@nikgraf

React VR




Who knows "A Hitchhiker's Guide to the Galaxy"?
Who of you heard of React VR?
Who of has tried React VR?






Head tracking
Lenses
Screen
The critical pieces
Head mount
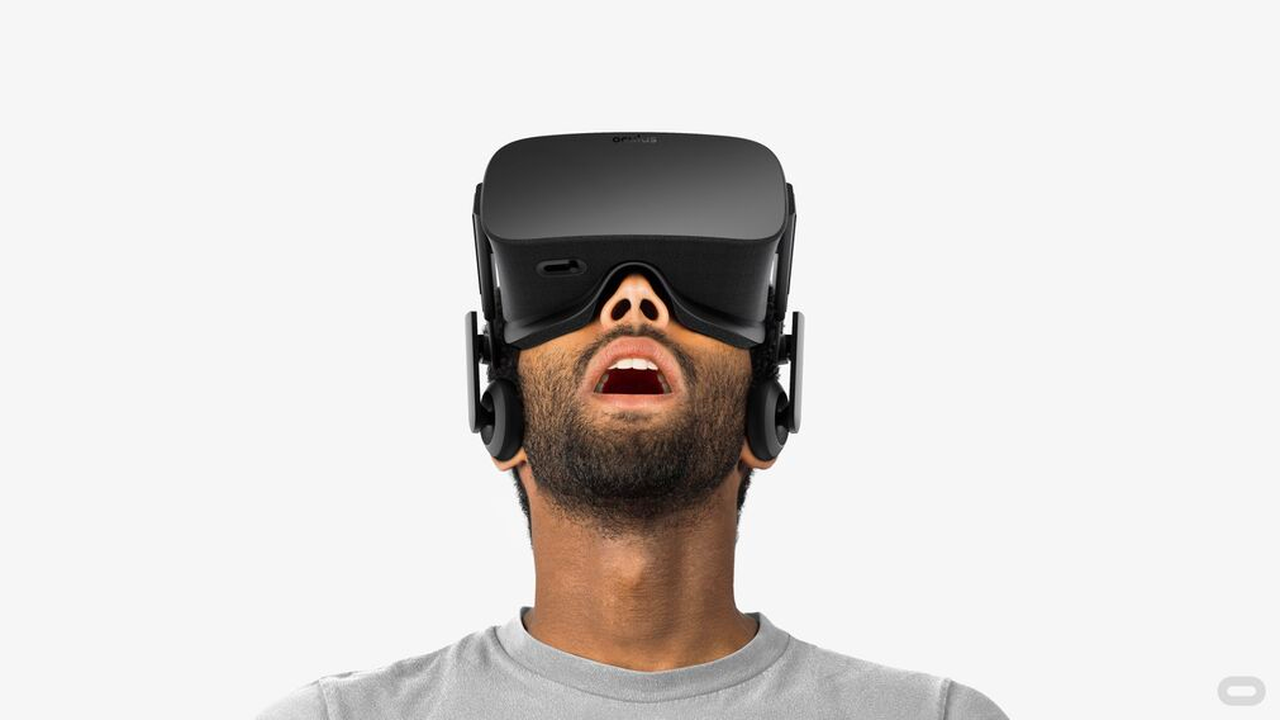



How do you make your users look like this?
Immersiveness through lens distortion


Lens*
Physical screen
Virtual Screen
With Lens
Without Lens
*Convex Fresnel Lens

Pincussion Distortion
Projected image
Perceived image


Barrel Distortion
Projected image
Perceived image


https://w3c.github.io/webvr/
WebVR is an experimental JavaScript API that provides access to Virtual Reality devices, such as the Oculus Rift, HTC Vive, Samsung Gear VR, or Google Daydream, in your browser.

Browser enthusiasm
Browser support

Browser installation and configuration instructions
Supported Web Browser
Supported Web Browser
Mobile
Desktop
Virtual Reality Headset
Smartphone and Lenses
Requirements
WebGL
WebVR


React VR

Let's travel a bit …
(Don't forget to bring a towel)

yarn global add react-vr-cli
or
npm install -g react-vr-cli
Files
- package.json
- yarn.lock
- node_modules
- rn-cli.config.js
- vr/client.js
- vr/index.html
- index.vr.js
- static_assets/chess-world.jpg
<!-- vr/index.html -->
<html>
<head>
<title>firstproject</title>
<style>body { margin: 0; }</style>
<head>
<body>
<script src="./client.bundle?platform=vr"></script>
<script>
ReactVR.init(
'../index.vr.bundle?platform=vr&dev=true',
document.body
);
</script>
</body>
</html>
// vr/client.js
import {VRInstance} from 'react-vr-web';
function init(bundle, parent, options) {
const vr = new VRInstance(
bundle,
'firstproject',
parent,
{ ...options }
);
vr.render = function() {
// Any custom behavior you want to perform
// on each frame goes here
};
// Begin the animation loop
vr.start();
return vr;
}
window.ReactVR = {init};
// index.vr.js (part 1)
import React from 'react';
import {
AppRegistry,
asset,
StyleSheet,
Pano,
Text,
View
} from 'react-vr';
// index.vr.js (part 2)
class firstproject extends React.Component {
render() { return (
<View>
<Pano source={asset('chess-world.jpg')}/>
<Text style={{
backgroundColor:'blue',
textAlign:'center',
textAlignVertical:'center',
layoutOrigin: [0.5, 0.5],
transform: [{translate: [0, 0, -3]}],
}}>
hello
</Text>
</View>
)}
};
AppRegistry.registerComponent(
'firstproject', () => firstproject
);



class firstproject extends React.Component {
render() { return (
<View>
<Pano source={asset('reactamsterdam.jpg')}/>
<Text style={{
fontSize: 0.4,
layoutOrigin: [0.5, 0.5],
transform: [{translate: [0, 0, -3]}],
}}>
Welcome to React Amsterdam!
</Text>
</View>
)}
};
AppRegistry.registerComponent(
'firstproject', () => firstproject
);
Let's travel to Forestonia, maybe learn about it's history.
How to create an Object!

cube.obj
cube.mtl
<View>
<PointLight style={{
color:'white',
transform:[{translate : [0, 400, 700]}]}}
/>
<Pano source={asset('chess-world.jpg')}/>
<Model
style={{ transform: [
{translate: [0, -5, -20]},
{rotateY: -30},
{rotateX: -40}
] }}
source={{
obj: asset('cube2.obj'),
mtl: asset('cube2.mtl')
}}
lit
/>
</View>



// Tree.js
import React from 'react';
import {asset, Model, View} from 'react-vr';
export default ({ style }) => (
<View
style={style}
>
<Model
source={{
obj: asset('tree-trunk.obj'),
mtl: asset('tree-trunk.mtl')
}}
lit
/>
<Model
source={{
obj: asset('tree-crown.obj'),
mtl: asset('tree-crown.mtl')
}}
lit
style={{
transform: [ {translate: [0, 2.5, 0]} ]
}}
/>
</View>
);
<Tree
style={{ transform: [{translate: [0, -1, -5]}] }}
/>
<Tree
style={{ transform: [{translate: [5, -1, -5]}] }}
/>
import Tree from './components/Tree';
class MyForest extends React.Component {
render() {
return (
<View>
<Tree
style={{ transform: [{translate: [0, -1, -5]}] }}
/>
<Tree
style={{ transform: [{translate: [5, -1, -5]}] }}
/>
<AmbientLight intensity={0.4} />
<PointLight
style={{color: 'white', transform: [{translate: [0, 400, 700]}]}}
/>
<Pano source={asset('heaven.png')} />
<Model
source={{obj: asset('plane.obj'), mtl: asset('plane.mtl')}}
lit
style={{ transform: [{translate: [0, -1, 0]}] }}
/>
</View>
);
}
}
AppRegistry.registerComponent('MyForest', () => MyForest);
// Forest.js Part 1
import { range, map, xprod, flatten } from 'ramda';
import Tree from './Tree';
const randomPosition = () => (Math.floor(Math.random() * 10) - 5);
const randomHeight = () => (Math.random() - 1);
const randomScale = () => (Math.random() * 0.25 + 1);
const grid = xprod(range(0, 10), range(0, 10));
const trees = grid.map((entry, index) => {
return {
x: entry[0] * 8 + randomPosition(),
y: entry[1] * 8 + randomPosition(),
id: index
};
});
// Forest.js
export default ({ style }) => (
<View style={style}>
{trees.map((tree) => {
return (
<Tree
key={tree.id}
style={{
transform: [
{translate: [
tree.x, randomHeight(),
tree.y
]},
]
}}
/>
);
})}
</View>
);
<Forest
style={{
transform: [{translate: [-35, -1, -35]}],
}}
/>

Who is going to start with React VR tonight?
export default class Planet extends React.Component {
constructor(props) {
super(props);
this.state = {
rotationX: new Animated.Value(0),
};
}
componentDidMount() {
Animated.timing(valueReference, {
duration: 300,
toValue: 68,
}).start();
}
render() {
return (
<View>
<Marvin
style={{
transform: [
{translate: [3, -2, -8]},
{rotateY: 20},
{rotateX: this.state.rotationX},
],
}}
/>
What's the meaning of all that?
https://github.com/nikgraf/webvr-experiments


A Hitchhiker's Guide to React VR
By nikgraf
A Hitchhiker's Guide to React VR
- 1,260