Od zera do JavaScript developera #1
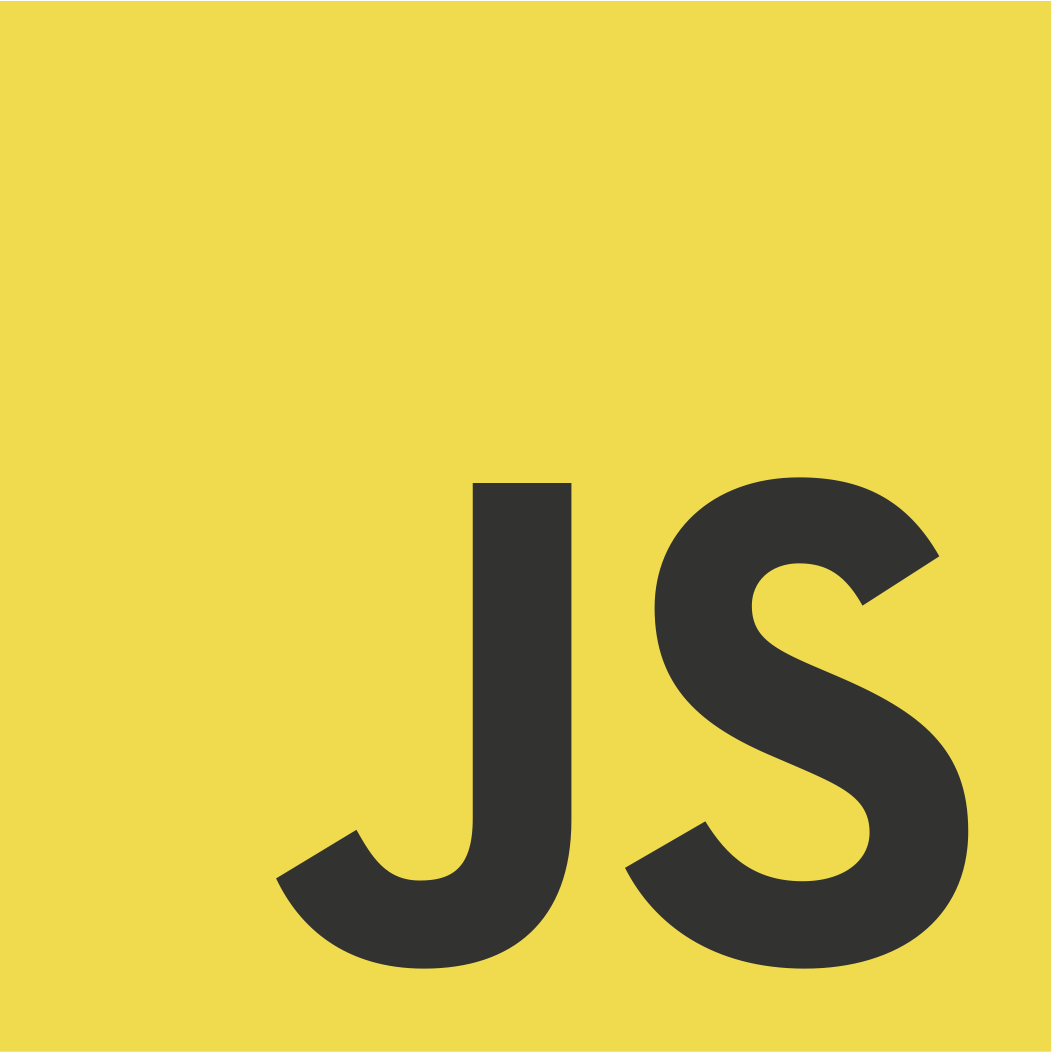

Michal Staskiewicz

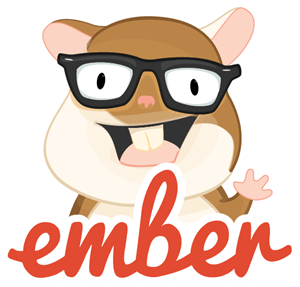
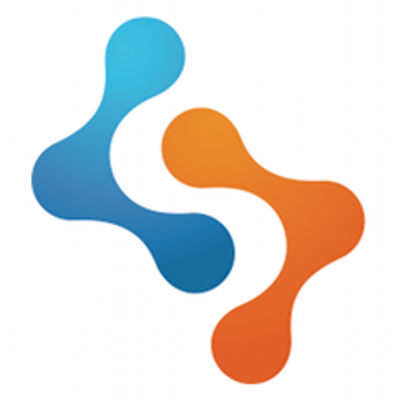



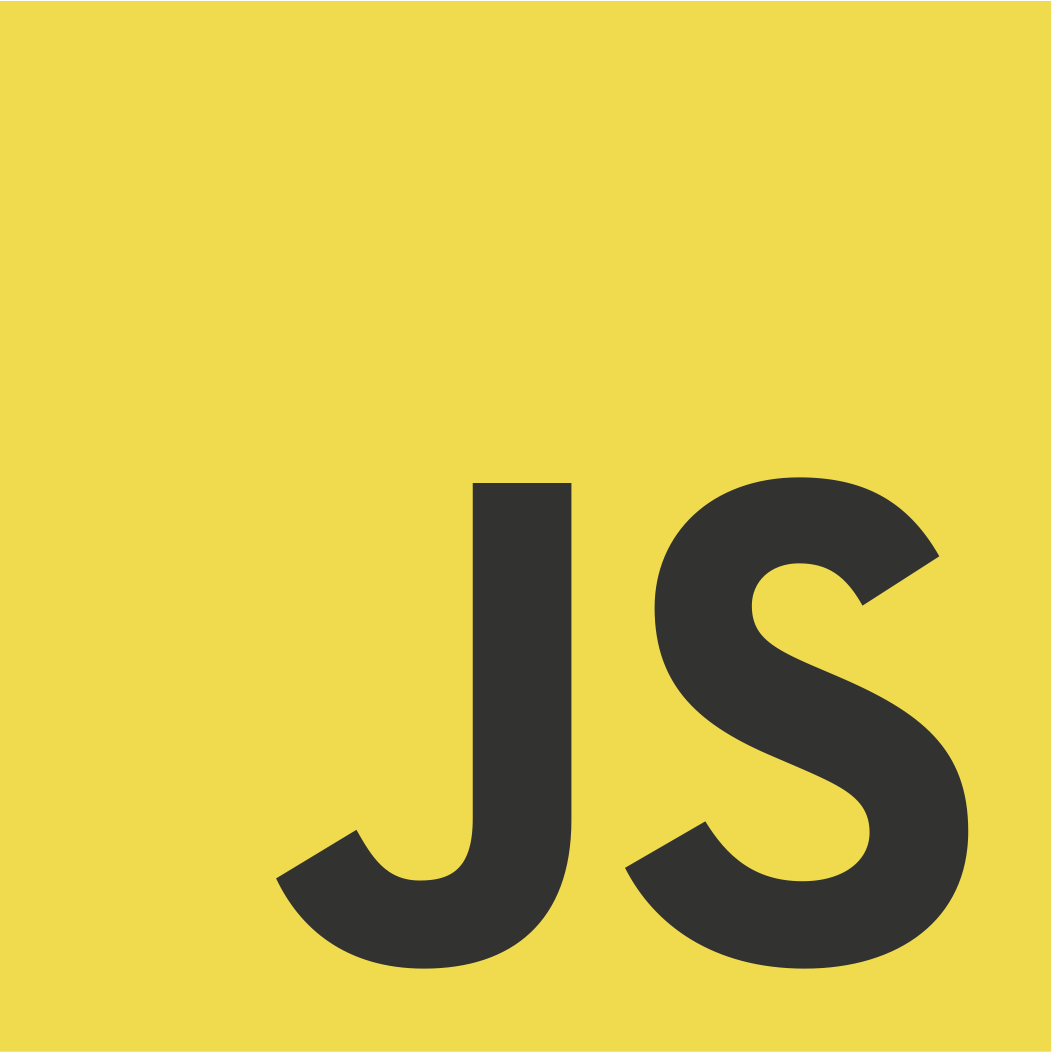

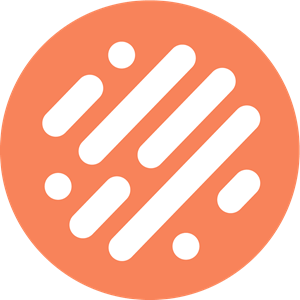


https://miksturait.slack.com/
http://bit.ly/miksturait
What exactly is programming?
Why should we care about JavaScript?
Web Frontend + Backend
IoT
Native mobile apps
Desktop apps
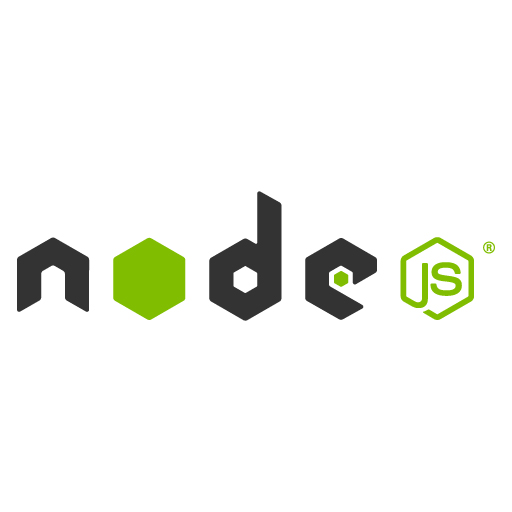
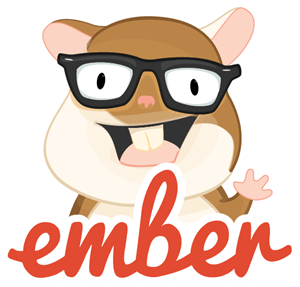
What we will learn on course?
Agenda
- Values, Types, and Operators
- Data Structures - Objects and Arrays
- Higher-order Functions
- Objects & first project
- Bugs, Errors & Regular Expression
- Modules & Asynchronous Programming
- Project - A Programming Language (review)
- Exercises - katas
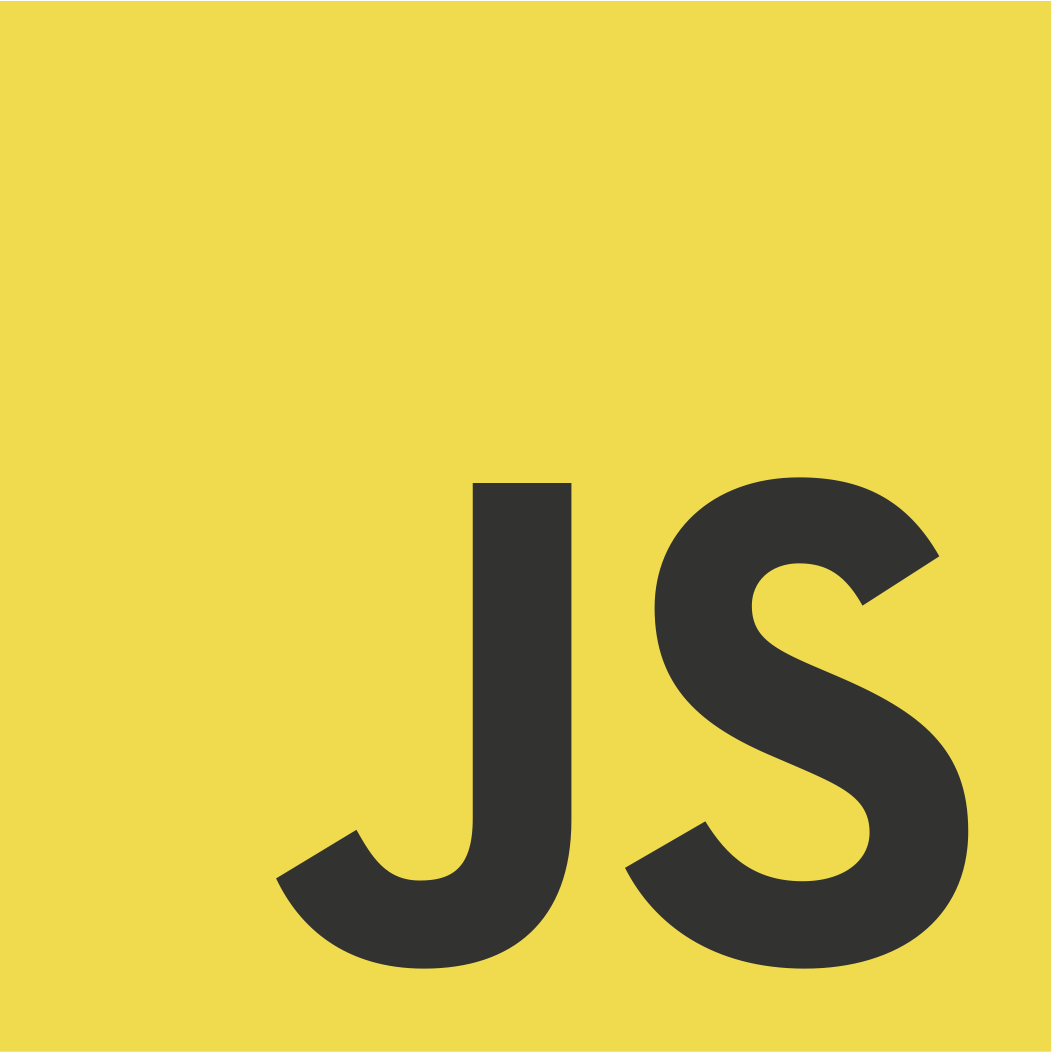
https://eloquentjavascript.net
The Console

Simple data types in JS
Number - 3, 2.1, 3.123e7
String - "hello world"
Boolean - true, false
Undefined
Number
2 - simple number
16.2 - floating-point number
10e1 - scientific notation number == (100)
Arithmetic
+
-
/
*
%
Operators:
10 * 10
100/2
11 % 10
2 + 2
Special Numbers
Infinity
-Infinity
NaN
Strings
`Down on the sea`
"Lie on the ocean"
'Float on the ocean'
" "
` `
' '
Newlines
"This is the first line\nAnd this is the second"
\n
Concatenate
'od' + 'zera' + 'do' + 'javascript' + 'developera' + '#1'
`some sting example with interpolation ${10 * 10}`
typeof operator
typeof "hello"
// "string"
typeof 10
// "number"
typeof {}
// "object"
typeof true
// "boolean"
typeof undefined
// "undefined"
Comparison operators
<
>
<=
>=
==
!=
===
!==
Logical operators
&& - and
|| - or
! - not
:? - ternary operator
Variables
let number = 10;
var number = 10;
const number = 10;
Built in functions
console.log()
console.table()
console.error()
console.warn()
prompt()
alert()
Control Flow
Top to bottom
let theNumber = Number(prompt("Pick a number"));
console.log("Your number is the square root of " +
theNumber * theNumber);
if
else
else if
switch
Conditionals - if
let theNumber = Number(prompt("Pick a number"));
if (!Number.isNaN(theNumber)) {
console.log("Your number is the square root of " +
theNumber * theNumber);
}
let theNumber = Number(prompt("Pick a number"));
if (!Number.isNaN(theNumber)) {
console.log("Your number is the square root of " +
theNumber * theNumber);
} else {
console.log("Hey. Why didn't you give me a number?");
}
else
else if
let num = Number(prompt("Pick a number"));
if (num < 10) {
console.log("Small");
} else if (num < 100) {
console.log("Medium");
} else {
console.log("Large");
}
nested else if
if (x == "value1") action1();
else if (x == "value2") action2();
else if (x == "value3") action3();
else defaultAction();
Switch
switch (prompt("What is the weather like?")) {
case "rainy":
console.log("Remember to bring an umbrella.");
break;
case "sunny":
console.log("Dress lightly.");
case "cloudy":
console.log("Go outside.");
break;
default:
console.log("Unknown weather type!");
break;
}
Loops
while
let number = 0;
while (number <= 12) {
console.log(number);
number = number + 2;
}
// → 0
// → 2
// … etcetera
do while
let yourName;
do {
yourName = prompt("Who are you?");
} while (!yourName);
console.log(yourName);
for
let result = 1;
for (let counter = 0; counter < 10; counter = counter + 1) {
result = result * 2;
}
console.log(result);
for with break
for (let current = 20; ; current = current + 1) {
if (current % 7 == 0) {
console.log(current);
break;
}
}
// → 21
shorthand syntax
value = value + 1
value++
Exercises
Homework
Thanks! =>
Od zera do JavaScript developera #1
By Michał Staśkiewicz
Od zera do JavaScript developera #1
- 1,084