Od zera do JavaScript developera #3
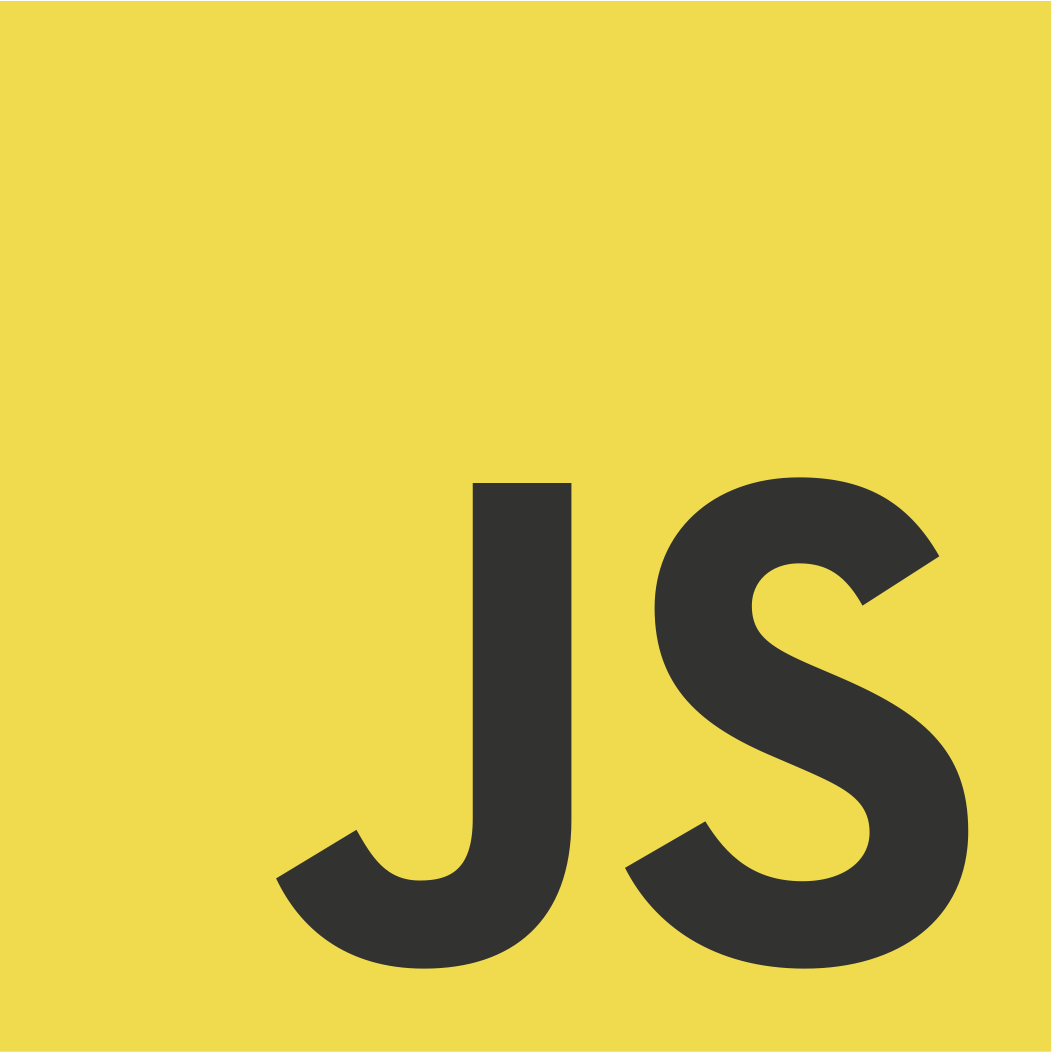

Michal Staskiewicz

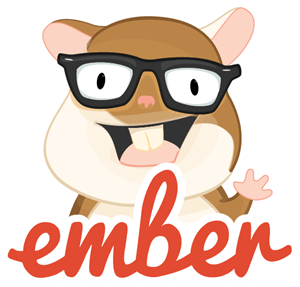
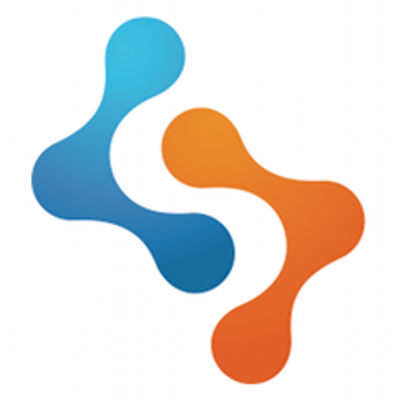



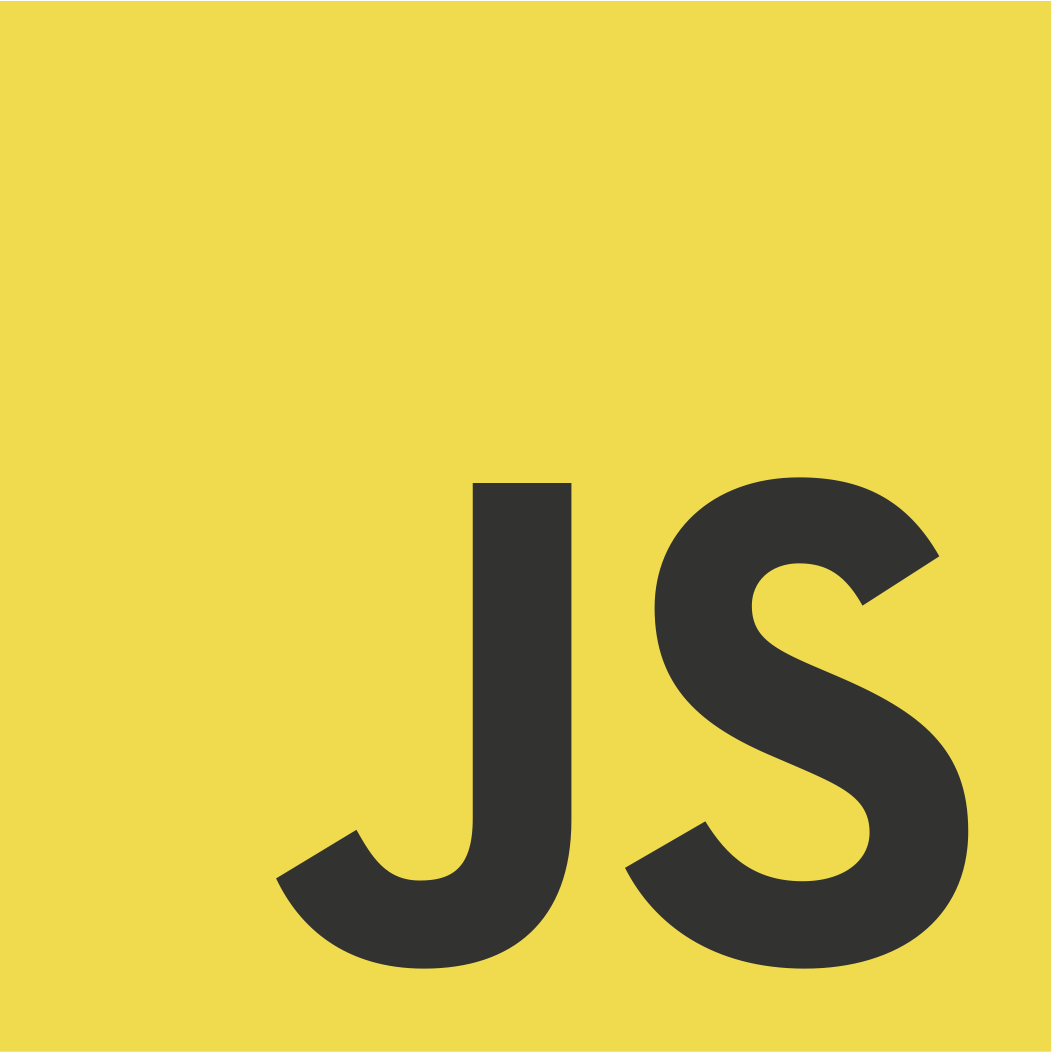

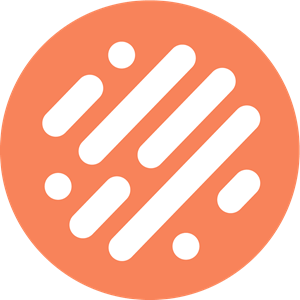


https://miksturait.slack.com/
http://bit.ly/miksturait
Agenda
- Values, Types, and Operators
- Functions &Data Structures - Objects and Arrays
- Higher-order Functions
- Objects & first project
- Bugs, Errors & Regular Expression
- Modules & Asynchronous Programming
- Project - A Programming Language (review)
- Exercises - katas
Beer Challenge Review
@Adrian
@kajetanboruta
@Paulina Wróbel
@Malwina
@Szymon
#1 ArrayToList & more
#2 deepEqual
Bug - what it is?
Less complexity === less bugs
let total = 0, count = 1;
while (count <= 10) {
total += count;
count += 1;
}
console.log(total);
console.log(sum(range(1, 10)));
VS
Abstractions
Put 1 cup of dried peas per person into a container. Add water until the peas are well covered. Leave the peas in water for at least 12 hours. Take the peas out of the water and put them in a cooking pan. Add 4 cups of water per person. Cover the pan and keep the peas simmering for two hours. Take half an onion per person. Cut it into pieces with a knife. Add it to the peas. Take a stalk of celery per person. Cut it into pieces with a knife. Add it to the peas. Take a carrot per person. Cut it into pieces. With a knife! Add it to the peas. Cook for 10 more minutes.
Per person: 1 cup dried split peas, half a chopped onion, a stalk of celery, and a carrot.
Soak peas for 12 hours. Simmer for 2 hours in 4 cups of water (per person). Chop and add vegetables. Cook for 10 more minutes.
Abstracting repetition
for (let i = 0; i < 10; i++) {
console.log(i);
}
function repeatLog(n) {
for (let i = 0; i < n; i++) {
console.log(i);
}
}
Pass action down!
function repeat(n, action) {
for (let i = 0; i < n; i++) {
action(i);
}
}
repeat(3, console.log);
// → 0
// → 1
// → 2
Define own function
let labels = [];
repeat(5, i => {
labels.push(`Unit ${i + 1}`);
});
console.log(labels);
// → ["Unit 1", "Unit 2", "Unit 3", "Unit 4", "Unit 5"]
Higher-order functions
Functions that manipulates or returns other function
Examples
function greaterThan(n) {
return m => m > n;
}
let greaterThan10 = greaterThan(10);
console.log(greaterThan10(11));
// → true
function noisy(f) {
return (...args) => {
console.log("calling with", args);
let result = f(...args);
console.log("called with", args, ", returned", result);
return result;
};
}
noisy(Math.min)(3, 2, 1);
// → calling with [3, 2, 1]
// → called with [3, 2, 1] , returned 1
function unless(test, then) {
if (!test) then();
}
repeat(3, n => {
unless(n % 2 == 1, () => {
console.log(n, "is even");
});
});
// → 0 is even
// → 2 is even
Data processing with High Order Function
Filtering arrays
Write Function That will filter array
interface: filter(array, test)
function filter(array, test) {
let passed = [];
for (let element of array) {
if (test(element)) {
passed.push(element);
}
}
return passed;
}
Exercise
We have an array with 1000 numbers;
Filter it and display only number that can be divided by 3
let array = [...Array(1000)].map((_e, i) => i)
The map method
Takes array and apply function to each element and then return that array
interface: map(array, transform)
function map(array, transform) {
let mapped = [];
for (let element of array) {
mapped.push(transform(element));
}
return mapped;
}
Exercise
We have an array with 1000 numbers;
Multiply every element in this array by two;
let array = [...Array(1000)].map((_e, i) => i)
Reduce
produce singe value from an array
interface: reduce(array, combine, start)
function reduce(array, combine, start) {
let current = start;
for (let element of array) {
current = combine(current, element);
}
return current;
}
Exercise
We have an array with 1000 numbers;
Sum all elements in array
let array = [...Array(1000)].map((_e, i) => i)
Array's built in methods
.forEach
.map
.reduce
.filter

Code Sandbox
Sandbox url
Emojis
// Two emoji characters, horse and shoe
let horseShoe = "🐴👟";
console.log(horseShoe.length);
// → 4
console.log(horseShoe[0]);
// → (Invalid half-character)
console.log(horseShoe.charCodeAt(0));
// → 55357 (Code of the half-character)
console.log(horseShoe.codePointAt(0));
// → 128052 (Actual code for horse emoji)
Exercies
#1 Count chars in every script
Dataset is available under SCRIPTS constant in sandbox
#2 Average creation year for living and dead scripts
Dataset is available under SCRIPTS constant in sandbox
#3 Flattening
#4 Your own loop
#5 Everything
Snack Challenge
Dominant writing direction
Deadline - Środa 4.07 18:00
Thanks! =>
Od zera do JavaScript developera #3
By Michał Staśkiewicz
Od zera do JavaScript developera #3
- 823