Introduction to Javascript

Topics
- What is Javascript
- Js basics: variables, functions, data types...
- Working with simple types
- Objects and arrays
- Conditionals
- Loops
- Introduction to functional programming
- Extra: managing asyncronism
What is Javascript?
Is
- A programming language
- Interpreted
- Dynamic (not compiled)
- Weakly typed
- Prototype based (object oriented)
- Multi-paradigm: event driven, functional, or imperative
Isn't
- Java
- Only for the client side
- NodeJs
A bit of history
Year | Version | Support |
---|---|---|
1997 | ECMAScript 1 | All browsers |
1998 | ECMAScript 2 | All browsers |
1999 | ECMAScript 3 | All browsers |
2009 | ECMAScript 5 | All modern browsers |
2015 | ECMAScript 6 | Partial |
? | ECMAScript 7 | Poor |
Retrocompatible
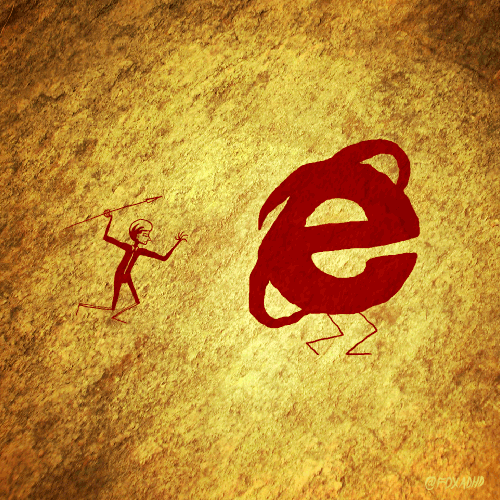
JS basics
A variable is a named container for a value
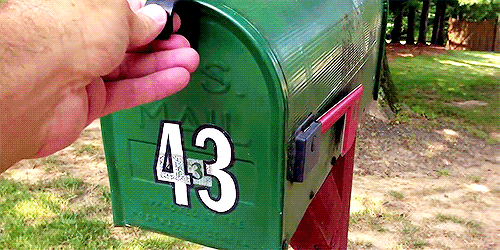
Three ways to declare it
- var
- let
- const
But you can leave it empty right now! (except constant)
var imperator = 'Furiosa';
let auntyEntity = 'Tina Turner';
const beyondThunderdome = 3;
var master;
let blaster;
const hasToBeInitialized = true;
Data types
string
let text = 'mediocre';
number
let pi = 3.141592;
null
let what = null;
undefined
let none = undefined;
boolean
let yesOrNo = true;
object
let complex = {
key: 'value',
evenMoreObjects: {
yes: false
}
};
Array
Function
built-in objects
error
console.log(Math.PI) // 3.141592653589793
console.log(Date.now()) // 1525979416506
const warlords = ['Immortan Joe', 'Bullet Farmer'];
new Error('I live, I die, I live again');
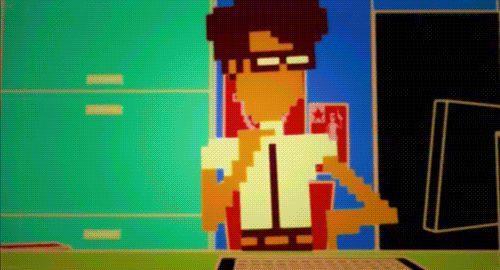
Play time!
A function is a fragment of code that can be invoked any time
When invoked, it can receive a list of arguments
Two different sintax, almost the same
function greet() { // ES5
console.log('hi!');
}
greet();
const sayBye = () => { // ES6
console.log('bye bye')
}
sayBye();
function ask(question) {
console.log(question + '?');
}
ask('who killed the world');
const yell = (text) => {
console.log(text + '!!')
}
yell('two enter, one leaves');
An array is a list of values, usually of the same type
Is an object with special methods, where the keys are automatically set
const originalSaga = [3, 'Mad Max', { bestOne: false }];
console.log(originalSaga[0]); // 3
console.log(originalSaga[2].bestOne); // false
working with simple types
arithmetic operators
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Remainder |
** | Exponential |
-- | Decrement |
++ | Increment |
function addOne(a) {
return ++a;
}
function squaredAdd(a, b) {
return (a ** 2) + (b ** 2) + (a * b);
}
Comparison operators
function areLoselyEqual(a, b) {
return a == b;
}
function isNegativeNumber(a) {
return a < 0;
}
< | Less |
<= | Less or equal |
> | Greater |
>= | Greater or equal |
== or === | Equal |
!= or !== | Not equal |
Logical operators
function isTheLetterA(letter) {
return letter === 'a' || letter === 'A';
}
function isBetween(target, min, max) {
return target >= min && target <= max;
}
&& | And |
|| | Or |
! | Not |
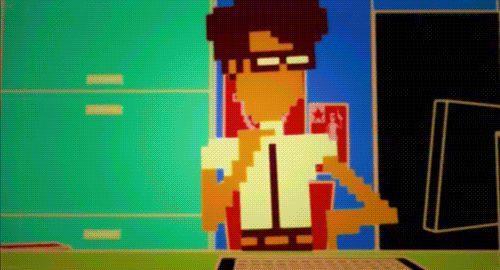
Practice time!
objects && arrays
accessing values
Object values can be accessed using the dot notation or the brackets notation
const madMax4 = {
year: new Date('7/May/2015'),
title: 'Mad Max: Fury Road',
director: 'George Miller'
};
console.log(madMax4.title);
console.log(madMax4['director']);
const someProp = 'year';
console.log(madMax4[someProp]);
accessing values
madMax4.stars = [
'Tom Hardy',
'Charlize Theron'
];
Array values can only be accessed with the brackets notation, using the index
We can add or modify values using the assignment operator (=)
console.log(madMax4.stars[1]);
madMax4.stars[1] = 'Charlize <3';
Object methods
entries
assign
keys
values
console.log(Object.values(madMax2));
console.log(Object.entries(madMax2));
console.log(Object.keys(madMax2));
const filmBase = {director: 'George Miller'};
const madMax2 = Object.assign(
{title: 'Road Warrior'},
filmBase
);
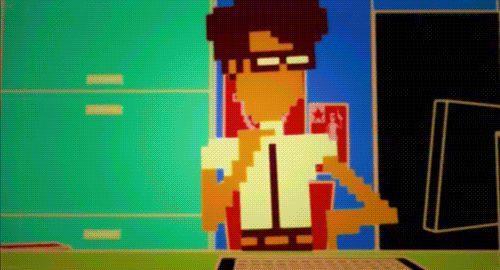
Practice time!
array props and methods
length
pop
push
soundtrack.push('Buzzards Arrive');
soundtrack.pop();
const soundtrack = [
'Survive',
'Escape',
'Immortan\'s citadel',
'Blood bag'
];
soundtrack.length; // 4
array props and methods
indexof
soundtrack.indexOf('Escape');
sort
soundtrack.sort();
objectsArray.sort((a, b) => {
return a.value - b.value;
});
concat
soundtrack.concat(['Spikey Cars']);
join
soundtrack.join(' - ')
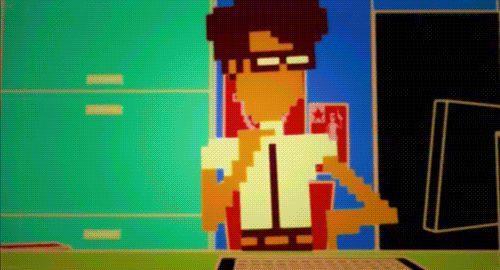
Practice time!
conditionals
Conditional structures allow to execute or not a piece of code depending on a decision
if else
The if...else structure is usefull to execute statements if a condition is true
function whoIsMadMax(film) {
if (film <= 3) {
return 'Mel Gibson';
} else {
return 'Tom Hardy';
}
}
if else
We can use only the if part, or chain any number of else if
function getArea(poligon, side) {
if (poligon === 'square') {
return side * side;
} else if (poligon === 'triangle') {
return side * side / 2;
} else {
return new Error('Sorry')
}
}
Ternary operator
The ternary operator is an abbreviated if else.
function whoIsMadMax(film) {
return film <= 3
? 'Mel Gibson'
: 'Tom Hardy';
}
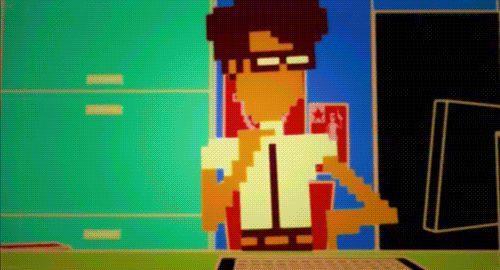
Practice time!
Loops
A loop is a structure that allow us to repeat the same code a certain number of times
Loops can be placed inside other loops, which is called nested loop. Be aware, so they can increase the execution time exponentially.
A loop has (implicit or explicitly) three elements:
- Counter
- Exit condition
- Iterator
for
The for loop is the most basic form of loop in javascript
const wives = [
'Angharad',
'Toast the Knowing',
'Capable',
'The Dag',
'Cheedo the Fragile'
];
for (let i = 0; i < wives.length; i++) {
console.log(`${i}: ${wives[i]}`);
}
Counter, exit condition and iterator are easily identifiable
for eACH
forEach is a shorter way to iterate over the elements of an array
const wives = [
'Angharad',
'Toast the Knowing',
'Capable',
'The Dag',
'Cheedo the Fragile'
];
wives.forEach((wife, i) => {
console.log(`${i}: ${wife});
});
for of
for ... in is the object-equivalent of forEach
const gangs = {
theAquifer: 'Immortan Joe',
bulletFarm: 'Bullet Farmer',
gasTown: 'People Eater'
};
for (const place in gangs) {
console.log(
`${place} is lead by ${gangs[place]}`
);
}
while
The while statement does not need an iterator, it will run until a condition is true
let theWivesAreSave = false;
while (immortanJoe.isAlive) {
tryToKill(immortanJoe);
}
theWivesAreSave = true;
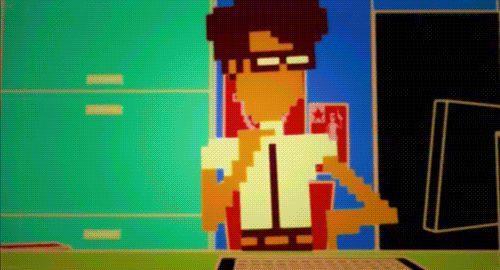
Practice time!
Functional programing 101
disclaimer: as a frontend dev, i'm not good at this
Functional programming (often abbreviated FP) is the process of building software by composing pure functions, avoiding shared state, mutable data, and side-effects.
Advantages
- Easier to test
- More predictable
- Smaller pieces = reusability
Pure functions
// very bad
const truck = { rider: 'Furiosa', gear: 0, started: false };
function startTruck() {
if (truck.rider !== 'Furiosa') {
return;
} else {
truck.gear = 1;
truck.started = true
}
}
startTruck();
- Given the same input, return the same output
- No side effects
shared state
// still bad
function startTruck(truck) {
if (truck.rider !== 'Furiosa') {
return false;
} else {
truck.gear = 1;
truck.started = true
}
}
startTruck({ rider: 'Furiosa', gear: 0, started: false });
Shared state is a variable, object, etc that exists in a shared scope.
// still bad
function startTruck(truck) {
if (truck.rider !== 'Furiosa') {
return;
} else {
truck.changeGear(1);
truck.started = true
}
}
startTruck({ rider: 'Furiosa', gear: 0, started: false });
Mutable data
Data shouldn't be modified after it has been created.
Be aware, that const does not mean the data is immutable
// not so bad
function startTruck(truck) {
if (truck.rider !== 'Furiosa') {
return Object.assign({}, truck, {started: false});
} else {
truck.changeGear(1);
return Object.assign({}, truck, {started: true});
}
}
startTruck({ rider: 'Furiosa', gear: 0, started: false });
side effects
A side effect is any change visible outside the called function
(apart from the returned value)
// done!
function startTruck(truck) {
return Object.assign(
{}, truck, {started: truck.rider === 'Furiosa'}
);
}
const truck = startTruck(
{ rider: 'Furiosa', gear: 0, started: false }
).changeGear(1);
map
Creates a new array with the results of calling a function over the elements of other array
const characters = [
'Imperator',
'Mad Max',
'Auntie',
'Toecutter'
];
const everybodyGetsACar = characters
.map(name => `${name} gets a car!`);
The function needs to return the new element
reduce
Similar to map, but reduces the array to a single value
const characters = [
'Imperator',
'Mad Max',
'Auntie',
'Toecutter'
];
const everybodyGetsACar = characters
.reduce((sentence, name) => {
return `${sentence} ${name}, `;
}, 'All of them get a car: ');
The initial value is optional
filter
Filter returns a new array containing only some values, depending on a filtering condition
const someNumbers= [15, 8, 4, 42, 23, 16];
const smallNumbers= someNumbers
.filter(num => num <= 15);
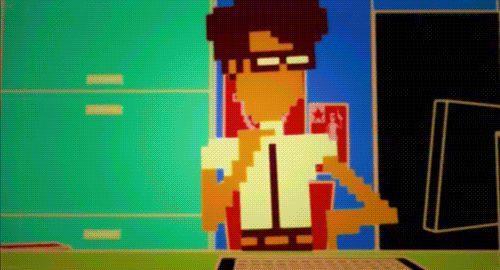
Practice time!
Managing asyncronism
Sometimes, a line of code will schedule something to be executed later, while the rest of the code continues running. This is called asyncronism
In javascript this is usually related to http calls or database modifications
callbacks (ES5)
promises (ES6)
async await (ES7)
asynFunction('p', result => {
console.log(result);
});
asynFunction('p').then(result => {
console.log(result);
});
async function asynFunction(p) {...}
const result = await asynFunction('p');
console.log(result);
Introduction to javascript
By Paqui Calabria
Introduction to javascript
- 1,588