C++ Basics
Muhammad Magdi
Hello World
#include <iostream> using namespace std; int main() { cout << "Hello World!" << endl; return 0; }
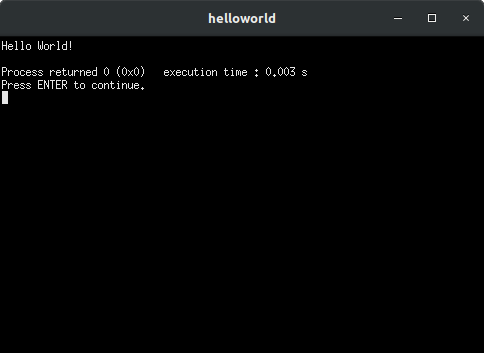
cout
Used to print something to the user screen.
cout << "Hello World!" << endl;
'endl' is used to leave a blank line.
Both:
- #include <iostream>
- using namespace std;
are essentials to be able to use the cout.
#include <iostream> using namespace std;
Notes:
- iostream is called a library.
- Libraries are pre-written codes.
- #include <libraryName> adds this library to our code.
Arithmetic Operators
Operator | Meaning |
---|---|
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Integer Division |
% | Remainder after Division (Mod) |
Operator Precedence
Note that the precedence of the multiplication is higher than that of the subtraction
#include <iostream> using namespace std; int main() { cout << 500 - 100 * 4 << endl; return 0; }
The Precedence of *, /, % is higher than that of +, -
Variable Declaration
#include <iostream> using namespace std; int main() { int x = 10; cout << x << endl; return 0; }
Declaring Variables
Reserves 4 bytes of memory to store integer values, calling these 4 bytes 'x' and giving 'x' an initial value = 10
int x = 10;
If we declared a variable without giving it an initial value, the compiler gives it a random initial value.
Taking Input
#include <iostream> using namespace std; int main() { int x = 0; cin >> x; cout << x*x << endl; }
cin
Is used to take an input value and store it in a variable.
cin >> x;
We can take more than one input value using only one 'cin':
cin >> x >> y >> z;
Data Types
Data Type | Description |
---|---|
int | Small integer number |
double |
Large real number |
long long | Large integer number |
string | A sequence of characters (word) |
char | A single character |
bool | Boolean number (0 or 1) |
Thanks
Content
- Hello World! Program
- Printing Output
- Arithmetic Operations
- Int Datatype
- Declaring Variables
- Taking Input
- More Data Types
C++ Basics Muhammad Magdi
C++ Basics
By Muhammad Magdi
C++ Basics
- 491