Learning Apollo:
GraphQLServer
Namir Sayed-Ahmad Baraza
@namirsab in GitHub
-
What is GraphQL?
-
What is Apollo?
-
Example server
GraphQL is a query language for your API, and a server-side runtime for executing queries by using a type system you define for your data
What is GraphQL?
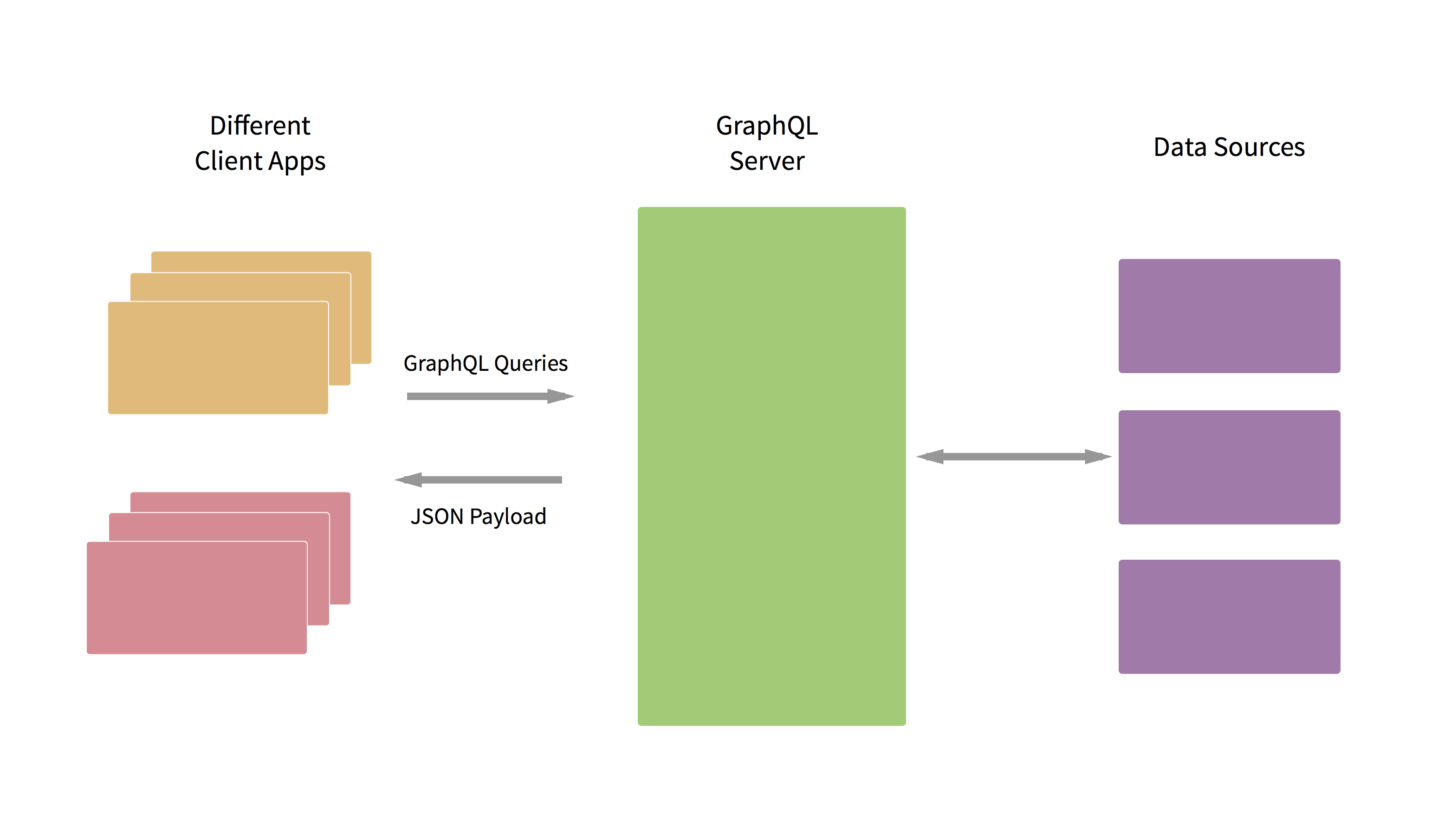
GraphQL Syntax
# Example of a Schema
type Book {
id: ID!,
title: String!
}
type Query {
books(search: String!) : [Book]!
}
# Example Query
{
books(search: "Javascript") {
id,
title
}
}
# Example Result
data: {
books: [{
"id": "_uTRAwAAQBAJ",
"title": "JavaScript and JQuery"
}, {
"id": "PXa2bby0oQ0C",
"title": "JavaScript: The Good Parts"
}, {
"id": "9U5I_tskq9MC",
"title": "Eloquent JavaScript"
}, {
"id": "2weL0iAfrEMC",
"title": "JavaScript: The Definitive Guide"
}]
}
Apollo is a project including a set of tools to develop GraphQL powered apps
What is Apollo?
- ApolloClient (React[Native], Angular, Ios)
-
GraphQL-Server (former ApolloServer):
- Express
- Koa
- Hapi
- Set of utility libraries to make developing with GraphQL super easy
GraphQL Server concepts
Root Schema
It's a GraphQL schema defining the following fields:
# Root Schema
schema {
query: Query
mutation: Mutation
}
- query defines all the queries we can do
- mutation defines all the mutations (queries with side effects) we can do.
Defines the entry point of our GraphQL Schema
Resolvers
A resolver is a function that we can assign to each fild in a type
// Resolvers for the Query type
`type Query {
books(search: String!) : [Book]!
}`
const queryResolvers = {
books: {
description: 'Return all books', // Description of the resolver, not necessary
// Actual resolver function
resolve(root, { search, maxResults, startIndex }) {
return searchBooks(search, { maxResults, startIndex });
}
}
}
We need resolvers in order to response the queries
Resolvers (2)
The resolver function signature looks like this:
function resolve(root, args, context) { return result }
-
root: is the result returned from the resolver in the parent field
-
args: An object arguments into the field query
- context: Shared object by all the resolvers in a particular query, and is used to contain per-request state
Resolvers (3)
The resolver function signature looks like this:
function resolve(root, args, context) { return result }
The result could be one of the following:
- null or undefined (indicates object couldn't be found)
- array
- Promises or array of promises
- Scalar or object value
So, enough words!
Lets go to the code!
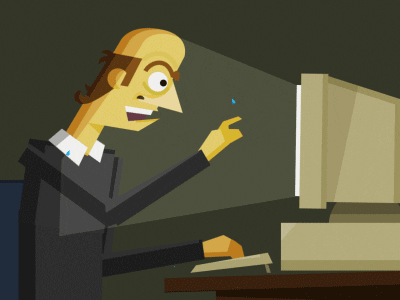
Resources
Learning GraphQL Server (Apollo)
By Namir Sayed-Ahmad Baraza
Learning GraphQL Server (Apollo)
- 944