IF Conditions
Muhammad Magdi
Triangle Type
You know that there are 3 types of triangles according to their sides.
You are given 3 integer numbers
(a, b, c) representing the lengths of the sides of a triangle.
Print the type of this triangle according to its sides.
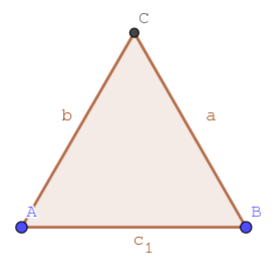
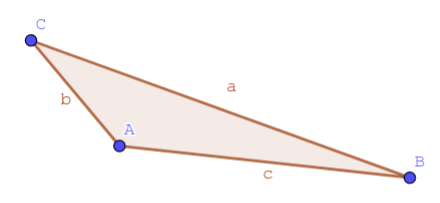
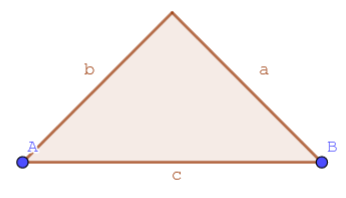
Equilateral
Isosceles
Scalene
If Statements
if ( CONDITION ) {
TRUE-STATEMENTS
}
When the CONDITION is true, the TRUE-STATEMENTS inside the if-body are executed.
Relational Operators
Operator | Meaning |
---|---|
== | equal to |
!= |
not equal to |
< | less than |
> | greater than |
<= | less than or equal |
>= | greater that or equal |
Example
#include <iostream>
using namespace std;
int main() {
int num;
cin >> num;
if (num == 100) {
cout << "It's a Hundred!" << endl;
}
cout << "Bye" << endl;
return 0;
}
Notes
- We used the '==' operator not the '=' operator.
- This program prints the message "It's a Hundred!", Only when the input number is equal to 100.
- This program prints the message "Bye", regardless the value of the input.
When the CONDITION is true, the TRUE-STATEMENTS inside the if-body are executed, otherwise the FALSE-STATEMENTS inside the else-body are executed.
if ( CONDITION ) {
TRUE-STATEMENTS
} else {
FALSE-STATEMENTS
}
if - else
if - else Example
#include <iostream>
using namespace std;
int main() {
int x;
cin >> x;
if (x%2 == 0) {
cout << "You entered an Even Number!" << endl;
} else {
cout << "You entered an Odd Number!" << endl;
}
return 0;
}
Assignment
Months:
January February March April May June July August September October November December
Thanks
Content
- Motivation
- If Statements - Syntax
- Relational Operators
- If ... else - Syntax
- Assignment
If
By Muhammad Magdi
If
- 188