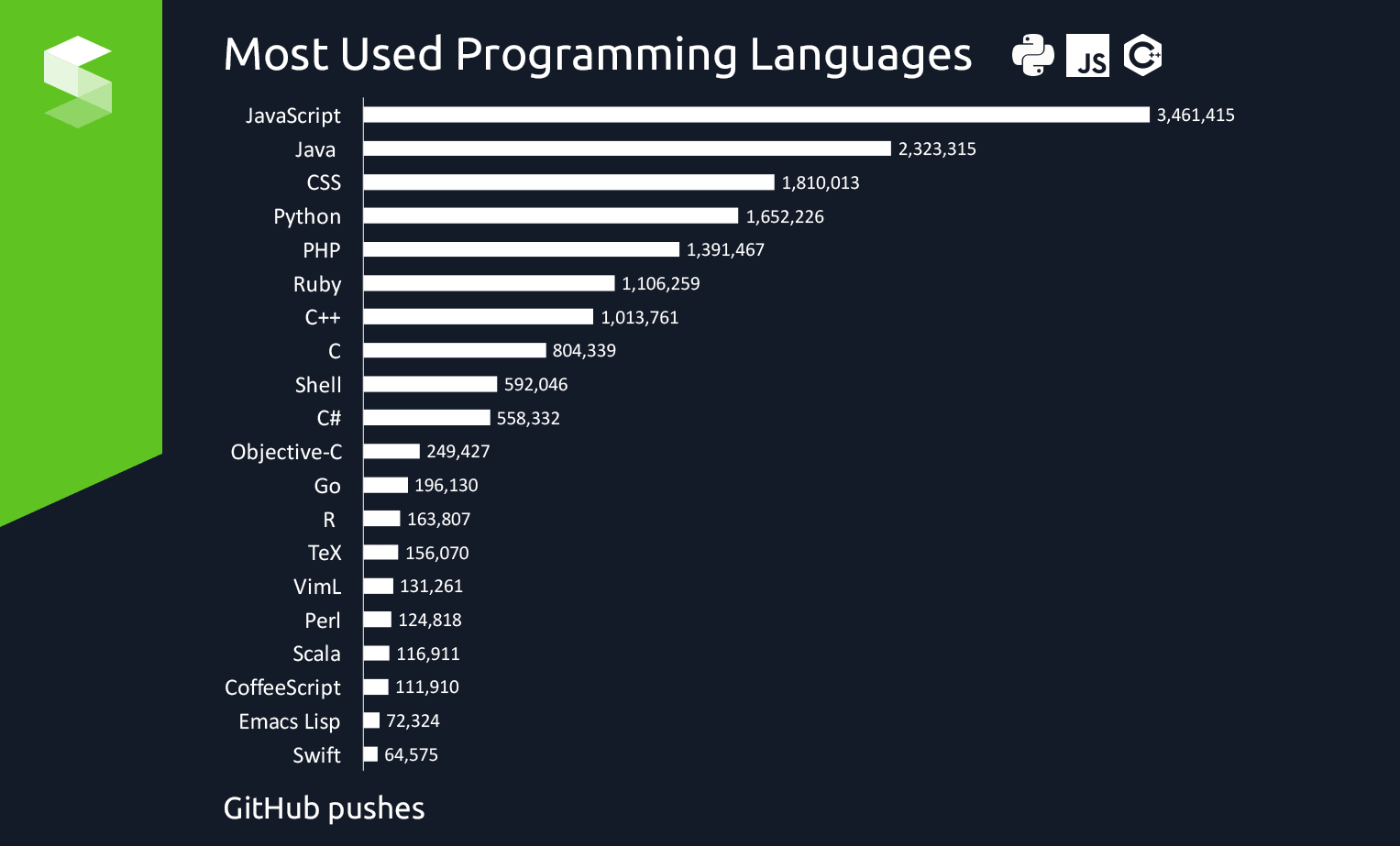
Programming Languages Trends
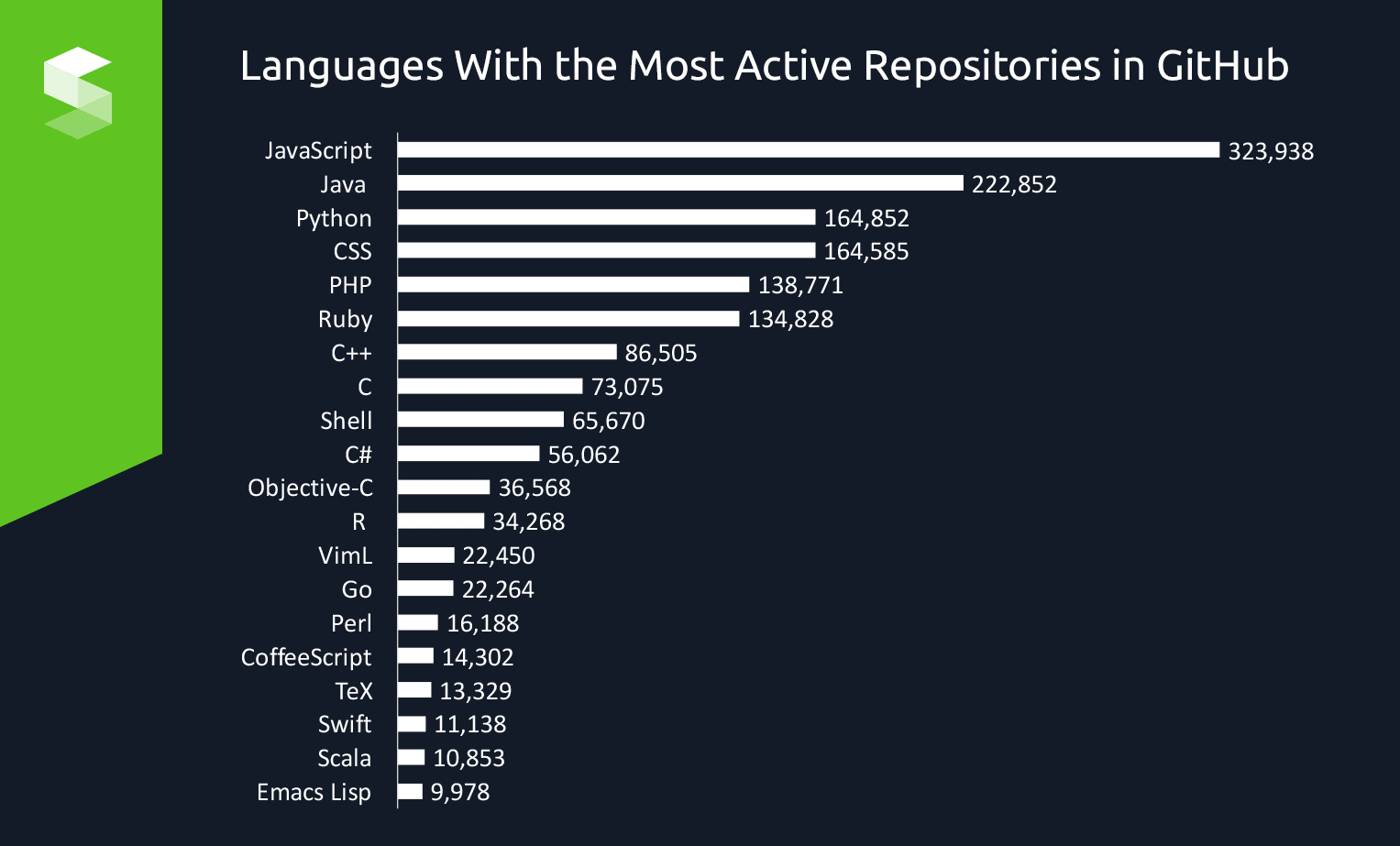
Programming Languages Trends
Programming Languages Trends

Why JavaScript?
-
JavaScript is the only client side scripting language supported by web engines (Browsers and WebViews)
-
Thanks to Google's V8 JavaScript engine, JavaScript is the most efficient (fastest to execute) interpreted/scripting language
JavaScript is not JAVA!!!
Why Python?
-
Python is the easiest/fastest to code general purpose programming language, and you can do anything with it!
-
Python is fast enough
Why C/C++?
If you need high performance or low level (microcontroller programming) C/C++ is the way to go
CUDA/OpenACC - Are extensions to C/C++ that allow you to program computing accelerators such as GPUs and Xeon Phi's
Arduino Language - Is a C/C++ extension that allows you to program arduino microcontrollers
Syntax Comparison
JavaScript (C like)
Python
def main():
guessTheNumber(1, 100);
def guessTheNumber(low, high):
inclusive_range = (1, 100)
print("Guess the number between %i and %i.\n"
% inclusive_range)
target = random.randint(*inclusive_range)
answer, i = None, 0
while answer != target:
i += 1
txt = input("Your guess(%i): " % i)
try:
answer = int(txt)
except ValueError:
print(" I don't understand your input of '%s' ?" % txt)
continue
if answer < inclusive_range[0] or answer > inclusive_range[1]:
print(" Out of range!")
continue
if answer == target:
print(" You got it!")
break
if answer < target: print(" You're too low.")
if answer > target: print(" You're too high.")
print("\nThanks for playing.")
main()
function main() {
guessTheNumber(1, 100);
}
function guessTheNumber(low, high) {
var num = randOnRange(low, high);
function checkGuess(n) {
if (n < low || n > high) {
print('That number is not between ' + low + ' and ' + high + '!');
return false;
}
if (n == num) {
print("You got it!");
return true;
}
if (n < num) {
print("You're too low.");
} else {
print("You're too high.");
}
return false;
}
print('I have picked a number between ' + low + ' and ' + high + '. Try to guess it!');
while (true) {
putstr(" Your guess: ");
var n = parseInt(readline());
if (checkGuess(n)) break;
}
}
function randOnRange(low, high) {
var r = Math.random();
return Math.floor(r * (high - low + 1)) + low;
}
main();
Programming Languages
By Miguel Rocha
Programming Languages
Exploring Computer Science: lecture 5
- 799