Understanding HTTPS and HTTPS Servers
for Web Developers
Introduction to HTTP and HTTPS
What is HTTP?
- HTTP (Hypertext Transfer Protocol) is the foundation of communication on the web.
- It is a stateless protocol used for transmitting hypermedia documents, such as HTML.
- Primarily responsible for the request-response cycle between a client (browser) and a server.
How HTTP Works
Basic HTTP Flow:
- Client (Browser) sends an HTTP request to the server.
- Server processes the request and sends back an HTTP response.
- The response typically includes an HTML document, but can also contain JSON, images, or other media.
HTTP Request Example:
GET /index.html HTTP/1.1
Host: example.com
User-Agent: Mozilla/5.0
Accept: text/html
HTTP Response Example:
HTTP/1.1 200 OK
Content-Type: text/html
<html>
<body>
<h1>Welcome to Example.com</h1>
</body>
</html>
Key Features of HTTP
1. Stateless:
- HTTP is stateless, meaning each request is independent and does not retain information about previous interactions.
Key Features of HTTP
2. HTTP Methods:
- GET: Retrieve data from the server.
- POST: Send data to the server.
- PUT: Update existing data.
- DELETE: Remove data from the server.
Key Features of HTTP
3. HTTP Status Codes:
- 200 OK: The request was successful.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: There was a server-side issue.
Limitations of HTTP
Why HTTP is Not Secure:
- Data sent via HTTP is unencrypted, meaning anyone can intercept the data (e.g., login credentials).
- Vulnerable to man-in-the-middle (MITM) attacks.
- Lack of data integrity and authentication mechanisms.
Transition to HTTPS
- To address these issues, the secure version of HTTP was developed: HTTPS (Hypertext Transfer Protocol Secure).
- Let's move forward to understand how HTTPS resolves the limitations of HTTP.
What is HTTPS?
- HTTPS (Hypertext Transfer Protocol Secure) is the secure version of HTTP.
- Encrypts the communication between your browser and the server.
- Ensures data integrity and confidentiality.
Key benefits:
- Data encryption
- Authentication
- Data integrity
How HTTPS Works
- Client sends a request to connect to an HTTPS server.
- Server sends a public key and a certificate to the client.
- Client verifies the certificate's validity.
- Handshake occurs, establishing a secure session.
- Encrypted data is transmitted using the agreed symmetric key.
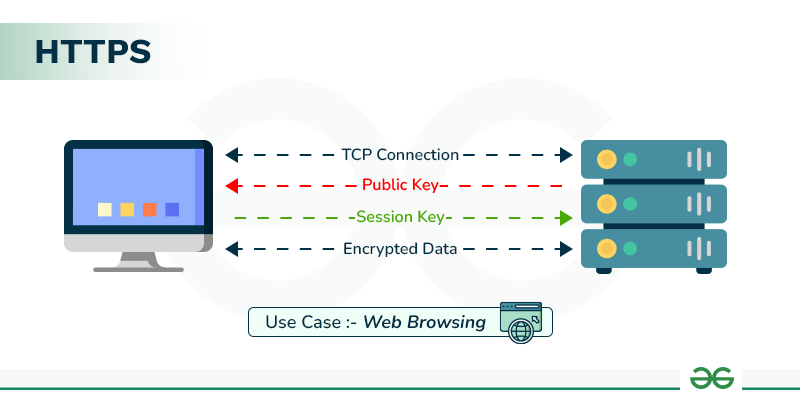
SSL/TLS: The Backbone of HTTPS
SSL (Secure Sockets Layer) vs. TLS (Transport Layer Security)
- SSL is the predecessor of TLS.
- TLS is more secure and is widely used.
Key Roles of SSL/TLS:
- Encryption of data
- Integrity check via hashing
- Authentication through certificates
// Example: HTTPS request in C++
#include <cpprest/http_client.h>
int main() {
web::http::client::http_client client(U("https://example.com"));
auto response = client.request(web::http::methods::GET).get();
std::wcout << "Response: " << response.status_code() << std::endl;
return 0;
}
// Example: HTTPS request in JavaScript (Web)
fetch('https://example.com', {
method: 'GET' // HTTP method (GET, POST, etc.)
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.text(); // or response.json() if the response is JSON
})
.then(data => {
console.log('Response Data:', data); // Log the response data
})
.catch(error => {
console.error('Error:', error); // Handle errors
});
// Example: HTTPS request in Node.js
const https = require('https');
const options = {
hostname: 'example.com',
port: 443,
path: '/',
method: 'GET'
};
const req = https.request(options, (res) => {
console.log(`Status Code: ${res.statusCode}`);
res.on('data', (d) => {
process.stdout.write(d);
});
});
req.on('error', (e) => {
console.error(`Error: ${e.message}`);
});
// End the request
req.end();
HTTPS Certificates
What is an SSL/TLS Certificate?
- A digital certificate that authenticates a server’s identity.
- Issued by a Certificate Authority (CA), such as Let's Encrypt or DigiCert.
Types of Certificates:
- Domain Validation (DV): Verifies ownership of the domain.
- Organization Validation (OV): Validates ownership and the organization.
- Extended Validation (EV): Highest level of validation, displaying a green address bar.
HTTPS Certificates
Types of Certificates:
- Domain Validation (DV): Verifies ownership of the domain.
- Organization Validation (OV): Validates ownership and the organization.
- Extended Validation (EV): Highest level of validation, displaying a green address bar.
How to Set Up HTTPS on Your Server
Steps to configure HTTPS:
- Obtain an SSL/TLS certificate from a CA (e.g., Let’s Encrypt).
- Install the certificate on your web server (Apache, Nginx, etc.).
- Update your web server configuration to support HTTPS.
- Redirect HTTP to HTTPS for secure access.
Example: Setting up HTTPS on Nginx
# Install Certbot to obtain a certificate
sudo apt install certbot python3-certbot-nginx
# Obtain an SSL certificate for your domain
sudo certbot --nginx -d yourdomain.com -d www.yourdomain.com
# Certbot will automatically configure Nginx to use the certificate
Best Practices for HTTPS
Things to Consider:
- Always use HTTPS across your site.
- Implement HSTS (HTTP Strict Transport Security) to enforce HTTPS.
- Renew your certificates before they expire.
- Enable HTTP/2 to improve performance over HTTPS.
# Example Nginx HTTPS configuration with HSTS
server {
listen 443 ssl;
server_name yourdomain.com;
ssl_certificate /etc/letsencrypt/live/yourdomain.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/yourdomain.com/privkey.pem;
add_header Strict-Transport-Security "max-age=31536000" always;
}
Why HTTPS Matters for SEO
SEO Benefits of Using HTTPS
- Google ranks HTTPS sites higher in search results.
- Improved user trust due to security indicators (padlock icon).
- Protects your site from MITM attacks and eavesdropping.
Example:
- HTTP site:
http://example.com
- HTTPS site:
https://example.com
(ranked better)
Conclusion
- HTTPS is essential for modern web development.
- It enhances security, builds trust, and improves SEO.
- Follow best practices to keep your HTTPS configurations secure.
HTTP/HTTPS Servers Overview
What is a Web Server?
- A web server is software that serves web content (like HTML, CSS, JS) to clients over HTTP or HTTPS.
- Popular options include:
- Apache HTTP Server
- Nginx
- LiteSpeed
- Caddy
- Node.js (when used with HTTP modules)
Apache HTTP Server
Overview of Apache
- Apache is one of the most widely used web servers, created in 1995.
- Developed by the Apache Software Foundation.
- Highly configurable and modular.
- Suitable for dynamic content (e.g., PHP) and shared hosting environments.
Key Features:
- .htaccess: Per-directory configuration files.
- Module-based architecture: Load or disable modules like SSL, rewrite, etc.
- Supports both process-based and thread-based handling.
Nginx
Overview of Nginx
- Nginx (pronounced "engine-x") was released in 2004.
- Known for its high performance and ability to handle a large number of concurrent connections.
- Commonly used as a reverse proxy, load balancer, and HTTP server.
Key Features:
- Event-driven architecture for high concurrency.
- Efficient handling of static content.
- Built-in support for reverse proxying and load balancing.
Feature | Apache | Nginx |
---|---|---|
Architecture | Process-based | Event-driven |
Static Content | Slower at serving static files | Optimized for static files |
Dynamic Content | Better suited for PHP/CGI | Usually paired with FastCGI |
Reverse Proxy | Supported via modules | Built-in reverse proxying |
Configuration | Per-directory (.htaccess ) |
Centralized (one config file) |
Concurrency | Not optimized for high concurrency | Excellent for handling many connections |
Memory Usage | Higher memory usage per connection | Low memory usage per connection |
LiteSpeed Web Server
Overview of LiteSpeed
- LiteSpeed is a commercial web server designed to offer a balance between performance and ease of use.
- Known for its high speed and compatibility with Apache configurations (e.g.,
.htaccess
).
Key Features:
-
Apache-compatible: Supports Apache's
.htaccess
and mod_rewrite. - Built-in caching: Integrated LSCache for speeding up dynamic content.
- Efficient handling of PHP with PHP LSAPI.
Caddy Web Server
Overview of Caddy
- Caddy is a modern web server with automatic HTTPS and easy configuration.
- Zero-configuration HTTPS: Automatically manages SSL certificates with Let's Encrypt.
Key Features:
- Automatic HTTPS: Obtain and renew SSL certificates automatically.
-
Simple configuration: Minimalist and easy-to-read
Caddyfile
. - HTTP/2 and HTTP/3 support out of the box.
Use Case Comparison
When to Use Apache:
-
Shared hosting: Apache’s
.htaccess
allows per-user configurations. - Dynamic content: Apache works well for PHP, Perl, or other CGI-based content.
- Highly configurable for complex setups (e.g., URL rewriting, authentication).
When to Use Nginx:
- High-traffic sites: Nginx handles a large number of concurrent users efficiently.
- Static content: Optimal for serving static assets like images and CSS.
- As a reverse proxy or load balancer in front of another web server.
Use Case Comparison
When to Use LiteSpeed:
- Performance focus: Websites needing high performance for dynamic content and caching.
- Compatible with existing Apache configurations while offering better performance.
When to Use Caddy:
- Simpler deployments: If you want automatic HTTPS without much configuration.
- Modern web stacks that need HTTP/2 or HTTP/3 and simple configuration.
Conclusion: Choosing the Right Server
- Apache: Best for dynamic content and shared hosting.
- Nginx: Great for high-performance static content and large-scale systems.
- LiteSpeed: A good balance between Apache compatibility and Nginx-like performance.
- Caddy: Perfect for automatic HTTPS and minimal configuration.
Choose the server that best fits your traffic, content, and infrastructure needs.
Overview of Each Server:
-
Apache: Flexible and robust, best suited for dynamic websites and complex configurations. It is ideal for hosting environments where
.htaccess
files are necessary for user-level customizations. - Nginx: Known for high efficiency and speed, Nginx is particularly good for handling high volumes of traffic and is often used as a reverse proxy and load balancer.
- LiteSpeed: Offers performance improvements over Apache, especially for WordPress and dynamic content-heavy websites, while maintaining compatibility with Apache configurations.
- Caddy: A modern, lightweight web server designed for developers who want automatic HTTPS and HTTP/2/3 support with minimal setup effort.
Overview of Each Server:
Introduction: HTTP/2 vs HTTP/3
- HTTP/2 and HTTP/3 are modern versions of the Hypertext Transfer Protocol (HTTP), designed to improve performance and security.
- HTTP/2: Released in 2015, bringing multiplexing and other optimizations.
- HTTP/3: Released in 2022, uses a different transport protocol (QUIC) for faster, more reliable connections.
HTTP/2: Key Features
Overview of HTTP/2:
- Officially standardized in 2015, it's the successor to HTTP/1.1.
- Primary goal: Performance improvements for web content delivery.
Key Features:
- Multiplexing: Multiple requests and responses can be sent over a single connection without blocking.
- Header compression: Uses HPACK to reduce the size of headers.
- Server push: Servers can "push" resources to the client before they are requested.
- Binary protocol: More efficient than HTTP/1.1’s text-based protocol.
HTTP/3: Key Features
Overview of HTTP/3:
- HTTP/3 was officially standardized in 2022.
- It uses the QUIC transport protocol, which is based on UDP instead of TCP.
Key Features:
- QUIC: A faster, low-latency transport layer using UDP.
- Faster connection establishment: Reduces round-trip times (RTT) by combining the transport and TLS handshake.
- Improved resilience: Less affected by packet loss due to stream-level error correction.
- Built-in encryption: Always encrypted, with TLS 1.3 as a default.
Feature | HTTP/2 | HTTP/3 |
---|---|---|
Transport Protocol | TCP (Transmission Control Protocol) | QUIC (built on UDP) |
Multiplexing | Supported, but head-of-line blocking can occur on packet loss | Fully multiplexed with no head-of-line blocking |
Connection Setup | Requires multiple RTTs for TCP and TLS | Faster connection setup due to combined transport and TLS handshake |
Encryption | Optional (but commonly used with HTTPS) | Always encrypted (TLS 1.3 by default) |
Packet Loss Handling | Affects all streams (head-of-line blocking) | Losses are handled per stream without blocking others |
Deployment | Widely deployed and mature | Newer, not as widespread but growing fast |
Performance Comparison
HTTP/2 Performance:
- Better than HTTP/1.1: Multiplexing significantly reduces latency.
- Head-of-line blocking: Still a problem when packet loss occurs (since HTTP/2 is over TCP).
HTTP/3 Performance:
- Lower latency: QUIC eliminates head-of-line blocking at the transport layer.
- More efficient on mobile networks: Faster recovery from packet loss.
- Quicker connection establishment: With QUIC, connections are established faster due to fewer round-trip times.
Server Support for HTTP/2 and HTTP/3
HTTP/2 Server Support:
- Widely supported by most modern web servers and CDNs:
-
Apache: Supports HTTP/2 via the
mod_http2
module. -
Nginx: HTTP/2 support is enabled with the
--with-http_v2_module
. - LiteSpeed: Fully supports HTTP/2.
- Caddy: Supports HTTP/2 out of the box.
-
Apache: Supports HTTP/2 via the
# Enabling HTTP/2 in Nginx
server {
listen 443 ssl http2;
ssl_certificate /etc/nginx/ssl/nginx.crt;
ssl_certificate_key /etc/nginx/ssl/nginx.key;
}
HTTP/3 Server Support:
- Growing adoption, but requires newer server versions:
- Nginx: HTTP/3 is supported experimentally since version 1.25 (requires QUIC).
- LiteSpeed: Full support for HTTP/3.
- Caddy: Supports HTTP/3 natively, with QUIC enabled by default.
- Cloudflare: Supports HTTP/3 on its network.
# Enabling HTTP/3 in Nginx (experimental)
server {
listen 443 ssl http2;
listen 443 quic reuseport;
ssl_certificate /etc/nginx/ssl/nginx.crt;
ssl_certificate_key /etc/nginx/ssl/nginx.key;
http3_max_concurrent_streams 128;
}
Browser Support for HTTP/2 and HTTP/3
HTTP/2 Browser Support:
-
Fully supported by all modern browsers:
- Chrome, Firefox, Edge, Safari, Opera.
HTTP/3 Browser Support:
-
Supported by major browsers, but some still mark it as experimental:
- Chrome (enabled by default).
- Firefox (enabled by default).
- Safari (enabled by default since Safari 14).
Use Cases for HTTP/2 vs HTTP/3
When to Use HTTP/2:
- General web content: HTTP/2 provides significant performance benefits for static and dynamic content.
- Websites with limited packet loss: Works well in stable network environments where packet loss is rare.
Use Cases for HTTP/2 vs HTTP/3
When to Use HTTP/3:
- Mobile networks or unreliable connections: HTTP/3 shines in networks with higher packet loss.
- Real-time applications: For apps that require low latency and fast reconnections (e.g., video streaming, gaming, VoIP).
- Websites with global users: HTTP/3 helps reduce latency for users far from your server.
Conclusion: Choosing Between HTTP/2 and HTTP/3
- HTTP/2: Excellent for improving web performance over stable connections, with widespread support.
- HTTP/3: A newer, faster protocol with significant improvements in connection handling and latency, especially on unreliable networks.
- Server Adoption: While HTTP/2 is ubiquitous, HTTP/3 adoption is growing rapidly, and major servers like Nginx, LiteSpeed, and Caddy already support it.
Conclusions:
- Introduction: Provides context about HTTP/2 and HTTP/3.
- HTTP/2 Key Features: Discusses the advantages of HTTP/2 over HTTP/1.1.
- HTTP/3 Key Features: Introduces the QUIC protocol and improvements with HTTP/3.
- Comparison: Detailed side-by-side comparison of HTTP/2 and HTTP/3 in terms of transport, encryption, and packet handling.
- Performance: Focuses on connection speed, latency, and how they handle packet loss.
- Server Support: Lists which web servers support HTTP/2 and HTTP/3, with configuration examples.
- Browser Support: Clarifies which browsers are compatible with both protocols.
- Use Cases: Recommends when to use HTTP/2 or HTTP/3 depending on your network and application needs.
Understanding HTTPS and HTTPS Servers for Web Developers
By Néstor Aldana
Understanding HTTPS and HTTPS Servers for Web Developers
- 108