Single-page Application
(SPA)
What is Single-page Application?
- a web application or website on a single web page with the goal of providing a more fluid user experience
- all necessary code (HTML, JS, CSS) is retrieved with a single page load or dynamically loaded and added to the page as necessary, usually in response to user actions
Characteristics
- "Desktop application" like interaction
- Back/Forward Button works as expected
- More JavaScript than actual HTML
- Ability to go Offline
- Dynamic data loading from the server-side API
- Fluid transitions between page states
- HTML, CSS and JS required for the application is sent with the initial request
- Page changes occur via JavaScript using templates and DOM manipulation
- URL hashes are used to track state and provide bookmark-able links
AngularJS
"Superheroic JavaScript MVW Framework"
http://angularjs.org
What is AngularJS?
- AngularJS is a structural framework for dynamic web apps
-
AngularJS takes care of advanced features that users have become accustomed to in
modern web applications, such as:
-
Separation of application logic, data models, and views
-
Ajax services
-
Dependency injection
-
Testing
-
And more
-
CONCEPTS
AngularJS
Data Binding
- Data-binding in Angular apps is the automatic synchronization of data between the model and view components
- When the model changes, the view reflects the change, and vice versa.
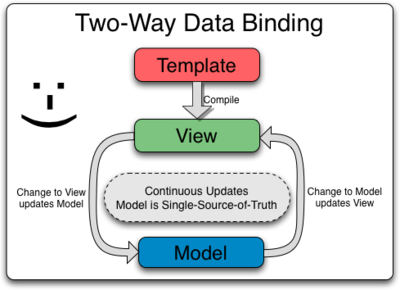
How Data Binding works?
-
Angular simple remembers the old value and compares with new one
- This process is called "dirty checking" - if the value gets dirty or changed from what it has maintained then events associated gets executed
- example : http://plnkr.co/edit/hHIeaiyY5k5sHPhpdjR5?p=preview
How Data Binding works?
-
Angular simple remembers the old value and compares with new one
- This process is called "dirty checking" - if the value gets dirty or changed from what it has maintained then events associated gets executed
How Data Binding works?
$scope.$watch
- This function takes an expression and a callback: the callback will be called when the value of the expression changes. For example, lets say our scope has a variable name, and we want to update the firstName and lastName variables every time name changes
- Under the hood, each scope has a list of watchers, internally called $scope.$$watchers, which contain the expression and the callback function. The $watch simply adds a new watcher to the $$watchers array, which AngularJS loops over when it thinks something that can change the state of the scope
$scope.$watch('name', function(value) {
var firstSpace = (value || "").indexOf(' ');
if (firstSpace == -1) {
$scope.firstName = value;
$scope.lastName = "";
} else {
$scope.firstName = value.substr(0, firstSpace);
$scope.lastName = value.substr(firstSpace + 1);
}
});
How Data Binding works?
$scope.$apply([expression])
- When called without arguments, $apply lets AngularJS know that something happened that may have changed the state of the scope
- used to execute an expression in angular from outside of the angular framework (For example from browser DOM events, setTimeout, XHR or third party libraries)
How Data Binding works?
$scope.$digest()
- $digest() runs through every watcher in the scope, evaluates the expression, and checks if the value of the expression has changed. If the value has changed, AngularJS calls the change callback with the new value and the old value
- you will never actually call $digest() directly, since $apply() does that for you
Modules
-
In Angular, a module is the main way to define an AngularJS app
- You can think of a module as a container for the different parts of your app – controllers, services, filters, directives, etc.
- Using modules gives us a lot of advantages, such as:
-
Keeping our global namespace clean
-
Making tests easier to write and keeping them clean so as to more easily target isolated
functionality
-
Making it easy to share code between applications
-
Defining a module
<script>
var app = angular.module('myModule', ['myDependency']);
</script>
<!-- Use It->
<!DOCTYPE html>
<html ng-app="myModule">
<head></head>
<body></body>
</html>
The Angular module API allows us to declare a module using the angular.module() API method.
We can always reference our module by using the same method with only one parameter. For instance, we can reference the "myModule" module like so:
var myModule = angular.module('myModule');
Scope
- serves as the glue between the controller and the view
- context where the model is stored so that controllers, directives and expressions can access it
- an object that refers to the application model
- the values that are stored in variables on the scope are referred to as the"model"
- plnkr: http://plnkr.co/edit/1Gu7GxKL1acW23QVbVNu
angular.module('scopeExample', [])
.controller('MyController', ['$scope', function($scope) {
$scope.username = 'World';
$scope.sayHello = function() {
$scope.greeting = 'Hello ' + $scope.username + '!';
};
}]);
<div ng-controller="MyController">
Your name:
<input type="text" ng-model="username">
<button ng-click='sayHello()'>greet</button>
<hr>
{{greeting}}
</div>
Controller
- Controllers in AngularJS is a function that adds additional functionality to the scope of the view
- We use it to set up an initial state and to add custom behavior to the $scope object
- When we create a new controller on a page, Angular passes it a new $scope . This new $scope is where we can set up the initial state of the scope on our controller
-
Do not use controllers to
- Manipulate DOM, use directives instead
- Format input — Use angular form controls instead.
- Filter output — Use angular filters instead.
- Share code or state across controllers — Use angular services instead.
- plnkr: http://plnkr.co/edit/6pdiyLvUJ4iSI5Qa1kWk?p=preview
Expressions
- Angular expressions are JavaScript-like code snippets that are usually placed in bindings such as {{ expression }}
-
For example, these are valid expressions in Angular
- 1 + 2
- a + b
- user.name
- items[index]
<span>
1+2={{1+2}}
</span>
Output:
1+2=3
Filters
- A filter formats the value of an expression for display to the user. They can be used in view templates, controllers or services
- E.g. the markup {{ 12 | currency }} formats the number 12 as a currency using the currency filter. The resulting value is $12.00
- we can also create custom filters:http://plnkr.co/edit/v8WegM7qFIvJrg71wjM6?p=preview
Directives
- directives are markers on a DOM element (such as an attribute, element name, comment or CSS class) that tell AngularJS's HTML compiler ($compile) to attach a specified behavior to that DOM element or even transform the DOM element and its children.
- Angular comes with built-in directives: ngBind, ngModel, ngClass, etc...
- We can also create custom directives (http://plnkr.co/edit/Vacj6Q6lQcdwqNlnwDYj)
angular.module('moduleName')
.directive('myDirective', function () {
return {
restrict: 'EA', //E = element, A = attribute, C = class, M = comment
link: function ($scope, element, attrs) {
//DOM manipulation
alert("Alert came from myDirective");
}
}
});
Usage:
<my-directive></my-directive>
OR
<div my-directive></div>
Service
- Angular services are objects that are wired together using dependency injection (DI). You can use services to organize and share code across your app.
var myModule = angular.module('myModule', []);
myModule.factory('MyService', function() {
var MyService = {};
// factory function body that constructs MyService
MyService.method1 = function() {
// do something
};
return MyService;
});
Dependency Injection
- Dependency Injection (DI) is a software design pattern that deals with how objects and functions get created and how they get a hold of their dependencies
Dependency Injection
- To use DI, there needs to be a place where all the things that should work together are registered. In Angular, this is the purpose of the so-called "modules".
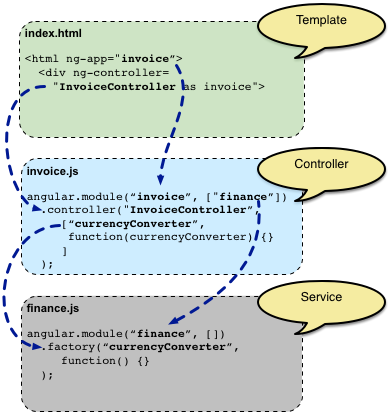
AngularJS AJAX
- The $http service is the easiest way to send AJAX calls to your web server
The $http service has several functions you can use to send AJAX requests. These are:
- $http.get(url, config)
- $http.post(url, data, config)
- $http.put(url, data, config)
- $http.delete(url, config)
- $http.head(url, config)
// EXAMPLE
angular.module("myapp", [])
.controller("MyController", function($scope, $http) {
$scope.albums = [];
$http.get('/albums')
.success(function(response, status, headers, config) {
$scope.albums = response.data;
})
.error(function(response, status, headers, config) {
alert("Failed");
});
} );
AngularJS AJAX
- Angular comes with another very handy optional service called the $resource service
- This service creates a resource object that allows us to intelligently work with RESTful server-side data sources
// USAGE
$resource(url, [paramDefaults], [actions], options);
AngularJS Routes
- AngularJS routes enable you to create different URLs for different content in your application.
<!DOCTYPE html>
<html lang="en">
<head>
<title>AngularJS Routes example</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.5/angular.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.5/angular-route.min.js"></script>
</head>
<body ng-app="sampleApp">
<a href="#/route1">Route 1</a><br/>
<a href="#/route2">Route 2</a><br/>
<div ng-view></div>
<script>
var module = angular.module("sampleApp", ['ngRoute']);
module.config(['$routeProvider',
function($routeProvider) {
$routeProvider.
when('/route1', {
templateUrl: 'angular-route-template-1.jsp',
controller: 'RouteController'
}).
when('/route2', {
templateUrl: 'angular-route-template-2.jsp',
controller: 'RouteController'
}).
otherwise({
redirectTo: '/'
});
}]);
module.controller("RouteController", function($scope) {
})
</script>
Testing Angular application
-
Karma
- http://karma-runner.github.io/0.12/index.html
- Spectacular Test Runner for JavaScript
-
Jasmine
- http://jasmine.github.io/1.3/introduction.html
- Jasmine is a behavior-driven development framework for testing JavaScript code.
- It does not depend on any other JavaScript frameworks.
- It does not require a DOM.
-
angular-mocks
- Angular also provides the ngMock module, which provides mocking for your tests. This is used to inject and mock Angular services within unit tests.
Sample Unit Tests : https://docs.angularjs.org/guide/unit-testing
Other useful tools...
-
Bower
- http://bower.io/
- used to install dependencies
-
Grunt
- http://gruntjs.com/
- JavaScript task runner
Resources:
- Writing AngularJS Directives
- AngularJS tutorials to help you get started
- AngularJS website
Single-Page Application with AngularJS
By pgamilde
Single-Page Application with AngularJS
- 1,297