Data Types in C++
Muhammad Magdi
Data Types
Data Type | Description |
---|---|
int | Small integer number |
double |
Large real number |
long long | Large integer number |
string | A sequence of characters (word) |
char | A single character |
bool | Boolean number (0 or 1) |
URI 1008 - Salary
[Problem Summary]
- Given:
- integer number representing employee's number.
- integer number representing the number of hours he worked.
- real number representing the amount of money he takes per hour.
- Print a report containing his number and the total money he should take rounded to exactly 2 decimal places.
URI 1008 - Salary
[Solution]
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
int id, hours;
double rate;
cin >> id >> hours >> rate;
cout << fixed << setprecision(2);
cout << "NUMBER = " << id << endl;
cout << "SALARY = U$ " << hours * rate << endl;
return 0;
}
URI 1008 - Salary
[Notes]
- In order to tell "cout" that we need only 2 places after the decimal point, we used "setprecision(2)".
- "setprecision()" is in the "iomanip" library so we had to #include it.
- the line:
"cout << fixed << setprecision(2);" doesn't print anything, it only tells "cout" how it should work with real numbers.
URI 1002 - Area of a Circle
[Problem Summary]
- Given:
- A real number representing
the radius of a circle.
- A real number representing
- Print:
- The message "A=" followed by
the area of that circle with 4 places
after the decimal point.
- The message "A=" followed by
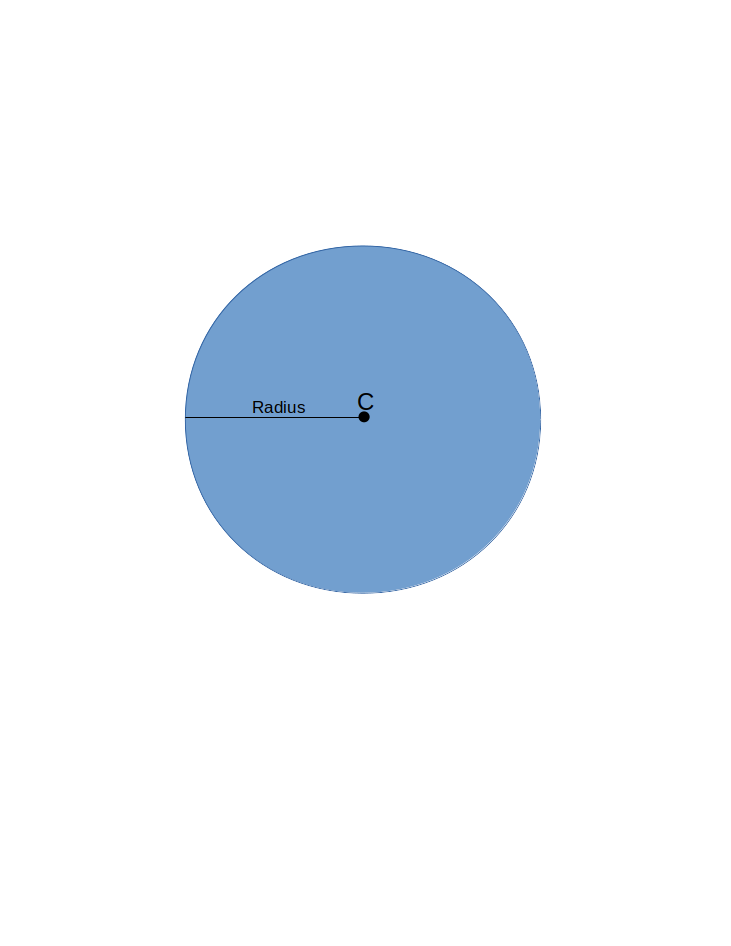
Area=π∗Radius2
Area = π*Radius^2
URI 1002 - Area of a Circle
[Solution]
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
double PI = 3.14159;
double radius;
cin >> radius;
cout << fixed << setprecision(4);
cout << "A=" << PI * radius * radius << endl;
return 0;
}
Assignment
Thanks
Content
- Data Types
- URI 1008 - Salary
- setprecision
- URI 1002 - Area of a Circle
- Assignment
Data Types
By Muhammad Magdi
Data Types
- 288