Streams
Probably the hardest concept in NodeJS
Streams are simple collections of data, the only difference is, they are not available at once
Let's try it out
Streams can be ...
- Readable
- Writeable
- Duplex (Both Readable & Writeable)
- Transform
Readable
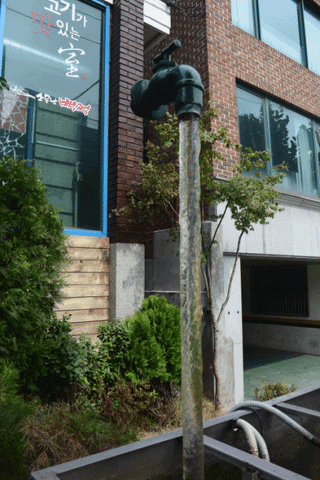
- Its the source
- Flowing data like water from faucet
- Is able to sink into any other container (writeable/duplex/transform)
Writeable
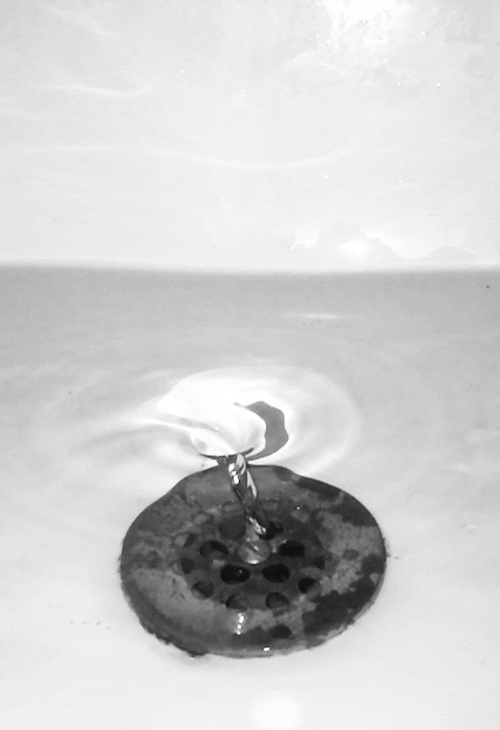
- Its the sink/drain
- Receives data
- Is able to sink data from any other container (readable/duplex/transform)
Duplex
- Imagine a server which receives as well as sends data
- Can do both read and write

Transform
- Transforms a readable stream and makes it available to write
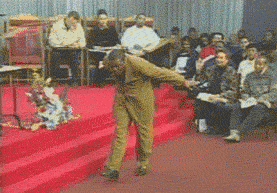
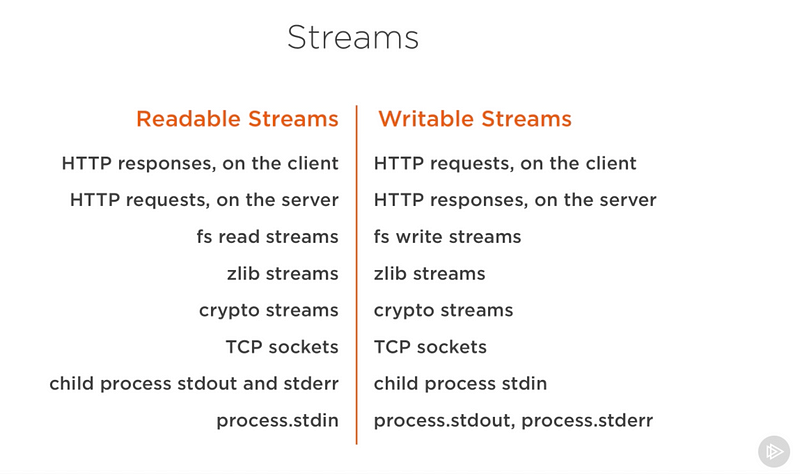
Streams implement the EventEmitter interface
Readable
- data - stream will keep on receiving this event as more data is available to read
- end - stream will receive this event once no more data is available to read
Writeable
- drain - stream will keep on receiving this event indicating it can receive more data
- finish - stream will receive this event once all data has been written/flushed
Implements
Event Emitter


Readable Stream
//since the process.stdin is a stream
//and a stream implements
//event emitter class,
//we are able to listen
//to its event when data
//is coming in stdin
process.stdin.on('data', handler)
const handler = (data) => {
console.log(data)
}

<Buffer 64 73 66 73 64 66 0a>
Buffers
All streams created by Node.js APIs operate exclusively on strings and Buffer (or Uint8Array) objects.
//set encoding
process.stdin.setEncoding('utf8')
//print data on standard input
process.stdin.on('data', console.log)
Lets read from a file

// lets read a file
const fs = require("fs")
const rs = fs.createReadStream("./003.js", "utf8");
rs.on("data", (chunk) => {
console.log(chunk);
});
rs.on("end", () => {
console.log("done reading from stream..");
});

end
The 'end' event is emitted when there is no more data to be consumed from the stream.
close
The 'close' event is emitted when the stream and any of its underlying resources (a file descriptor, for example) have been closed. The event indicates that no more events will be emitted, and no further computation will occur.

Writable Stream
const { Writable } = require('stream');
const ws = new Writable({
write(chunk) {
process.stdout.write(chunk)
}
})
process.stdin.pipe(ws)
uncork()
cork()
const fs = require("fs");
const { Writable } = require('stream');
const ws = new Writable({
write(c) {
process.stdout.write(c)
}
})
ws.cork()
ws.write('hello!')
ws.write('hello agian!')
setImmediate(() => {
console.log('uncorking...')
ws.uncork();
})
const { Writable } = require('stream');
const ws = new Writable({
write(chunk, _, cb) {
process.stdout.write(chunk)
setTimeout(() => {
cb();
}, 10)
}
})
let isDrained = true;
ws.on('drain', () => {
console.log('Drain event!')
})
setInterval(() => {
// console.log('is writable?', ws.writable)
if(isDrained) {
isDrained = ws.write("Hello!", () => {
// console.log("written!")
})
console.log(isDrained)
}
}, 1)
Streams
By Minhaj Khan
Streams
- 128