If Conditions #2
Muhammad Magdi
if statements
if ( CONDITION#1 ) {
TRUE-STATEMENTS#1
} else if ( CONDITION#2 ) {
TRUE-STATEMENTS#2
} else if ( CONDITION#3 ) {
TRUE-STATEMENTS#3
} ... and so on
... else {
FALSE-STATEMENTS
}
Logical Operators
Operator | Meaning |
---|---|
&& | AND |
|| | OR |
! | NOT |
AND Operator
if ( CONDITION1 && CONDITION2 && CONDITION3 && ... ) {
TRUE-STATEMENTS
}
The True-Body (True Statements) is only executed when all the ANDed conditions are true.
OR Operator
if ( CONDITION1 || CONDITION2 || CONDITION3 || ... ) {
TRUE-STATEMENTS
}
The True-Body (True Statements) is executed when one or more of the ORed conditions is true.
Triangle Type
You know that there are 3 types of triangles according to their sides.
You are given 3 integer numbers
(a, b, c) representing the lengths of the sides of a triangle.
Print the type of this triangle according to its sides.
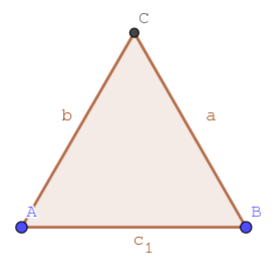
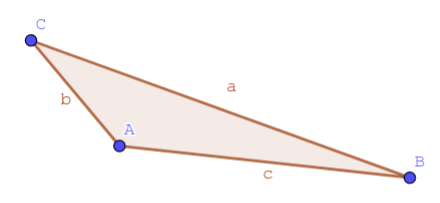
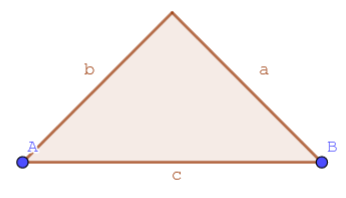
Equilateral
Isosceles
Scalene
Triangle Type [Solution]
#include <iostream>
using namespace std;
int main() {
int a, b, c;
cin >> a >> b >> c;
if (a == b && b == c) {
cout << "Equilateral" << endl;
} else if (a == b || a == c || b == c) {
cout << "Isosceles" << endl;
} else {
cout << "Scalene" << endl;
}
return 0;
}
URI 1052 - Month [Solution]
#include <iostream>
using namespace std;
int main (){
int x;
cin >> x;
if (x == 1) {
cout << "January" << endl;
} else if (x == 2) {
cout << "February" << endl;
} else if (x == 3) {
cout << "March" << endl;
} else if (x == 4) {
cout << "April" << endl;
} else if (x == 5) {
cout << "May" << endl;
} else if (x == 6) {
cout << "June" << endl;
} else if (x == 7) {
cout << "July" << endl;
} else if (x == 8) {
cout << "August" << endl;
} else if (x == 9) {
cout << "September" << endl;
} else if (x == 10) {
cout << "October" << endl;
} else if (x == 11) {
cout << "November" << endl;
} else {
cout << "December" << endl;
}
return 0;
}
URI 1050 - DDD [Solution]
#include <iostream>
using namespace std;
int main() {
int x;
cin >> x;
if (x == 61) {
cout << "Brasilia" << endl;
} else if (x == 71) {
cout << "Salvador" << endl;
} else if (x == 11) {
cout << "Sao Paulo" << endl;
} else if (x == 21) {
cout << "Rio de Janeiro" << endl;
} else if (x == 32) {
cout << "Juiz de Fora" << endl;
} else if (x == 19) {
cout << "Campinas" << endl;
} else if (x == 27) {
cout << "Vitoria" << endl;
} else if (x == 31) {
cout << "Belo Horizonte" << endl;
} else {
cout << "DDD nao cadastrado" << endl;
}
return 0;
}
URI 1044 - Multiples [Solution]
#include <iostream>
using namespace std;
int main (){
int a, b;
cin >> a >> b;
if (a % b == 0 || b % a == 0) {
cout << "Sao Multiplos" << endl;
} else {
cout << "Nao sao Multiplos" << endl;
}
return 0;
}
Thanks
Content
- if ... else if ... else - syntax
- Logical Operators
- AND Operator
- OR Operator
- Triangle type [solution]
- URI 1052 - Month [Solution]
- URI 1050 - DDD [Solution]
- URI 1044 - Multiples [Solution]
If 2
By Muhammad Magdi
If 2
- 185