Od zera do JavaScript developera #2
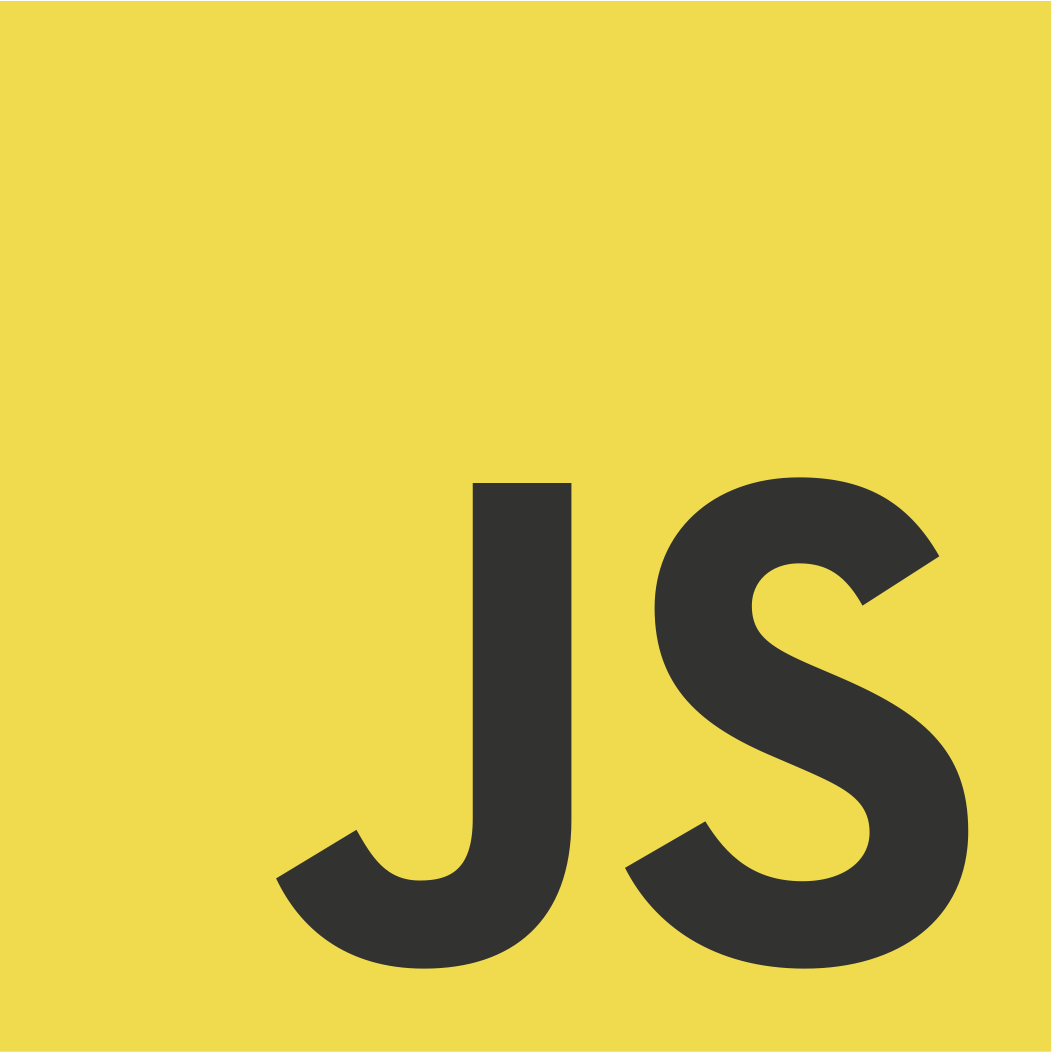

Michal Staskiewicz

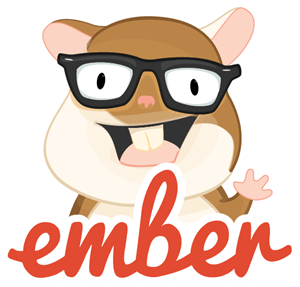
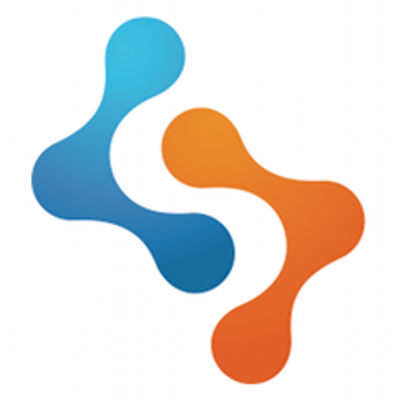



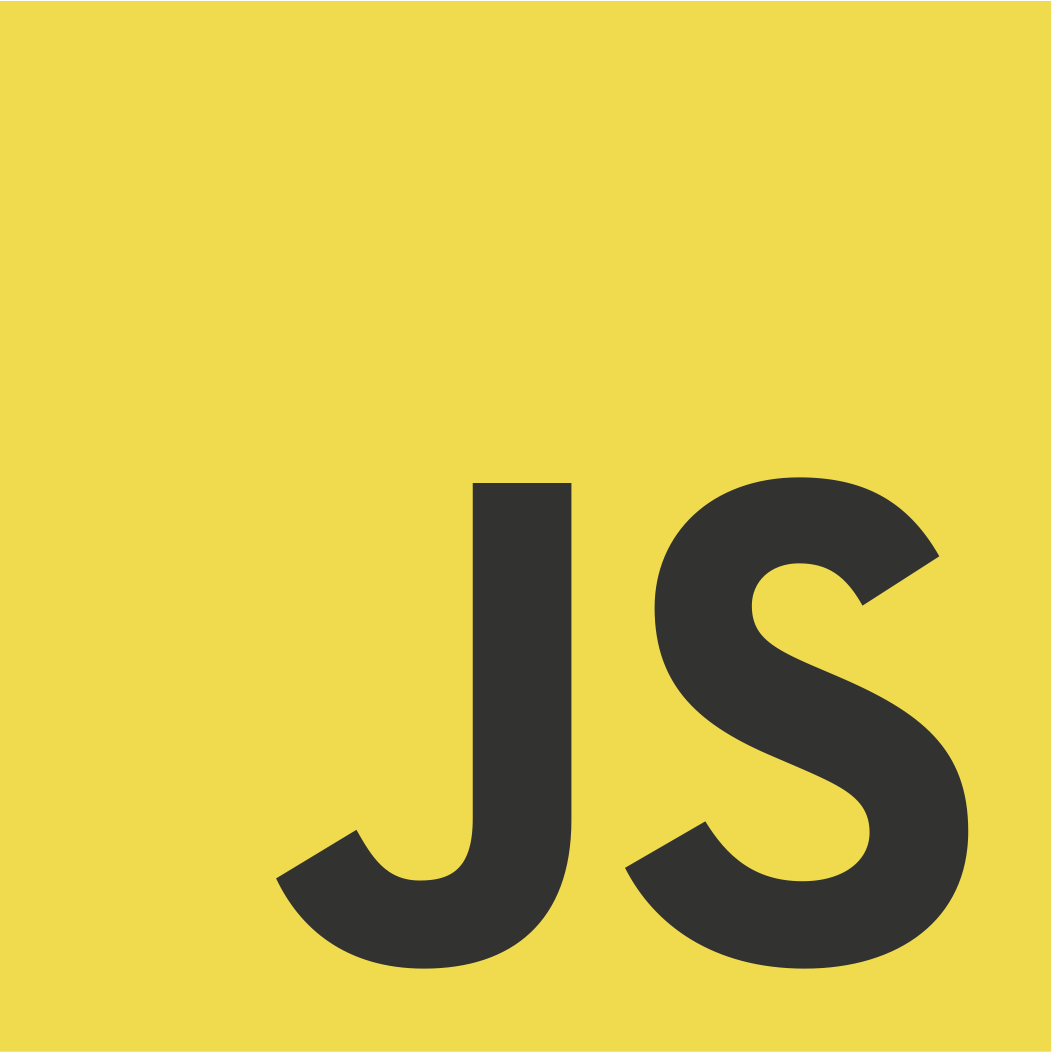

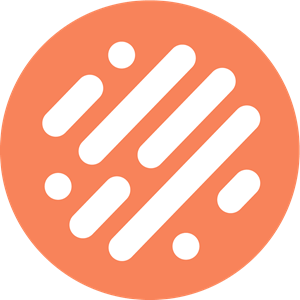


https://miksturait.slack.com/
http://bit.ly/miksturait
Agenda
- Values, Types, and Operators
- Functions &Data Structures - Objects and Arrays
- Higher-order Functions
- Objects & first project
- Bugs, Errors & Regular Expression
- Modules & Asynchronous Programming
- Project - A Programming Language (review)
- Exercises - katas
Exercises review
#1 Prime numbers
#2 Triangle area
#3 Print Triangle
Deep dive into functions!
const square = function(x) {
return x * x;
};
Params
Name
Output
const power = function(base, exponent) {
let result = 1;
for (let count = 0; count < exponent; count++) {
result *= base;
}
return result;
};
let x = 10;
if (true) {
let y = 20;
var z = 30;
console.log(x + y + z);
// → 60
}
// y is not visible here
console.log(x + z);
// → 40
Scopes
const halve = function(n) {
return n / 2;
};
let n = 10;
console.log(halve(100));
// → 50
console.log(n);
// → 10
function square(x) {
return x * x;
}
const square1 = (x) => { return x * x; };
const square2 = x => x * x;
Alternative Syntax
Call Stack
function greet(who) {
console.log("Hello " + who);
}
greet("Harry");
console.log("Bye");
Call stack:
not in function
in greet
in console.log
in greet
not in function
in console.log
not in function
Exercices
Write a function that adds two numbers
Write a function that subtract two numbers
Write a function that multiply two numbers
Write a function that divide two numbers
Write a function that calculate the power with given base and exponent
recursion(recursion(recursion))
function power(base, exponent) {
if (exponent == 0) {
return 1;
} else {
return base * power(base, exponent - 1);
}
}
power(2, 3)
power(2, 3)
power(2, 2)
power(2, 1)
power(2, 0) -> stop(if === 0)
power(2, 0) -> 1
power(2, 1) -> 2
power(2, 2) -> 4
power(2, 3) -> 8
Hard Exercise?
Write triangle printer(ex. 3 from the beginning) without using loops - instead use recursion
Easy Exercise?
Arrays
let listOfNumbers = [2, 3, 5, 7, 11];
Accessing data
let listOfNumbers = [2, 3, 5, 7, 11];
console.log(listOfNumbers[2]);
// → 5
console.log(listOfNumbers[0]);
// → 2
console.log(listOfNumbers[2 - 1]);
// → 3
typeof array //?
Objects!
Properties
const apple = {
color: 'red',
shape: 'round'
}
let doh = "Doh";
console.log(typeof doh.toUpperCase);
// → function
console.log(doh.toUpperCase());
// → DOH
Methods
Array methods
.pop()
.push()
.shift()
.unshift()
Exercise - TODO list
addTask('task')
nextTask()
addUrgentTask('task')
['first task', 'second task', 'third task']
['first task', 'second task', 'third task']
['first task', 'second task', 'third task']
Creating own methods
let myObject = {
todos: [],
showTodoList() {
console.log(this.todos);
}
}
'this'
Todo List improvements - transform TODO list into object
Exercies
Sum of range
Reversing Array
Beer Challange
Środa - 27.06.2018 do 18:00
Thanks! =>
Od zera do JavaScript developera #2
By Michał Staśkiewicz
Od zera do JavaScript developera #2
- 885